Top 5 Techniques for Creating Fast and Engaging User Experiences
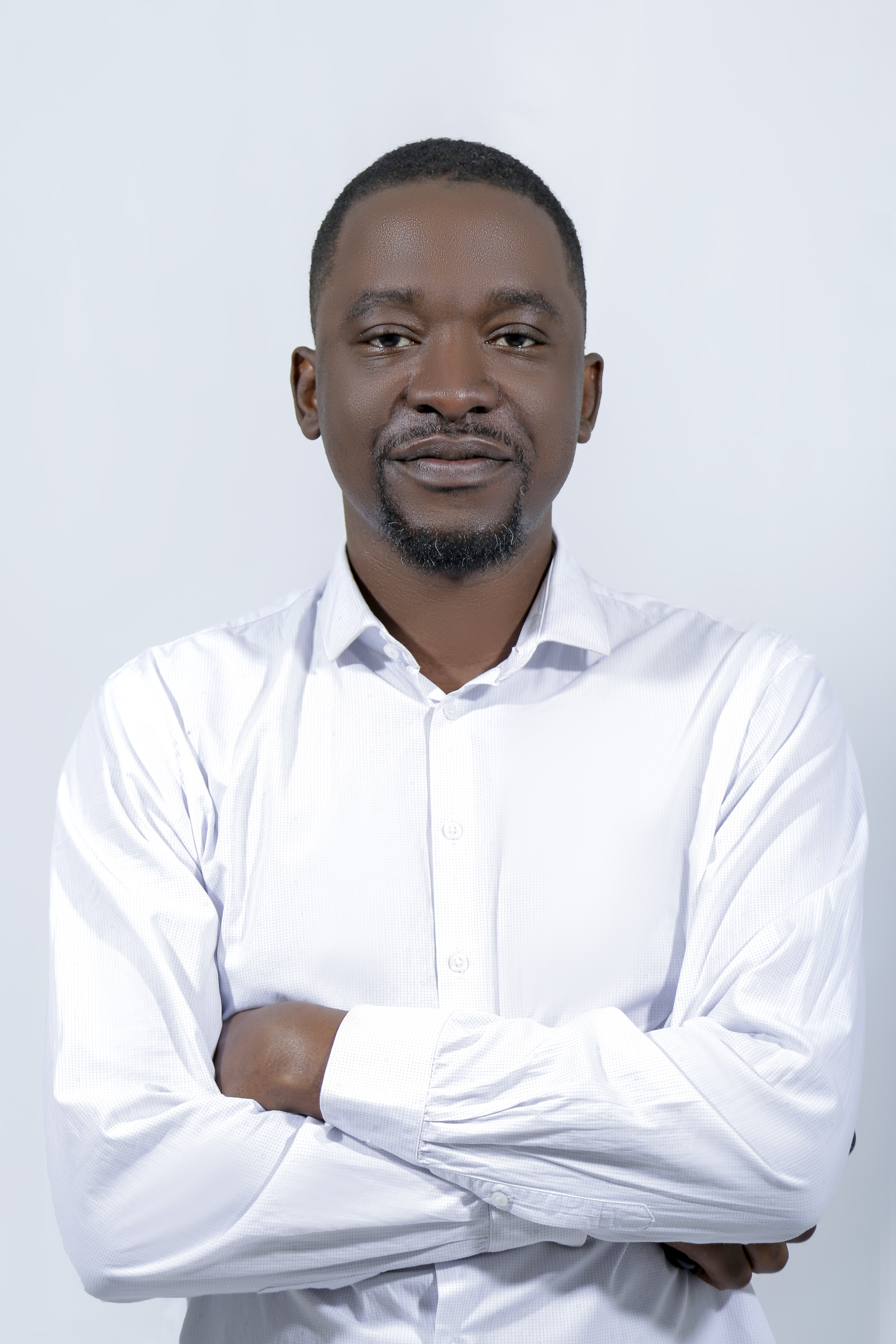
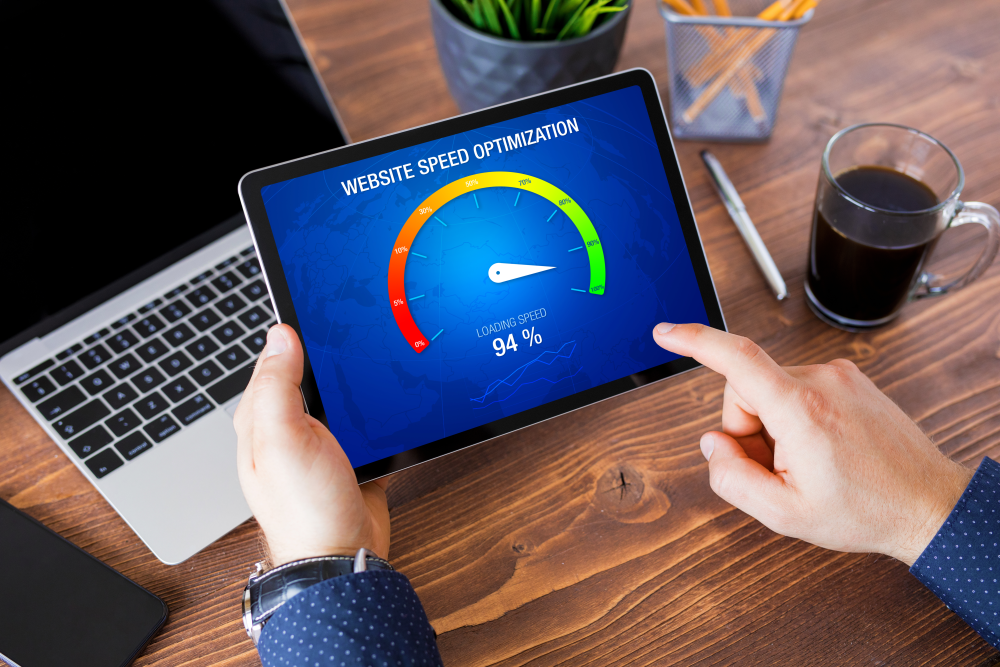
Developers build swift and engaging web experiences on the foundation of frontend optimization. In this comprehensive guide, we'll explore practical strategies aimed at accelerating web experiences while delving into hands-on insights for optimizing front-end performance. My hope through this journey is to equip developers with tips and tricks to build lightning-fast websites that are more engaging for users.
Understanding the Significance of Front-end Optimization
As attention spans shrink in this digital age, a slow-loading website becomes a one-way ticket for users to swiftly seek more captivating alternatives. Frontend optimization has become a critical aspect of web development, focusing on improving the speed, efficiency, and overall performance of web applications. Optimized frontend performance significantly enhances user experiences by minimizing load times and ensuring seamless navigation.
Exploring Practical Strategies for Front-end Optimization
Code Minification and Bundle Optimization
One of the fundamental strategies for optimizing front-end performance revolves around reducing file sizes and optimizing bundles. Let's jump into how minimizing your code and bundle optimization play a pivotal role in speeding up web applications.
// Example of code minification
const uncompressedCode = functionOne() {
// Long lines of code
// Repeated sections
// Unused variables
// Comments and white spaces
};
// Minified version
const compressedCode = functionOne(){/*Shortened code*/}
In this code, we have our code modification. Think of it as having a big puzzle with lots of pieces. In the first part, const uncompressedCode = functionOne()
, the puzzle pieces are spread out and some of them are big and not needed for the picture. So, what we do next is make them smaller and keep only the pieces we need for the full picture. That's what the second part, const compressedCode = functionOne(){/*Shortened code*/}
, does. It's like having the same puzzle but with smaller and only the most important pieces, so it's faster to finish the puzzle!
Image Optimization Techniques
Efficient image optimization is crucial for faster loading times because images play a huge role in your website's loading speed. By employing image compression and implementing lazy loading, developers can significantly enhance web performance.
<!-- Example of lazy loading -->
<img src="placeholder.jpg" data-src="actual-image.jpg" loading="lazy" alt="Image description">
Let's imagine our website is like a big book with lots of pictures. Sometimes, these pictures can take a long time to appear when someone is reading your book (visiting your website). One way to get ahead of this is "lazy-loading" the images. Before the user is ready to see the image the website will render the placeholder image whilst the superhero (actual-image) is waiting in the shadows until they're needed.
So, if someone's reading your website and hasn't reached a certain picture yet, that picture stays hidden until they get closer to it. It helps your website load faster because it doesn't have to show all the pictures right away, only when they're needed!
Caching Strategies
Leveraging browser caching and optimizing caching policies is essential for quicker access to resources. Even though server-side implementation is carried out in the back-end development, it significantly influences front-end performance by controlling how resources are stored and retrieved by the browser. Let's look at how this can be implemented to improve your website's performance.
// Example of setting caching headers in Node.js
app.use((req, res, next) => {
res.setHeader('Cache-Control', 'max-age=3600'); // Cache for 1 hour
next();
});
Imagine your website as a treasure chest full of cool things. Browser caching is like giving visitors a map that helps them quickly find the treasures they want. The code you see is a note telling browsers to remember where things are for about an hour, making everything load faster when visitors come back.
Asynchronous Script Loading
To prevent render-blocking scripts, asynchronous script loading techniques can be employed. Deferred script loading allows non-essential scripts to load after critical content, which increases the overall page speed.
<!-- Example of asynchronous script loading -->
<script src="script.js" async defer></script>
To avoid scripts from slowing down the loading of a web page, we can use asynchronous script loading techniques. With deferred script loading, less important scripts wait until the essential parts of the page load first. The code example you see allows scripts to load without blocking the main content, helping the web page load faster and smoother.
Network Resource Optimization
Prioritizing critical resources and reducing unnecessary HTTP requests significantly impacts front-end performance. Below is a method to optimize network resources for a smoother user experience.
// Example of optimizing network requests
const optimizedRequest = async () => {
const data = await fetch('api/data', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
// Other headers
},
});
return data.json();
};
This code is like a special instruction telling the computer to go and get some important information from the internet ('api/data'). It's making sure to ask for this information in a way that's quick and organized, like asking for it in a language the internet understands ('application/json'). When it gets this information, it knows how to handle it so that it can be used in the computer program. It's all about making sure the computer gets the right stuff from the internet in a fast and efficient way to make things smoother for the people using the website!
Summary
In line with optimizing code efficiency, this summary distills the core concepts from the article for a more comprehensive understanding. It delves into the fundamental strategies of code minimization, image optimization, caching, asynchronous loading, and network resource management. By focusing on these pillars, it equips developers with the tools necessary to create faster, more immersive web experiences.
Subscribe to my newsletter
Read articles from Lesley Mashaya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
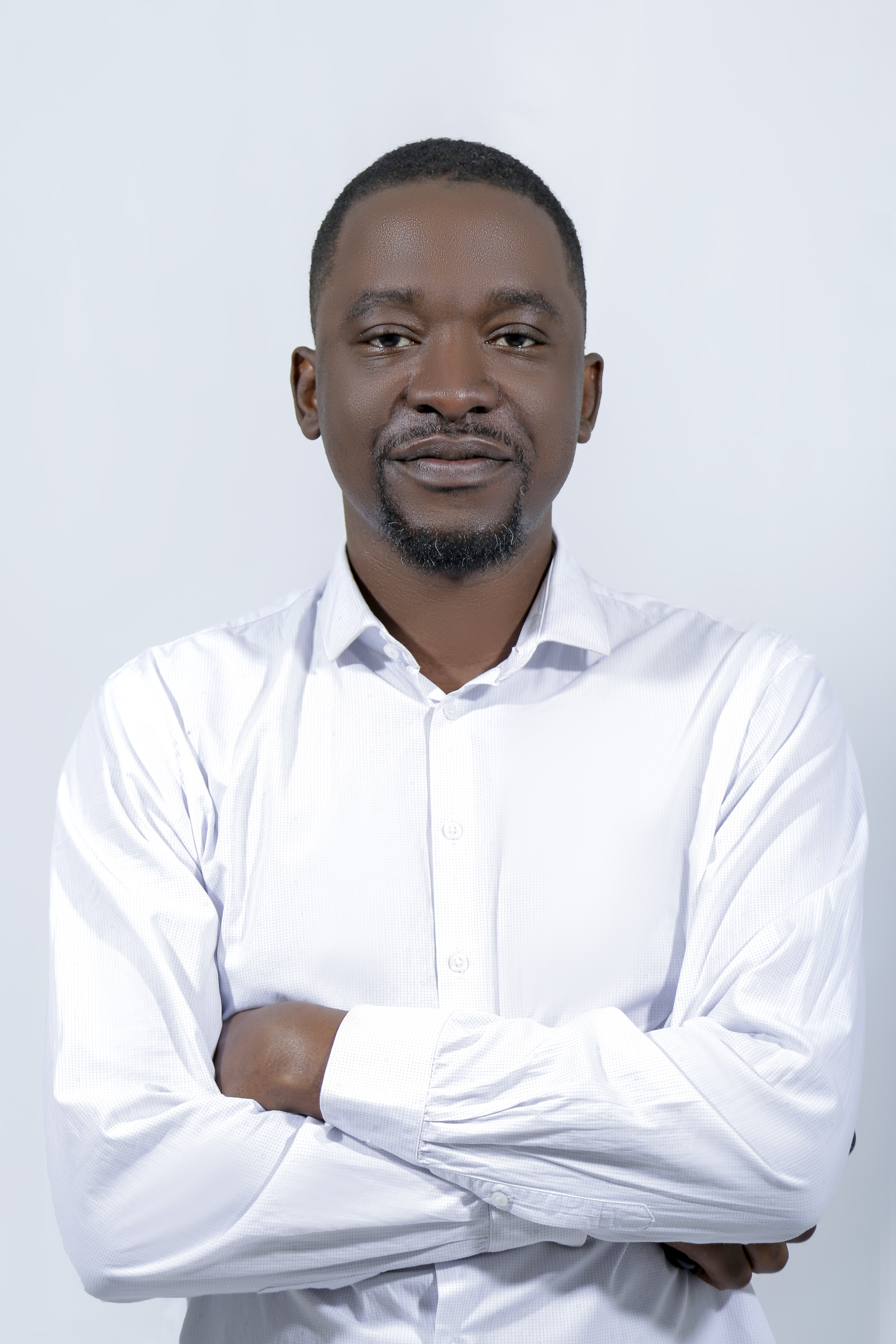
Lesley Mashaya
Lesley Mashaya
Frontend developer | Technical Writer