Visiting elements in Array
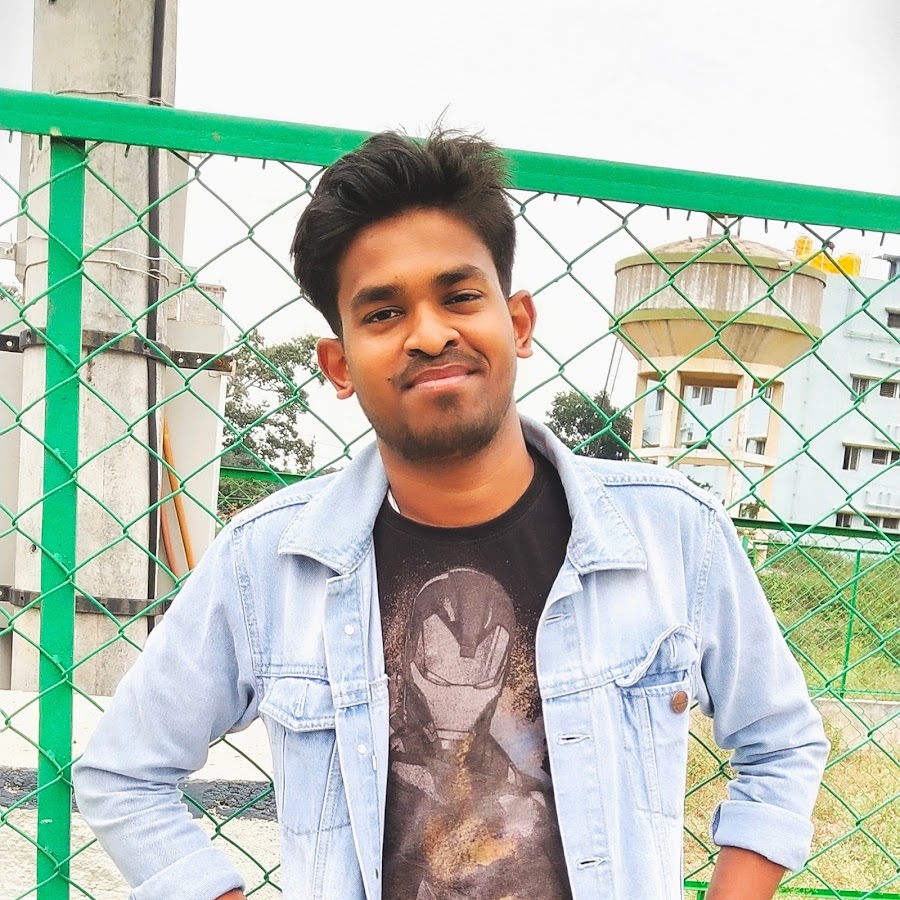
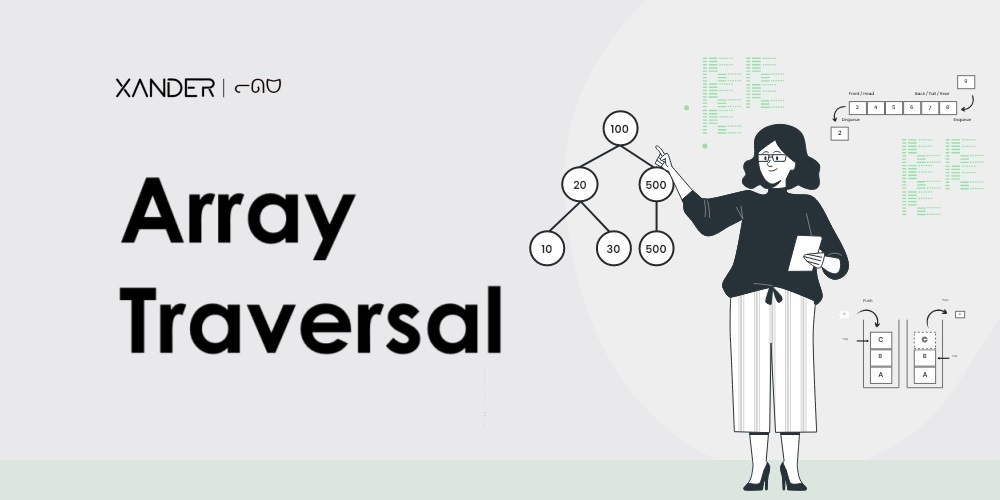
Traversal means visiting each element at least once in Data structure here in our case it's array.
To perform traversal we use loop in our programme where we Take a pointer and start from the first element that is the zeroth index access the zeroth element; after that we increase the pointer with 1 to move to the next element.
Pseudocode for Traversal
// Initialize an array
array = [value1, value2, value3, ..., valueN]
// Using a loop to traverse the array
for index from 0 to length(array) - 1 do
// Access each element using the index
currentElement = array[index]
// Process or print the current element
print(currentElement)
end for
Program for Traversal
#include<iostream>
using std::cout;
using std::endl;
// Pass the size of the array as a separate parameter
void display(int arr[], int size) {
for (int i = 0; i < size; i++) {
cout << arr[i] << " "; // 1 2 3 4 5
}
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
// Calculate the size of the array
int size = sizeof(arr) / sizeof(arr[0]);
// Pass the array and its size to the display function
display(arr, size);
return 0;
}
Time and space complexity
Time Complexity for Array Traversal: The time complexity for traversing an array in a linear fashion is O(n), where "n" is the number of elements in the array. This is because the algorithm must visit each element in the array once.
Space Complexity for Array Traversal: The space complexity for array traversal is O(1), which indicates constant space usage. This is because the algorithm typically uses a constant amount of additional space regardless of the size of the array. The space required is only for loop control variables, and it does not depend on the input size.
Subscribe to my newsletter
Read articles from Xander Billa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
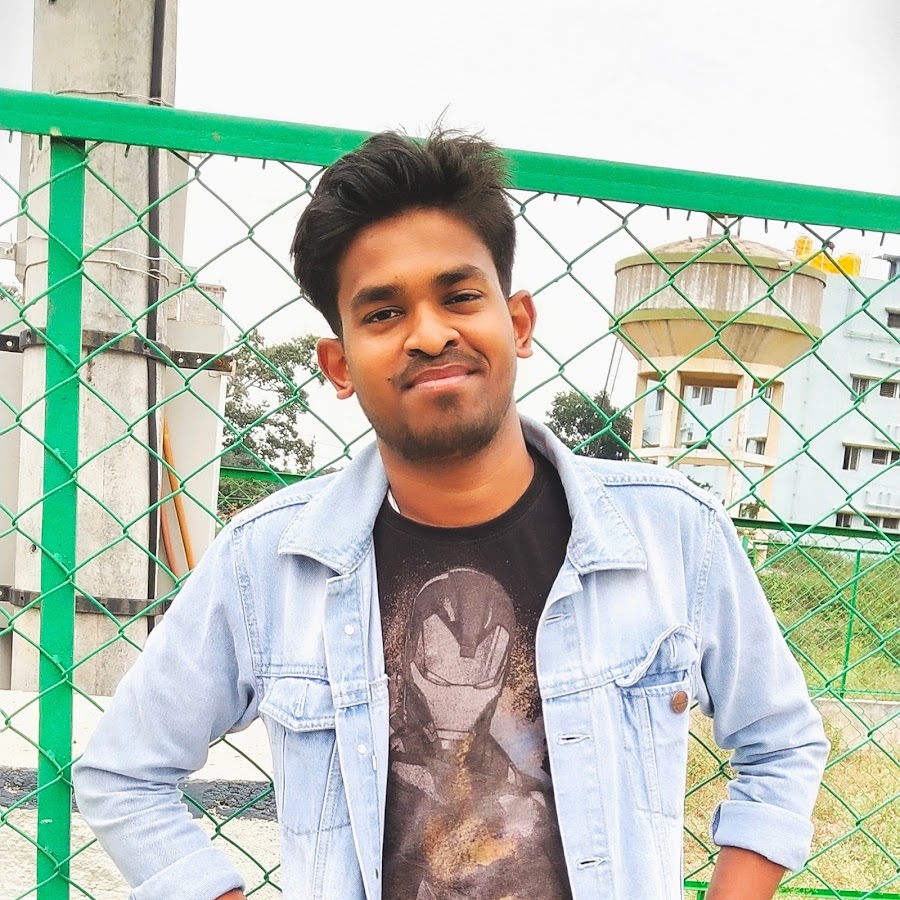
Xander Billa
Xander Billa
Myself Vikas Singh from Varanasi, Uttar Pradesh. Learning and exploring technical domains at Acharya Institute, Bangalore (IN) from the last two years. The main goal is to learn as much domains, tool