Regression models for market prices predictions. Part 2
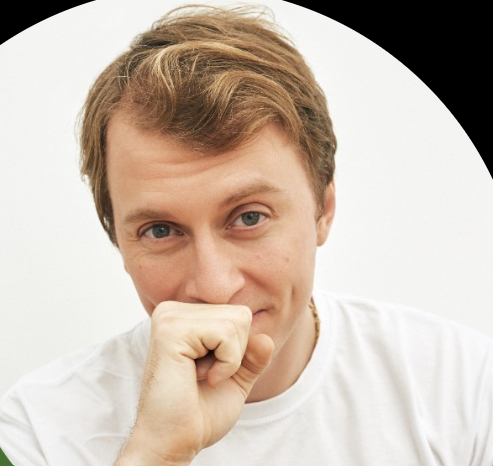
In the previous part of this article we discussed the basics of regression analysis, briefly reviewed data gathering and preprocessing techniques, and built a simple linear regression model. Toiday, we continue to dive deeper in the science of building efficient regression models for market price prediction.
Advanced Regression Techniques
Once you're comfortable with simple linear regression, you can continue to use advanced techniques to capture complex market behaviors and improve prediction accuracy. These advanced models can account for non-linear relationships, interaction terms, and other intricate dynamics often found in financial markets. Let’s explore some of these advanced methods!
Polynomial Regression
Polynomial regression extends linear regression by considering polynomial features of the input data. While it is capable of capturing non-linear trends, one must be cautious of overfitting, especially as the polynomial degree increases. In financial markets, Polynomial regression can be employed to model non-linear relationships such as volatility curves or growth trajectories.
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
poly = PolynomialFeatures(degree=2)
X_poly =
poly.fit
_transform(X_train)
poly_reg = LinearRegression()
poly_
reg.fit
(X_poly, y_train)
Handling Multicollinearity and Overfitting
Elastic Net Regression is a hybrid of Ridge and Lasso regressions, designed to optimize the penalty term by adding both L1 and L2 regularization components. It is particularly useful in financial applications where multicollinearity can often be an issue, such as when using multiple economic indicators to forecast financial markets.
from sklearn.linear_model import ElasticNet
elastic_net = ElasticNet(alpha=1.0, l1_ratio=0.5)
elastic_
net.fit
(X_train, y_train)
Principal Component Regression combines Principal Component Analysis (PCA) and Linear Regression. PCA is first applied to the independent variables, and the principal components obtained are then used to build a Linear Regression model. This reduces the impact of multicollinearity and can make the model more stable and interpretable. In financial applications, PCR is often used in portfolio optimization and risk management, where multicollinearity among different financial instruments can be problematic.
from sklearn.decomposition import PCA
from sklearn.linear_model import LinearRegression
pca = PCA(n_components=3)
X_train_pca =
pca.fit
_transform(X_train)
pcr = LinearRegression()
pcr.fit
(X_train_pca, y_train)
Ensemble Methods
Random Forest Regression is an ensemble learning method that leverages multiple decision trees during training and outputs the mean prediction of individual trees for regression problems. In financial markets, this approach is often employed in algorithmic trading strategies to predict asset prices, offering robustness against overfitting and the ability to handle a large number of features.
Gradient Boosting Regression leverages the power of ensemble learning by building multiple decision trees sequentially, each correcting the errors of its predecessor. Unlike Random Forest, Gradient Boosting focuses on the residuals – errors made by the previous trees – and aims to minimize them, making the model highly adaptive to the intricacies of the dataset. The method is especially useful in financial applications that demand high predictive accuracy.
from sklearn.ensemble import RandomForestRegressor, GradientBoostingRegressor
# Initialize and train the Random Forest model
rf_reg = RandomForestRegressor(n_estimators=100)
rf_
reg.fit
(X_train, y_train)
# Initialize and train the Gradient Boosting model
gb_reg = GradientBoostingRegressor(n_estimators=100)
gb_
reg.fit
(X_train, y_train)
By integrating these advanced regression techniques, traders and financial analysts can better adapt to the complexities and volatilities inherent in financial markets. The choice of method, of course, hinges on the specific problem at hand, underscoring the need for a thorough understanding of both the models and the market dynamics.
Evaluating and Tuning Regression Models
After implementing advanced regression techniques, the focus shifts to model evaluation and tuning. These critical steps ensure that your predictive model not only captures the complexity of financial markets but also performs reliably with new data. We delve into metrics that are particularly salient for trading strategies and discuss methodologies to fine-tune your models.
Metrics for Assessing Model Performance
In the financial domain, traditional metrics like Mean Squared Error or R-squared are often supplemented with trading-specific measures. The Sharpe Ratio, for example, calculates the risk-adjusted returns, allowing for a holistic view of the model's profitability versus its risk. Drawdown measures the largest single drop from peak to bottom in the value of a portfolio, providing insights into potential losses. Profit and Loss (PnL) tracks the model's ability to generate profit over a specific period.
# Calculating the Sharpe Ratio
sharpe_ratio = np.mean(returns) / np.std(returns) * np.sqrt(252)
Cross-Validation to Prevent Overfitting
Given the time-sensitive nature of financial data, time-series cross-validation is often more appropriate than standard k-fold cross-validation. This method involves creating multiple training/test splits so that all observations up to a time point t are used for training, while all observations after t are used for testing. This ensures that the temporal order of the data is respected.
from sklearn.model_selection import TimeSeriesSplit
tscv = TimeSeriesSplit(n_splits=5)
for train_index, test_index in tscv.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
Hyperparameter Tuning for Optimal Results
Hyperparameter tuning aims to find the optimal set of hyperparameters for your regression model. Techniques such as grid search or random search can systematically work through multiple combinations of parameter tunes, cross-validating as it goes to determine which tune gives the best performance.
from sklearn.model_selection import GridSearchCV
# Parameters for Random Forest
param_grid = {'n_estimators': [50, 100, 200],
'max_depth': [10, 20, 30],
'min_samples_split': [2, 5, 10]}
grid_search = GridSearchCV(RandomForestRegressor(), param_grid, cv=5)
grid_
search.fit
(X_train, y_train)
Understanding how to evaluate and tune your models is key to refining the performance of your trading strategy. The metrics and techniques demonstrated above are instrumental in achieving this, offering robust ways to assess the utility and reliability of your predictive models. Armed with a finely tuned model, you're in an excellent position to navigate the complex landscape of financial trading.
Predicting Market Prices
After you have mastered the mechanics of model building, evaluation, and tuning, the next step is to apply these regression models to real-world financial datasets. Here, we will take a case study approach, focusing on the USD/BRL currency pair, to illustrate the potential and pitfalls of employing regression models in the volatile domain of financial markets.
Application of Regression Models to Financial Datasets
Regression models are particularly adept at capturing the multifaceted dynamics of financial markets. Whether predicting stock prices, bond yields, or currency exchange rates, these models can harness the power of historical data and multiple features to forecast future price movements. In doing so, they serve as pivotal tools for traders and financial analysts, enabling evidence-based decision-making.
# Example of applying a Random Forest model to predict USD/BRL
rf_reg = RandomForestRegressor(n_estimators=100)
rf_
reg.fit
(X_train_USDBRL, y_train_USDBRL)
y_pred_USDBRL = rf_reg.predict(X_test_USDBRL)
A Case Study: Predicting the USD/BRL Currency Pair
The USD/BRL currency pair is influenced by a slew of factors—trade balances, interest rates, geopolitical developments, and more. For this case study, we used a Random Forest model, accounting for features like trading volume, economic indicators, and currency pair-specific factors like country risk premiums. The model showed promising results, accurately capturing trend movements, albeit with some limitations.
# Features for USD/BRL prediction
features_USDBRL = ['Trade_Balance', 'Interest_Rate_Differential', 'Risk_Premium']
X_train_USDBRL = df_train[features_USDBRL]
Y_train_USDBRL = df_train['USD/BRL']
# Model Training and Prediction
rf_reg_USDBRL = RandomForestRegressor(n_estimators=100)
rf_reg_
USDBRL.fit
(X_train_USDBRL, Y_train_USDBRL)
The Challenges and Limitations of Using Regression Models in Financial Markets
While regression models offer a robust methodology for price prediction, they are not without their limitations. Market conditions are continually evolving, and a model trained on past data may not necessarily adapt well to future conditions. Issues like overfitting, multicollinearity, and the “curse of dimensionality” can also plague even the most meticulously crafted models. Moreover, financial markets are susceptible to human behaviors, geopolitical events, and market sentiments that are often hard to quantify, posing additional challenges for predictive modeling.
Conclusion
The power and versatility of regression models in predicting market trends cannot be overstated. These tools, ranging from simple linear to advanced ensemble methods, offer invaluable insights into financial markets. But understanding them is just the starting point; the true power lies in application and continuous refinement.
Finance and data science are fields of ceaseless evolution. Today's ingenious strategy could become tomorrow's outdated method, making lifelong learning a necessity. So, don't just stop at comprehension—experiment, innovate, and keep refining your models. The objective is not just to navigate the complexities of financial markets but to master them. Your exploration shouldn't stop here; consider it the beginning of a broader quest for predictive precision in a world where every fraction of a percentage point counts.
Subscribe to my newsletter
Read articles from Pavel Ianonis directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
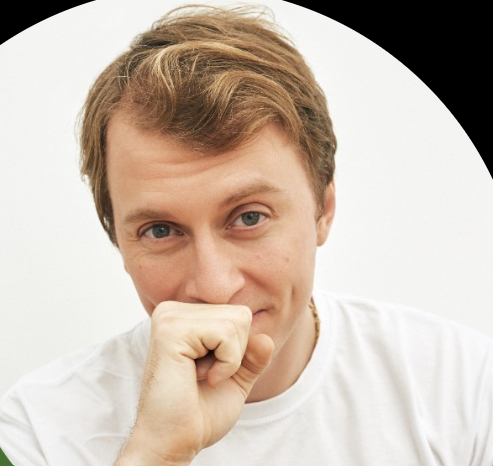
Pavel Ianonis
Pavel Ianonis
Software engineer in fintech with more than 6 years of experience