Best ways to implement a gateway in Spring
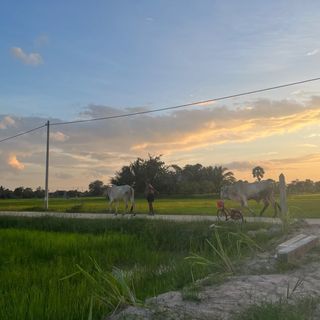
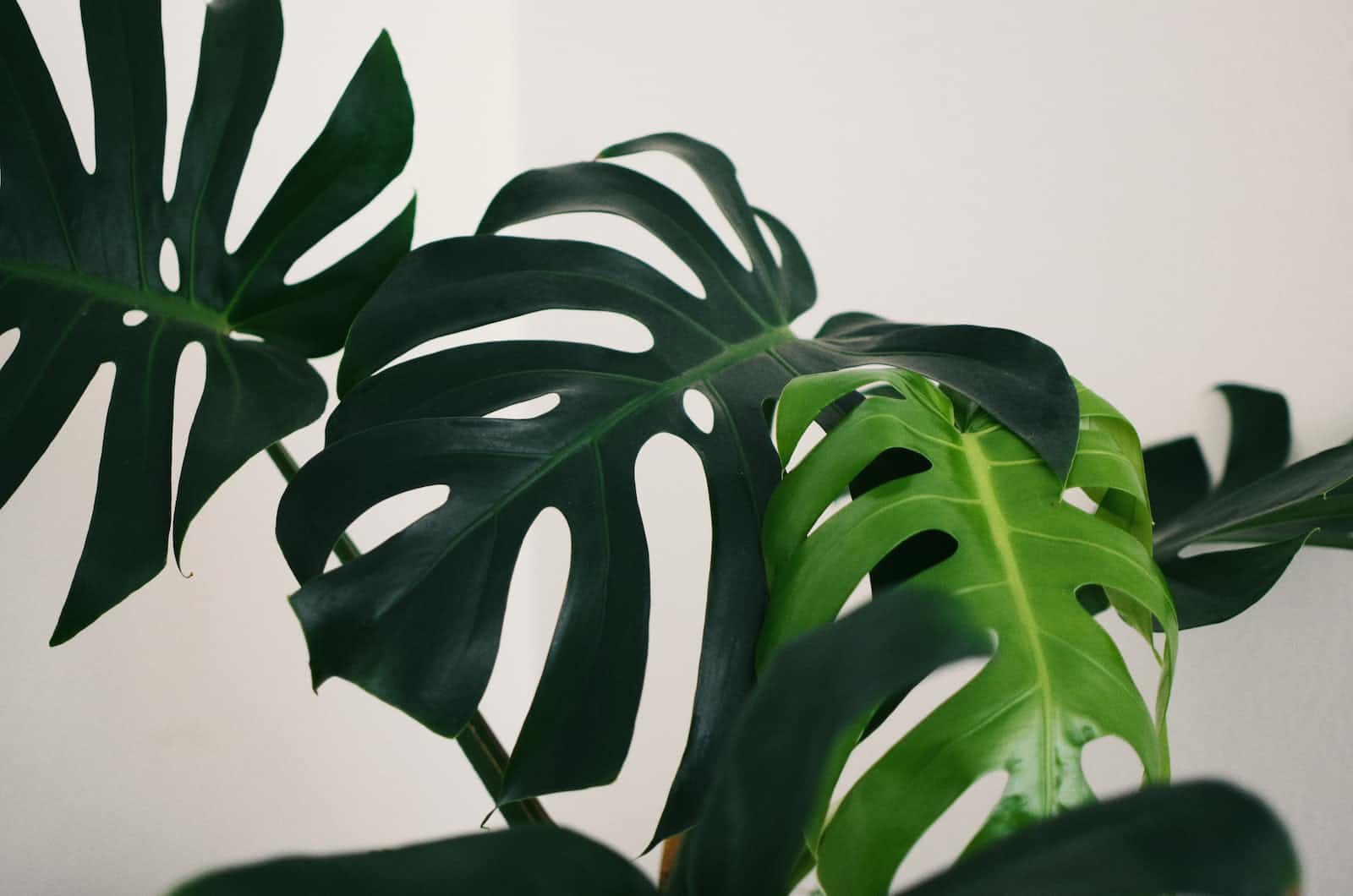
1. Use Spring Cloud Gateway:
- Spring Cloud Gateway provides a flexible and powerful API gateway solution based on reactive programming. It leverages Spring WebFlux to handle a large number of concurrent requests efficiently.
2. Define Routes Using Predicates and Filters:
Predicates: These are conditions used to match incoming requests. Predicates can be based on URL paths, HTTP methods, headers, query parameters, etc.
Filters: Filters are applied to modify or enhance the request or response. They can perform tasks like authentication, logging, request/response modification, rate limiting, etc.
3. Design Resilient and Robust Configurations:
Implement resilience patterns such as circuit breakers (e.g., using Netflix Hystrix), retries, timeouts, and load balancing (using technologies like Spring Cloud LoadBalancer or Netflix Ribbon).
Handle failures gracefully to prevent cascading failures and improve the system's overall reliability.
4. Security Measures:
Integrate Spring Security to implement authentication and authorization mechanisms. Configure security filters to secure routes and endpoints based on roles or access levels.
Employ HTTPS for secure data transmission and enforce strict security configurations.
5. Error Handling and Monitoring:
Define error handling mechanisms for handling exceptions and errors gracefully. Customize error responses with appropriate HTTP status codes and error messages.
Integrate monitoring tools (e.g., Spring Boot Actuator, Prometheus, or Micrometer) for real-time visibility into gateway performance and issues.
6. Configuration Management:
- Externalize configurations using Spring Cloud Config or other external configuration management tools. Centralize and manage configurations for easier updates and maintenance.
7. Load Balancing and Routing:
Configure load balancing strategies to distribute traffic across backend services efficiently. Use dynamic service discovery mechanisms (e.g., Spring Cloud Discovery Clients) for dynamic routing based on service availability and health.
Employ routing mechanisms that handle failovers and dynamic changes in service instances.
8. Testing and Documentation:
Conduct comprehensive testing, including unit tests, integration tests, and end-to-end tests, to ensure the gateway's functionality and behavior under different scenarios.
Maintain detailed documentation outlining the gateway's functionalities, routes, filters, security configurations, and usage guidelines.
9. Performance Optimization:
Optimize gateway performance by implementing caching strategies for static content, reducing unnecessary network calls, and optimizing routing rules to minimize latency.
Continuously monitor and fine-tune the gateway's performance to ensure high throughput and responsiveness.
10. Scalability and High Availability:
Design the gateway for scalability by implementing strategies for horizontal scaling, dynamic load balancing, and handling increased traffic.
Ensure high availability by employing redundancy, failover mechanisms, and strategies for fault tolerance.
11. Versioning and Compatibility:
Implement versioning strategies for APIs to maintain backward compatibility while introducing new features or changes.
Handle different API versions gracefully to ensure smooth transitions for clients using the gateway.
12. Continuous Integration and Deployment (CI/CD):
Implement CI/CD pipelines using tools like Jenkins, GitLab CI/CD, or Travis CI to automate the build, testing, and deployment processes for the gateway application.
Embrace automation for faster and more reliable software delivery.
By meticulously considering and implementing these detailed best practices, developers can construct a robust, efficient, and highly functional API gateway in a Spring-based environment, catering to various requirements such as security, scalability, performance, and maintainability.
Subscribe to my newsletter
Read articles from Vattanac SIM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
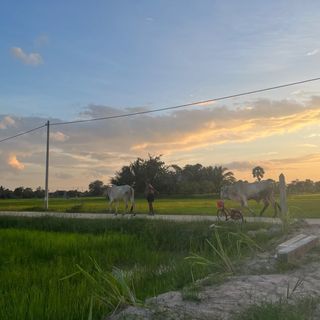
Vattanac SIM
Vattanac SIM
Rome wasn't built in a day.