Arrays in JavaScript - Methods for JavaScript Arrays

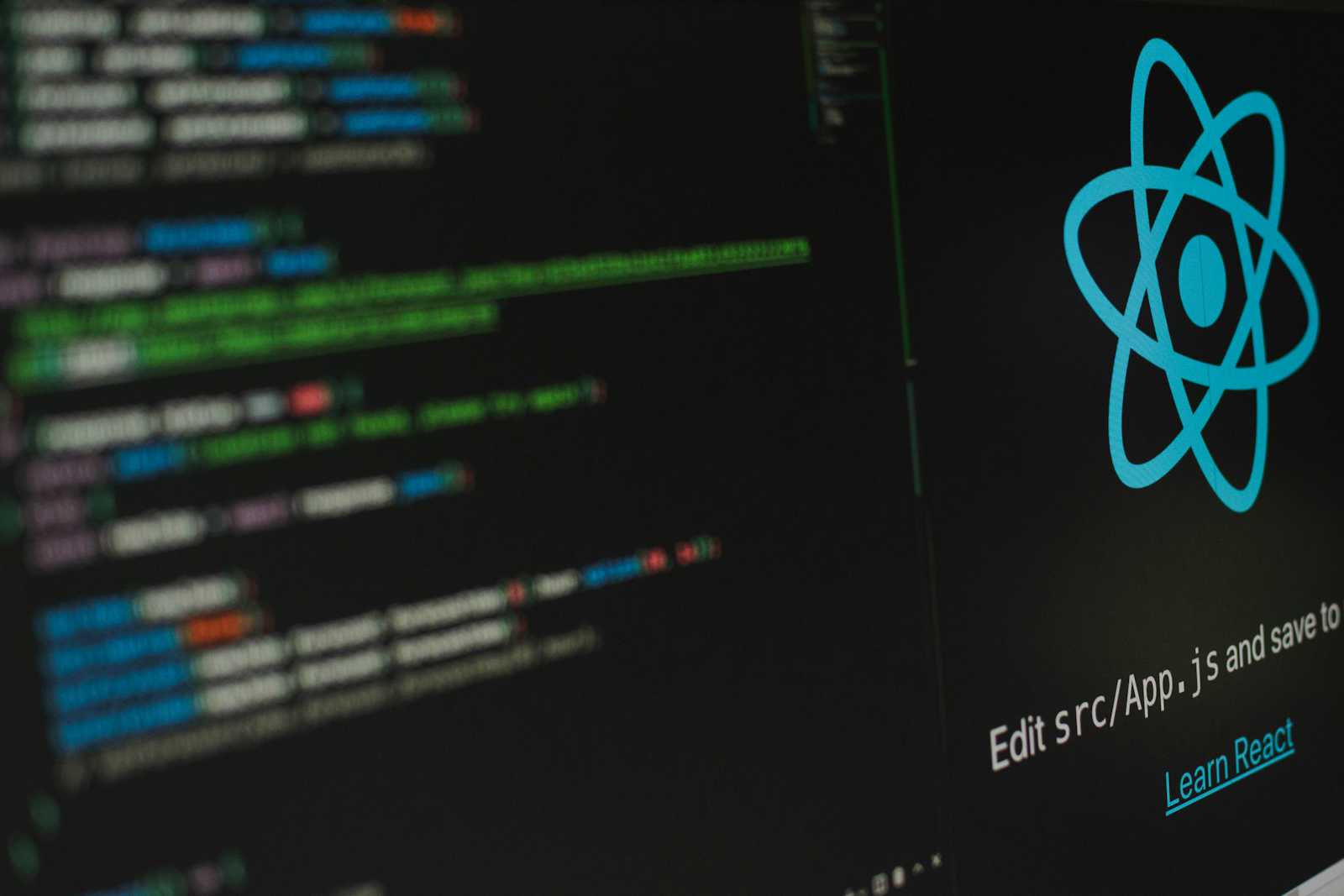
Arrays play a fundamental role in JavaScript, offering a versatile and dynamic way to store and manipulate data. In this article, we will delve into the world of JavaScript arrays, focusing on essential array methods that empower developers to efficiently handle and transform data.
Understanding JavaScript Arrays
At its core, an array is a data structure that allows you to store multiple values within a single variable. In JavaScript, arrays can hold various data types, making them incredibly flexible.
Creating Arrays
Before we dive into array methods, let's explore how to create arrays in JavaScript:
// Creating an array of numbers
let numbers = [1, 2, 3, 4, 5];
// Creating an array of strings
let fruits = ['apple', 'orange', 'banana'];
// Creating a mixed-type array
let mixedArray = [1, 'hello', true, null];
Essential JavaScript Array Methods
1. push()
and pop()
The push()
method adds one or more elements to the end of an array, while pop()
removes the last element from the array.
let colors = ['red', 'green', 'blue'];
colors.push('yellow'); // Adds 'yellow' to the end
let removedColor = colors.pop(); // Removes and returns 'blue'
2. shift()
and unshift()
shift()
removes the first element from an array, and unshift()
adds one or more elements to the beginning.
let cities = ['New York', 'Paris', 'Tokyo'];
cities.shift(); // Removes 'New York'
cities.unshift('London'); // Adds 'London' to the beginning
3. slice()
The slice()
method extracts a portion of an array and returns a new array without modifying the original.
let numbers = [1, 2, 3, 4, 5];
let slicedNumbers = numbers.slice(1, 4); // Returns [2, 3, 4]
4. splice()
splice()
is used to add or remove elements from a specific index in an array.
let fruits = ['apple', 'orange', 'banana'];
fruits.splice(1, 1, 'grape', 'kiwi'); // Removes 'orange' and adds 'grape' and 'kiwi'
5. map()
The map()
method creates a new array by applying a function to each element of the original array.
let numbers = [1, 2, 3, 4, 5];
let doubledNumbers = numbers.map(num => num * 2); // Returns [2, 4, 6, 8, 10]
6. filter()
filter()
creates a new array with elements that pass a specific condition.
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(num => num % 2 === 0); // Returns [2, 4]
Conclusion
JavaScript array methods provide powerful tools for manipulating data efficiently. By mastering these methods, developers can streamline their code and enhance the functionality of their applications. Whether you're a beginner or an experienced developer, a solid understanding of JavaScript arrays and their methods is crucial for building robust and dynamic web applications.
Subscribe to my newsletter
Read articles from Awais Ahmad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Awais Ahmad
Awais Ahmad
Software Engineer, Dev-ops, AWS