Argon Hashing Algorithm vs. Bcrypt: A Laravel Developer’s Guide
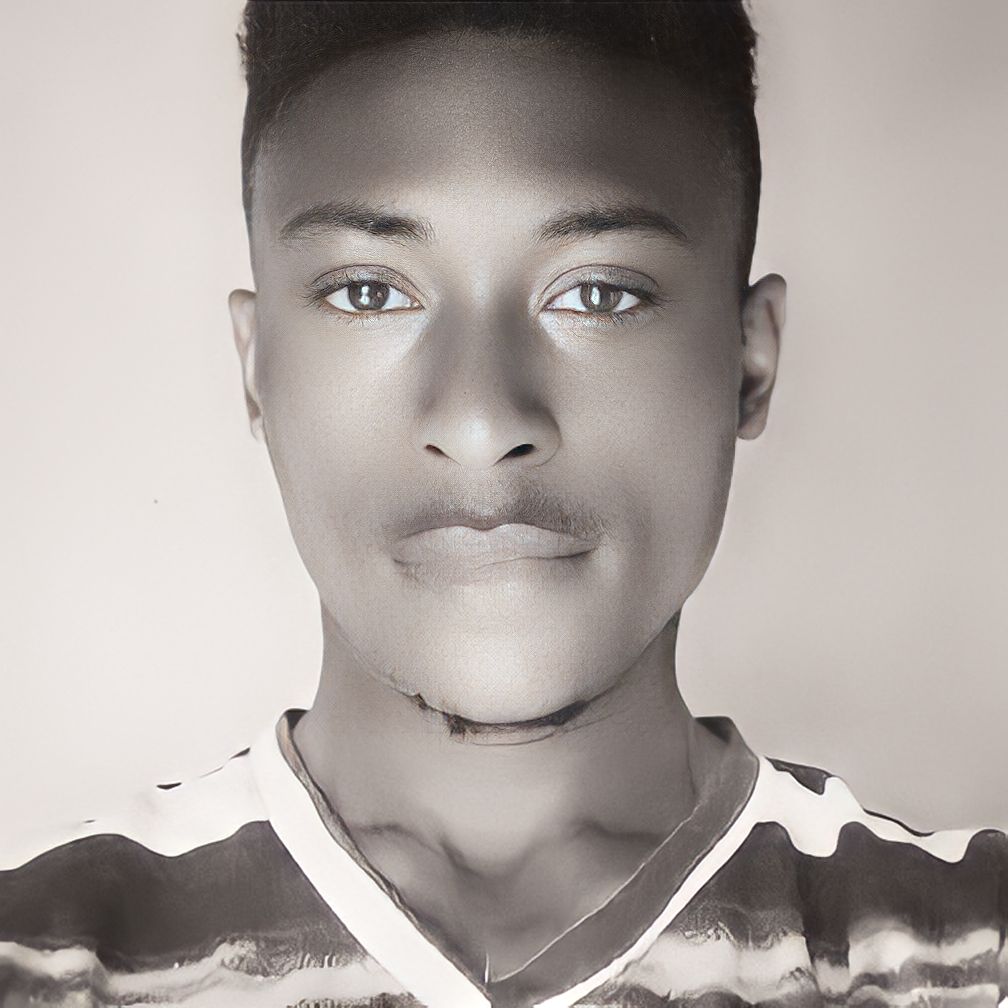
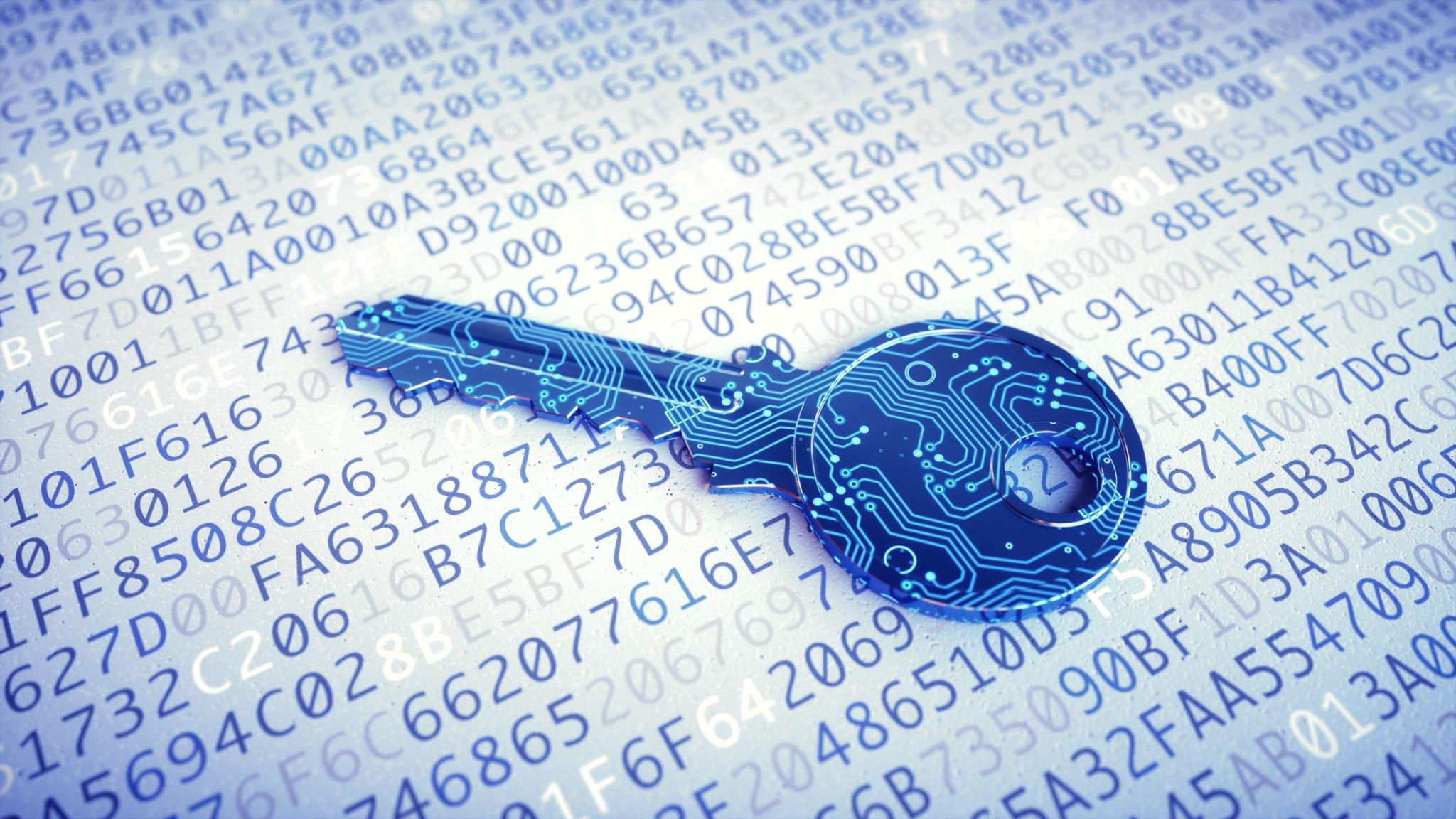
Hey Laravel Enthusiasts! 🌐🔐
In the world of web security, hashing algorithms are unsung heroes, and today we’re pitting two heavyweights against each other: Argon2 and bcrypt. While both bring their A-game to security, let’s dissect their strengths and quirks, especially from a Laravel framework perspective.
Understanding Argon2 and Bcrypt
The Argon2 Algorithm
1. Memory-Hard Approach: Argon2 thrives on being memory-intensive. This feature makes it harder for attackers to use parallel processing to crack passwords.
2. Flexible Parameters: It allows tweaking of memory usage, time cost, and parallelism. This level of customization is a boon for tailor-made security strategies.
The Bcrypt Algorithm
1. Time-Tested Reliability: Bcrypt has been a go-to for years, known for its reliability and simplicity.
2. Moderate Resource Requirement: It doesn’t require as much memory as Argon2, making it a more lightweight option.
The Trade-Offs
1. Performance vs. Security
— Argon2: More secure but demands more resources. It’s excellent for offline key derivation but can be overkill for some web applications.
— Bcrypt: Offers a balanced approach. It’s less resource-intensive, making it a suitable choice for web apps where response time is critical.
2. Customization
— Argon2: Wins in flexibility. The ability to adjust memory, CPU, and thread usage makes it adaptable to various scenarios.
— Bcrypt: Lacks this level of customization, sticking to a one-size-fits-all approach.
3. Resistance to Attacks
— Argon2: Better equipped to handle GPU-based attacks due to its memory-hard nature.
— Bcrypt: Provides moderate resistance but falls short compared to Argon2.
Integrating with Laravel
Sure, let’s delve into more details on setting up both Argon2 and bcrypt in Laravel. Laravel’s flexibility makes it relatively straightforward to switch between these hashing algorithms.
Setting Up Argon2
- Update .env
and configure Argon parameters in config/hashing.php
.
- Use Laravel’s Hash facade for hashing and verification.
1. Update .env
File
First, specify Argon2 as your default hashing driver in the .env
file:
HASH_DRIVER=argon2
This change tells Laravel to use Argon2 for all hashing operations.
2. Configure Argon Parameters
Laravel allows you to customize the Argon2 parameters in the config/hashing.php
file. Here’s how you can do it:
'argon' => [
'memory' => 1024, // Amount of memory allocated (in kilobytes)
'threads' => 2, // Number of threads to use
'time' => 2, // Amount of time it should take
],
These settings determine the computational cost of the hashing process. Adjust them based on your application’s security needs and server capabilities.
3. Using Laravel’s Hash Facade
With Argon2 set as the default, use Laravel’s Hash facade for hashing and password verification. Here’s an example:
Hashing a Password:
use Illuminate\Support\Facades\Hash;
$passwordHash = Hash::make('your_plain_text_password');
Verifying a Password:
if (Hash::check('plain_text_password', $hashedPassword)) {
// Password is correct.
}
Setting Up Bcrypt
- Set Bcrypt as your default in .env
.
- Laravel’s Hash facade seamlessly integrates with Bcrypt as well.
- Update
.env
File
To use bcrypt, update the.env
file as follows:
HASH_DRIVER=bcrypt
This sets bcrypt as the default hashing algorithm.
2. Bcrypt Configuration
Unlike Argon2, Bcrypt has fewer configuration options. You can set the cost (or computational complexity) in config/hashing.php
:
'bcrypt' => [
'rounds' => 10, // Higher means more computationally expensive
'verify' => true, // Determines if password entered is being verified on entry
],
Adjust the rounds
parameter to increase or decrease the hashing time. A higher number means a more secure hash but takes longer to compute.
3. Using Laravel’s Hash Facade
With bcrypt as the default, the Hash facade works similarly:
Hashing a Password:
$passwordHash = Hash::make('your_plain_text_password');
Verifying a Password:
if (Hash::check('plain_text_password', $hashedPassword)) {
// Password is correct.
}
Conclusion: Which One to Choose?
In the Laravel universe, the choice between Argon2 and Bcrypt isn’t black and white. Consider these factors:
- Application Type: If your app deals with highly sensitive data and can afford slightly longer response times, Argon2’s robust security model is a clear winner. For general web applications where speed is crucial, Bcrypt’s efficiency might be more appealing.
- Resource Availability: Argon2 is more resource-hungry. Ensure your server environment can handle it without compromising performance.
- Future-Proofing: Argon2, with its customizable parameters, might be more future-ready, especially as hardware capabilities evolve.
Points and takeaways 🥡
- Argon2 offers superior security with customizable parameters but is more resource-intensive — ideal for high-security applications.
- Bcrypt is less demanding on resources and offers a quick, reliable solution for general web applications.
- Laravel developers should weigh their app’s security needs against performance requirements when choosing between the two.
So, Laravel devs, whether you go with the sturdy Bcrypt or the versatile Argon2, you’re in good hands. Just align your choice with your application’s needs, and you’ll bolster your app’s defenses like a pro! 🛡️💻🚀
Subscribe to my newsletter
Read articles from Favour Orukpe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
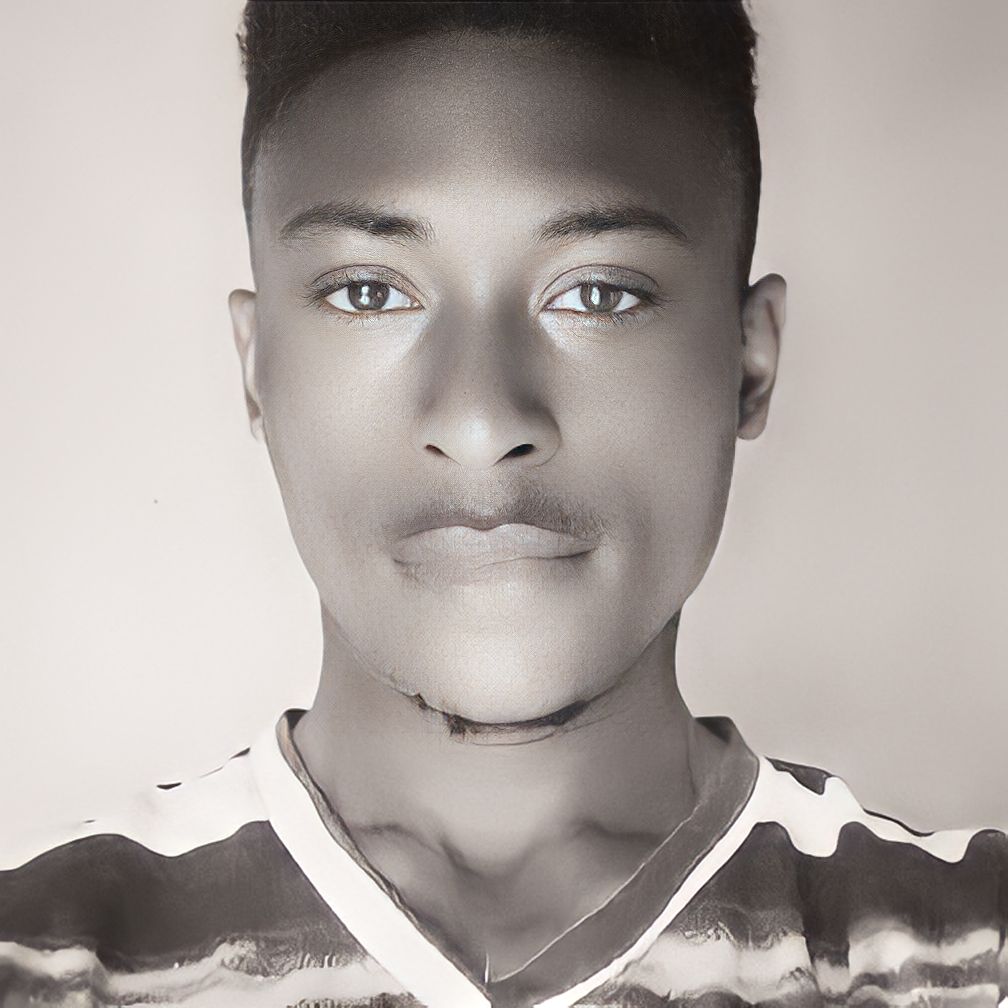
Favour Orukpe
Favour Orukpe
Favour Orukpe is a Full-Stack Software Engineer proficient in React, React Native, TypeScript, PHP, Laravel, and Django. As a key member of the $mart Earners Team™, Favour focuses on innovative solutions. With a passion for seamless user experiences, Favour excels in both frontend and backend development, contributing to diverse projects with efficiency and creativity.