Unveiling the Differences: a_dic.keys() vs. list(a_dic) in Python
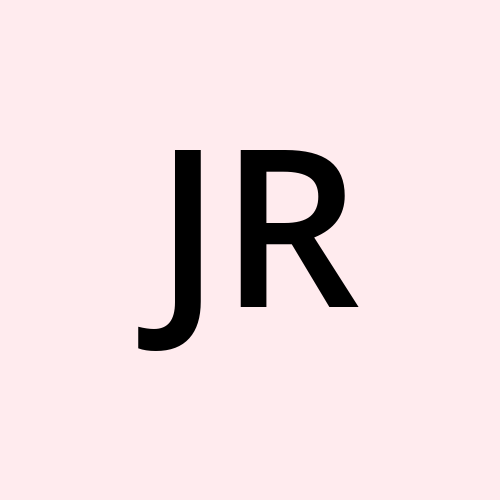
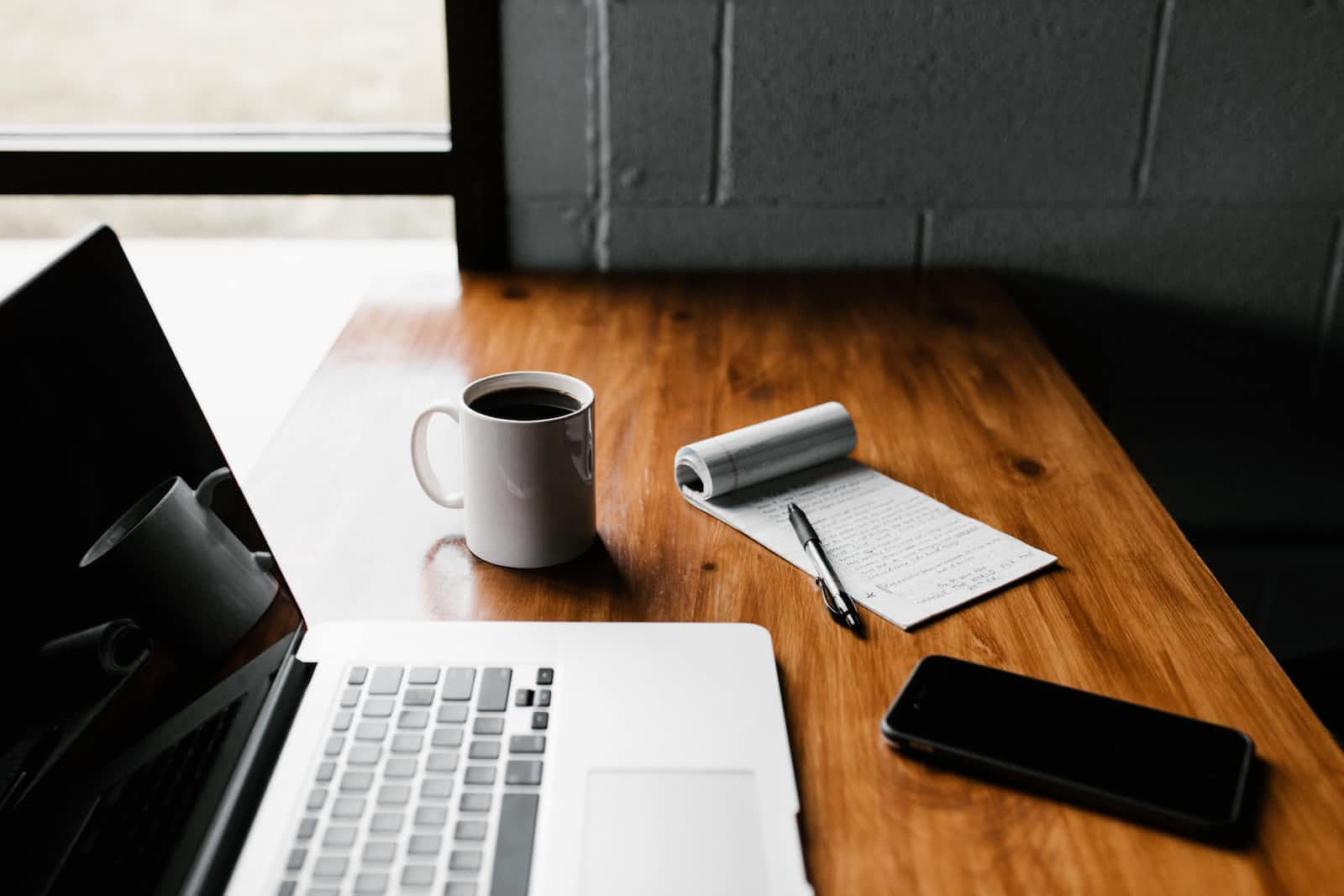
In Python we often encounter situations where we need to extract a list of keys from a dictionary. While both dic.keys()
and list(dic)
achieve this seemingly identical task, they operate in subtly different ways, with distinct implications for performance and functionality.
Nature of the Object:
dic.keys()
: This method returns a dictionary view object, which reflects the current state of the dictionary. It remains linked to the original dictionary, automatically updating as the dictionary changes.list(dic)
: This approach converts the dictionary keys into a regular Python list. The list is a snapshot of the keys at the time of creation and will not reflect any subsequent changes made to the dictionary.
Performance:
dic.keys()
: Accessing elements through a dictionary view is generally considered faster than iterating over a list. This is because the view object is dynamically linked to the dictionary, avoiding the overhead of creating and maintaining a separate data structure.list(dic)
: Creating a list involves copying the dictionary keys, which can be slower for larger dictionaries. Additionally, modifying the original dictionary after creating the list will not affect the list itself, potentially leading to inconsistencies and unexpected behavior.
Memory Consumption:
dic.keys()
: Dictionary views generally have a smaller memory footprint compared to lists. This is because they only store a reference to the original dictionary, instead of duplicating the data.list(dic)
: Creating a list consumes additional memory, as it allocates space for storing the copied key values. This can be a significant concern for memory-constrained environments.
Use Cases:
dic.keys()
: Ideal for scenarios where you need a dynamic view of the dictionary keys. This is useful for iterating over the keys, checking for key existence, or performing operations that require the latest state of the dictionary.list(dic)
: This approach is suitable when you need a static snapshot of the dictionary keys at a specific point in time. This is useful for passing the list to other functions, preserving the key order, or using the list for further processing without affecting the original dictionary.
Additional Considerations:
For Python versions 3.7 and above,
dic.keys()
can be used directly in afor
loop, eliminating the need to explicitly convert it to a list.dict.fromkeys()
can be used to create a new dictionary with a list of keys. This is useful for initializing a dictionary with specific keys without assigning values initially.
Conclusion:
Choosing between dic.keys()
and list(dic)
depends on your specific needs and the context of your code. While both methods retrieve the dictionary keys, understanding their underlying differences allows you to make informed decisions and optimize your code for performance and efficiency.
Subscribe to my newsletter
Read articles from J. V. G. Ransika directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
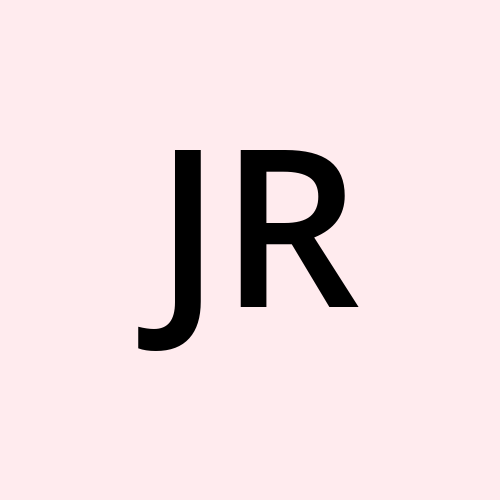