Push and update object using destructuring
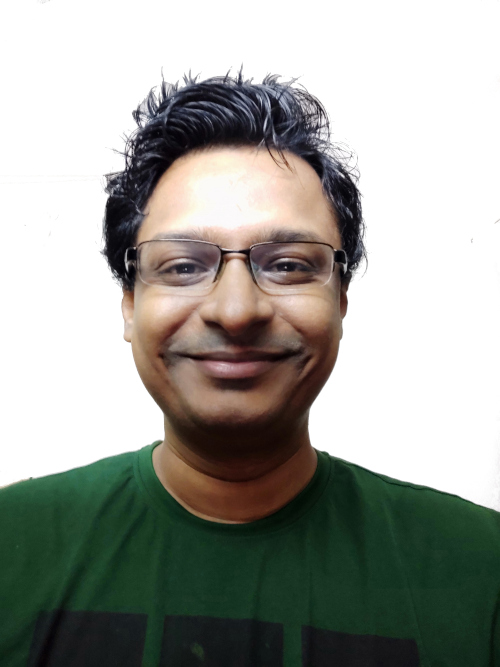
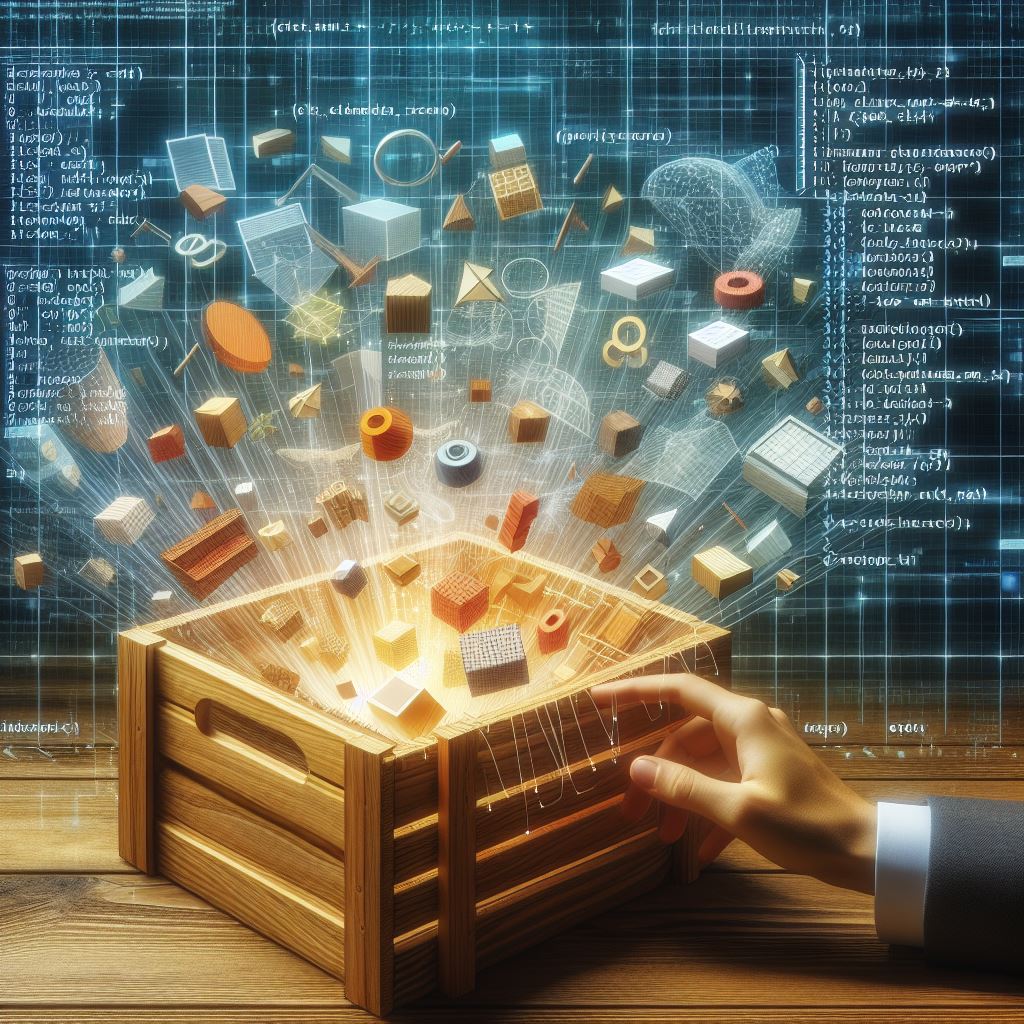
If you have an object like this :
const default_object = {
vehicle: 'car',
color: 'red',
make: 'Honda',
};
and want to update the color
then we would simply do default_object.color = 'green'
Let's say we have an array of objects like this :
const objects = [];
and we push as in objects.push(default_object);
But what if you wanted to add the default_object
and at the same time update the color
of the newly pushed object? This is what is done to update the color
attribute.
objects.push({ ...default_object, color: 'green' });
This is equivalent to this writing like this :
objects.push({ vehicle: 'car', color: 'red', make: 'Honda', color: 'green' });
Adding color
attribute twice only overwrites the first added color
. So green gets assigned to the color
value.
In objects.push({ ...default_object, color: 'green' });
, ...default_object
is destructured, meaning it's "expanded" to vehicle: 'car', color: 'red', make: 'Honda' and then color: 'green' is added to the object overwriting color: 'red' in the expansion.
const objects = [];
const default_object = {
vehicle: 'car',
color: 'red',
make: 'Honda',
};
console.log(objects);
objects.push(default_object);
console.log(objects);
objects.push({ vehicle: 'car', color: 'red', make: 'Honda', color: 'green' });
objects.push({ ...default_object, color: 'blue' });
console.log(objects);
Subscribe to my newsletter
Read articles from Anjanesh Lekshminarayanan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
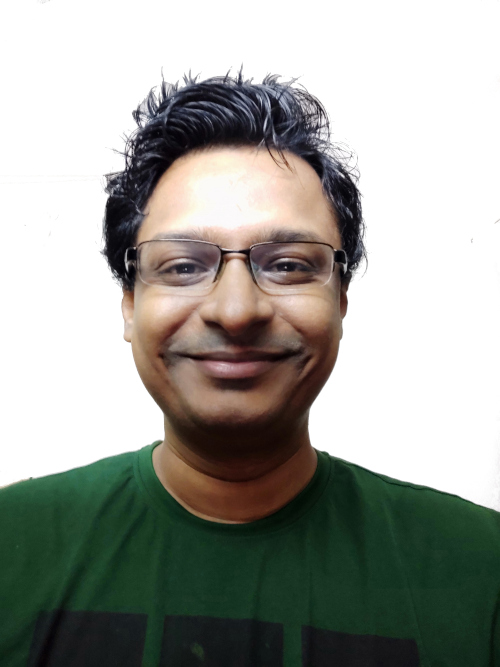
Anjanesh Lekshminarayanan
Anjanesh Lekshminarayanan
I am a web developer from Navi Mumbai working as a consultant for cloudxchange.io. Mainly dealt with LAMP stack, now into Django and trying to learn Laravel and Google Cloud. TensorFlow in the near future. Founder of nerul.in