Mastering JSON Mapping with data.map() in JavaScript

Table of contents
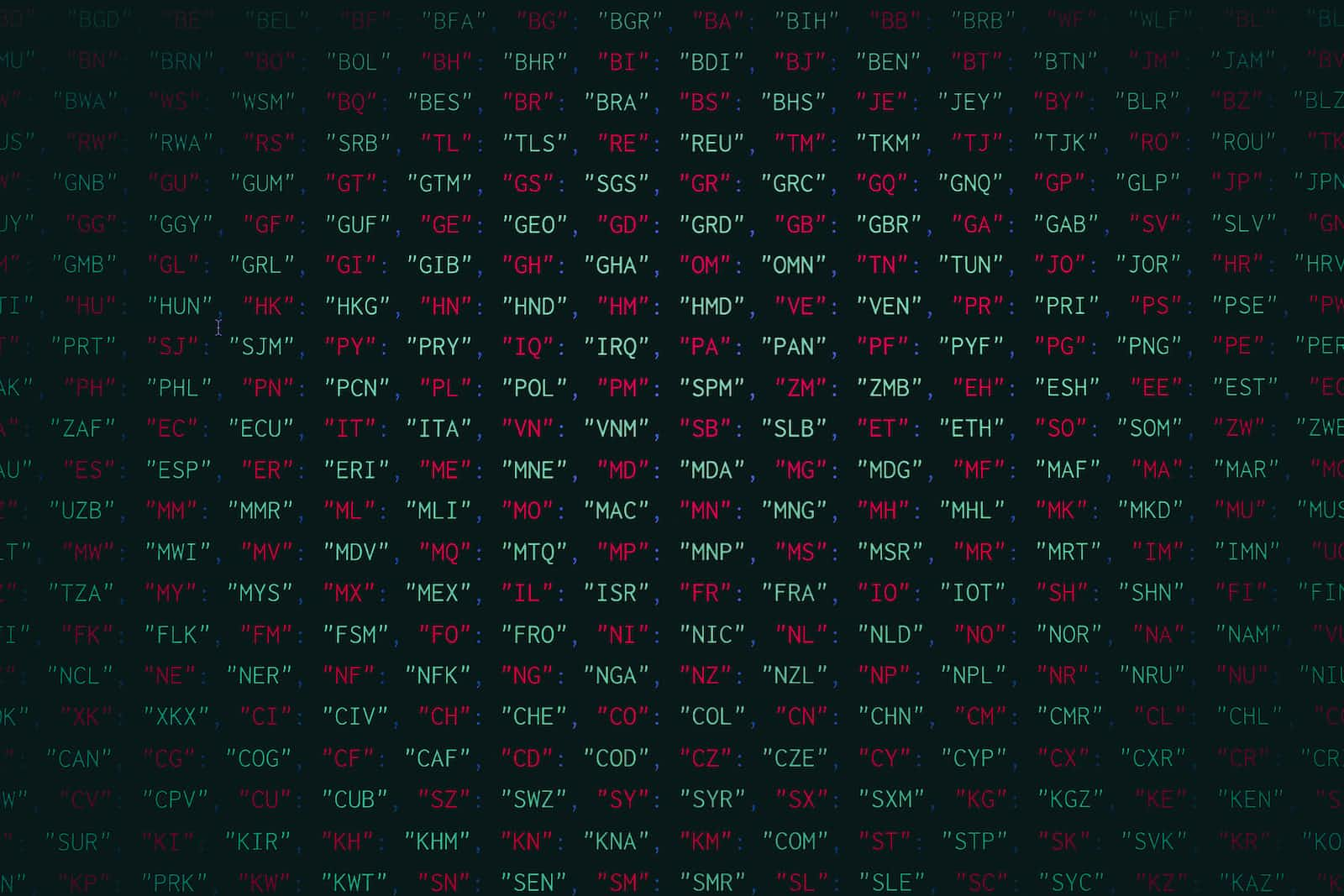
Mapping over JSON data is a common operation in JavaScript, especially when dealing with arrays of objects. The map()
function provides a concise and efficient way to iterate through each element of an array, perform a transformation, and return a new array. Let's explore some best practices and common pitfalls to ensure you're making the most out of this powerful method.
Best Practices for JSON Mapping
1. Use Arrow Functions for Conciseness
const mappedData = jsonData.map(item => item.property);
Arrow functions provide a concise syntax, making your code cleaner and more readable. They are particularly handy when the mapping logic is short.
2. De-structure Object Properties
const mappedData = jsonData.map(({ property }) => property);
If you only need specific properties of each object, use object de-structuring. It enhances code clarity and reduces redundancy.
3. Handle Undefined or Missing Data
const mappedData = jsonData.map(item => item.property || 'Default');
Account for undefined or missing data by using default values. This prevents unexpected errors when dealing with incomplete JSON structures.
4. Ensure Consistency in Return Values
const mappedData = jsonData.map(item => {
return {
label: item.name,
value: item.amount,
};
});
Ensure that your mapping function consistently returns the same structure. This makes downstream processing more predictable.
5. Avoid Modifying Original Data
const mappedData = jsonData.map(item => ({ ...item, newValue: 'New Value' }));
If you need to add or modify properties, create a new object to avoid unintentional modification of the original data.
Common Mistakes to Avoid
1. Not Checking for Valid JSON
Always ensure that your JSON data is valid before attempting to map over it. Invalid JSON can lead to runtime errors.
2. Forgetting the 'key' Parameter
const mappedData = jsonData.map(item => (
<Component key={item.id} data={item} />
));
When rendering components in React (or any other library/framework), don't forget to include a unique 'key' attribute to each element for efficient rendering.
3. Overusing map for Side Effects
jsonData.map(item => console.log(item.property));
Avoid using map
solely for side effects. If you don't need the resulting array, consider using forEach
instead.
In conclusion, mastering data.map
()
is essential for effective data transformation in JavaScript. By following these best practices and avoiding common mistakes, you'll write cleaner and more maintainable code.
Happy Mapping!
Subscribe to my newsletter
Read articles from Mohsin Tahir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
