How to build Docker image of Golang project?
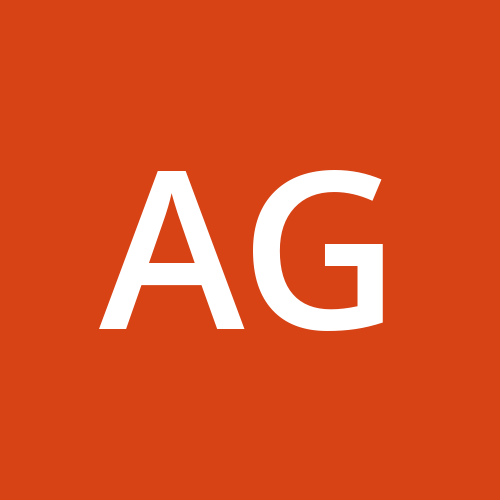
Requirements
Go version 1.19 or later. Visit the download page for Goopen_in_new first and install the toolchain.
Docker running locally. Follow the instructions to download and install Docker.
An IDE or a text editor to edit files. Visual Studio Codeopen_in_new is a free and popular choice but you can use anything you feel comfortable with.
A Git client. This guide uses a command-line based
git
client, but you are free to use whatever works for you.A command-line terminal application. The examples shown in this module are from the Linux shell, but they should work in PowerShell, Windows Command Prompt, or OS X Terminal with minimal, if any, modifications.
Steps :
Create .dockerignore file.
This file created to ignore those file which we don't want to push to docker registry. It is same like .gitignore file which is created to ignore the files from git directory.
/vendor /.gitignore /.vscode
Create a Dockerfile for application.
To build a container image with Docker, a
Dockerfile
with build instructions is required.a. Tell Docker what base image you would like to use for your application:
FROM golang:1.19
Docker image is inherited from official Go image that already has all necessary tools and libraries to compile and run a Go application.
b. Create directory inside the image that you are building. This instructs Docker to use this directory as the default destination for all subsequent commands.
WORKDIR /app
c. Usually the very first thing you do once you’ve downloaded a project written in Go is to install the modules necessary to compile it. Note, that the base image has the toolchain already, but your source code isn't in it yet.
So before you can run
go mod download
inside your image, you need to get yourgo.mod
andgo.sum
files copied into it. Use theCOPY
command to do this.COPY source dest
COPY go.mod go.sum ./
COPY
command takes two parameters. The first parameter tells Docker what files you want to copy into the image. The last parameter tells Docker where you want that file to be copied to.you have the module files inside docker image so now you can run using run command below. This works exactly the same as if you were running
go
locally on your machine, but this time these Go modules will be installed into a directory inside the image.RUN go mod download
At this point, you have a Go toolchain version 1.19.x and all your Go dependencies installed inside the image.
4. Next you need to copy your source code to docker image.
COPY source dest
COPY . .
COPY .env .env
This command copy all the .go extension file from your current directory of host (the directory where the Dockerfile
is located) into docker image directory.
5. Compile your Go application for container.
Go create single binary file to run inside container which doesn't need additional runtimes or dependencies.
RUN CGO_ENABLED=0 go build -o /image-name
CGO_ENABLED=0
: Enables statically linked binaries to make the application more portable. It allows you to use the binary with source images that don’t support shared libraries when building your container image.
GOOS=linux
: Since containers run Linux, set this option to enable repeatable builds even when building the application on a different platform.
optional : To bind to a TCP port, runtime parameters must be supplied to the docker command.
Define the network port
s that this container will listen on at runtime.
EXPOSE 8080
Now, It need to tell Docker what command to run when your image is used to start a container.CMD [ "executable" ]
CMD [ "/image-name" ]
Build the image
Now that you've created your Dockerfile
, build an image from it. The docker build
command creates Docker images from the Dockerfile
and a context.
The build command optionally takes a --tag
flag. This flag is used to label the image with a string value, which is easy for humans to read and recognise. If you don't pass a --tag
, Docker will use latest
as the default value.
Build your first Docker image.
docker build --tag image-name .
The build process will print some diagnostic messages as it goes through the build steps. you should see the word FINISHED
in the first line of output. This means Docker has successfully built your image named image-name
.
Create a docker container by running
docker run -d -it –-rm -p [host_port]:[container_port] --name [container_name] [image_id/image_tag]
Now, you can test using postman as :
http://localhost:[host_port]
View local images
To see the list of images you have on your local machine, you have two options. One is to use the CLI and the other is to use Docker Desktop.
To list images, run the docker image ls
command , you will able to see the docker image image-name
Subscribe to my newsletter
Read articles from Aashutosh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
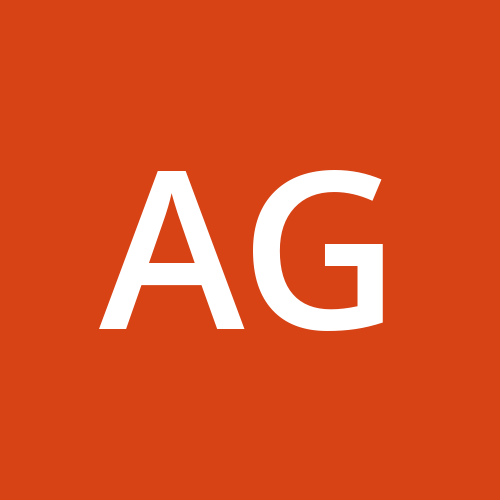