151 DSA Problem journey

2 min read
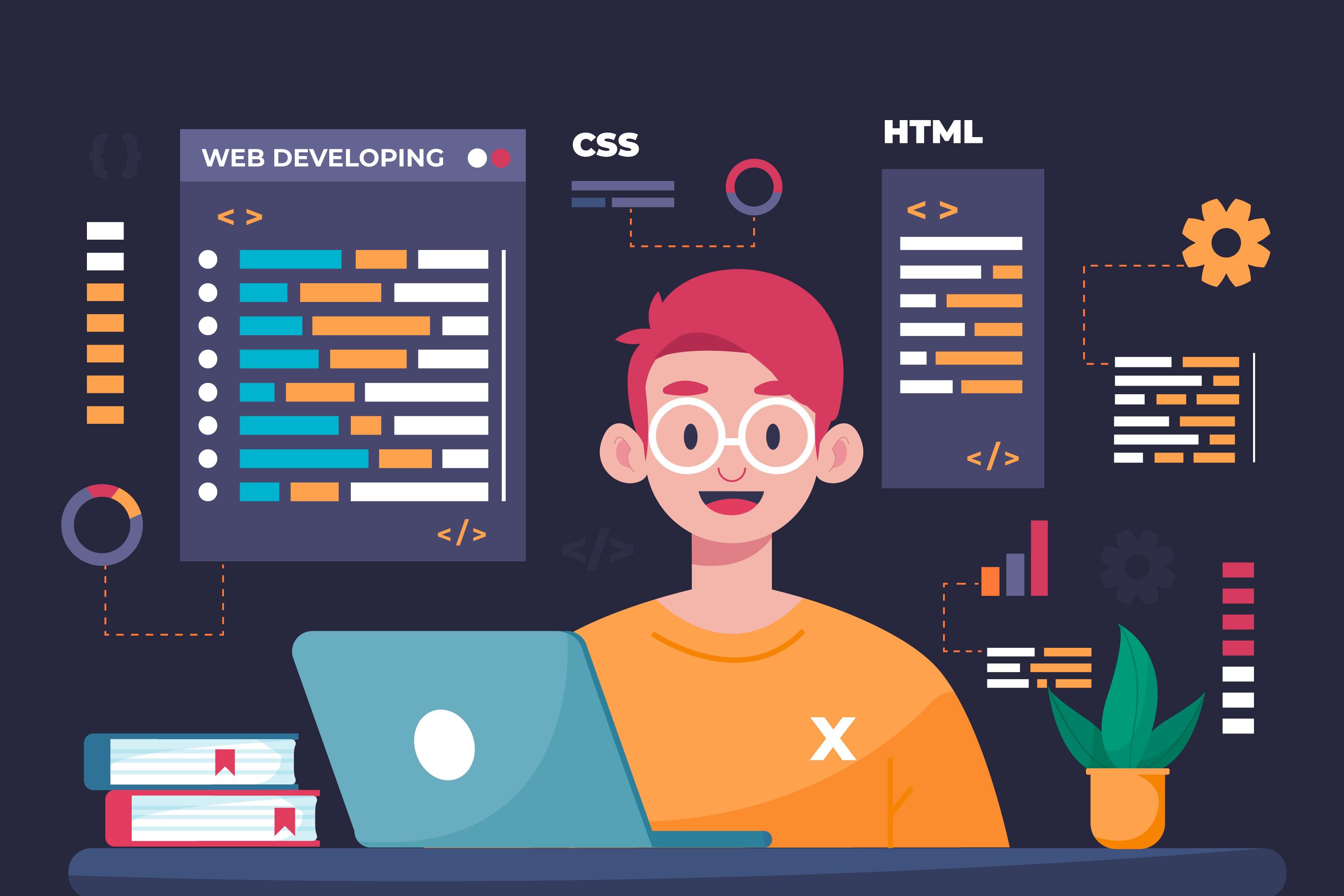
Q5:)Given an array nums
of size n
, return the majority element.The majority element is the element that appears more than ⌊n / 2⌋
times. You may assume that the majority element always exists in the array.
Example:
Input: nums = [3,2,3]
Output: 3
Solution:
class Solution {
public:
int majorityElement(vector<int>& nums) {
int major=nums[0], count = 1;
for(int i=1; i<nums.size();i++){
if(count==0){
count++;
major=nums[i];
}else if(major==nums[i]){
count++;
}else count--;
}
return major;
}
};
Explantion:
.)Initialize major to the first element of the nums vector, and count to 1.
.)Iterate through the elements of the nums vector starting from the second element.
.)If count is zero, reset count to 1, and update major to the current element.
.)If the current element is equal to major, increment count.
.)If the current element is different from major, decrement count.
.)The final value of major is the majority element in the vector.
.)Return the majority element (major).
>>This code efficiently finds the majority element (an element that appears more than n/2 times,
where n is the size of the array) using the Boyer-Moore Voting Algorithm.
It traverses the array, keeping track of a potential majority element and a count.
If the count becomes zero, it switches the potential majority element to the current element.
If the current element is the same as the potential majority element, it increments the count;
otherwise,it decrements the count. The final potential majority element is returned.
11
Subscribe to my newsletter
Read articles from GAURAV YADAV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
C++cppCpp Tutorial DSAcpProgramming Blogsproblem solving skills#codingNewbiescodingcoding challengecoding journeylearningskills
Written by

GAURAV YADAV
GAURAV YADAV
Experienced Full Stack Developer with proficiency in comprehensive coding skills, adept at crafting end-to-end solutions.