How to write JavaScript indexOf and lastIndexOf array methods from scratch
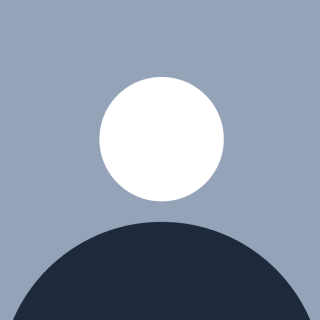
1 min read
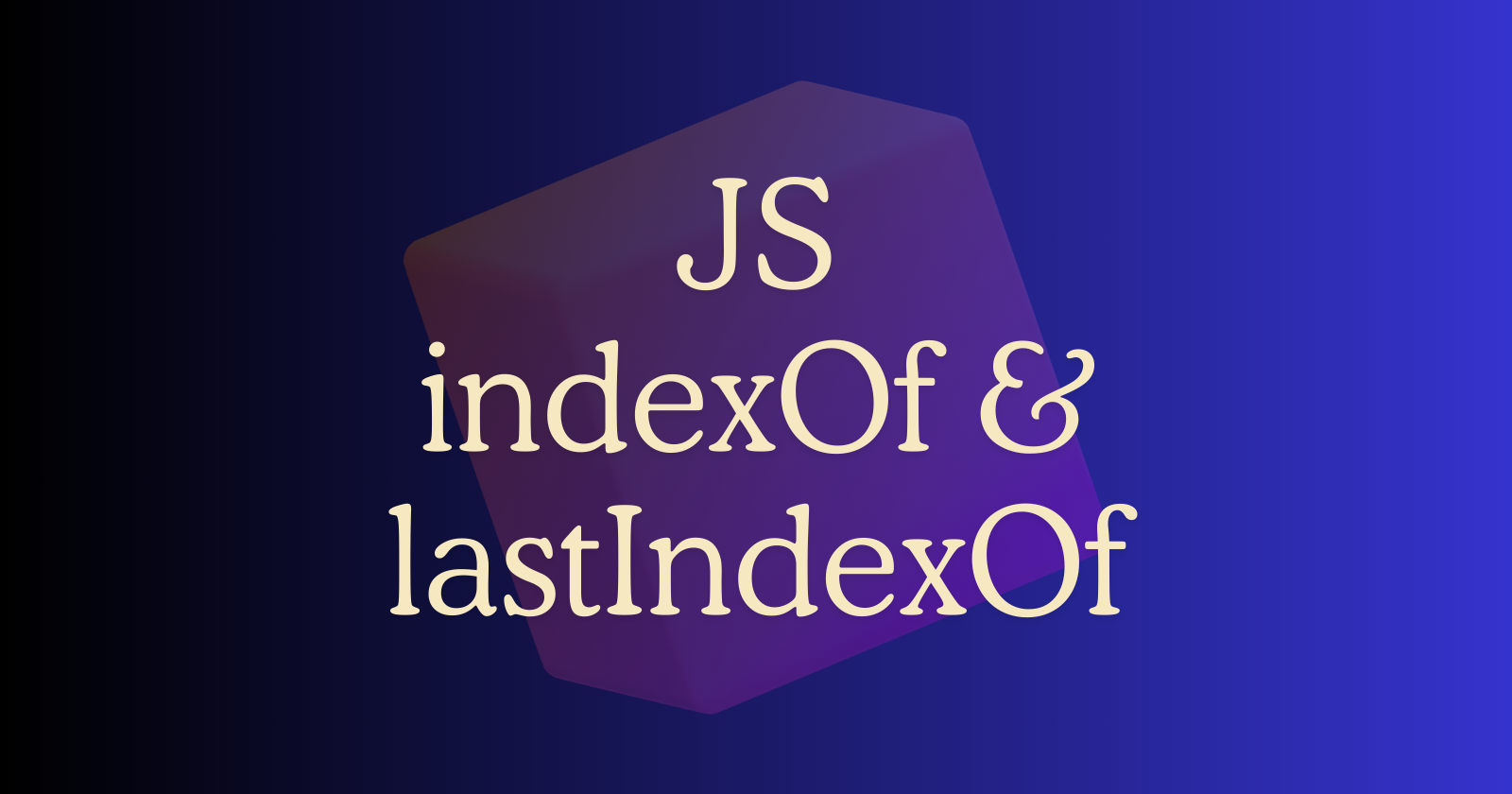
indexOf
Returns first index of the found element otherwise -1.
Array.prototype._indexOf = function(searchElement, fromIndex = 0) {
for(let i = fromIndex; i < this.length; i++) {
if(searchElement === this[i]) {
return i
}
}
return -1
}
const fruits = [ 'apple', 'mango', 'banana', 'mango', 'orange' ]
console.log(fruits._indexOf('mango'))
// Output
// 1
function accepts two parameters
searchElement
and optionalfromIndex
if found return index otherwise return -1
lastIndexOf
Returns the lastIndex if elements are found otherwise returns -1.
Array.prototype._lastIndexOf = function(searchElement, fromIndex = 0) {
let index = -1;
for(let i = fromIndex; i < this.length; i++) {
if(searchElement === this[i]) {
index = i
}
}
return index
}
const fruits = [ 'apple', 'mango', 'banana', 'mango', 'orange' ]
console.log(fruits._lastIndexOf('mango'))
// Output
// 3
- here only difference in code is we store the index whenever we get a match and then return after the loop is complete.
0
Subscribe to my newsletter
Read articles from DevBitByte directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by