Arrays Easy - Part III (XOR)
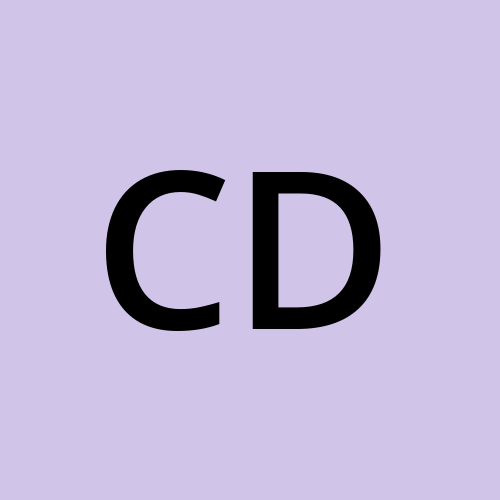
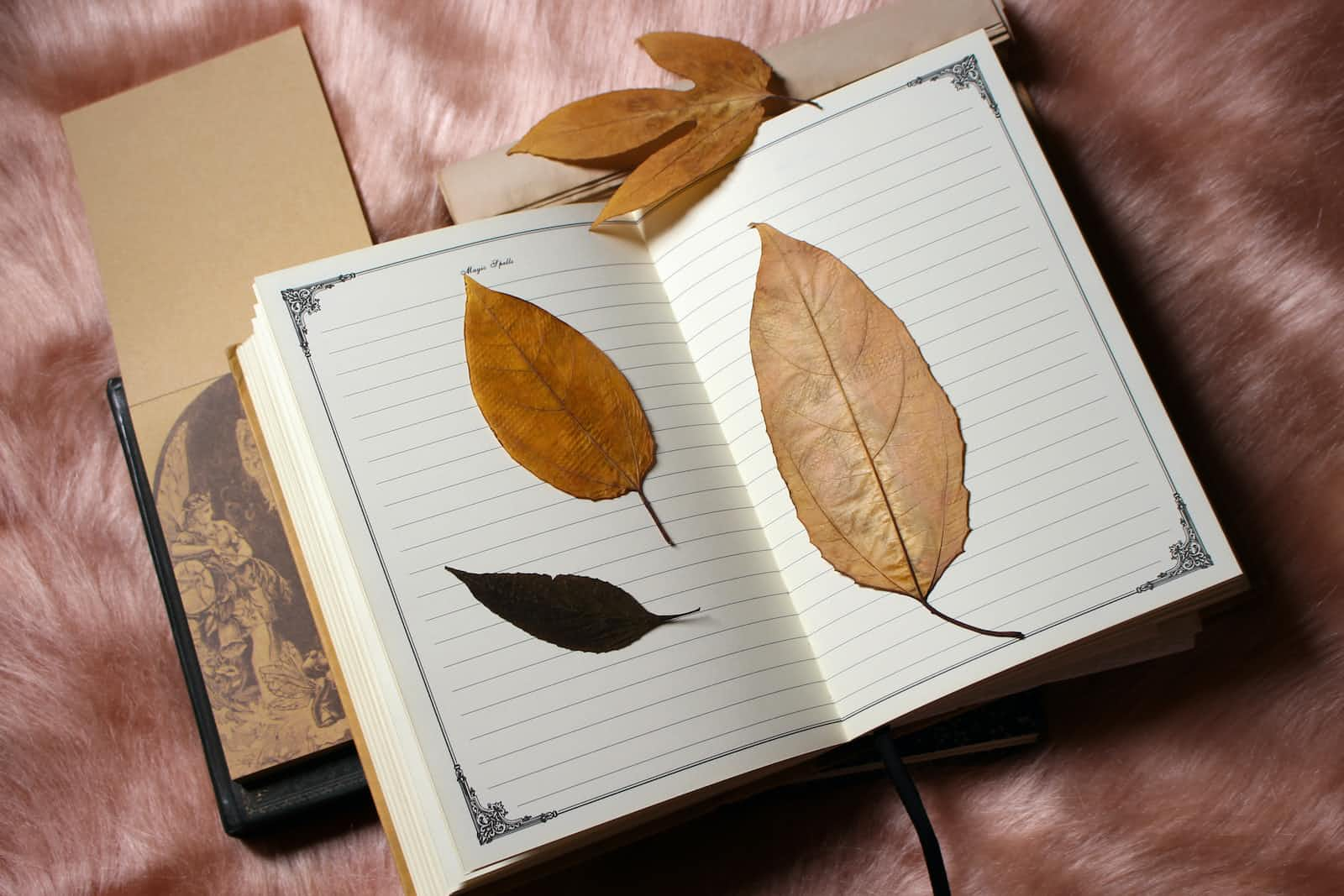
268. Missing Number
Problem Statement
Given an array nums
containing n
distinct numbers in the range [0, n]
, return the only number in the range that is missing from the array.
(link)
Example 1:
Input: nums = [3,0,1]
Output: 2
Explanation: n = 3 since there are 3 numbers, so all numbers are in the range [0,3].
2 is the missing number in the range since it does not appear in nums.
Optimal Approach
N-Natural Numbers sum Approach
Calculate the expected sum by directly using the formula n*(n+1)/2
. If we subtract this from the actual or current sum, we get the missing element.
class Solution {
public int missingNumber(int[] nums) {
int n = nums.length;
int expectedSum = (n * (n+1)) >> 1;
int actualSum = 0;
for(int element: nums){
actualSum+=element;
}
return expectedSum - actualSum;
}
}
XOR Approach
a ^ a = 0
The same element XOR is 0. First, we find out the XOR of a given array, and then we find out the XOR of the actual elements. If we XOR xor1 (given numbers) and xor2 (expected numbers), it will give the missing number.
class Solution {
public int missingNumber(int[] nums) {
int xor1 = 0;
int xor2 = 0;
int n = nums.length;
for(int i=0; i<n; i++){
xor1 = xor1 ^ nums[i];
xor2 = xor2 ^(i+1);
}
return xor1 ^ xor2;
}
}
485. Max Consecutive Ones
Problem Statement
Given a binary array nums
, return the maximum number of consecutive 1
's in the array.
(link)
Example 1:
Input: nums = [1,1,0,1,1,1]
Output: 3
Explanation: The first two digits or the last three digits are consecutive 1s.
The maximum number of consecutive 1s is 3.
Optimal Approach
Take two counters. One counter will keep track of the maximum consecutive ones observed, while the second counter (current counter) will count the current consecutive ones. Note that the current counter will be reset to zero if it observes a 0, as this breaks the consecutive 1 chain.
class Solution {
public int findMaxConsecutiveOnes(int[] nums) {
int ans = 0;
int count = 0;
for(int element: nums){
if(element==1){
count+=1;
ans = Math.max(ans, count);
}
else{
count = 0;
}
}
return ans;
}
}
136. Single Number
Given a non-empty array of integers nums
, every element appears twice except for one. Find that single one. (link)
Example 1:
Input: nums = [2,2,1]
Output: 1
Brute Force Approach (Extra Space)
Count the frequency of each element and store the results in a map, where the keys represent the elements from the array, and the values indicate the number of times each element is repeated. Later, iterate through the map to identify the element with a frequency of 1, and return its key
class Solution {
public int singleNumber(int[] nums) {
Map<Integer, Integer> dict = new HashMap<>();
for(int element: nums){
if(!dict.containsKey(element)){
dict.put(element, 0);
}
dict.put(element, dict.get(element)+1);
}
for(Map.Entry<Integer, Integer> entry: dict.entrySet()){
if(entry.getValue()==1) return entry.getKey();
}
return -1;
}
}
Optimal Approach (XOR)
Since each element repeats twice except for one, if we perform the XOR operation on the full array, we will be left with a number that doesn't have any duplicates.
class Solution {
public int singleNumber(int[] nums) {
int xor = 0;
for(int element: nums){
xor = xor ^ element;
}
return xor;
}
}
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
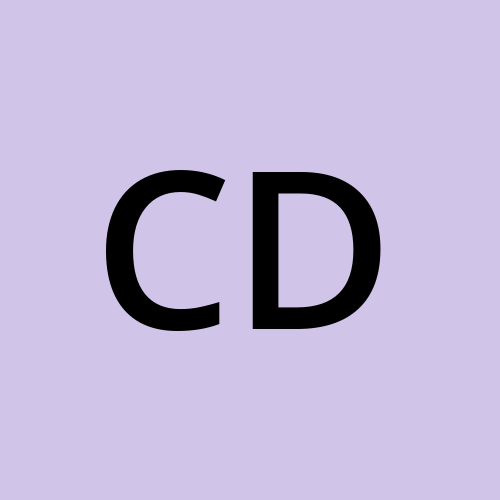
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.