Pythonic Paradox: Dancing with @classmethod and @staticmethod
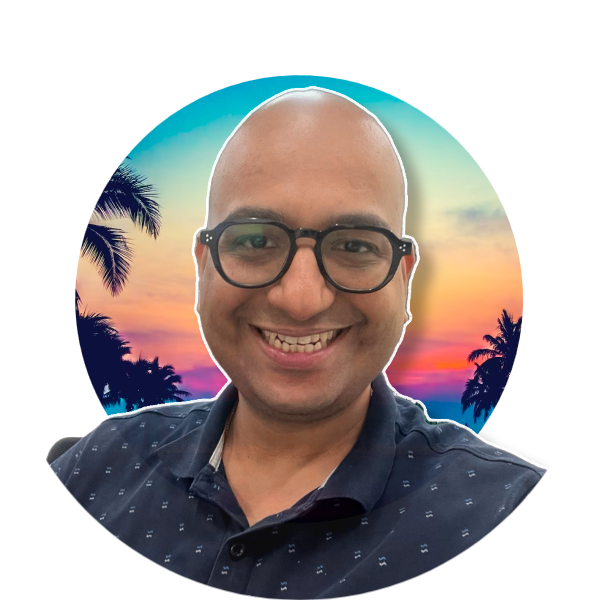
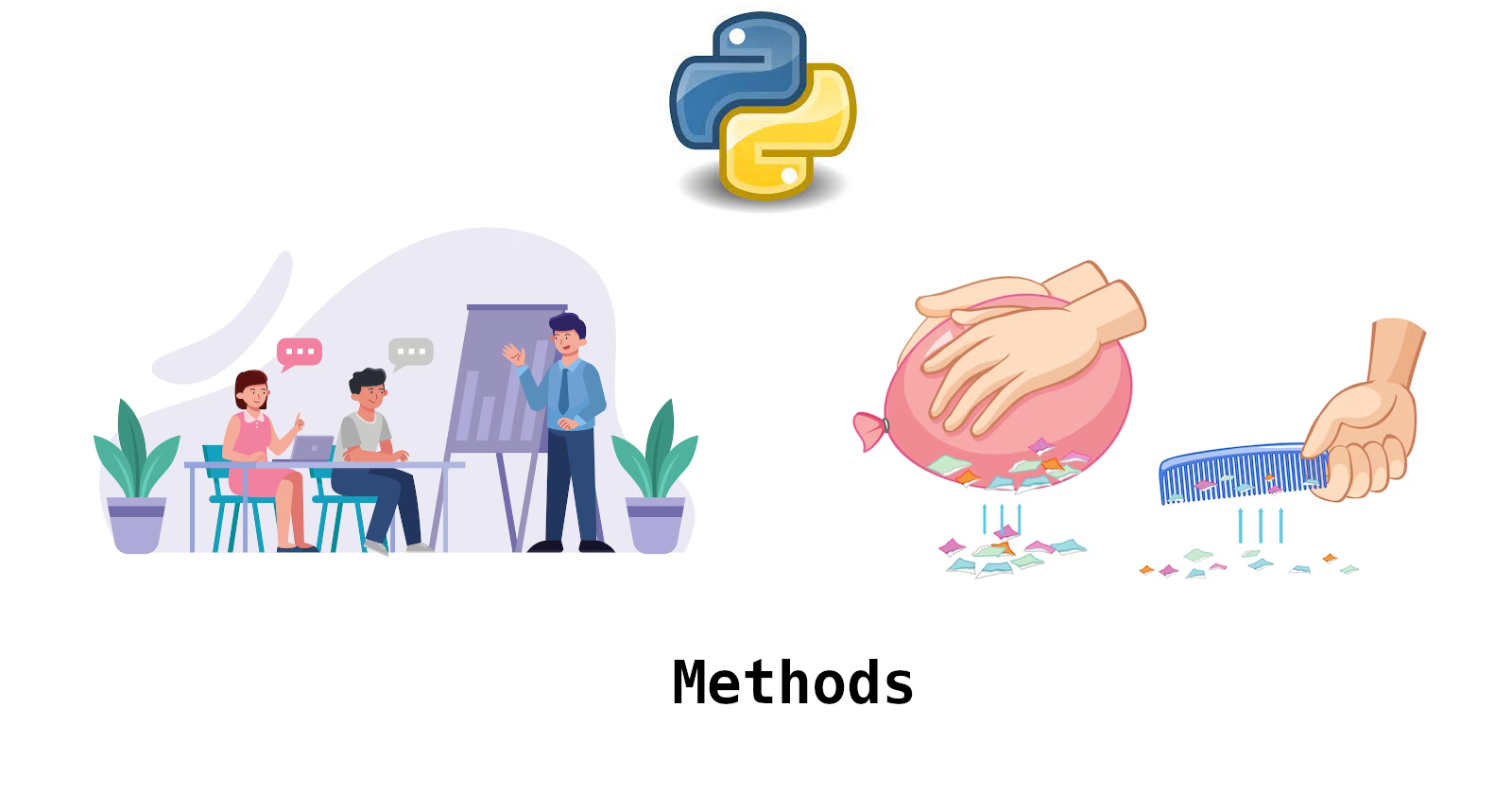
Intro
In Python, both classmethod
and staticmethod
are used to define methods that are bound to a class rather than an instance. However, they serve different purposes.
@classmethod
: This decorator is used to define a method that takes the class itself as its first argument. Conventionally, the first parameter is namedcls
. It can be used to create class-specific methods that have access to the class itself, allowing them to modify class state or create instances of that class.class MyClass: class_variable = "class_variable" @classmethod def class_method(cls): print(f"Accessing class variable: {cls.class_variable}") # Call the class method MyClass.class_method()
@staticmethod
: This decorator is used to define a method that does not take the class or instance as its first parameter. It behaves like a regular function but is included in a class for organizational purposes. It cannot access or modify class state.class MyClass: @staticmethod def static_method(): print("This is a static method") # Call the static method MyClass.static_method()
Summary table
Aspect | @classmethod | @staticmethod |
First Parameter | cls (class itself) | None (neither class nor instance) |
Access to Class State | Yes | No |
Access to Instance State | No | No |
Example Usage | Defining methods that modify class | Organizing utility functions within |
state or create instances | a class without access to instance | |
or class state |
Takeaway
In summary, use @classmethod
when you need access to the class itself within the method, and use @staticmethod
when you don't need access to either the instance or the class.
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
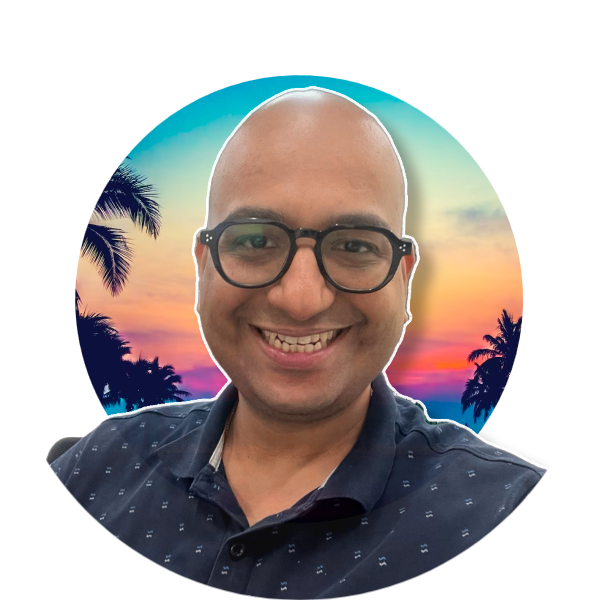
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.