Building a Self-Healing API in C#: A Developer's Guide

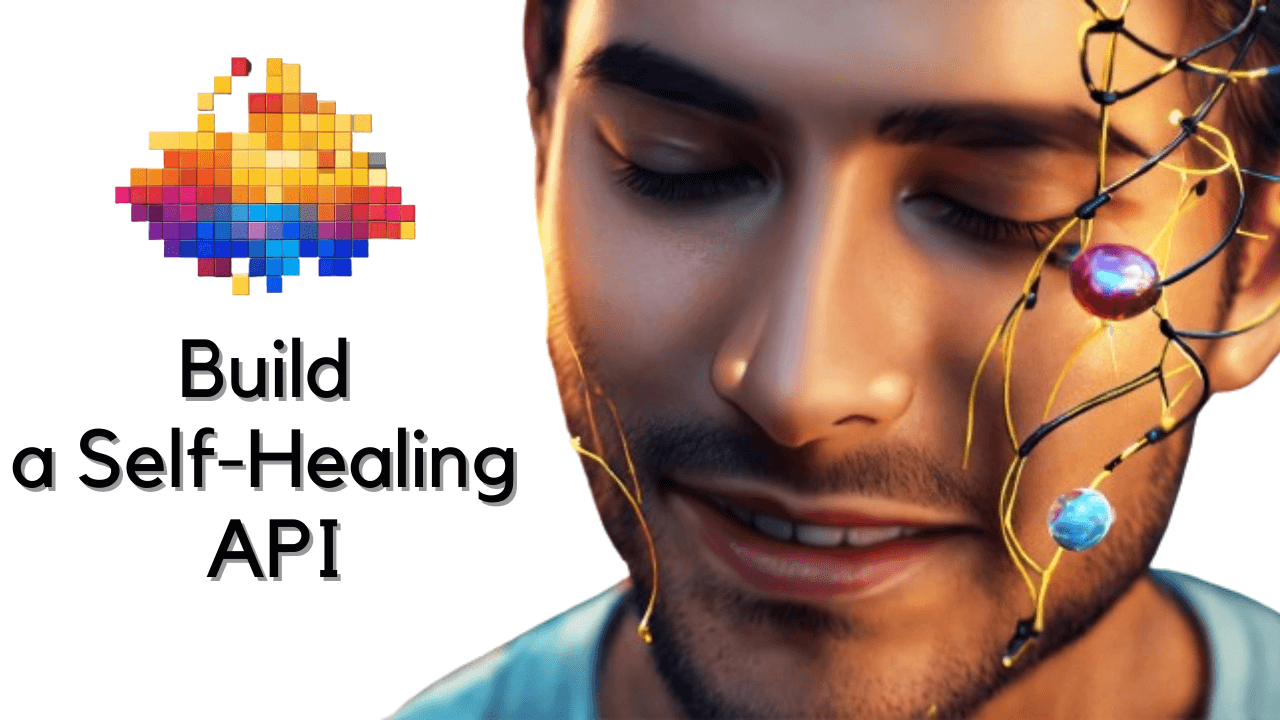
Building a Self-Healing API
In the fast-paced world of software development, creating robust APIs that can recover from failures is crucial. A self-healing API is designed to automatically detect and recover from errors, minimizing downtime and ensuring a seamless user experience. In this guide, we'll explore key concepts and provide practical examples using C#.
Retry Policies with Polly
One common strategy for handling transient failures is to implement a retry policy. We'll use the Polly library to demonstrate this approach.
Step 1: Install Polly
bashCopy codeInstall-Package Polly
Step 2: Implement Retry Policy
csharpCopy codeusing Polly;
using System;
public class ApiService
{
private readonly Policy _retryPolicy;
public ApiService()
{
_retryPolicy = Policy
.Handle<Exception>()
.WaitAndRetry(3, retryAttempt => TimeSpan.FromSeconds(Math.Pow(2, retryAttempt)));
}
public void CallApiWithRetry()
{
_retryPolicy.Execute(() =>
{
// Call your API here
// If an exception occurs, Polly will automatically retry according to the policy.
});
}
}
SignalR for Real-time Communication
Real-time communication is essential for monitoring API performance. We can use SignalR to broadcast performance data to connected clients.
Step 1: Install SignalR Client
bashCopy codeInstall-Package Microsoft.AspNet.SignalR.Client
Step 2: Configure SignalR in API
csharpCopy code// Refer to the previous example for configuring SignalR in your API
Step 3: Send Performance Data to SignalR Hub
csharpCopy codepublic class ApiService
{
private readonly HubConnection _hubConnection;
private readonly IHubProxy _hubProxy;
public ApiService()
{
_hubConnection = new HubConnection("http://your-api-url/signalr");
_hubProxy = _hubConnection.CreateHubProxy("PerformanceHub");
_hubConnection.Start().Wait();
}
public void CallApiWithSignalR()
{
try
{
// Call your API here
// If successful, send performance data to SignalR Hub
_hubProxy.Invoke("SendPerformanceData", "API call successful");
}
catch (Exception ex)
{
// Log or handle the exception
// Retry or fallback mechanisms can be added here
}
}
}
Circuit Breaker and Fallback Mechanism
A circuit breaker helps prevent unnecessary calls to a failing service. If the number of failures exceeds a threshold, the circuit is opened, and calls are redirected to a fallback mechanism.
Step 1: Install Polly
bashCopy codeInstall-Package Polly
Step 2: Implement Circuit Breaker and Fallback
csharpCopy codeusing Polly;
using Polly.CircuitBreaker;
using System;
public class ApiService
{
private readonly CircuitBreakerPolicy _circuitBreakerPolicy;
public ApiService()
{
_circuitBreakerPolicy = Policy
.Handle<Exception>()
.CircuitBreaker(3, TimeSpan.FromSeconds(30),
onBreak: (ex, timespan) => { Console.WriteLine("Circuit is open"); },
onReset: () => { Console.WriteLine("Circuit is reset"); });
}
public void CallApiWithCircuitBreaker()
{
try
{
_circuitBreakerPolicy.Execute(() =>
{
// Call your API here
// If the circuit is open, an exception will be thrown.
});
}
catch (BrokenCircuitException)
{
// Handle the case when the circuit is open
Console.WriteLine("Circuit is open. Handle accordingly.");
}
}
}
Health Checks with Endpoint Monitoring
Regular health checks of API endpoints are vital for proactive issue detection. Let's implement a simple health check mechanism.
Step 1: Implement Health Check
csharpCopy codepublic class HealthCheckService
{
public bool IsApiHealthy()
{
// Implement your health check logic, e.g., check database connectivity, external dependencies, etc.
return true; // Change to return the actual health status
}
}
Step 2: Use Health Check in API
csharpCopy codepublic class ApiService
{
private readonly HealthCheckService _healthCheckService;
public ApiService(HealthCheckService healthCheckService)
{
_healthCheckService = healthCheckService;
}
public void PerformHealthCheck()
{
if (_healthCheckService.IsApiHealthy())
{
// API is healthy, continue with operations
}
else
{
// API is not healthy, take necessary actions
}
}
}
Conclusion
Building a self-healing API involves combining various strategies like retry policies, real-time communication, circuit breakers, fallback mechanisms, and health checks. Using tools like Polly and SignalR, developers can create resilient APIs that automatically recover from failures, providing a more reliable and responsive experience for users. By implementing these concepts, you can enhance the robustness of your APIs and contribute to a more resilient software ecosystem. Happy coding!
Subscribe to my newsletter
Read articles from sameer bahad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
