JavaScript Boolean's, Truthy/Falsy Values, and Numeric Operations
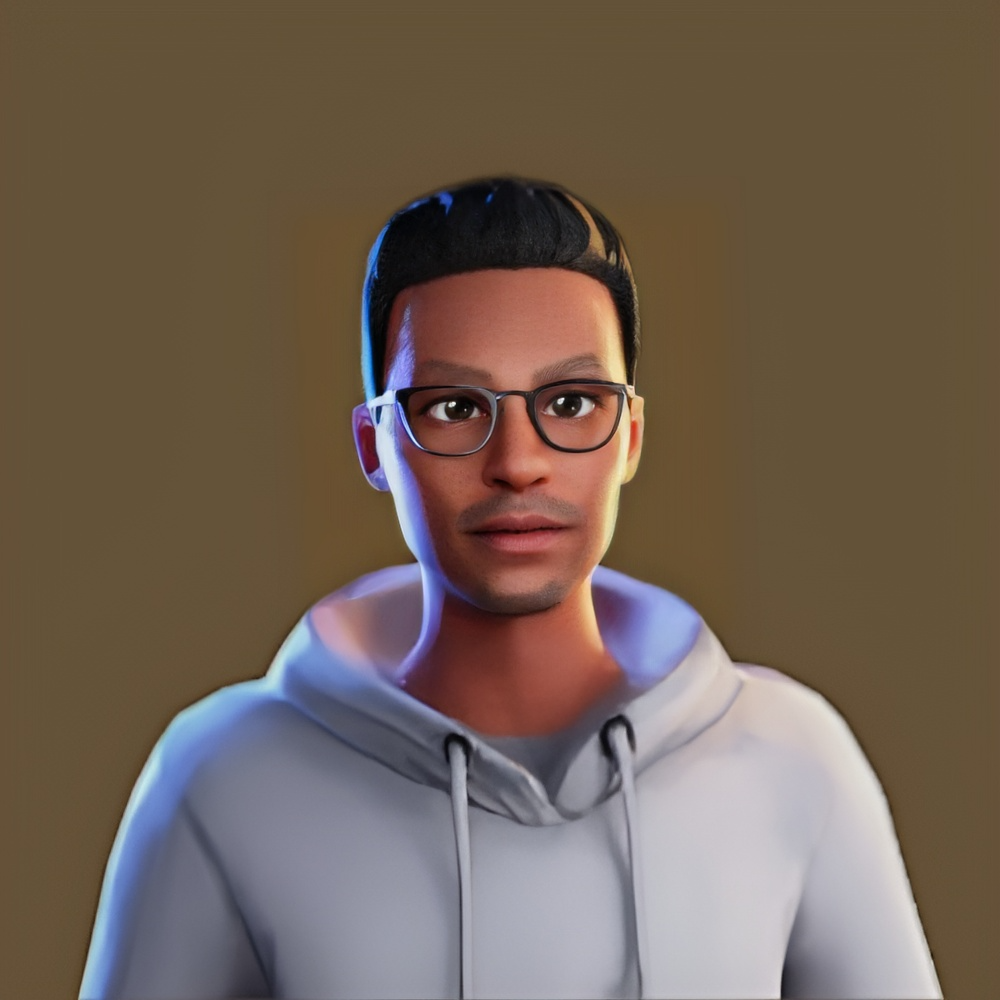
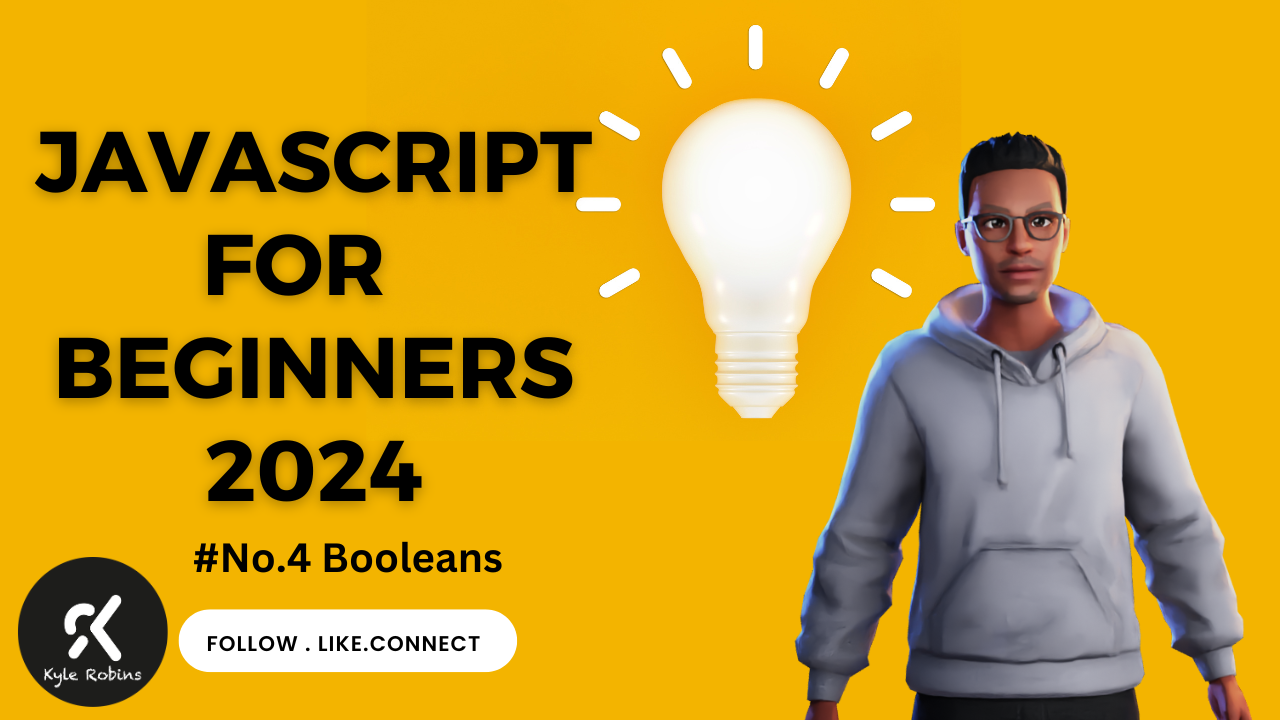
JavaScript Boolean's, Truthy/Falsy Values, and Numeric Operations
In JavaScript, Boolean's are a fundamental data type used to represent truth values: true
or false
. Boolean's are crucial for control flow, conditional statements, and logical operations in your code.
Boolean Primitives
JavaScript has two Boolean primitives:
true
: Represents a true or affirmative value.false
: Represents a false or negative value.
Example:
let isTrue = true;
let isFalse = false;
Logical Operators
JavaScript provides logical operators for working with Boolean values. The common ones include:
&&
(Logical AND): Returnstrue
if both operands are true.||
(Logical OR): Returnstrue
if at least one operand is true.!
(Logical NOT): Returns the opposite of the operand's truthiness.
Example:
let resultAnd = true && false; // Evaluates to false
let resultOr = true || false; // Evaluates to true
let resultNot = !true; // Evaluates to false
Comparison Operators
Comparison operators also return Boolean values. Common ones include:
==
(Equality): Returnstrue
if operands are equal.===
(Strict Equality): Returnstrue
if operands are equal in value and type.!=
(Inequality): Returnstrue
if operands are not equal.!==
(Strict Inequality): Returnstrue
if operands are not equal in value or type.
Example:
let isEqual = (5 == '5'); // Evaluates to true
let isStrictEqual = (5 === '5');// Evaluates to false
let isNotEqual = (5 != 10); // Evaluates to true
let isStrictNotEqual = (5 !== '5'); // Evaluates to true
Truthy Values
In JavaScript, the following values are considered truthy:
- Non-empty Strings: Any string with at least one character.
- Non-zero Numbers: Any number other than zero.
- Objects: Including arrays and functions.
- The special value
true
.
Example:
let truthyString = "Hello, World!";
let truthyNumber = 42;
let truthyObject = { key: 'value' };
if (truthyString && truthyNumber && truthyObject) {
// This block will execute because all values are truthy
}
Falsy Values
Conversely, the following values are considered falsy:
- Empty Strings: A string with no characters (
''
). - The Number
0
. null
.undefined
.- The special value
false
. NaN
(Not a Number).
Example:
let falsyString = "";
let falsyNumber = 0;
let falsyNull = null;
if (!falsyString || falsyNumber === 0 || falsyNull === null) {
// This block will execute because at least one value is falsy
}
Implicit Type Conversion
JavaScript performs implicit type conversion when evaluating truthy/falsy values in certain contexts, such as conditional statements. This can lead to unexpected behavior, so it's essential to be aware of how JavaScript treats different types in Boolean contexts.
Example:
let value = "Hello";
if (value) {
// This block will execute because the string is truthy
}
Conclusion
Understanding Boolean's, logical operators, truthy/falsy values, and numeric operations is fundamental for building logical structures in your JavaScript programs. Whether you're working with conditional statements, logical operations, or comparisons, mastering these concepts is essential for effective and expressive code.
Subscribe to my newsletter
Read articles from Kyle Robins directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
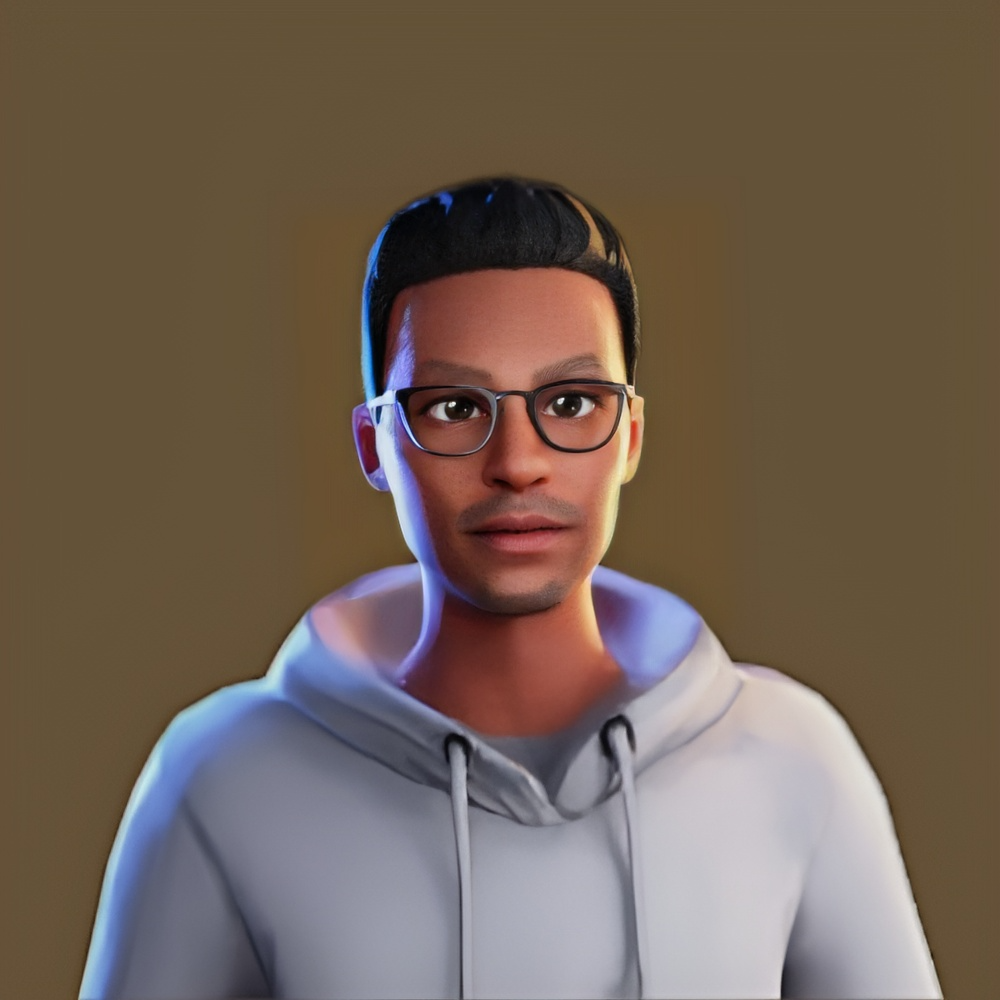
Kyle Robins
Kyle Robins
Hey Viewer, I'm Kyle Robins, Devops / Full-stack Engineer based in Nairobi Kenya. Am passionate about Web development & Content Creation.