Math Object In Depth: Its Properties and Methods in JavaScript
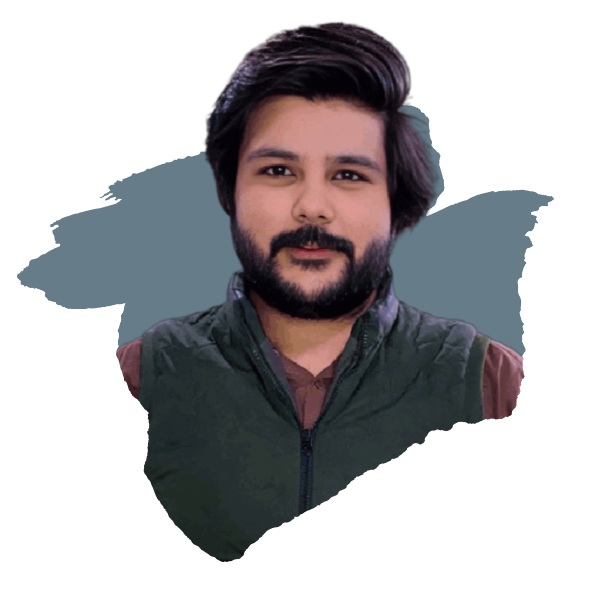
Table of contents
- Math Object in JavaScript:
- Properties of Math objects:
- Methods of Math objects:
- 1. Math.round()
- 2. Math.ceil()
- 3. Math.floor()
- 4. Math.trunc()
- 5. Math.sign()
- 6. Math.pow()
- 7. Math.sqrt()
- 8. Math.abs()
- 9. Math.min()
- 10. Math.max()
- 11. Math.random()
- Real World Example of Math.random() Generating unique Id Like captcha:
- 12. Math.sin()
- Note:
- 13. Math.cos()
- 14. Math.tan()
- 15. Math.asin() (arcsine)
- 16. Math.acos() (arccosine)
- 17. Math.atan() (arctangent)
- 18. Math.atan2()
- 19. Math.sinh() (hyperbolic sine)
- 20. Math.cosh() (hyperbolic cosine)
- 21. Math.tanh() (hyperbolic tangent)
- 22. Math.asinh()
- 23. Math.acosh()
- 24. Math.atanh()
- 25. Math.cbrt()
- 26. Math.clz32() (Count Leading Zeros 32)
- 27. Math.exp()
- 28. Math.expm1()
- 29. Math.log()
- 30. Math.fround()
- Important Point:
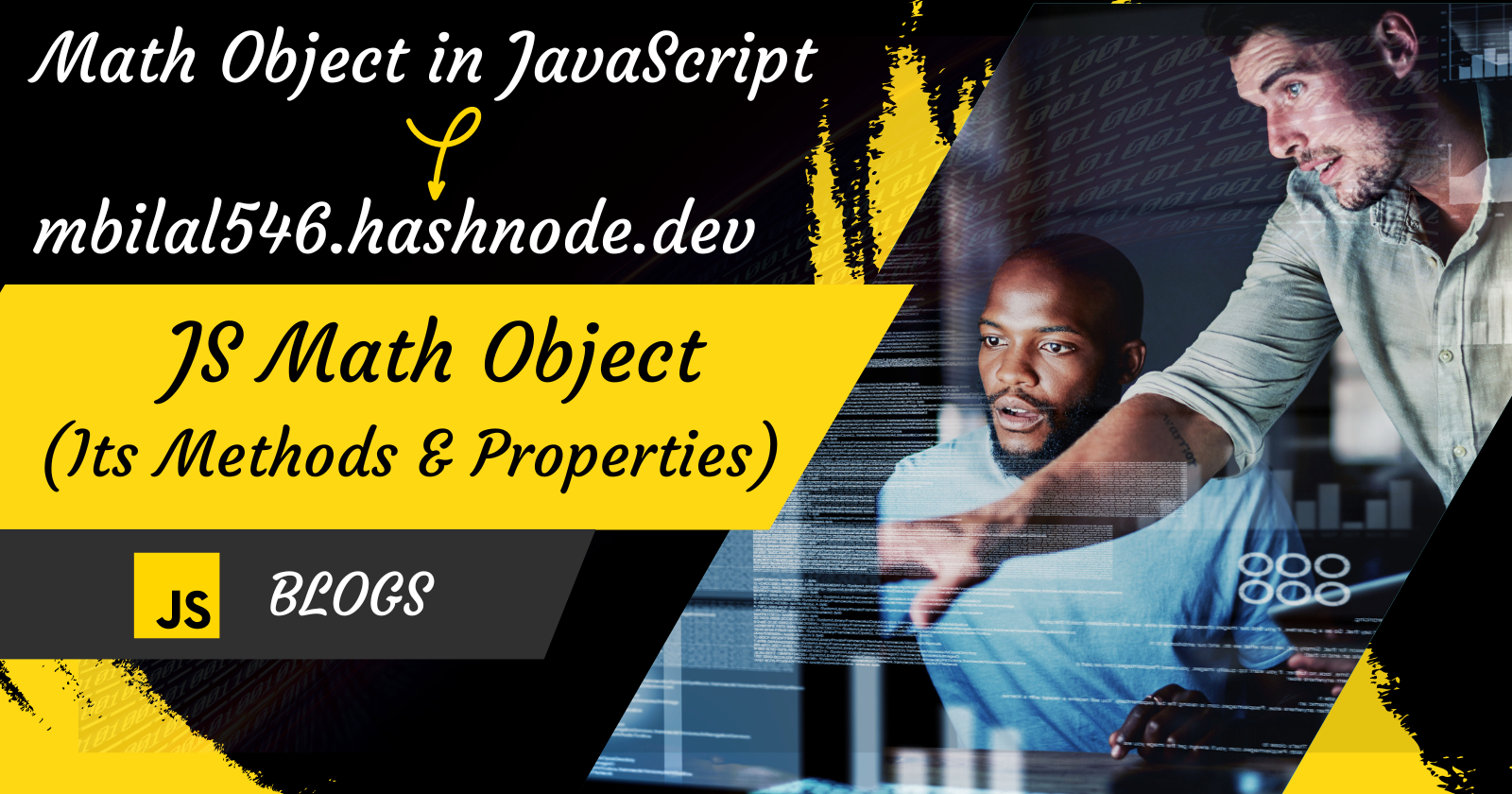
Math Object in JavaScript:
The Math
object in JavaScript is a built-in object that offers different mathematical functionalities. It is not a constructor function but a namespace with static methods and properties for mathematical constants and functions. Math
object has several properties and methods. Let us discuss them one by one.
Properties of Math objects:
The Math
object in JavaScript is a built-in object containing static properties for mathematical constants. It should be written as Math.property
.
1. Math.E
TheMath.E
property in JavaScript represents Euler's number, which is the base of natural logarithms. It is a mathematical constant approximately equal to 2.718281828459045
.
For Example:
const mathProperty = Math.E;
console.log(`The value of Math.E is : ${mathProperty}`);
Output: The value of Math.E is : 2.718281828459045
2. Math.PI
The Math.PI
property in JavaScript represents the mathematical constant pi (π), which is the ratio of a circle's circumference to its diameter. It is a fundamental constant used in various geometric and trigonometric calculations.
For Example:
const mathProperty = Math.PI;
console.log(`The value of Math.PI is : ${mathProperty}`);
Output: The value of Math.PI is : 3.141592653589793
// Or it can be written 3.14
3. Math.SQRT2
The Math.SQRT2
property in JavaScript represents the square root of 2, which is a mathematical constant approximately equal to 1.4142135623730951.
For Example:
const mathProperty = Math.SQRT2;
console.log(`The value of Math.SQRT2 is : ${mathProperty}`);
Output: The value of Math.SQRT2 is : 1.4142135623730951
4. Math.SQRT1_2
The Math.SQRT1_2
property in JavaScript represents the square root of 1/2, which is approximately equal to 0.7071067811865475
.
For Example:
const mathProperty = Math.SQRT1_2;
console.log(`The value of Math.SQRT1_2 is : ${mathProperty}`);
Output: The value of Math.SQRT1_2 is : 0.7071067811865476
5. Math.LN2
The Math.LN2
property in JavaScript represents the natural logarithm of 2, often denoted as ln(2). It is a mathematical constant with an approximately equal to 0.6931471805599453
.
For Example:
const mathProperty = Math.LN2;
console.log(`The value of Math.LN2 is : ${mathProperty}`);
Output: The value of Math.LN2 is : 0.6931471805599453
6. Math.LN10
In JavaScript, Math.LN10
is a static property of the Math
object that represents the natural logarithm of 10. It is a mathematical constant with an approximately equal to 2.302585092994046
.
For Example:
const mathProperty = Math.LN10;
console.log(`The value of Math.LN10 is : ${mathProperty}`);
Output: The value of Math.LN10 is : 2.302585092994046
7. Math.LOG2E
In JavaScript, Math.LOG2E
is a property of the Math
object that represents the base-2 logarithm of the mathematical constant "e" (Euler's number). It is used in various mathematical calculations involving logarithms and base 2. The value of Math.LOG2E
is approximately equal to 1.4426950408889634
.
For Example:
const mathProperty = Math.LOG2E;
console.log(`The value of Math.LOG2E is : ${mathProperty}`);
Output: The value of Math.LOG2E is : 1.4426950408889634
8. Math.LOG10E
In JavaScript, Math.LOG10E
is a property of the Math
object that represents the base-10 logarithm of the mathematical constant "e" (Euler's number). It is used in various mathematical calculations involving logarithms and base 10. The value of Math.LOG10E
is approximately 0.4342944819032518
.
For Example:
const mathProperty = Math.LOG10E;
console.log(`The value of Math.LOG10E is : ${mathProperty}`);
Output: The value of Math.LOG10E is : 0.4342944819032518
Methods of Math objects:
The Math
object in JavaScript is a built-in object containing static methods for mathematical functions. It should be written as Math.method(any value)
.
1. Math.round()
The Math.round()
method is a built-in function in JavaScript that rounds a number to the nearest integer. It is a static method of the Math
object, meaning you can access it directly without creating an instance. It rounds a number to the nearest integer:
Values less than or equal to 0.5 are rounded down.
Values greater than 0.5 are rounded up.
Values of -0.5 are rounded down.
For Example:
const mathMethod = Math.round(5.62); // Argument 5.62
console.log(`The value of Math.round is : ${mathMethod}`);
Output: The value of Math.round is : 6
const mathMethod = Math.round(0.5); // Argument 0.5
console.log(`The value of Math.round is : ${mathMethod}`);
Output: The value of Math.round is : 1
const mathMethod = Math.round(-0.5); // Argument -0.5
console.log(`The value of Math.round is : ${mathMethod}`);
Output: The value of Math.round is : 0
2. Math.ceil()
The Math.ceil()
method is a built-in function in JavaScript that rounds a number up to the nearest integer. It is a static method of the Math
object, meaning you can access it directly without creating an instance. It always rounds away from zero.
For Example:
const mathMethod = Math.ceil(5.26); // As it rounds number up so,
// it does't matter after decimal number is 5 or greater than 5
// It always round up
console.log(`The value of Math.ceil is : ${mathMethod}`);
Output: The value of Math.ceil is : 6
3. Math.floor()
The Math.floor()
method is a built-in function in JavaScript that rounds a number down to the nearest integer. Similar to other Math methods, it is a static method of the Math object, accessible directly without creating an instance. It always rounds toward zero.
For Example:
const mathMethod = Math.floor(5.66); // As it rounds number down so,
// it does't matter after decimal number is 5 or greater than 5.
// It always round down
console.log(`The value of Math.floor is : ${mathMethod}`);
Output: The value of Math.floor is : 5
4. Math.trunc()
TheMath.trunc()
method is a recent addition (ES6) to JavaScript that offers a simple way to truncate a number to its integer part. It removes the decimal part of a number, effectively "truncating" it to the nearest integer. It works regardless of whether the number is positive, negative, or zero. It offers a more concise and efficient alternative to achieving the same outcome with other methods, likeMath.floor()
.
For Example:
const mathMethod = Math.trunc(0.66);
console.log(`The value of Math.trunc is : ${mathMethod}`);
Output: The value of Math.trunc is : 0
const mathMethod = Math.trunc(5.66);
console.log(`The value of Math.trunc is : ${mathMethod}`);
Output: The value of Math.trunc is : 5
const mathMethod = Math.trunc(-3.46);
console.log(`The value of Math.trunc is : ${mathMethod}`);
Output: The value of Math.trunc is : -3
5. Math.sign()
TheMath.sign()
method is a built-in function in JavaScript that returns the sign of a number. It returns1
for positive numbers, -1
for negative numbers, and 0
for zero.
For Example:
const mathMethod = Math.sign(3.46); // For positive number
console.log(`The value of Math.sign is : ${mathMethod}`);
Output: The value of Math.sign is : 1
const mathMethod = Math.sign(-3.46); // For negative number
console.log(`The value of Math.sign is : ${mathMethod}`);
Output: The value of Math.sign is : -1
const mathMethod = Math.sign(0); // For zero
console.log(`The value of Math.sign is : ${mathMethod}`);
Output: The value of Math.sign is : 0
6. Math.pow()
The Math.pow()
method is a built-in function in JavaScript that raises a number to a power. It is a static method of the Math
object, meaning you can access it directly without creating an instance.
It takes two arguments:
base
: The number to be raised to the power.
exponent
: The power to which the base is raised.
it returns a number representing the base raised to the exponent.
For Example:
const mathMethod = Math.pow(2, 5); // It means 2 x 2 x 2 x 2 x 2 = 32
console.log(`The value of Math.pow is : ${mathMethod}`);
Output: The value of Math.pow is : 32
7. Math.sqrt()
The Math.sqrt()
method in JavaScript is a built-in function that calculates the square root of a non-negative number. It returns NaN
if the input is negative. It is used for calculations involving complex numbers or negative numbers requiring imaginary roots, consider using libraries that support complex numbers.
For Example:
const mathMethod = Math.sqrt(49);
console.log(`The value of Math.sqrt is : ${mathMethod}`);
Output: The value of Math.sqrt is : 7
const mathMethod = Math.sqrt(-49);
console.log(`The value of Math.sqrt is : ${mathMethod}`);
Output: The value of Math.sqrt is : NaN // Means not a number
8. Math.abs()
The Math.abs()
method in JavaScript is a built-in function used to calculate the absolute value of a number. This means it returns the non-negative version of the provided number. It is used for error handling or checking for positive or negative values.
For Example:
const mathMethod = Math.abs(-10);
console.log(`The value of Math.abs is : ${mathMethod}`);
Output: The value of Math.abs is : 10
9. Math.min()
The Math.min()
method in JavaScript is used to find the smallest of the numbers passed as arguments. If no argument is passed then it returns infinity
. If the non-numeric argument is passed then it returns NaN
.
ForExample:
let minNum = Math.min(1, 21, 40, 35, 0, 22);
console.log(minNum);
Output: 0
10. Math.max()
The Math.max()
method in JavaScript is used to find the largest of the numbers passed as arguments. If no argument is passed then it returns infinity
. If the non-numeric argument is passed then it returns NaN
.
ForExample:
let maxNum = Math.max(1, 21, 40, 35, 0, 22);
console.log(maxNum);
Output: 40
11. Math.random()
The Math.random()
method in JavaScript is a powerful tool for generating random numbers. It returns a floating-point number between 0 (included) and 1 (not included).
For Example:
const generateRandom = Math.random();
console.log(generateRandom);
Output: 0.31660780905155805
// On every referesh it return different number between 0 and 1
// If you want a number without decimal then you have to round off it
const generateRandom = Math.round(Math.random() * 10);
console.log(generateRandom);
Output: This return different number from 0 to 10 on every referesh.
Real World Example of Math.random()
Generating unique Id Like captcha:
function generateRandomChar(length = 6) {
const characters = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
let randomCharacters = "";
for (let i = 0; i < length; i++) {
randomCharacters += characters[Math.floor(Math.random() * characters.length)];
}
return randomCharacters;
}
const generateRandom = generateRandomChar();
console.log(generateRandom);
// You can test it. Also, you can change its length = 6
// You can add special characters set "!@#$%^&*()" into it.
12. Math.sin()
The Math.sin()
method in JavaScript is a built-in function that calculates the sine of an angle. However, it's crucial to note that this function expects the angle to be in radians, not degrees. Values range between -1 (for sine of -π/2) and 1 (for sine of π/2).
For Example:
// As you know PI is the static value so we can access is by Math.PI
const mathMethod = Math.sin(Math.PI / 2);
console.log(`The value of Math.sin is : ${mathMethod}`);
Output: The value of Math.sin is : 1
const mathMethod = Math.sin(- Math.PI / 2);
console.log(`The value of Math.sin is : ${mathMethod}`);
Output: The value of Math.sin is : -1
Note:
It takes value angle in radians. If you need to convert from degrees to radians before using Math.sin()
, you can use the formula: radians = degrees * Math.PI / 180
.
Libraries and frameworks often provide helper functions to perform calculations with different units.
13. Math.cos()
The Math.cos()
method in JavaScript is a built-in function that calculates the cosine of an angle. Similarly Math.sin()
, it requires the angle to be in radians, not degrees. Values range between -1 (for cosine of π) and 1 (for cosine of 0).
For Example:
const mathMethod = Math.cos(0);
console.log(`The value of Math.cos is : ${mathMethod}`);
Output: The value of Math.cos is : 1
const mathMethodDeg = Math.cos(30);
// Here 30 is in degree but this method accept value in radian
// To convert that into the radian
const mathMethodRad = Math.cos(30 * Math.PI / 180);
console.log(`The value of Math.cos is : ${mathMethodRad}`);
Output: The value of Math.cos is : 0.866
14. Math.tan()
The Math.tan()
method in JavaScript is used to calculate the tangent of a given angle. It takes one argument, which is the angle in radians.
For Example:
// Get the tangent of 30 degrees
const angleInRadians = Math.tan(30 * (Math.PI / 180));
console.log(`The tangent of 30 degree is: ${angleInRadians}`);
Output: The tangent of 30 degree is: 0.57
15. Math.asin() (arcsine)
The Math.asin()
method in JavaScript returns the inverse sine (arcsine) of a number in radians. It takes one argument, a number between -1
and 1
, representing the sine value. It returns the angle in radians between -π/2
and π/2
. It returns NaN
if the argument is less than -1
or greater than 1
.
For Example:
const asinNum = Math.asin(1);
console.log(asinNum);
Output: 1.570796
// If value is greater than 1
const asinNum = Math.asin(1.5);
console.log(asinNum);
Output: NaN
16. Math.acos() (arccosine)
The Math.acos()
method in JavaScript returns the inverse cosine (arccosine) of a number in radians. It takes one argument, a number between -1
and 1
, representing the cosine value. It returns the angle in radians between 0 and π. It returnsNaN
if the argument is less than -1
or greater than 1
.
For Example:
const acosNum = Math.acos(1);
console.log(acosNum);
Output: 0
// If value is greater than 1
const acosNum = Math.acos(1.5);
console.log(acosNum);
Output: NaN
17. Math.atan() (arctangent)
The Math.atan()
method in JavaScript calculates the arctangent of a number, which is the inverse of the tangent function. It takes one argument, representing the tangent value. It returns the angle in radians between -π/2
and π/2
. If the argument is Infinity
, the return value is π/2
. If the argument is -Infinity
, the return value is -π/2
. It returns NaN
if the argument is not a number.
For Example:
const atanNum = Math.atan(Infinity);
console.log(atanNum);
Output: 1.5707963267948966
// If argument is not a number
const atanNum = Math.atan('Hello');
console.log(atanNum);
Output: NaN
18. Math.atan2()
The Math.atan2()
method in JavaScript is a powerful tool for calculating angles in the plane based on two coordinates. It takes two arguments: The y-coordinate
of the point. That x-coordinate
of the point. It returns the angle in radians between -π
and π
. It handles special cases like quadrant changes and zero coordinates.
For Example:
const atan2Num = Math.atan2(0, 0);
console.log(atan2Num);
Output: 0
const atan2Num = Math.atan2(-3, -4);
console.log(atan2Num);
Output: -2.498091544796509
19. Math.sinh() (hyperbolic sine)
The Math.sinh()
method in JavaScript returns the hyperbolic sine of a number. The hyperbolic sine of a number is defined as:sinh(x) = (e^x - e^(-x)) / 2
. It returns the hyperbolic sine of the given number. It takes one argument, representing the number. It returns a number. It returns NaN
if the argument is not a number.
For Example:
const sinhNum = Math.sinh(0);
console.log(sinhNum);
Output: 0
// If argument is not a number
const sinhNum = Math.sinh('Hello');
console.log(sinhNum);
Output: NaN
20. Math.cosh() (hyperbolic cosine)
The Math.cosh()
method in JavaScript calculates the hyperbolic cosine of a number. It is defined as: cosh(x) = (e^x + e^(-x)) / 2
. It returns the hyperbolic cosine of a number. It takes one argument, representing the number. It returns a number. It returns NaN
if the argument is not a number.
For Example:
const coshNum = Math.cosh(0);
console.log(coshNum);
Output: 1
// If argument is not a number
const coshNum = Math.cosh('Hello');
console.log(coshNum);
Output: NaN
21. Math.tanh() (hyperbolic tangent)
The Math.tanh()
method in JavaScript calculates the hyperbolic tangent of a number. It is defined as: tanh(x) = sinh(x) / cosh(x)
. It returns the hyperbolic tangent of a number. It takes one argument, representing the number. It returns a number between -1
and 1
. It returns NaN
if the argument is not a number.
For Example:
const tanhNum = Math.tanh(1);
console.log(tanhNum);
Output: 0.7615941559557649
// If argument is not a number
const tanhNum = Math.tanh('Hello');
console.log(tanhNum);
Output: NaN
22. Math.asinh()
The Math.asinh()
method in JavaScript calculates the inverse hyperbolic sine (or arcsinh) of a number. It's essentially the opposite of the Math.sinh()
function. It returns the angle whose hyperbolic sine is the given number. It takes one argument, representing the number. It is available since ECMAScript 6 (ES6). If you need to support older browsers, you might need to use a polyfill. It takes the value range of -Infinity
to Infinity
. It returns the output range is also
-Infinity
to Infinity
.
For Example:
const asinhNum = Math.asinh(1);
console.log(asinhNum);
Output: 881373587019543
// If argument is infinity
const asinhNum = Math.asinh(Infinity);
console.log(asinhNum);
Output: Infinity
23. Math.acosh()
The Math.acosh()
method in JavaScript calculates the inverse hyperbolic cosine (arccosh) of a number. It's the inverse of the Math.cosh()
function. It returns the angle whose hyperbolic cosine is the given number. It takes one argument, representing the number. It returns the angle in radians between 0
and Infinity
. It returns NaN
if the argument is less than 1
.
For Example:
const acoshNum = Math.acosh(1);
console.log(acoshNum);
Output: 0
// If argument is less then 1
const acoshNum = Math.acosh(-1);
console.log(acoshNum);
Output: NaN
24. Math.atanh()
The Math.atanh()
method in JavaScript returns the inverse hyperbolic tangent (also known as the arctanh) of a number. It takes a single number parameter, which should be between -1
and 1
(inclusive). It returns the hyperbolic arctangent of the provided number. If the argument is 1
, it returns Infinity
. If the argument is -1
, it returns -Infinity
.
For Example:
const atanhNum = Math.atanh(0.5);
console.log(atanhNum);
Output: 0.5493061443340548
// If argument is 1
const atanhNum = Math.atanh(1);
console.log(atanhNum);
Output: Infinity
// If argument is -1
const atanhNum = Math.atanh(1);
console.log(atanhNum);
Output: -Infinity
25. Math.cbrt()
The Math.cbrt()
method is a built-in function in JavaScript that returns the cube root of a number. In other words, it finds the value that, when multiplied by itself three times, equals the given number. Math.cbrt()
is an ECMAScript 6 (ES6) feature. It is not supported in Internet Explorer 11 (or earlier). It returns the floating point numbers.
For Example:
const cbrtNum = Math.cbrt(125);
console.log(cbrtNum);
Output: 5
26. Math.clz32() (Count Leading Zeros 32)
The Math.clz32()
method is a built-in function in JavaScript that returns the number of leading zero bits in the 32-bit binary representation of a number. It takes a single argument number, If the argument is not a number, it will be converted to a number first. The number is then converted to an 32-bit
unsigned integer. The function then counts the number of leading zero bits
in the binary representation of the 32-bit
unsigned integer. The number of leading zeros in the 32-bit
binary representation of the number. If the number is 0
, it returns 32
(all bits are 0
). The Math.clz32()
the method is not supported by all browsers. It is recommended to use a polyfill if you need to support older browsers. There is also a Math.imul()
method that can be used to perform bitwise operations.
For Example:
const clz32Num = Math.clz32(0);
console.log(clz32Num);
Output: 32
const clz32Num = Math.clz32(1);
console.log(clz32Num);
Output: 31
// with non-numeric argument
const clz32Num = Math.clz32('100');
console.log(clz32Num);
Output: 25
27. Math.exp()
The Math.exp()
method is a built-in function in JavaScript that returns the natural exponential (e raised to the power of x) of a number. In other words, it calculates e^x
, where e is Euler's constant (approximately 2.71828
). If the argument is not a number, it will be converted to a number first. The function then calculates the natural exponential of the exponent. A non-negative number representing e^x
, where e
is the base of the natural logarithm. It is supported by all browsers.
For Example:
const expNum = Math.exp(1);
console.log(expNum);
Output: 2.718281828459045
const expNum = Math.exp(-2);
console.log(expNum);
Output: 0.1353352832366127
28. Math.expm1()
The Math.expm1()
function is a built-in JavaScript function that calculates the value of e^x - 1
, where: e
is Euler's number (approximately 2.7183
) and x
is the number passed as an argument to the function. This function is more accurate than calculating Math.exp(x) - 1
for small values of x
. This is because directly subtracting 1
can lead to rounding errors and loss of precision. This function accepts any number as an argument. However, for very small values of x
, adding 1 (to get e^x)
can reduce or eliminate precision due to limitations in the double-precision floating-point numbers used in JavaScript.
For Example:
const expm1Num = Math.expm1(1);
console.log(expm1Num);
Output: 1.718281828459045
const expm1Num = Math.expm1(0);
console.log(expm1Num);
Output: 0
29. Math.log()
The Math.log()
method in JavaScript returns the natural logarithm (base e) of a number. It is equivalent to the ln(x)
function in mathematics. If x
is less than 0
, it returns NaN
and if x
is 0
, it returns -Infinity
.
For Example:
const logNum = Math.log(10);
console.log(logNum);
Output: 2.302585092994046
// If argument (x) is 0
const logNum = Math.log(0);
console.log(logNum);
Output: -Infinity
// If argument (x) is less than 0
const logNum = Math.log(-10);
console.log(logNum);
Output: NaN
It only calculates the natural logarithm (base e)
. If you need the logarithm with a different base, you will need to use a different function or calculate it manually.
Due to floating-point precision limitations
, the results may not be perfectly accurate.
30. Math.fround()
Math.fround()
is a static method in JavaScript that returns the nearest 32-bit
single-precision float representation of a number. It is useful for situations where memory usage is a concern and the full precision of a 64-bit
double-precision float is not required. It can be used to improve performance in some cases, as single-precision operations are generally faster than double-precision operations.
For Example:
const froundNum = Math.fround(1.9999999999999998);
console.log(froundNum);
Output: 2
const froundNum = Math.fround(Math.PI);
console.log(froundNum);
Output: 3.14
Important Point:
The original value of the number is not modified. Math.fround()
can cause precision loss, especially for large numbers. You can use Math.round()
if you need to round a number to the nearest integer.
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
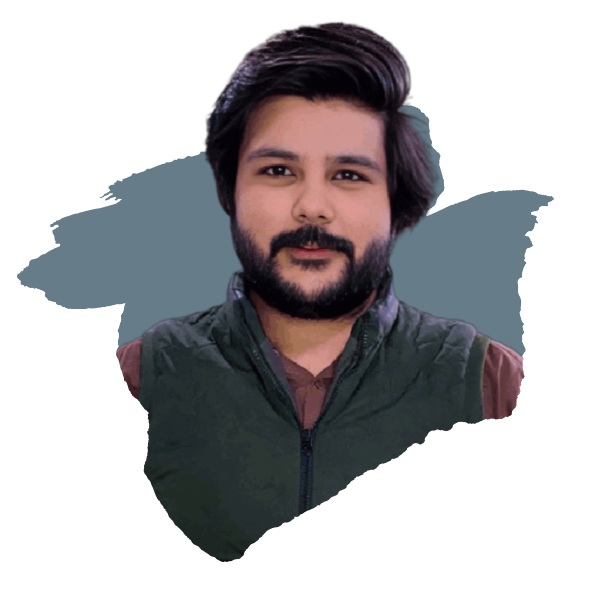
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. 📈 Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. 🔊 Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub 🖥 Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. ✨ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. 🎓 Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. 👥 Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.