đDay 16- Jenkins Interview Questions and Answers
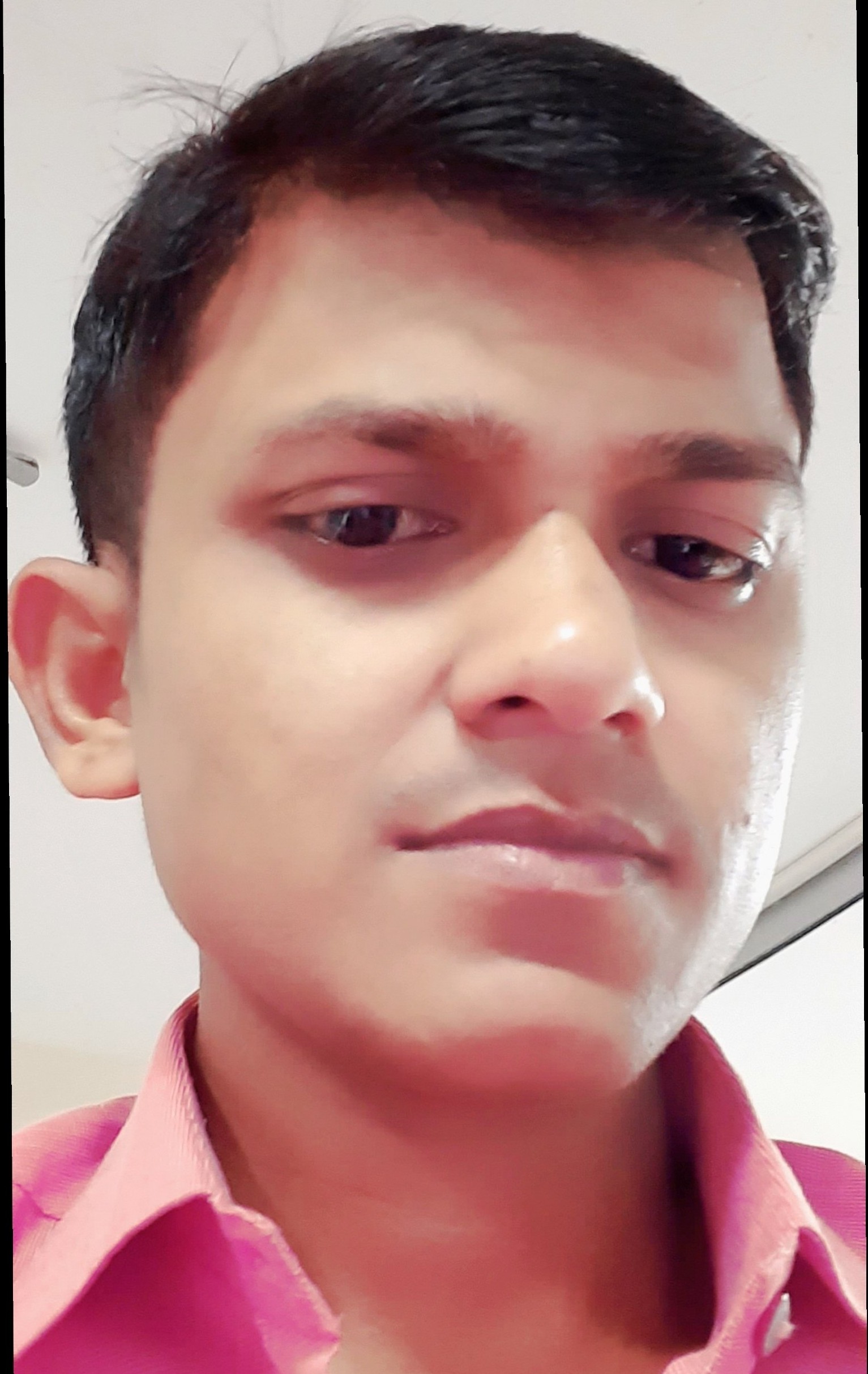
Table of contents
- Whatâs the difference between continuous integration, continuous delivery, and continuous deployment?
- What are the benefits of CI/CD?
- What is meant by CI/CD?
- What is Jenkins Pipeline?
- In Jenkins where do you find log files?
- Jenkins workflow and write a script for this workflow
- How to create a continuous deployment in Jenkins?
- How to build a job in Jenkins?
- Manually Building a Job:
- Automatically Building a Job:
- Why do we use pipelines in Jenkins?
- Is Only Jenkins enough for automation?
- How to handle secrets in Jenkins?
- 1. Credentials Plugin:
- 2. Jenkins Credentials Binding Plugin:
- 3. Secrets Management Tools:
- 4. Mask Passwords:
- 5. Use Jenkins Credentials in Pipelines:
- 6. Restrict Access:
- 7. Job-specific Credentials:
- 8. Plugin Integrations:
- 9. Regularly Rotate Secrets:
- Explain different stages in CI-CD setup
- 1. Version Control:
- 2. Continuous Integration (CI):
- 3. Code Quality Analysis:
- 4. Automated Testing:
- 5. Artifact Generation:
- 6. Deployment to Staging:
- 7. User Acceptance Testing (UAT):
- 8. Deployment to Production:
- 9. Post-Deployment Activities:
- 10. Monitoring and Logging:
- 11. Rollback (Optional):
- Name some of the plugins in Jenkins
- Source Code Management (SCM) Plugins:
- Build and Test Plugins:
- Deployment and Containerization Plugins:
- Artifact Management Plugins:
- Notification and Collaboration Plugins:
- Security and Authentication Plugins:
- Monitoring and Reporting Plugins:
- Infrastructure as Code (IaC) Plugins:
- Cloud and Virtualization Plugins:
- Miscellaneous Plugins:
Whatâs the difference between continuous integration, continuous delivery, and continuous deployment?
Continuous Integration (CI): CI is a software development practice in which code changes are frequently and automatically integrated into a shared repository. This practice aims to identify and fix integration issues early in the development process. It typically involves running automated tests and code analysis after each code commit.
Continuous Delivery (CD): CD extends CI by automating the process of deploying code changes to various environments (e.g., staging, testing, and production) after they have passed all tests. However, in the CD approach, the deployment to production is still a manual decision.
Continuous Deployment (CD): CD takes the automation one step further. It automatically deploys code changes to production as soon as they pass all tests in earlier environments. This approach reduces manual intervention and accelerates the delivery of new features or fixes to end-users.
What are the benefits of CI/CD?
Continuous Integration and Continuous Delivery (CI/CD) practices offer numerous benefits to development and operations teams, as well as the overall software delivery process. Here are some key advantages:
Faster Time to Market:
CI/CD automates the build, test, and deployment processes, reducing the time it takes to deliver new features or updates to end-users.
Faster releases enable organizations to respond more quickly to market demands and stay competitive.
Automated Testing:
CI/CD pipelines include automated testing, ensuring that new code changes are thoroughly tested before being deployed.
Automated tests help catch bugs and issues early in the development process, improving overall software quality.
Reduced Manual Intervention:
Automation in CI/CD reduces the need for manual intervention in the software delivery process.
This minimizes the risk of human errors, improves consistency, and allows teams to focus on more strategic tasks.
Increased Collaboration:
CI/CD encourages collaboration between development, testing, and operations teams.
Teams work together to define automated pipelines, share code, and resolve issues efficiently, fostering a culture of collaboration.
Early Detection of Defects:
Automated testing and continuous integration detect defects early in the development process.
Identifying and fixing issues during development prevents the accumulation of bugs, making the software more reliable and stable.
Consistent Build and Deployment Process:
CI/CD ensures a consistent and repeatable process for building, testing, and deploying applications.
Consistency reduces the likelihood of deployment failures caused by environmental differences.
Frequent and Smaller Releases:
CI/CD promotes the practice of releasing smaller increments of functionality more frequently.
Smaller releases are easier to manage, and they allow for faster user feedback and the ability to address issues promptly.
Improved Code Quality:
Automated builds and tests in CI/CD pipelines help maintain code quality standards.
Code quality checks, static code analysis, and automated code reviews contribute to a cleaner and more maintainable codebase.
Rapid Feedback Loop:
CI/CD provides a rapid feedback loop to developers by quickly identifying build and test failures.
Developers receive immediate feedback on the impact of their changes, facilitating faster problem resolution.
Efficient Rollback Mechanism:
CI/CD pipelines include efficient rollback mechanisms in case of deployment failures.
The ability to roll back to a known good state quickly reduces downtime and minimizes the impact of issues on end-users.
Enhanced Security Practices:
CI/CD allows for the integration of security checks and scans into the pipeline.
Security practices such as vulnerability scanning and code analysis can be automated, ensuring that security is an integral part of the development process.
Infrastructure as Code (IaC):
CI/CD encourages the use of Infrastructure as Code, allowing teams to automate the provisioning and management of infrastructure.
IaC promotes consistency, reproducibility, and versioning of infrastructure configurations.
Scalability and Flexibility:
CI/CD pipelines can scale to accommodate the growing needs of development teams and applications.
The flexibility of CI/CD pipelines allows teams to adapt and optimize processes based on project requirements.
Data-Driven Decision-Making:
CI/CD pipelines generate data and metrics that can be used for performance analysis and improvement.
Data-driven insights enable teams to make informed decisions about the efficiency and effectiveness of their processes.
Adopting CI/CD practices is crucial for organizations aiming to enhance their software development lifecycle, improve overall software quality, and deliver value to end-users more rapidly and consistently.
What is meant by CI/CD?
CI/CD stands for Continuous Integration and Continuous Delivery (or Continuous Deployment), and it represents a set of modern software development practices aimed at automating and streamlining the process of delivering software changes to production. These practices are often associated with the broader DevOps movement.
or
CI/CD is a combination of Continuous Integration and Continuous Delivery/Deployment practices. It represents a holistic approach to automating and streamlining the software development process, from code integration through testing and deployment to production.
What is Jenkins Pipeline?
Jenkins Pipeline is a set of plugins and tools that allows you to define and manage your build, test, and deployment process as code. You can define complex, multi-step workflows using a domain-specific language called "Pipeline DSL." Jenkins Pipeline allows you to version control your build and deployment pipelines, making them more maintainable and transparent.
In Jenkins where do you find log files?
In Jenkins, log files for build jobs and pipeline executions can be found in the build's workspace on the Jenkins master or the build agent (also known as a node or slave) where the job ran. The location of log files may vary based on the type of job and the specific configuration of the Jenkins instance. Here are common places to find log files:
Jenkins Job Console Output:
The primary location to view log output is on the Jenkins web interface.
Navigate to the specific build job and select the build number you are interested in.
Click on "Console Output" or "Console Log" to view the live log or download the log file.
Workspace Directory:
Log files are usually generated in the workspace directory where the build or pipeline is executed.
The workspace directory is typically located on the Jenkins master or on the build agent machine (node) where the job ran.
The exact path can be found in the Jenkins job configuration under "Build Environment" or "Advanced Project Options."
Build Artifacts:
Some builds or pipelines generate log files as artifacts, and these can be archived and accessed after the build completes.
If the build job archives artifacts, you can find them in the "Artifacts" section of the build page.
Jenkins Master Log Files:
Logs related to Jenkins master itself, including startup logs, can be found in the Jenkins home directory.
The default location is often
/var/jenkins_home
on Linux systems.
Build History:
The build history page in Jenkins provides a summary of all builds for a specific job.
From this page, you can click on a specific build number to access the console output.
Log Rotation:
Jenkins may rotate and archive log files based on its configuration.
The rotation settings can be found in the Jenkins system configuration.
Keep in mind that the specific locations and configurations may vary depending on how Jenkins is installed, the operating system, and the job configurations. If Jenkins is running in a distributed environment with multiple nodes, you may need to check the workspace of the specific node where the job executed. Always refer to the Jenkins job configuration and documentation for the most accurate information.
Jenkins workflow and write a script for this workflow
Jenkins Workflow is a way to define and orchestrate complex, multi-step workflows in Jenkins using the Workflow DSL (Domain-Specific Language). It allows you to express your entire build and deployment pipeline as code. Below is an example of a simple Jenkins Workflow script using the Declarative Pipeline syntax.
This example assumes a workflow with the following stages:
Build: Compile the source code and generate artifacts.
Test: Run automated tests.
Deploy to Staging: Deploy the application to a staging environment.
Manual Approval: Require manual approval before deploying to production.
Deploy to Production: Deploy the application to the production environment.
groovyCopy codepipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building the application...'
// Add your build steps here
}
}
stage('Test') {
steps {
echo 'Running automated tests...'
// Add your test steps here
}
}
stage('Deploy to Staging') {
steps {
echo 'Deploying to staging environment...'
// Add your deployment steps for staging here
}
}
stage('Manual Approval') {
steps {
script {
def userInput = input(
id: 'userInput',
message: 'Do you approve deploying to production?',
parameters: [booleanParam(defaultValue: true, description: 'Approve?', name: 'Proceed')]
)
if (!userInput.Proceed) {
error 'Production deployment not approved. Aborting.'
}
}
}
}
stage('Deploy to Production') {
steps {
echo 'Deploying to production environment...'
// Add your deployment steps for production here
}
}
}
post {
success {
echo 'Workflow completed successfully. Ready for the next iteration!'
}
failure {
echo 'Workflow failed. Please check the logs for details.'
}
}
}
Explanation:
The
pipeline
block defines the overall structure of the pipeline.The
agent any
specifies that the pipeline can run on any available agent.The
stages
block defines different stages of the pipeline: Build, Test, Deploy to Staging, Manual Approval, and Deploy to Production.Inside each
stage
, thesteps
block contains the specific actions for that stage. Replace theecho
statements with actual build, test, and deployment commands.The
post
block defines post-build actions. In this example, it prints a message based on the success or failure of the pipeline.The
input
step is used in the 'Manual Approval' stage to pause the pipeline and wait for manual input before proceeding to deploy to production.
This script represents a simple CI/CD workflow with manual approval before deploying to production. Customize the script according to your specific build and deployment requirements.
How to create a continuous deployment in Jenkins?
Creating a continuous deployment (CD) pipeline in Jenkins involves defining a set of automated steps to deploy your application to production or a production-like environment. Below, I'll guide you through the process of setting up a basic continuous deployment pipeline using Jenkins. This example assumes you have already set up Jenkins and have a working pipeline for building and testing your application.
Install Necessary Plugins:
- Ensure that you have the necessary plugins installed in Jenkins to support your deployment targets (e.g., plugins for Docker, Kubernetes, AWS, etc.).
Set Up Deployment Environment Credentials:
If your deployment involves external services or environments, such as AWS, Docker Hub, or a Kubernetes cluster, you'll need to configure credentials in Jenkins to access these services.
Go to "Manage Jenkins" > "Manage Credentials" > "(global)" and add the necessary credentials.
Adjust Your Jenkinsfile:
Open your Jenkinsfile (or create one if you don't have it).
Add a new stage for deployment, specifying the deployment steps based on your deployment target.
groovyCopy codepipeline {
agent any
stages {
stage('Build') {
// Your build steps
}
stage('Test') {
// Your test steps
}
stage('Deploy to Production') {
steps {
// Add deployment steps here
// Example: Deploying a Docker container to a production environment
script {
withCredentials([usernamePassword(credentialsId: 'DOCKER_HUB_CREDENTIALS', usernameVariable: 'DOCKER_USERNAME', passwordVariable: 'DOCKER_PASSWORD')]) {
sh 'docker login -u $DOCKER_USERNAME -p $DOCKER_PASSWORD'
sh 'docker build -t your-image:latest .'
sh 'docker push your-image:latest'
// Additional deployment steps...
}
}
}
}
}
post {
success {
echo 'Pipeline completed successfully. Ready for the next iteration!'
}
failure {
echo 'Pipeline failed. Please check the logs for details.'
}
}
}
Configure Jenkins Pipeline Job:
Create a new Jenkins pipeline job or update your existing job.
Configure the job to use your Jenkinsfile (located in your version control system, e.g., Git).
Save the job configuration.
Run the Pipeline:
Trigger the pipeline manually or configure it to be triggered automatically on code changes.
Observe the pipeline execution in the Jenkins interface, including the deployment stage.
This is a simple example, and the deployment steps will depend on your specific deployment target and strategy. Make sure to adapt the script and steps to match your deployment requirements, whether you're deploying to cloud services, container orchestration platforms, or traditional server environments.
Remember to exercise caution when implementing continuous deployment, especially in production environments, and consider incorporating approval steps or additional checks to ensure the safety and reliability of your deployments.
How to build a job in Jenkins?
Building a job in Jenkins typically involves triggering a Jenkins job manually or automatically, and Jenkins will then execute the defined steps in the job. Here are the steps to manually build a job in Jenkins:
Manually Building a Job:
Access Jenkins:
- Open a web browser and navigate to the Jenkins web interface. Typically, it's accessible at
http://your-jenkins-server:8080
.
- Open a web browser and navigate to the Jenkins web interface. Typically, it's accessible at
Log In:
- Log in to Jenkins with your username and password.
Navigate to the Job:
- From the Jenkins dashboard, locate and click on the name of the job you want to build. This will take you to the job's details page.
Start a Build:
- On the job details page, you'll find a menu on the left. Click on "Build Now" to manually trigger a build of the selected job.
Monitor Build Progress:
Once the build is triggered, you can monitor its progress on the job details page.
Click on the build number to view the console output, which shows the live log of the build process.
Automatically Building a Job:
Jenkins can also be configured to automatically trigger a build based on various events, such as code commits, pull requests, or scheduled intervals. Here's a brief overview of how you can set up automatic builds:
Configure SCM (Source Code Management):
In the job configuration page, under the "Source Code Management" section, configure the details for your version control system (e.g., Git).
Provide the repository URL and credentials if needed.
Configure Build Triggers:
On the job configuration page, find the "Build Triggers" section.
Choose the trigger that suits your needs. Common options include:
Poll SCM: Jenkins periodically checks for changes in the version control system.
GitHub/GitLab Hook: Jenkins is notified by a webhook when a code change occurs.
Bitbucket Webhook: Similar to GitHub/GitLab, Jenkins is notified by a webhook in Bitbucket.
Build after other projects are built: Trigger a build when another job is completed.
Save the Job Configuration:
- Save the job configuration after making changes.
Automatic Builds:
- After configuring triggers, Jenkins will automatically start a build when the specified conditions are met.
Remember to configure your job and triggers based on the requirements of your project and team. Automatic builds are beneficial for continuous integration, ensuring that code changes are regularly tested and integrated.
Why do we use pipelines in Jenkins?
Pipelines in Jenkins offer a structured and automated way to define, orchestrate, and visualize complex software delivery processes. Here are several reasons why pipelines are widely used in Jenkins and the broader context of continuous integration and continuous delivery (CI/CD):
Code as Infrastructure:
- Pipelines allow you to define your entire CI/CD process as code, known as "Pipeline as Code." This provides version control, collaboration, and the ability to track changes over time.
End-to-End Automation:
Pipelines automate the entire software delivery process, from code integration and testing to deployment and delivery.
Automation reduces manual errors, improves consistency, and accelerates the delivery pipeline.
Continuous Integration (CI):
Pipelines facilitate continuous integration by automating the build and test phases whenever changes are pushed to the version control system.
This ensures that code changes are regularly and automatically validated.
Continuous Delivery (CD):
Pipelines extend CI to continuous delivery by automating the deployment of tested and validated code to staging or production environments.
CD pipelines allow for rapid, reliable, and repeatable software releases.
Flexibility and Customization:
Jenkins Pipelines are highly flexible and can be customized to suit the specific needs and workflows of different projects and teams.
Pipelines support both declarative and scripted syntax, providing options for simplicity or advanced customization using Groovy scripting.
Parallel and Sequential Execution:
Pipelines support parallel and sequential execution of stages and steps.
Parallelization improves efficiency by running tasks concurrently, while sequential execution ensures dependencies are met.
Visualization and Monitoring:
Pipelines offer a visual representation of the software delivery process.
The Jenkins interface provides a real-time view of pipeline progress, making it easy to monitor and troubleshoot.
Infrastructure as Code (IaC):
- Pipelines can include Infrastructure as Code (IaC) practices, allowing you to automate the provisioning and management of infrastructure alongside application code.
Collaboration:
Pipelines encourage collaboration between development, testing, and operations teams.
The pipeline definition is stored in version control, making it accessible to all team members.
Reusability with Shared Libraries:
- Shared libraries in Jenkins allow teams to reuse and share common pipeline components, promoting consistency and best practices across projects.
Parameterization and Dynamism:
Pipelines support parameterization, allowing users to provide input when triggering a build or deployment.
Dynamic pipeline constructs enable the creation of flexible and adaptable workflows.
Integrated Security Practices:
- Pipelines can include security practices such as vulnerability scanning, code analysis, and compliance checks as integral parts of the process.
Scaling CI/CD:
Pipelines are scalable and can handle the automation of CI/CD processes for both small and large projects.
Scaling is achieved by distributing builds across multiple agents or by parallelizing tasks within a single pipeline.
By leveraging pipelines in Jenkins, teams can achieve a more efficient, transparent, and reliable software delivery process, enabling them to respond rapidly to changing requirements and deliver value to end-users consistently.
Is Only Jenkins enough for automation?
While Jenkins is a popular and powerful automation tool, it may not be the only tool needed for comprehensive automation, depending on the specific requirements of your organization, projects, and workflows. Automation in the software development lifecycle often involves various aspects, and different tools may be used to address specific needs. Here are some considerations:
Continuous Integration and Continuous Delivery (CI/CD):
- Jenkins excels at CI/CD automation, managing build processes, and orchestrating deployment pipelines. However, other tools like GitLab CI/CD, Travis CI, CircleCI, and GitHub Actions also offer robust CI/CD capabilities.
Source Code Management (SCM):
- Jenkins integrates with various version control systems (e.g., Git, SVN), but SCM tools like Git, GitHub, GitLab, and Bitbucket are commonly used independently for version control and collaboration.
Configuration Management:
- Tools like Ansible, Puppet, and Chef are used for configuration management, automating the provisioning and configuration of infrastructure.
Container Orchestration:
- Kubernetes is widely used for container orchestration, and tools like Docker Swarm and Amazon ECS are also popular. Jenkins can be integrated with these tools for container-based deployments.
Artifact Repository:
- Tools like Artifactory and Nexus are commonly used for managing and storing artifacts, such as binaries and libraries, in a centralized repository.
Infrastructure as Code (IaC):
- Terraform, AWS CloudFormation, and Azure Resource Manager are used for defining and managing infrastructure as code. Jenkins can integrate with these tools to automate infrastructure provisioning.
Automated Testing:
- Selenium, JUnit, TestNG, and other testing frameworks are often used for automated testing. Jenkins can be configured to trigger and manage test execution.
Monitoring and Logging:
- Tools like Prometheus, Grafana, ELK Stack (Elasticsearch, Logstash, Kibana), and Splunk are used for monitoring and logging. Jenkins may integrate with these tools to provide visibility into the CI/CD pipeline and application performance.
Security Scanning:
- SonarQube, OWASP ZAP, and other security scanning tools can be used for code analysis and vulnerability scanning. Jenkins can incorporate these tools as part of the CI/CD pipeline.
Collaboration and Communication:
- Tools like Slack, Microsoft Teams, and Jira are used for team collaboration and communication. Jenkins can be configured to send notifications and updates to these collaboration tools.
Cloud Services:
- Cloud providers such as AWS, Azure, and Google Cloud Platform offer a variety of services for infrastructure, storage, and other cloud-based requirements. Jenkins can interact with these services as part of the automation process.
In summary, Jenkins plays a crucial role in CI/CD automation, but a comprehensive automation strategy often involves a combination of tools to address various aspects of the software development lifecycle. The choice of tools depends on the specific needs, preferences, and technology stack of your organization. Integrating these tools effectively ensures a seamless and efficient automation process from code development to production deployment.
How to handle secrets in Jenkins?
Handling secrets securely in Jenkins is crucial to maintaining the integrity and confidentiality of sensitive information, such as API keys, passwords, and other credentials. Jenkins provides several mechanisms to manage secrets, and here are some best practices:
1. Credentials Plugin:
Use the built-in Credentials plugin in Jenkins to store and manage secrets securely.
Go to "Jenkins" > "Manage Jenkins" > "Manage Credentials" to add, update, or remove credentials.
Different types of credentials are supported, including username/password, secret text, and secret file.
2. Jenkins Credentials Binding Plugin:
Use the Credentials Binding plugin to inject secrets directly into your build jobs or pipelines.
This plugin allows you to bind credentials to environment variables or files securely.
It prevents secrets from being exposed in the console output.
3. Secrets Management Tools:
Consider using external secrets management tools like HashiCorp Vault or AWS Secrets Manager.
These tools allow you to centralize and manage secrets independently of Jenkins, providing additional security features.
4. Mask Passwords:
Configure Jenkins to mask passwords in the build console output to prevent accidental exposure.
Go to "Jenkins" > "Manage Jenkins" > "Configure Global Security" and check the option "Mask passwords that appear in the console output."
5. Use Jenkins Credentials in Pipelines:
In Jenkins Pipeline scripts, use the
withCredentials
step to safely inject secrets into the environment.Example:
pipeline { agent any stages { stage('Example') { steps { script { withCredentials([usernamePassword(credentialsId: 'my-credentials-id', usernameVariable: 'USERNAME', passwordVariable: 'PASSWORD')]) { echo "Username: $USERNAME" echo "Password: $PASSWORD" } } } } } }
6. Restrict Access:
Restrict access to Jenkins and sensitive information to authorized personnel.
Use Jenkins security settings to define user roles and permissions carefully.
7. Job-specific Credentials:
Whenever possible, use job-specific credentials with the principle of least privilege.
Avoid using global credentials that have broader access than necessary.
8. Plugin Integrations:
- When using Jenkins plugins (e.g., for cloud providers, and source control systems), configure them to use credentials from the Jenkins credential store.
9. Regularly Rotate Secrets:
- Implement a regular rotation policy for sensitive credentials to minimize the impact of potential leaks.
Remember that securing secrets is a shared responsibility, and it's essential to educate Jenkins users and administrators about best practices for handling sensitive information. Additionally, integrate Jenkins with other security measures such as firewalls, secure network configurations, and regular security audits.
Explain different stages in CI-CD setup
In a Continuous Integration/Continuous Deployment (CI/CD) setup, different stages are used to automate and orchestrate the software delivery process. Each stage represents a phase in the pipeline, and the pipeline as a whole ensures that code changes are automatically and systematically tested, validated, and deployed. Here are common stages in a CI/CD setup:
1. Version Control:
Objective: Ensure that all code changes are versioned and tracked.
Activities:
Developers commit code changes to a version control system (e.g., Git).
Branching strategies are employed for feature development, bug fixes, and release preparation.
2. Continuous Integration (CI):
Objective: Automatically integrate code changes and perform basic tests.
Activities:
Triggered whenever code changes are pushed to the version control system.
Build the application to check for compilation errors.
Run unit tests to validate basic functionality.
3. Code Quality Analysis:
Objective: Analyze code for adherence to coding standards and identify potential issues.
Activities:
Run static code analysis tools (e.g., SonarQube) to check code quality.
Identify code smells, security vulnerabilities, and maintainability issues.
4. Automated Testing:
Objective: Ensure the stability and reliability of the application through automated tests.
Activities:
Run various types of automated tests, including unit tests, integration tests, and end-to-end tests.
Validate functional requirements, edge cases, and performance aspects.
5. Artifact Generation:
Objective: Generate deployable artifacts (e.g., binaries, packages).
Activities:
- Create artifacts that can be deployed to various environments.
6. Deployment to Staging:
Objective: Deploy the application to a staging environment for further testing.
Activities:
Deploy the generated artifacts to a staging or pre-production environment.
Conduct additional testing, including user acceptance testing (UAT).
7. User Acceptance Testing (UAT):
Objective: Allow end-users or stakeholders to validate the application in a production-like environment.
Activities:
Invite stakeholders to test the application in the staging environment.
Collect feedback and address any issues identified during UAT.
8. Deployment to Production:
Objective: Deploy the application to the production environment.
Activities:
Automated deployment of the artifacts to the production environment.
May include steps for database migrations, cache warming, and other production-specific tasks.
9. Post-Deployment Activities:
Objective: Perform activities after the deployment to ensure a smooth transition to the new version.
Activities:
Monitor the application's performance and error rates.
Trigger any post-deployment scripts or tasks.
Update documentation and inform relevant stakeholders.\
10. Monitoring and Logging:
Continuously monitor the application's performance and health.
11. Rollback (Optional):
Revert to a previous version in case of issues.
Define a rollback strategy and automate the rollback process. Trigger the rollback if critical issues are identified after deployment.
These stages collectively form a CI/CD pipeline, ensuring that code changes are automatically validated and delivered through various environments before reaching production. The specific stages and activities can be customized based on the project's requirements and the desired level of automation.
Name some of the plugins in Jenkins
Jenkins supports a vast ecosystem of plugins that extend its functionality and integrate with various tools and technologies. Here are some commonly used Jenkins plugins across different categories:
Source Code Management (SCM) Plugins:
Git Plugin: Integrates Jenkins with Git, enabling the use of Git repositories in Jenkins jobs.
GitHub Plugin: Provides integration with GitHub, allowing Jenkins to trigger builds on GitHub events and report build status.
Build and Test Plugins:
Maven Integration Plugin: Adds support for Apache Maven, facilitating the building and managing of Java projects.
Gradle Plugin: Integrates Jenkins with the Gradle build tool for building and testing projects.
JUnit Plugin: Parses JUnit test results and generates reports in Jenkins.
Pipeline Plugin: Enables the use of Jenkins Pipeline, allowing users to define their build process as code.
Deployment and Containerization Plugins:
Docker Plugin: Integrates Jenkins with Docker, allowing the building and running of Docker containers.
Kubernetes Continuous Deploy Plugin: Facilitates the deployment of applications to Kubernetes clusters.
Artifact Management Plugins:
Artifactory Plugin: Integrates Jenkins with JFrog Artifactory for artifact management and distribution.
Nexus Platform Plugin: Integrates Jenkins with Sonatype Nexus for artifact storage and management.
Notification and Collaboration Plugins:
Email Extension Plugin: Extends Jenkins email notification capabilities, providing more flexibility in email content and recipients.
Slack Notification Plugin: Sends build notifications to Slack channels.
HipChat Plugin: Integrates Jenkins with Atlassian HipChat for team communication.
Security and Authentication Plugins:
Role-based Authorization Strategy: Allows the configuration of fine-grained access control and permissions based on roles.
Active Directory Plugin: Integrates Jenkins with Microsoft Active Directory for authentication and authorization.
Monitoring and Reporting Plugins:
Prometheus Plugin: Exposes Jenkins metrics in Prometheus format for monitoring.
SonarQube Plugin: Integrates Jenkins with SonarQube for code quality analysis and reporting.
Infrastructure as Code (IaC) Plugins:
Terraform Plugin: Integrates Jenkins with HashiCorp Terraform for managing infrastructure as code.
Cloud and Virtualization Plugins:
Amazon EC2 Plugin: Allows Jenkins to dynamically provision and manage Amazon EC2 instances.
Google Compute Engine Plugin: Integrates Jenkins with Google Compute Engine for cloud-based build agents.
Miscellaneous Plugins:
EnvInject Plugin: Injects environment variables into the build process.
Git Parameter Plugin: Allows Jenkins jobs to take parameters from Git repositories.
Job DSL Plugin: Enables the use of Domain-Specific Language (DSL) for defining jobs as code.
These are just a few examples, and the Jenkins Plugin Index includes a wide range of plugins covering diverse use cases. When using plugins, it's essential to keep them updated to leverage new features, improvements, and security patches. Always refer to the Jenkins Plugin Index for the latest and most comprehensive list of available plugins.
Subscribe to my newsletter
Read articles from Mohd Ishtikhar Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
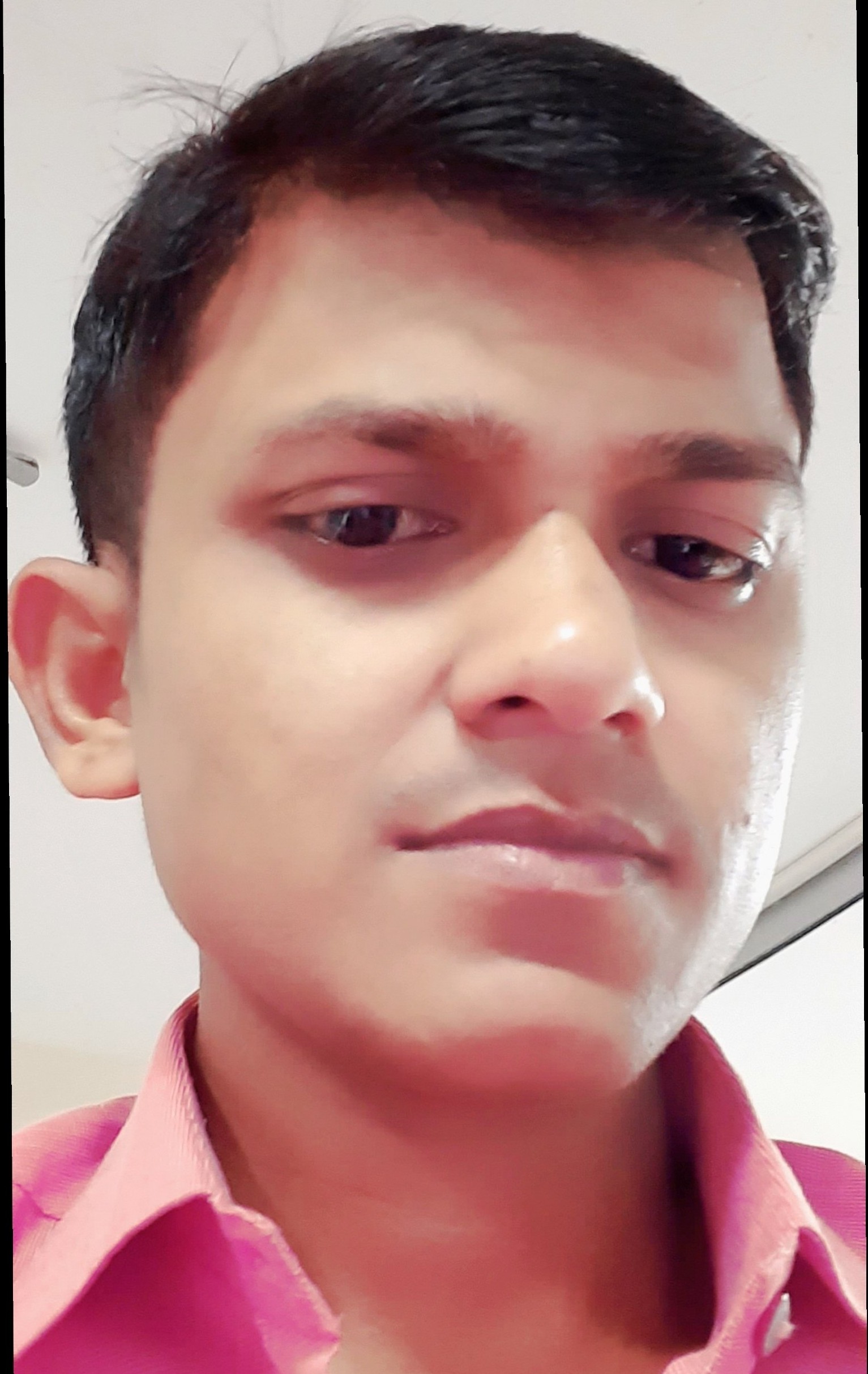
Mohd Ishtikhar Khan
Mohd Ishtikhar Khan
đ Hello There!!! I am Mohd Ishtikhar Khan, a skilled Cloud & DevOps engineer, having experience in automating various aspects of software development and deployment, including code integration, testing, and deployment processes and Planning and designing the cloud infrastructure in AWS. âď¸ I thrive in bridging the gap between development and operations teams, with the goal of automating and streamlining the software development and deployment process. As Cloud and DevOps Engineers, we work to improve the speed, efficiency, and quality of software delivery, making it possible to release code faster and more reliably. Experience in Designing and deploying dynamically scalable, available, fault-tolerant, and reliable applications on the Cloud. Maintenance and support of cloud infrastructure.Implementing cost-control strategies and Troubleshooting and resolving issues with the cloud infrastructure. Experience in designing solutions that will help customers migrate, operate, deploy, optimize, and execute the DevOps vision of a project and also have a strong passion for technology exploration and development. I believe that I am somebody with a very strong work ethic because I thrive in challenging environments and I also love building relationships and going out of the way to help a client. đ¤Let's connect! If you're looking to discuss DevOps fundamentals or if you want to share your experience, feel free to reach out to me at mohdishtikhar1786@gmail.com.