How to create a user with a strong password using Bash Script
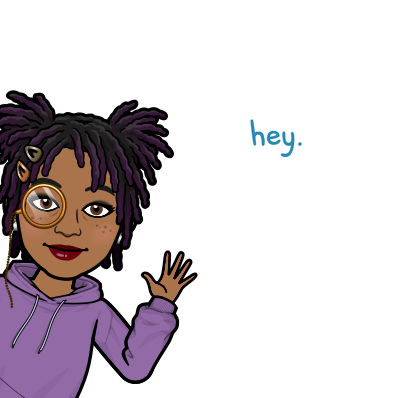
Table of contents
It's been a long time I documented how I accomplished a task and I have decided to change that going forward so help me God. I am picking up my hypothetical pen again to face the world of automation heads on ๐.
Today, I'm going to walk you through how I created a user with a strong password using bash script. This code can be used to create users in a school or work system or any Linux system where you need users and passwords .
Using Bashcript will help automate the process so you don't have to run multiple commands every time you have to create a new user.
A basic knowledge of Bash-scripting is required to understand what I did as I didn't exactly break down the concepts in bits.
For this task, I customized the code to fit a school system, but you can totally change it to fit your scenario with just a few echos ๐
Steps
First we write a function to confirm that the username is avaliable
#!/bin/bash
echo "Welcome to Laurel's High School!"
echo "Kindly create your account"
read -p "Enter a username: " username
# Check if the username is already taken
function check_username {
useradd -n $1 >/dev/null 2>&1
if [ $? -eq 0 ]; then
echo "Username '$1' is already taken. Please choose a different username."
return 1
fi
}
check_username $username
Then we prompt our user for password ensuring they reconfirm the password
# prompt for password
echo "Password must be at least 8 characters long and include an uppercase, lowercase, number and special characters): "
echo "Enter a strong password(hidden):"
read -s password
echo "Confirm your password match: "
read -s password2
Then we set our conditions ensuring that the password is strong and meets the requirements of a mix of uppercase, lowercase, numbers, and special characters.
# Define and ensure password condtions are met
# Check password match
if [[ $password != $password2 ]]; then
echo -e $WARNING"Password does not match."$CLEAR
else
echo -e $SUCCESS"Password matches."$CLEAR
fi
# check for length
if [[ ${#password} -ge 8 ]]; then
echo -e $SUCCESS"+Password length is good."$CLEAR
else
echo -e $WARNING"-Password must be at least 8 characters long."$CLEAR
fi
#check for special characters
if [[ $password =~ [[:punct:]] ]]; then
echo -e $SUCCESS"+Password has a special character."$CLEAR
else
echo -e $WARNING"-Password must include at least one special character (!@#$%^&*()+)."$CLEAR
fi
#check for upper and lower case letters
if [[ $password =~ [[:upper:]] && $password =~ [[:lower:]] ]]; then
echo -e $SUCCESS"+Password contains both uppercase and lowercase letters."$CLEAR
else
echo -e $WARNING"-Password should contain both uppercase and lowercase letters."$CLEAR
fi
# check for digits
if [[ $password =~ [[:digit:]] ]]; then
echo -e $SUCCESS"+Password contains at least one digit."$CLEAR
else
echo -e $WARNING"-Password should contain at least one digit."$CLEAR
fi
Then we validate all our conditions in a function before we confirm password as successful and account creation confirmed.
function validate_password {
if [[ ${#password} -ge 8 && "$password" =~ [[:punct:]] && $password =~ [[:upper:]] && $password =~ [[:lower:]] && $password =~ [[:digit:]] && $password == $password2 ]]; then
echo -e "$SUCCESS Password is strong!"$CLEAR
# Create user with username and hashed password
sudo useradd -m "$username" -p "$hashed_password"
echo "Account created successfully!"
echo -e "Username: $username"
echo -e "User ID: $(id -u "$username")"
else
echo -e "$WARNING Error creating password. kindly comply with the requirements"$CLEAR
fi
}
validate_password $password
We used the variables below to add colours to our outputs 31 is for red, 32 green, and 33 gold/yellow. Declare the variable at the top of the script
#!/bin/bash
WARNING="\e[31m"
SUCCESS="\e[32m"
CLEAR="\e[1;33m"
Save your script with the .sh extention for bash eg create-user.sh, and run on a linux server withthe command
bash create-user.sh
Also you can make your file executable by running the chmod command to make your file executable and run the command
./ create-user.sh
You can get the complete %[https://github.com/EjiroLaurelD/SCA-Devops/blob/main/linux/create-user.sh]
I hope this helps someone ๐
Thank you for reading
Subscribe to my newsletter
Read articles from Ejiroghene Laurel Dafe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
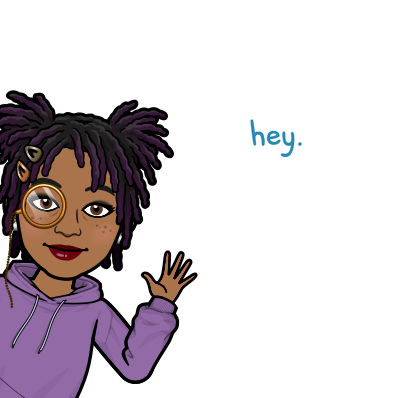
Ejiroghene Laurel Dafe
Ejiroghene Laurel Dafe
I am a DevOps Engineer passionate about learning and writing about my experiences on my journey to be a world class Cloud/Devops Engineer. I am passionate about sharing knowledge, learning and mastering cloud technologies, making sweet treats, and putting smiles on the faces of people.