Find the Maximum Product of Two Elements in an Array
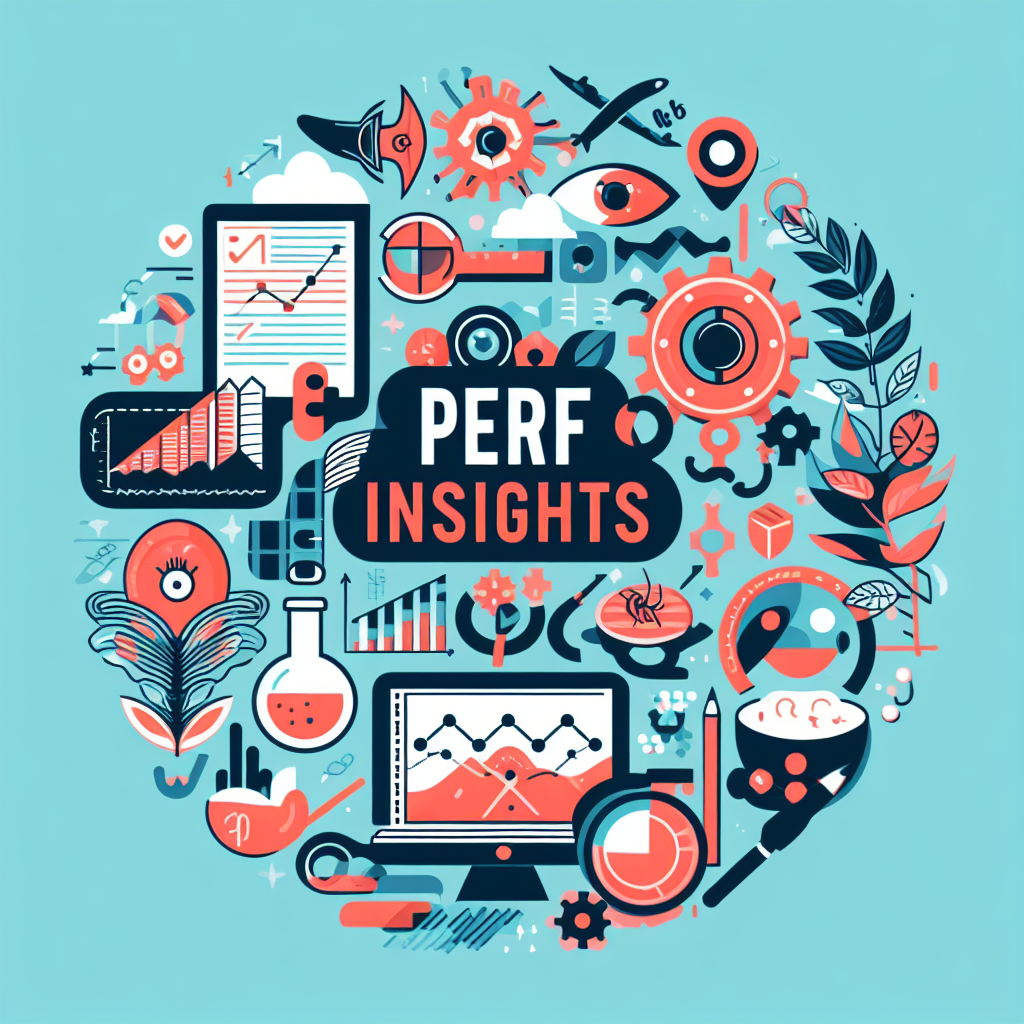
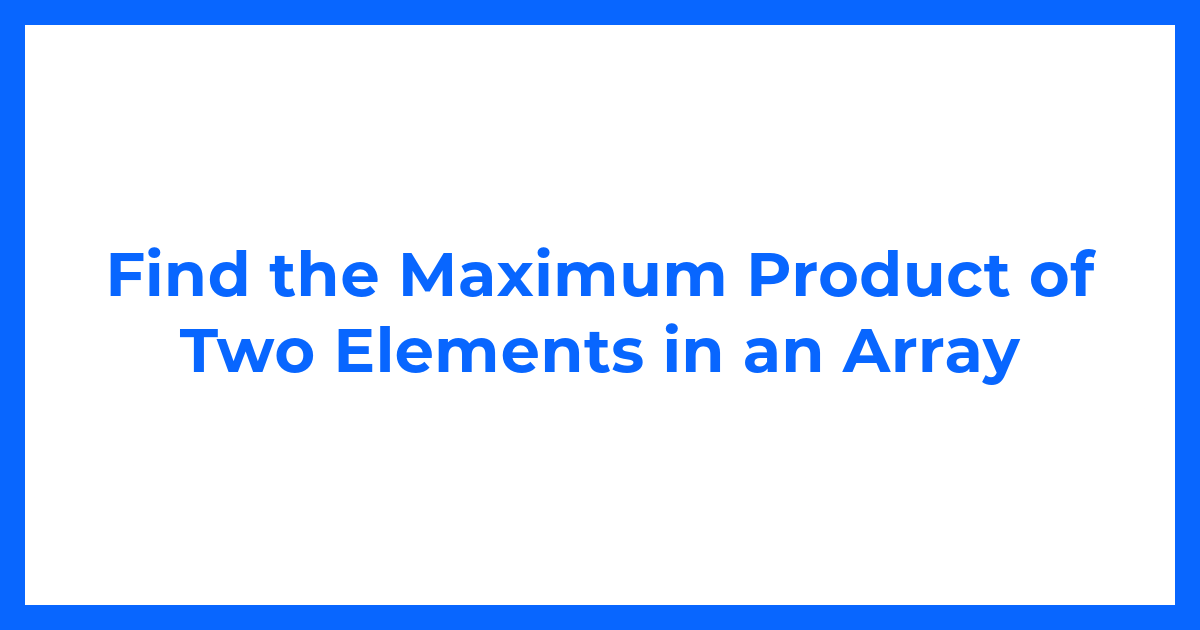
Given the array of integers nums
, you will choose two different indices i
and j
of that array. Return the maximum value of (nums[i]-1)*(nums[j]-1)
.
LeetCode Problem: Link | Click Here
class Solution {
public int maxProduct(int[] nums) {
// Initialize variables
int startVal = (nums[0] - 1) * (nums[1] - 1); // Calculate product of first two elements minus 1
int len = nums.length; // Get the length of the array
int finalVal = 0; // Initialize variable to store the final maximum product
int preFinal = 0; // Temporary variable to store intermediate products
// Check if array length is greater than 2
if (len > 2) {
// Loop through the array elements for product calculation
for (int i = 0; i < len - 1; i++) {
for (int j = i + 1; j < len; j++) {
preFinal = (nums[i] - 1) * (nums[j] - 1); // Calculate product of selected elements minus 1
if (preFinal > finalVal) {
finalVal = preFinal; // Update finalVal if preFinal is greater
}
}
}
return finalVal; // Return the maximum product
} else {
return startVal; // If array length is less than or equal to 2, return startVal
}
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
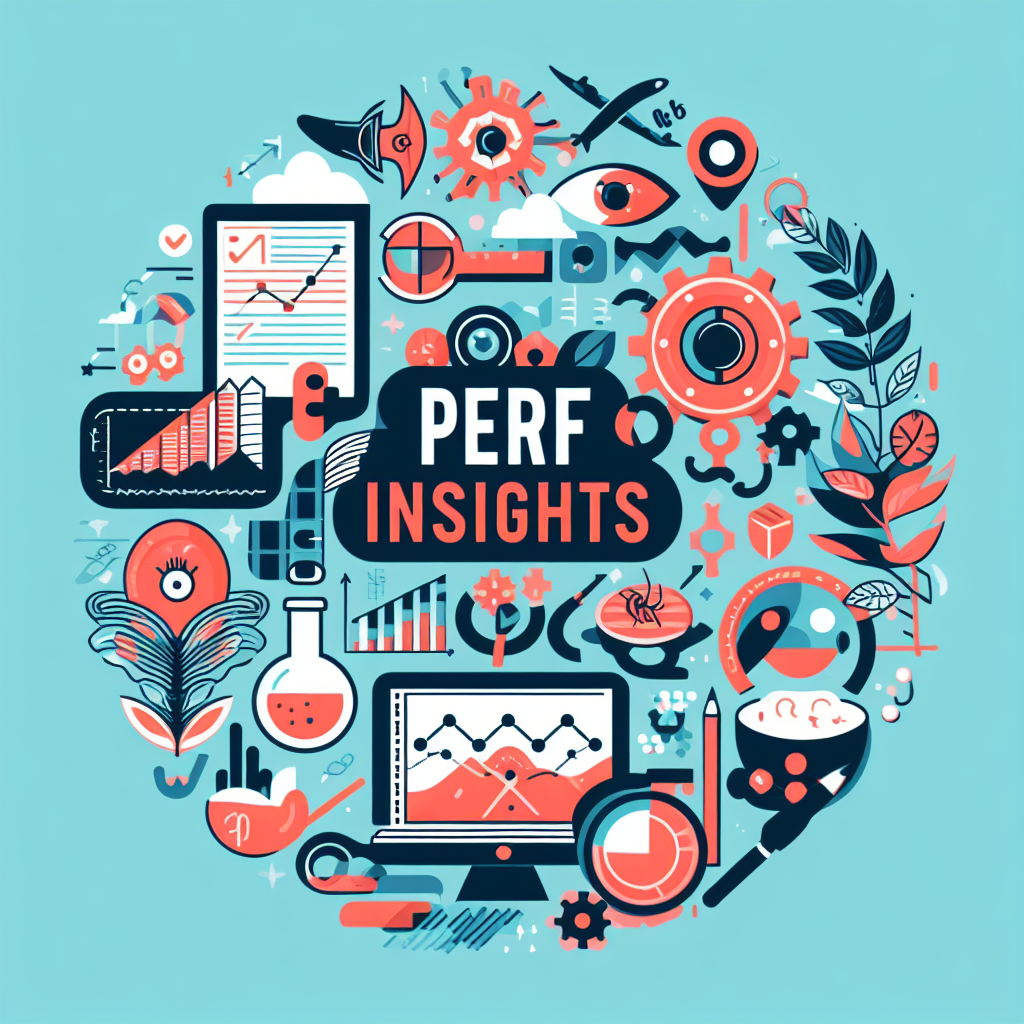
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.