151 DSA Problem journey

2 min read
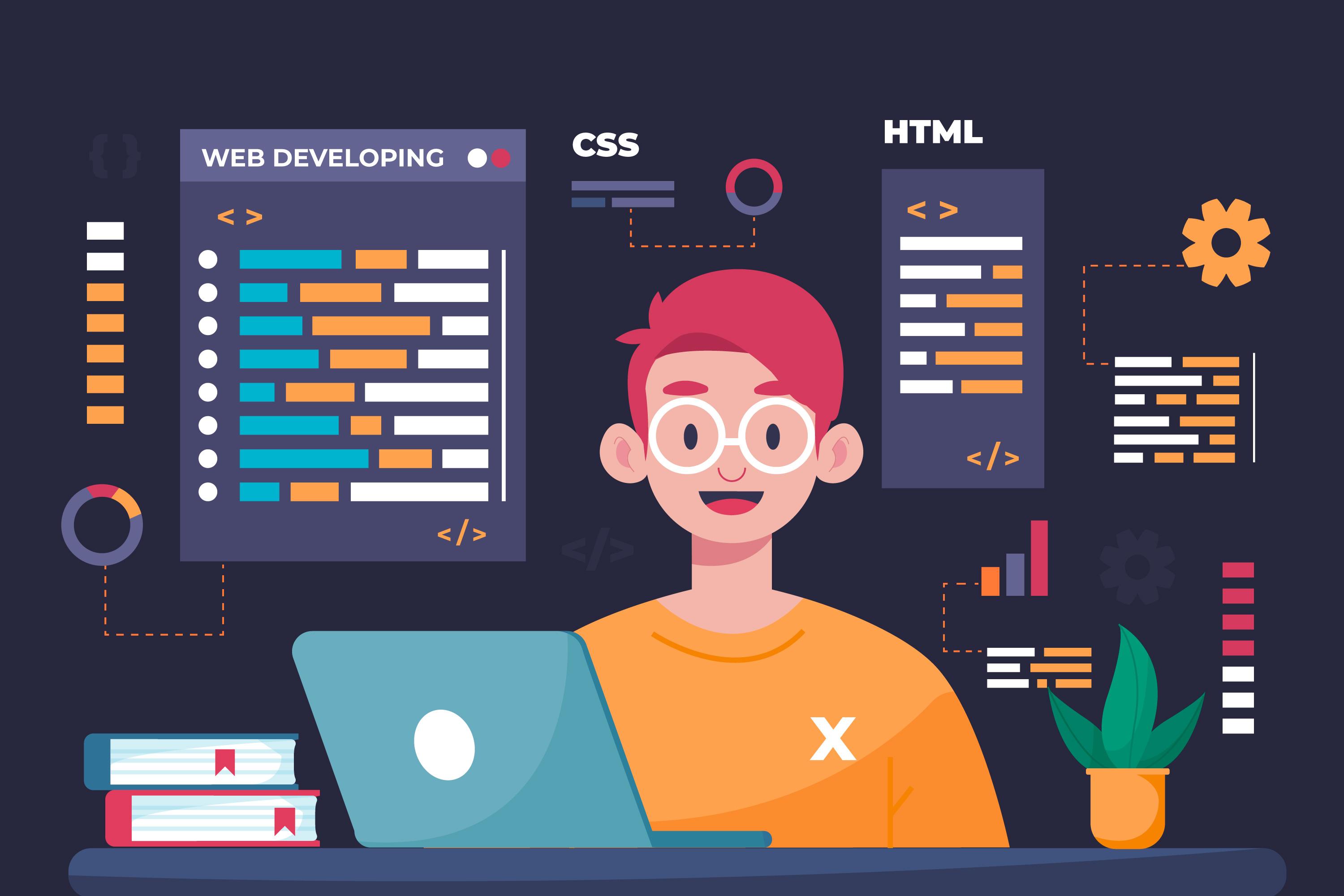
Q6:)Given an integer array of size n
, find all elements that appear more than ⌊ n/3 ⌋
times.
Example:
Input: nums = [3,2,3]
Output: [3]
Solution:
class Solution {
public:
vector<int> majorityElement(vector<int>& nums) {
int countMajority = nums.size()/3;
unordered_map<int, int> map;
vector<int> output;
for(auto i : nums){
map[i]++;
}
for(auto i : map){
if(i.second > countMajority){
output.push_back(i.first);
}
}
return output;
}
};
>Explantion:
The code aims to find elements in a given array (nums) that appear more than ⌊n/3⌋ times,
where n is the size of the array.
>Approach:
Initialize a variable countMajority to ⌊n/3⌋,representing the minimum count required
for an element to be considered a majority element.
Use an unordered map (map) to store the count of each unique element in the array.
Initialize an empty vector output to store the majority elements found.
>Counting Element Occurrences:
Iterate through the nums array using a range-based for loop.
For each element i, increment its count in the unordered map (map).
>Identifying Majority Elements:
Iterate through the key-value pairs in the unordered map using another range-based for loop.
If the count of an element (i.second) is greater than countMajority, it is considered a majority element.
Push the majority element (i.first) into the output vector.
>Result:
Return the output vector containing the majority elements found in the array.
If anyone have better solution so please comment:)
11
Subscribe to my newsletter
Read articles from GAURAV YADAV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
C++cppcodingcoding challenge#codingNewbiescoding journeyDSAcpProgramming Blogsprogramming languageslearningLearning Journey
Written by

GAURAV YADAV
GAURAV YADAV
Experienced Full Stack Developer with proficiency in comprehensive coding skills, adept at crafting end-to-end solutions.