Unlocking Observables with toSignal in Angular v16

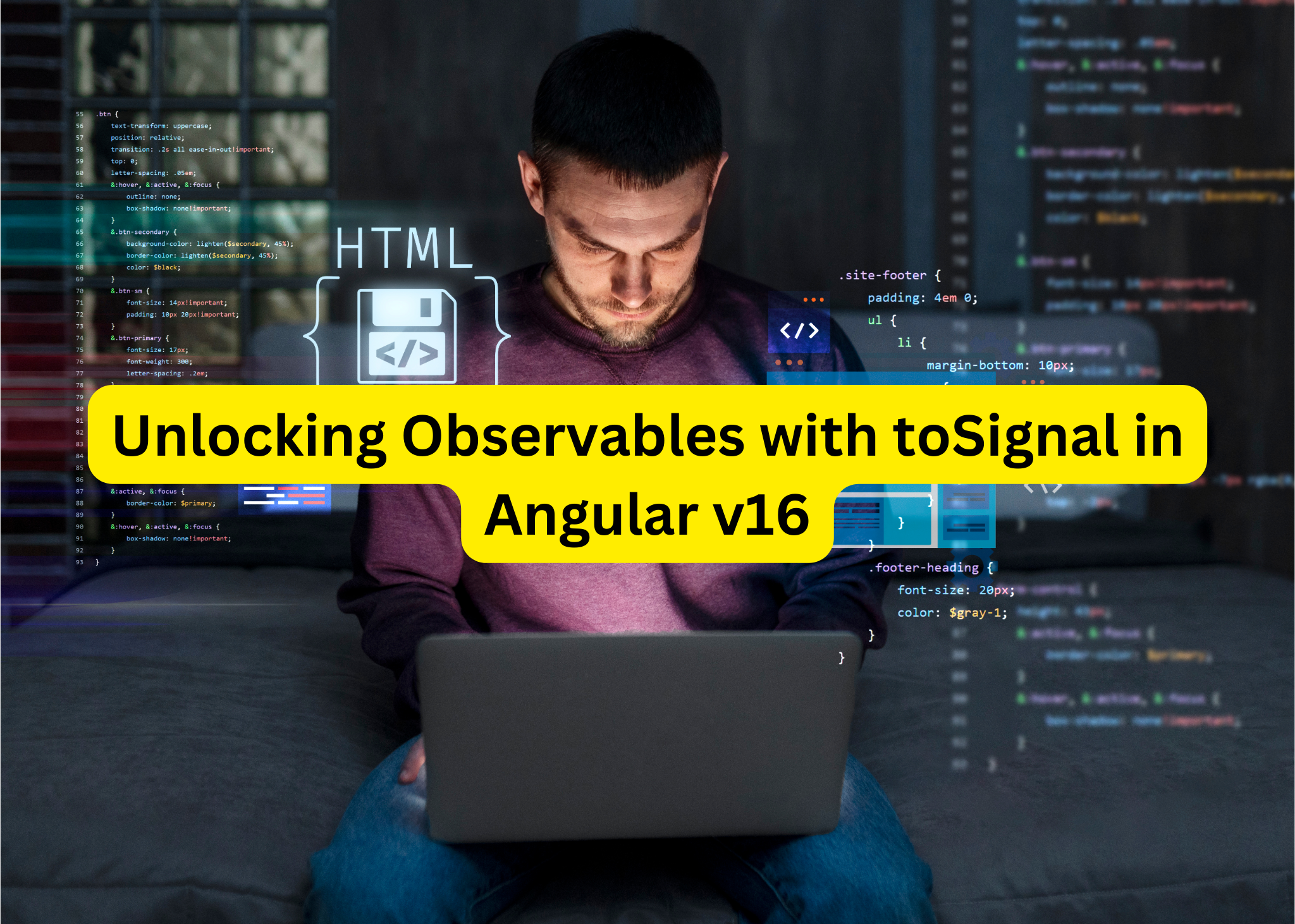
Angular v16 introduces a groundbreaking feature through the rxjs-interop package – the toSignal function. This function takes observables to a new level by converting them into signals. In this article, we'll explore the nuances of this feature and how to wield it effectively in your Angular applications.
Getting Started with toSignal
To leverage the toSignal function, we first need to import it from the @angular/core/rxjs-interop module. Let's dive into a practical example to showcase its usage:
typescript
import { toSignal } from '@angular/core/rxjs-interop';
import { interval } from 'rxjs';
@Component({
standalone: true,
template: {{ counter() }},
})
export class FooComponent {
counter$ = interval(1000);
counter = toSignal(this.counter$);
}
In this example, we create an observable using an interval with a one-second period. The toSignal function then transforms this observable into a signal. The resulting signal has the type Signal<number | undefined, indicating that it can produce undefined values due to the absence of an initial value for the observable.
Immediate Access and Subscription Considerations
A notable difference from the async pipe is that we can immediately read the value of the signal in our component, even if it produces undefined. However, caution is advised, as the toSignal function subscribes to the observable immediately, potentially leading to unintended consequences with side effects.
Contrastingly, when using the async pipe with an ngIf directive, subscription to the observable only occurs when rendering the template. If transitioning to toSignal, it subscribes immediately, irrespective of the ngIf condition's result.
Managing Undefined Values
To address the issue of undefined values in the resulting signal, two options are available:
Passing an Initial Value:
typescript
counter$ = interval(1000);
counter = toSignal(this.counter$, { initialValue: 0 });
Using requireSync Option:
typescript
counter$ = interval(1000).pipe(startWith(0));
counter = toSignal(this.counter$, { requireSync: true });
Note: If the observable doesn't emit immediately, using requireSync: true will throw an error in Angular.
Contextual Usage and Auto-Unsubscription
Angular enforces a context check when toSignal is called, ensuring it's within an injection context. This check prevents errors related to auto-unsubscription when the wrapping context is destroyed. The inject() function must be available, except when using the manualCleanup option or providing an injector explicitly.
Persistent Subscriptions and Cleanup Options
If the subscription should persist until the observable completes, the manualCleanup option can be specified:
typescript
counter$ = interval(1000).pipe(take(3));
counter = toSignal(this.counter$, { manualCleanup: true });
Error Handling with toSignal
Handling errors with the toSignal function is straightforward. When the observable emits an error, Angular throws it, and developers can use a try-catch block for handling:
typescript
counter$ = interval(1000);
counter = toSignal(this.counter$, { initialValue: 0 });
ngOnInit() {
try {
this.counter();
} catch (e) {
console.log(e);
}
}
Conclusion
In conclusion, the rxjs-interop package in Angular v16 introduces the toSignal function, a powerful tool for converting observables into signals. While its usage is straightforward, developers must exercise caution to avoid unexpected outcomes. By understanding the considerations mentioned in this article, you can harness the full potential of toSignal in Angular v16 and enhance your reactive programming experience.
To explore more innovative solutions, consider partnering with Bacha Software, where our experienced Dedicated Development Team stands ready to transform your ideas into reality.
Subscribe to my newsletter
Read articles from Phạm Vân Anh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Phạm Vân Anh
Phạm Vân Anh
Currently I am working as Marketing Executive at Bac Ha Software - Leading software development company in Vietnam https://bachasoftware.com/