All about Numbers: Their Properties and Methods in JavaScript
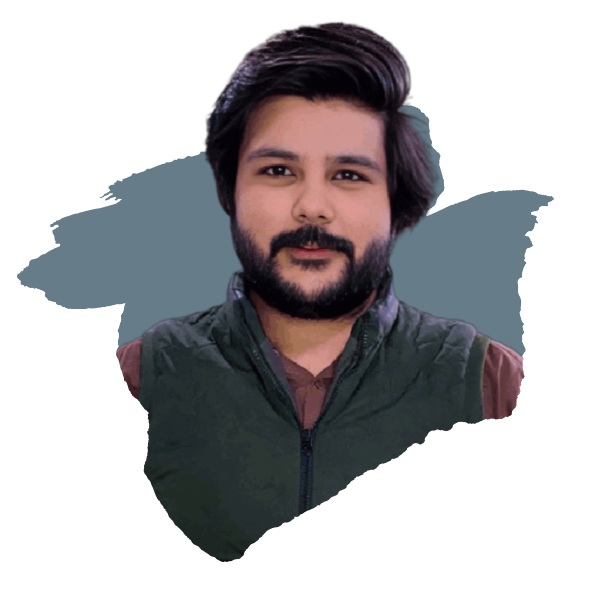
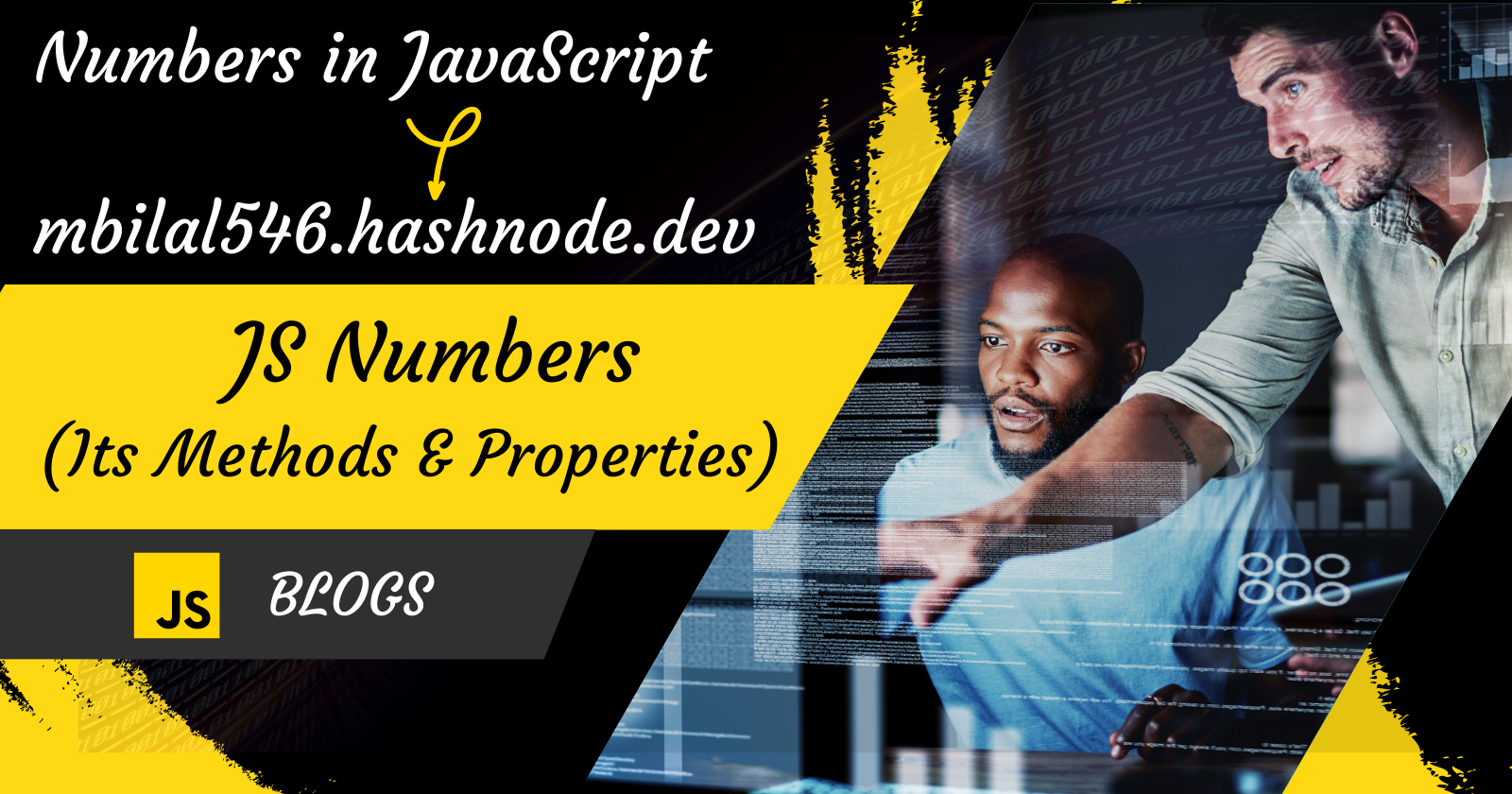
JavaScript Numbers: In Depth
In JavaScript, numbers are fundamental data types used to represent numerical values. They can be integers, floating-point numbers, or exponential notation.
Integers:
They should be positive, negative, or zero.
For Example:
let integer1 = 10;
let integer2 = -5;
let integer3 = 0;
Floating-Point Numbers:
Numbers with decimal points are known as floating-point numbers.
For Example:
let floatingNumber1 = 2.23;
let floatingNumber2 = -0.36;
Exponential Notation (Scientific Notation):
Larger numbers are expressed using scientific notation.
For Example:
let integer1 = 20000000000; // integer form
let expNotation2 = 2e10; // Scientific Notation form
// Here integer1 and expNotation2 are same
let expNotation3 = 2e-7;
Number Object:
We rarely use number objects. It specifically displays its type, which is a number. You can see this clearly in the given example.
For Example:
let number1 = 10;
console.log(number1);
Output: 10 // Here JavaScript automatically consider its type as number
let numberObj = new Number(10);
console.log(numberObj);
Output: [Number: 10] // Here JavaScript display its type as number
Uses of numbers:
- Numbers can be used for basic arithmetic operations such as addition, subtraction, multiplication, division, etc.
For Example:
// Addition
let integer1 = 10;
let integer2 = 5;
console.log(integer1 + integer2);
Output: 15
// Subtraction
let integer1 = 10;
let integer2 = 5;
console.log(integer1 - integer2);
Output: 5
// Multiplication
let integer1 = 10;
let integer2 = 5;
console.log(integer1 * integer2);
Output: 50
// Division
let integer1 = 10;
let integer2 = 5;
console.log(integer1 / integer2);
Output: 2
- Numbers can be used for comparison, such as less than or greater than.
For Example:
// Less Than
let integer1 = 10;
let integer2 = 5;
console.log(integer1 < integer2);
Output: false
// Greater Than
let integer1 = 10;
let integer2 = 5;
console.log(integer1 > integer2);
Output: true
Number Methods in JavaScript:
JavaScript has some useful methods for manipulating and working with numbers.
1. toString():
ThetoString
method is one of the most common methods available on the Number
object in JavaScript. It allows you to convert a number value to a string representation.
let num = 150; // Here it is a integer
let numStr = num.toString(); // Now it become string & store in numStr
console.log(numStr);
Output: 150 // It is looking like a number but when you check its type, so
let checkType = typeof(numStr);
console.log(checkType);
Output: string
// typeof() operator
typeof() operator is used for checking the type of any variable.
Note:
After converting the number into a string, you can further apply string methods to that value.
For Example:
let num = 150;
let numStr = num.toString();
let checkLength = numStr.length;
console.log(checkLength);
Output: 3 // Because 150 has 3 characters
2. toExponential():
The toExponential()
method is a JavaScript function used to convert a number into its exponential notation (Scientific Notation). This format expresses the number as a product of a significant digit (mantissa) and a power of 10 (exponent). It is useful for scientific and technical applications where large numbers need to be represented in a concise format.
For Example:
let expo = 12305012;
let convertExpo = expo.toExponential();
console.log(convertExpo);
Output: 1.2305012e+7
How Scientific Notation Works:
Let's suppose you have a number as an integer. 123456789
You have a point after a digit 9
at the end position. When you start moving the point toward the left side, you increase the positive numbers in ten raised to the power, which is written in exponentiation as e
. If you move two points to the left side the the integer number is 1234567.89e+2
. Same as for the negative value of e
. If you move the point towards the right side in the integer then the value of e
raising is negative. Let me show you a practical example.
let expo1 = 123456789;
let convertPositiveExpo = expo1.toExponential();
console.log(convertPositiveExpo);
Output: 1.23456789e+8 // Here the point move 8 times towards right in expo1
// side so (e) has the power of +8
let expo2 = 0.31000;
let convertNegativeExpo = expo2.toExponential();
console.log(convertNegativeExpo);
Output: 3.1e-1 // Here the point move 1 time towards left in expo2
// side so (e) has the power of -1
3. valueOf():
The valueOf()
method simply returns the primitive value of an object. Primitive types of JavaScript like string, number, bigint, boolean, null, etc. JavaScript calls the valueOf()
method itself where appropriate.
For Example:
// For Number
let numVal = 120;
console.log(numVal.valueOf());
Output: 120
// For String
let strVal = "Muhammad Bilal";
console.log(strVal.valueOf());
Output: Muhammad Bilal
// For Object
let objVal = {
fName: "Muhammad",
lName: "Bilal",
age: 21,
};
console.log(objVal.valueOf());
Output: { fName: 'Muhammad', lName: 'Bilal', age: 21 }
Note:
There is rarely a need to explicitly call the
valueOf()
method in code.However, understanding its internal workings can help debug and understand JavaScript's type conversion mechanisms.
4. toPrecision():
The toPrecision()
method in JavaScript is used to format a number to a specific length. This method is useful when you need to control the number of decimal places displayed. The number will be rounded to the specified number of significant digits. If the formatted number requires more digits than the original number, decimals, and zeros will be added as needed. If the specified length is not given, then this method returns the entire number (without any formatting).
For Example:
let num = 156.1516;
let precise = num.toPrecision();
// Given no argument then it returns the value as it is
console.log(precise);
Output: 156.1516
let num = 156.1516;
let precise = num.toPrecision(4);
// Given argument 4 then it precise to the 4 digits with rounding of the
// last digit Without keeping the point in mind
console.log(precise);
Output: 156.2
let num = 156.1516;
let precise = num.toPrecision(9);
// Given argument 9 which is more than the given number
// Then it added the zero's itself to complete the specific lenght
console.log(precise);
Output: 156.151600
5. toFixed():
ThetoFixed()
method is used to format a number to a fixed number of decimal places. Also, it rounds the decimal. If the desired number of decimals is higher than the actual number, extra zeros are added to create the desired length. The toFixed()
method returns a string representation of the number with the specified number of decimal places.
For Example:
let num = 1561.5165;
let fixedVal = num.toFixed();
// Given no arguments then it returns the fixed
// number before point with rounding off the value
console.log(fixedVal);
// Output: 1562
let num = 156.15165;
let fixedVal = num.toFixed(3);
// If you given the argument 3 then it will give 3 fixed number
// after the point with rounding off the value
console.log(fixedVal);
// Output: 156.152
let num = 156.15165;
let fixedVal = num.toFixed(7);
// Given argument 7 which is more than the given number after point
// Then it added the points itself to complete the specific lenght
console.log(fixedVal);
// Output: 156.1516500
Number Properties in JavaScript:
JavaScript's Number
object provides various properties for working with numbers. JavaScript has some useful properties for manipulating and working with numbers.
1. EPSILON
The Number.EPSILON
property is a static property of the Number
object in JavaScript. It represents the smallest positive number approaching zero. In simpler terms, it's the difference between 1 and the next smallest representable floating-point number greater than 1.
For Example:
const epl = Number.EPSILON;
console.log(epl);
Output: 2.220446049250313e-16
// Here is the note for this value.
Note:
It is a static data property. It cannot be modified. These attributes cannot be changed. Number.EPSILON
is an ES6 feature. It does not work in Internet Explorer.
Due to limitations in how floating-point numbers are stored, comparing them directly using strict equality (===) can lead to unexpected results. Number.EPSILON can be used to account for this precision error.
// Checking for equality with EPSILON
let num1 = 1.000000000000001;
let num2 = 1;
if (Math.abs(num1 - num2) <= Number.EPSILON) {
console.log("num1 and num2 are equal");
} else {
console.log("num1 and num2 are not equal");
}
Output: num1 and num2 are not equal
2. MAX_VALUE
The Number.MAX_VALUE
the property represents the largest positive number representable in JavaScript. It's a static data property of the Number
object and it cannot be modified. Numbers that are smaller than Number.MAX_VALUE
is safe. if it becomes greater than it then it will lose precision.
For Example:
const maxNum = Number.MAX_VALUE;
console.log(maxNum);
Output: 1.7976931348623157e+308
Note:
You can use Number.MAX_VALUE
to validate user input or programmatically generated values to ensure they don't exceed the maximum representable number.
// Validating user input
const userInput = prompt("Enter a number:");
const number = Number(userInput);
if (number > Number.MAX_VALUE) {
alert("The number you entered is too large!");
}
3. MIN_VALUE
The Number.MIN_VALUE
the property represents the smallest positive numeric value representable in JavaScript. It's not the most negative number, but rather the number nearest to zero that can still be accurately represented within the limits of floating-point precision. It is also a static data property of an object. You cannot change or modify it. If the numbers are smaller than Number.MIN_VALUE
, are automatically converted to zero.
For Example:
const minNum = Number.MIN_VALUE;
console.log(minNum);
Output: 5e-324
// If numbers are smaller than Number.MIN_VALUE
if (number < Number.MIN_VALUE) {
Consider the number as practically zero
} else {
Perform calculations or comparisons
}
4. MAX_SAFE_INTEGER
The Number.MAX_SAFE_INTEGER
property represents the largest integer that is accurately representable in JavaScript. It is a constant value of 2⁵³ - 1
, which translates to 9007199254740991
. It is also a static value that cannot be modified.
For Example:
const maxSafeInt = Number.MAX_SAFE_INTEGER;
console.log(maxSafeInt);
Output: 9007199254740991
Integers larger than Number.MAX_SAFE_INTEGER
lose precision and are subject to rounding errors. Comparisons become unreliable.
For larger integers, JavaScript provides the BigInt
type. Number.MAX_SAFE_INTEGER
allow you to work with integers beyond the safe range with full accuracy.
Note: What is the meaning of lost precision?
I talked about it many times, and now let us check this. Let us take an example
const maxSafeInt = Number.MAX_SAFE_INTEGER;
console.log(maxSafeInt);
Output: 9007199254740991
// Now what if I plus 1 into it
let maxIntSum1 = Number.MAX_SAFE_INTEGER + 1;
console.log(maxIntSum1);
Output: 9007199254740992
// Now what if I plus 2 into it
let maxIntSum2 = Number.MAX_SAFE_INTEGER + 2;
console.log(maxIntSum2);
Output: 9007199254740992
// It prints the same result as before.
// So, here JavaScript didn't know what to do, this is maximum safe integer
This is I mean to say lost precision
5. MIN_SAFE_INTEGER
Number.MIN_SAFE_INTEGER
is a static data property of the Number object that represents the smallest integer that can be accurately and safely represented in JavaScript using the standard double-precision floating-point format (IEEE 754).
The integers are smaller than Number.MIN_SAFE_INTEGER
or larger than Number.MAX_SAFE_INTEGER
(which is 2⁵³ - 1
), consider using BigInt, a special data type introduced in ES2020 for representing arbitrarily large integers without precision loss.
For Example:
const minSafeInt = Number.MIN_SAFE_INTEGER;
console.log(minSafeInt);
Output: -9007199254740991
// If a number is smaller then this, then that is not a safe integer
It has some uses:
Validating input values for numerical calculations.
Detecting potential precision issues when working with numbers near the safe integer limits.
6. POSITIVE_INFINITY
The Number.POSITIVE_INFINITY
property represents the largest positive value that can be represented in JavaScript. This means it's "greater than any other number". It is a constant value always equal to infinity. It is a static value that cannot be modified.
For Example:
const posInf = Number.POSITIVE_INFINITY;
console.log(posInf);
Output: Infinity
// It occur when a number is divisible by 0
let num = 102 / 0;
console.log(num);
Output: Infinity
It has some uses:
Checking for overflow conditions in calculations.
Setting a maximum value for loops or iterations.
Indicating an error condition that returns a finite number in case of success (though
NaN
might be more appropriate).
7. NEGATIVE_INFINITY
The Number.NEGATIVE_INFINITY
the property represents negative infinity in JavaScript. You can think of it as a number that is smaller than any other number. It is a static value, meaning you cannot change its value.
For Example:
const negInf = Number.NEGATIVE_INFINITY;
console.log(negInf);
Output: -Infinity
// It occur when a number with (-) is divisible by 0
let num = -102 / 0;
console.log(num);
Output: -Infinity
8. NaN (Not a Number)
In JavaScript, NaN
stands for "Not a Number". It is a special value that is not equal to any other value, including itself. NaN
is used to represent results of operations that are invalid or undefined for numbers.
For Example:
// Check if a variable is NaN
const variable1 = "abc";
if (isNaN(variable1)) {
console.log("This is not a number");
}
Output: This is not a number
Note about NaN:
You can use the isNaN()
function to check if a value is NaN
. This function returns true
if the value isNaN
, and false
otherwise.
NaN
is not equal to any other value, including itself means:
isNaN(NaN)
is true.
NaN
is a member of the Number type. However, you cannot use arithmetic operations directly on NaN
. Trying to do so will always result in NaN
.
By understanding the NaN
property, you can write more robust and reliable JavaScript code.
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
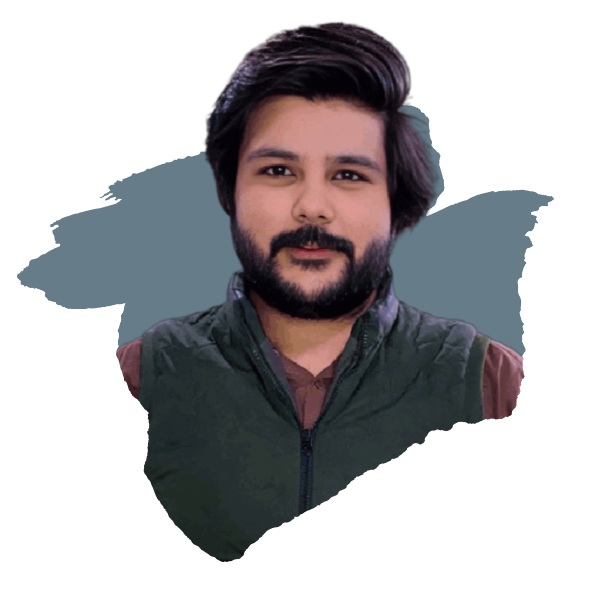
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. 📈 Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. 🔊 Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub 🖥 Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. ✨ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. 🎓 Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. 👥 Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.