151 DSA Problem journey

2 min read
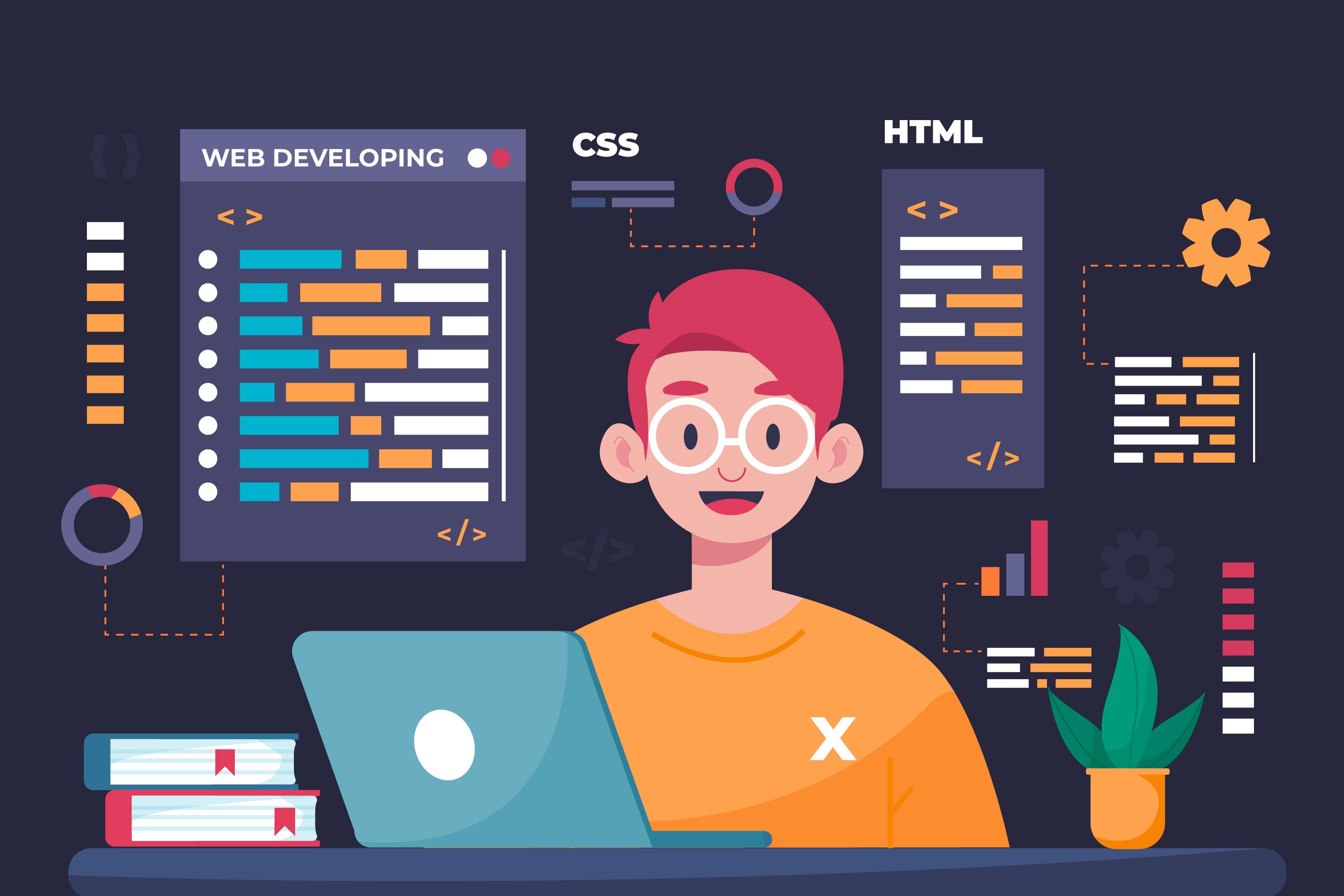
Q7:)Given a positive integer, find the smallest integer that has exactly the same digits existing in the integer n
and is greater in value than n
. If no such positive integer exists, return -1
.
Note that the returned integer should fit in a 32-bit integer, if there is a valid answer but it does not fit in a 32-bit integer, it returns -1
.
Example:
>Input: n = 12
Output: 21
>Input: n = 21
Output: -1
Solution:
class Solution {
public:
int nextGreaterElement(int n) {
auto digits = to_string(n);
next_permutation(begin(digits), end(digits));
auto res = stoll(digits);
return (res > INT_MAX || res <= n) ? -1 : res;
}
};
Explantion:
The given C++ code defines a class Solution with a public method nextGreaterElement.
This method takes an integer n as input and returns the next greater permutation of its digits.
If there is no such permutation, it returns -1.
Here's a breakdown of the code:
.)auto digits = to_string(n);:
Convert the integer n to a string, which makes it easier to work with individual digits.
.)next_permutation(begin(digits), end(digits));:
Rearrange the characters in the digits string to generate the next lexicographically greater permutation.
This function is part of the C++ Standard Template Library (STL).
.)auto res = stoll(digits);:
Convert the rearranged string back to an integer using stoll (string to long long).
.)return (res > INT_MAX || res <= n) ? -1 : res;:
Check if the result is greater than the maximum value of an integer (INT_MAX) or if it's less than or equal to the original number n.
If either condition is true, return -1; otherwise, return the result.
.)It's worth noting that this implementation assumes the input n is a 32-bit signed integer.
If the result is outside the range of a 32-bit integer, it returns -1. If there is no greater permutation,
it also returns -1.
If anyone have better solution so please comment:)
7
Subscribe to my newsletter
Read articles from GAURAV YADAV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
C++cppDSAcoding#codenewbiescoding challengecoding journeyLearning Journeylearn coding#LearningJourneyProgramming BlogsProgramming Tipsprogramming languages
Written by

GAURAV YADAV
GAURAV YADAV
Experienced Full Stack Developer with proficiency in comprehensive coding skills, adept at crafting end-to-end solutions.