Python Dictionaries

3 min read
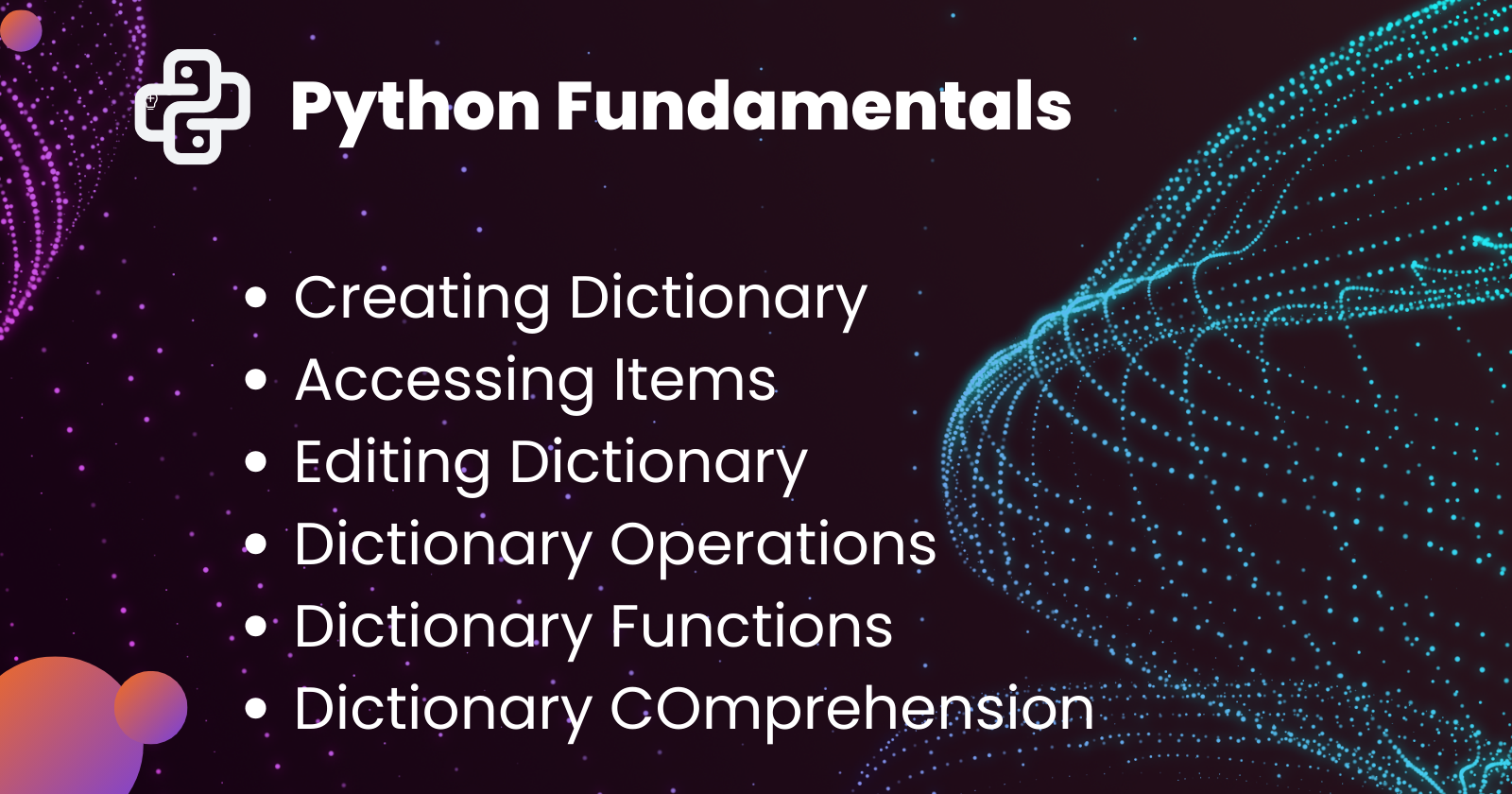
Dictionaries in Python is a collection that is used to store values in key: value pairs. A dictionary is ordered, changeable and does not allow duplicates. The values in dictionary items can be of any data type.
Create Dictionary:
# empty dictionary
this_dict = {}
# 1D dictionary
this_dict = {"brand" : "tesla",
"year" : 1965,
"color" : ['red', 'blue', 'yellow'],
(1,2,3) : 123 }
print(this_dict)
# Output: {'brand': 'tesla', 'year': 1965, 'color': ['red', 'blue', 'yellow'], (1, 2, 3): 123}
Accessing Items
We can access items from the dictionary using corresponding keys.
dict = {'name' : 'Maham', 'age' : 25}
# using key ---> Output: Maham
dict['name']
# using get function ---> Output: Maham
dict.get('name')
Adding & Removing key-value pairs
Dictionaries are mutable, we can add new key-value pairs and remove the existing ones.
dict = {'name' : 'Maham', 'age' : 25}
# Syntax: name[key] = value
dict['gender'] = 'female'
print(dict)
# Output: {'name': 'Maham', 'age': 25, 'gender': 'female'}
dict = {'name': 'Maham', 'age': 25, 'gender': 'female'}
# pop(key) ---> Output: {'age': 25, 'gender': 'female'}
dict.pop('name')
print(dict)
# using popitem : deletes the last key-value pair
dict.popitem()
print(dict) # Output: {'age': 25}
dict = {'name': 'Maham', 'age': 25, 'gender': 'female'}
# using del ---> Output: {'age': 25, 'gender': 'female'}
del dict['name']
print(dict)
# using clear() ---> This will clear the entire dictionary and returns empyty
dict.clear()
print(dict) # Output: {}
Dictionary Operations
In dictionaries, we can perform operations using the keys.
dict = {'name': 'Maham', 'age': 25, 'gender': 'female'}
# Membership
'name' in dict
# Output: True
# Iteration
for i in dict:
print(i, dict[i])
# Output :
# name Maham
# age 25
# gender female
Dictionary functions
dict = {'name': 'Maham', 'age': 25, 'gender': 'female'}
# len --> Gives the number of key-values pairs
len(dict) # 3
# sorted() ---> sorts the keys
sorted(dict) # Output: ['age', 'gender', 'name']
# items() --> displays the list of key-value pairs in the form of tuples
print(dict.items()) # Output: dict_items([('name', 'Maham'), ('age', 25), ('gender', 'female')])
# keys() ---> displays the list of keys
print(dict.keys()) # Output: dict_keys(['name', 'age', 'gender'])
# values()---> displays the list of all the values of the dictionary
print(dict.values) # Output: dict_values(['Maham', 25, 'female'])
# update() ---> update the dictionary with given dictionary
d = {'name': 'Sana', 'weight': 56}
dict.update(d)
print(dict) # Output:{'name': 'Sana', 'age': 25, 'gender': 'female', 'weight': 56}
Dictionary comprehension
Syntax:
{ key: value for vars in iterable}
# Create a dictionary whose key are the numbers from 1 to 10 and values are their sqaures
{i:i**2 for i in range(1,11)}
# Output:
# {1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81, 10: 100}
# Create a new dictionary using zip()
days = ["Sunday", "Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"]
temps = [30.5,32.6,31.8,33.4,29.8,30.2,29.9]
dict = {i:j for (i,j) in zip(days,temps)}
print(dict)
# Output:
# {'Sunday': 30.5, 'Monday': 32.6, 'Tuesday': 31.8, 'Wednesday': 33.4, 'Thursday': 29.8, 'Friday': 30.2, 'Saturday': 29.9}
# Dictionary comprehension using if condition
products = {'phone':10,'laptop':0,'charger':32,'tablet':0}
# Create a dictionary containing non-zero values
dict = {key: value for (key,value) in products.items() if value >0}
print(dict)
# Output: {'phone': 10, 'charger': 32}
# Nested Dictionary
# print tables from 2 to 4 ---> {2 : {1:2, 2:4......}}
dict = {i:{j:i*j for j in range(1,11)} for i in range(2,5)}
print(dict)
# Output:
# {2: {1: 2, 2: 4, 3: 6, 4: 8, 5: 10, 6: 12, 7: 14, 8: 16, 9: 18, 10: 20},
# 3: {1: 3, 2: 6, 3: 9, 4: 12, 5: 15, 6: 18, 7: 21, 8: 24, 9: 27, 10: 30},
# 4: {1: 4, 2: 8, 3: 12, 4: 16, 5: 20, 6: 24, 7: 28, 8: 32, 9: 36, 10: 40}}
0
Subscribe to my newsletter
Read articles from maham tariq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
