Date Object In Depth: Its Properties and Methods in JavaScript
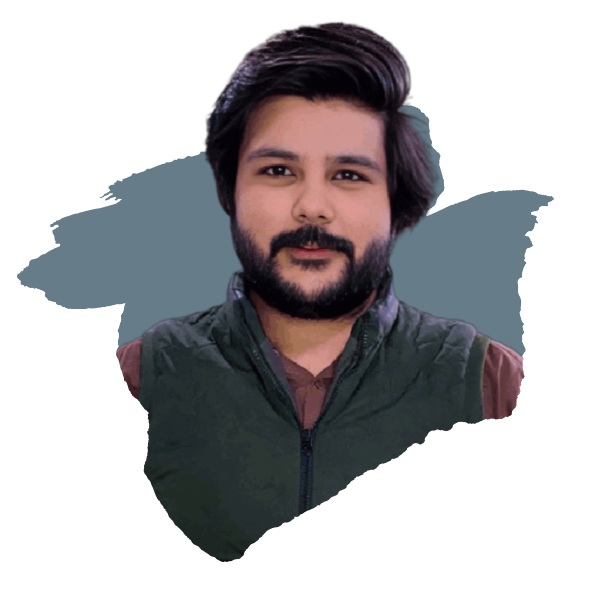
Table of contents
- Date Object
- Properties and Methods of a Date Object:
- 1. constructor
- What is an instance in JavaScript?
- JavaScript Date Get Methods
- 2. getDate()
- 3. getFullYear()
- 4. getMonth()
- 5. getDay()
- 6. getHours()
- Note:
- 7. getMinutes()
- 8. getSeconds()
- 9. getMilliseconds()
- 10. getTime()
- 11. getTimezoneOffset()
- 12. getUTCDate()
- 13. getUTCFullYear()
- 14. getUTCMonth()
- 15. getUTCDay()
- 16. getUTCHours()
- 17. getUTCMinutes()
- 18. getUTCSeconds()
- 19. getUTCMilliseconds()
- 20. Date.now()
- Note:
- 21. Date.parse()
- Note:
- 22. setDate()
- 23. setTime()
- 24. setFullYear()
- 25. setMonth()
- 26. setHours()
- 27. setMinutes()
- 28. setSeconds()
- 29. setMilliseconds()
- 30. setUTCDate()
- 31. setUTCFullYear()
- 32. setUTCMonth()
- 33. setUTCHours()
- 34. setUTCMinutes()
- 35. setUTCSeconds()
- 36. setUTCMilliseconds()
- 37. toString()
- 38. toUTCString()
- 39. toTimeString()
- 40. toDateString()
- 41. toISOString()
- 42. toLocaleString()
- 43. toLocaleDateString()
- 44. toLocaleTimeString()
- 45. toJSON()
- Note:
- 46. valueOf()
- Note:
- 47. UTC()
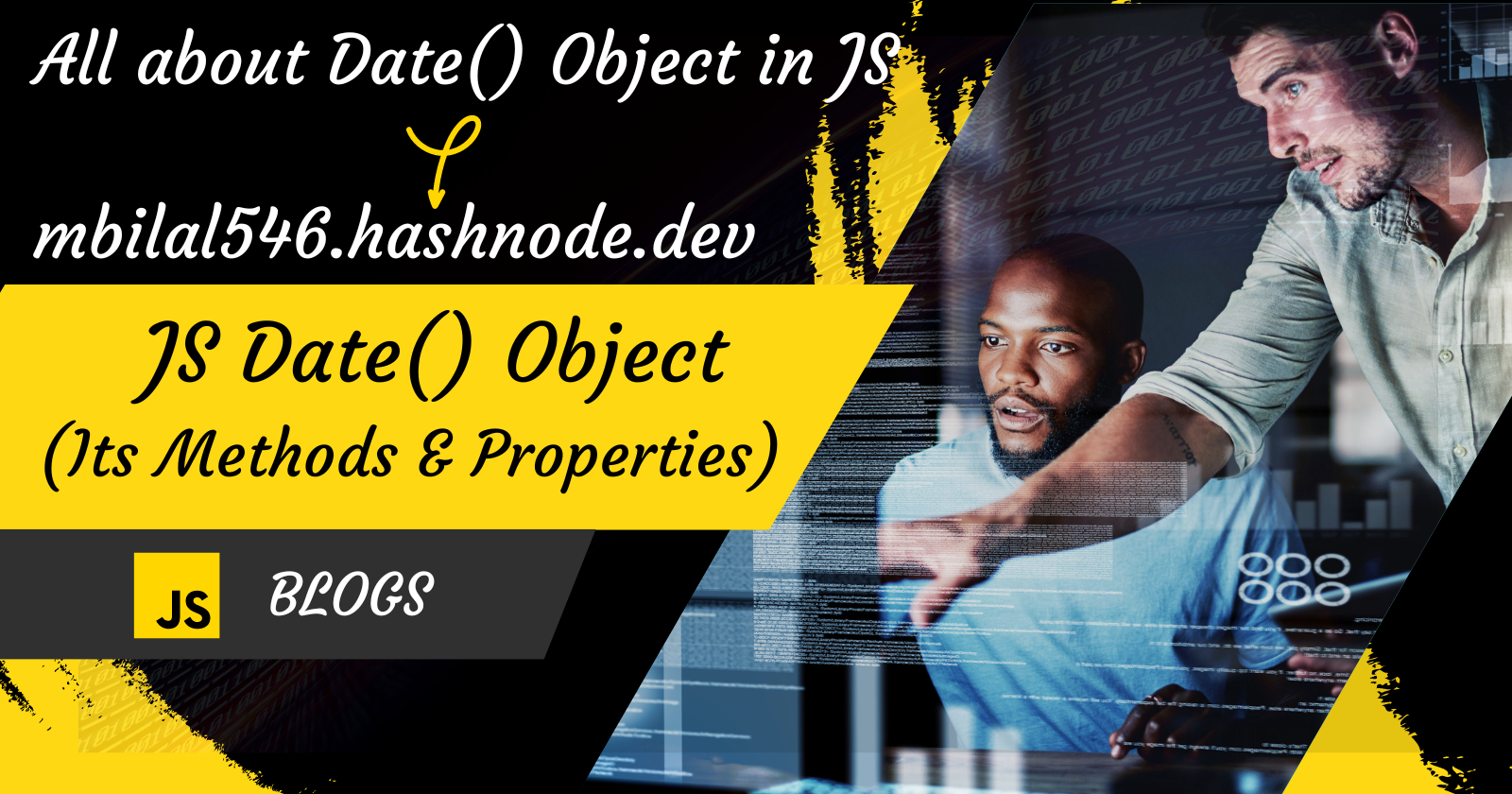
Date Object
In JavaScript, the Date
object is used to work with dates and times. It provides methods for creating, manipulating, formatting, and parsing dates. We will explore every method here to gain knowledge and become experts in Date
objects. To create it, Date
we have to write a keyword new
and then write Date
a keyword.
There are three ways of making Date
object.
// 1st method to create date object
const dateChapter = new Date();
console.log(dateChapter);
Output: 2023-12-11T22:24:48.521Z
// 2nd method to create date object in string
const specificDate = Date("December 12, 2023");
console.log(specificDate);
Output: Tue Dec 12 2023 13:41:33 GMT+0500 (Pakistan Standard Time)
// 3rd method to creatr date object in numbers
const numDate = new Date(2023, 11, 12, 15, 25, 50, 0);
// Format is: Year, month, day, hours, minutes, seconds, milliseconds
// Here Months are 0-based (0 - January, 1 - February, ..., 11 - December)
console.log(numDate);
Output: 2023-12-12T10:25:50.000Z
Properties and Methods of a Date Object:
1. constructor
In JavaScript, the Date
object has aconstructor
property that refers to the constructor function that creates an instance of the Date
object. The Date
constructor property is read-only and allows you to identify the function used to create a specific Date
object.
For Example:
const constDate = new Date();
console.log(constDate.constructor);
Output: [Function: Date]
What is an instance in JavaScript?
In JavaScript, an instance refers to an individual occurrence of an object created from a particular class or constructor function. In object-oriented programming terminology, an instance is an instantiation of a class. In our series, we will discuss it in depth.
JavaScript Date Get Methods
2. getDate()
JavaScript provides several methods for working with dates
and time
. The getDate()
method specifically returns the day of the month (an integer between 1 and 31) of the date object in local time.
For Example:
const dDate = new Date();
console.log(dDate.getDate());
Output: 12
// Today is 12 December so, output is 12. When you will run this program
// then it display date of that day
3. getFullYear()
The getFullYear()
method is used to retrieve the year of a date object in JavaScript. It is a member of the Date
object prototype and can be accessed on any valid date object.
For Example:
const yearDate = new Date();
console.log(yearDate.getFullYear());
Output: 2023
4. getMonth()
The getMonth()
the method is used to retrieve the month (0-11) from an Date
object. It is a part of the Date object methods and is available for all Date
objects created in JavaScript. The getMonth()
method retrieves the month based on the local time zone of the user.
For Example:
const monthDate = new Date();
console.log(monthDate.getMonth());
Output: 11 // It is December because it start counts from 0
// Practical example to show day as a string
const allMonths = [
"January",
"February",
"March",
"April",
"May",
"June",
"July",
"August",
"September",
"October",
"November",
"December"
];
const date = new Date();
const getMonth = date.getMonth();console.log(allMonths[getMonth]);
Output: December
5. getDay()
The getDay()
method in JavaScript is used to retrieve the day of the week for a specified date object. It returns an integer between 0 and 6. (It starts from Sunday so 0 is for Sunday and then so on).
For Example:
const dayDate = new Date();
console.log(dayDate.getDay());
Output: 2 // Here 2 is for Tuesday.
// Practical example to show day as a string
const AllDaysOfTheWeek = [
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday",
];
const currentDay = new Date();
const today = currentDay.getDay();
console.log(AllDaysOfTheWeek[today]);
// This will output current day of week as a string
Output: Tuesday // This is mine, you can check yours output also
6. getHours()
The getHours()
method in JavaScript that retrieves the hour (0 to 23) of the date
represented by the object, based on the local time.
For Example:
const hoursDate = new Date();
console.log(hoursDate.getHours());
Output: 17
Note:
Keep in mind that the getHours()
method returns the hour in local time. If you need to work with UTC (Coordinated Universal Time), you can use getUTCHours()
instead.
7. getMinutes()
The getMinutes()
method JavaScript returns the current minute (0-59) of the date associated with the Date
object. It returns NaN
if the Date
object is invalid.
For Example:
const minutesDate = new Date();
console.log(minutesDate.getMinutes());
Output: 51
// You can also get minutes of specific date
const minutesDate = new Date();
console.log(minutesDate.getMinutes(2023, 11, 12, 17, 54)); // Dec 12, 2023, 5:54 PM
Output:54
8. getSeconds()
The getSeconds()
method is used to retrieve the seconds (0-59) of the specified date according to local time. It returns NaN
if the Date
object is invalid.
For Example:
const secondsDate = new Date();
console.log(secondsDate.getSeconds());
Output: 11 // Here it will print your current seconds
9. getMilliseconds()
The getMilliseconds()
method in JavaScript is used to retrieve the milliseconds of a date object, according to local time. It returns the milliseconds (0 to 999) of a date.
For Example:
const millisecondsDate = new Date();
console.log(millisecondsDate.getMilliseconds());
Output: 277 // Here it will print your current milliseconds
10. getTime()
The getTime()
the method in Javascript returns the number of milliseconds elapsed since midnight on January 1, 1970, UT197C (Unix epoch).
For Example:
const timeDate = new Date();
console.log(timeDate.getTime());
Output: 1702391840510
// It is a large number representing milliseconds since January 1, 1970.
11. getTimezoneOffset()
The getTimezoneOffset()
method in JavaScript that returns the difference, in minutes, between the current local time and the Universal Coordinated Time (UTC). It returns an integer representing the offset in minutes. A positive value indicates that the local time is ahead of UTC, while a negative value means it is behind UTC.
For Example:
const timeZoneDate = new Date();
console.log(timeZoneDate.getTimezoneOffset());
Output: -300
12. getUTCDate()
The getUTCDate()
method is used to retrieve the day of the month (represented as an integer between 1 and 31) for a given date object, according to Universal Coordinated Time (UTC), regardless of the local time zone.
For Example:
const UTCDate = new Date();
console.log(UTCDate.getUTCDate());
Output: 13
// It prints date according to your present date when you are using it
getUTCDate()
method is useful when dealing with dates and times that need to be interpreted globally.
13. getUTCFullYear()
The getUTCFullYear()
method in Javascript is used to retrieve the full year (four digits) according to the Coordinated Universal Time (UTC) from a given Date object, regardless of the local time zone of the user.
For Example:
const UTCDate = new Date();
console.log(UTCDate.getUTCFullYear());
Output: 2023
// It prints year according to your present time when you are using it.
// If you write date manually, it retrieve the full year from it.
const UTCDate = new Date("2024-5-22");
console.log(UTCDate.getUTCFullYear());
Output: 2024
14. getUTCMonth()
The getUTCMonth()
method in JavaScript is used to retrieve the month of a date according to Coordinated Universal Time (UTC). It starts counting 0 as January and ends at 11 as December.
For Example:
const UTCMonth = new Date();
console.log(UTCMonth.getUTCMonth());
Output: 11 // Here 11 is December because it start count from 0.
// It prints month according to your present time when you are using it.
// If you want to print from 1 to 12 as months are so,
// you need to add 1 in it.
const UTCMonth = new Date();
console.log(UTCMonth.getUTCMonth() + 1);
Output: 12 // December
15. getUTCDay()
The getUTCDay()
method is a built-in function of the Date object in JavaScript. It returns the day of the week (0-6) for a given date, based on Universal Coordinated Time (UTC).
For Example:
const UTCDay = new Date();
console.log(UTCDay.getUTCDay());
Output: 5 // Friday
// It start from sunday and print the present day in the output
// If you want to print from 1 to 6 as days are so,
// you need to add 1 in it.
const UTCDate = new Date();
console.log(UTCDate.getUTCDay() + 1);
Output: 6 // Friday
16. getUTCHours()
ThegetUTCHours()
method in JavaScript is used to retrieve the hour component of a date according to Coordinated Universal Time (UTC). It returns an integer value between 0 and 23, where 0 represents midnight UTC and 23 represents 11 pm UTC.
For Example:
const UTCHour = new Date();
console.log(UTCHour.getUTCHours());
Output: 16
// It prints hour according to your present time when you are using it.
17. getUTCMinutes()
The getUTCMinutes()
method in JavaScript is used to retrieve the minute component of a date object in Coordinated Universal Time (UTC). It returns an integer between 0 and 59, representing the minutes from the start of the hour in UTC.
For Example:
const UTCMinutes = new Date();
console.log(UTCMinutes.getUTCMinutes());
Output: 28
// It prints minutes according to your present time when you are using it.
18. getUTCSeconds()
The getUTCSeconds()
method in JavaScript is used to retrieve the seconds component of a date object. It returns an integer value between 0 and 59, representing the current second according to UTC (Universal Coordinated Time).
For Example:
const UTCSeconds = new Date();
console.log(UTCSeconds.getUTCSeconds());
Output: 28
// It prints seconds according to your present time when you are using it.
19. getUTCMilliseconds()
The getUTCMilliseconds()
method in JavaScript is used to retrieve the millisecond portion of a date object, specifically in the context of Universal Coordinated Time (UTC). An integer between 0 and 999, represents the millisecond part of the date in UTC.
For Example:
const UTCMilliSeconds = new Date();
console.log(UTCMilliSeconds.getUTCMilliseconds());
Output: 950
// It prints milliSeconds according to your present time when you are using it.
20. Date.now()
The Date.now()
method in JavaScript is a handy tool for working with time in your code. It returns the current timestamp as the number of milliseconds elapsed since January 1, 1970, 00:00:00 UTC. This point in time is often referred to as the "Unix epoch."
For Example:
const dateNow = Date.now();
console.log(dateNow);
// Output: 1702659109060
// It is number of milliseconds elapsed since January 1, 1970.
Note:
You access it using Date.now()
, not on a specific date object. It provides millisecond accuracy, allowing for precise timing calculations. It is used in various applications like measuring performance, tracking user interactions, and scheduling tasks.
21. Date.parse()
The Date.parse()
method in JavaScript is a powerful tool for parsing date strings and converting them into timestamps. It takes a string representation of a date as input and it returns the number of milliseconds that have elapsed since midnight, January 1, 1970, UTC. It can understand and parse, including ISO 8601, RFC 2822, and even some custom formats.
For Example:
const dateParse = Date.parse("2023-12-01");
console.log(dateParse);
Output: 1701388800000
// Here we are Parsing two dates
const startDate = Date.parse("2023-12-01");
const endDate = Date.parse("2023-12-15");
const difference = endDate - startDate;
console.log(difference);
Output: 1209600000
// If you want seconds from the difference
const seconds = difference / 1000;
console.log(seconds);
Output: 1209600
Note:
For forms or user interactions where dates are entered as strings, Date.parse()
can validate and convert them into usable timestamps. By comparing the timestamps obtained through Date.parse()
, you can easily calculate the elapsed time between two dates.
22. setDate()
The setDate()
method in JavaScript is used to change the day of the month within an Date
object. It's a powerful tool for manipulating dates and working with calendars.
For Example:
const setDt = new Date();
setDt.setDate(25);
console.log(`The Set Date is : ${setDt}`);
Output:
The Set Date is : Mon Dec 25 2023 16:31:03 GMT+0500 (Pakistan Standard Time)
// Here the Date is 25 that you set above
23. setTime()
The setTime()
method in JavaScript is used to modify the internal timestamp of a Date
object by setting the new timestamp in milliseconds since the epoch, which is midnight at the start of January 1, 1970 UTC.
For Example:
const setT = new Date();
setT.setTime(1676451300000);
console.log(setT);
Output: 2023-02-15T08:55:00.000Z
// Prints "Sun Dec 17 2023 02:05:00 GMT+0000 (Coordinated Universal Time)
24. setFullYear()
The setFullYear()
method in JavaScript is used to change the year of an Date
object. It provides versatility in its arguments, enabling the adjustment of not just the year
, but also the month
and day
if desired and returns the updated Date
object.
For Example:
const setY = new Date();
setY.setFullYear(2025);
console.log(`The Set Year is : ${setY}`);
Output:
The Set Year is : Wed Dec 17 2025 16:28:32 GMT+0500 (Pakistan Standard Time)
// Here the year is 2025 that you set above
25. setMonth()
The setMonth()
method in JavaScript is used to change the month of an Date
object. It's a handy tool for manipulating dates and performing calculations involving months. It takes argument (0-11) and returns the updated Date
object.
For Example:
const setM = new Date();
setM.setMonth(1); // as it take arrguments (0-11) so 1 mean feb
console.log(`The Set Month is : ${setM}`);
Output:
The Set Month is : Fri Feb 17 2023 16:25:48 GMT+0500 (Pakistan Standard Time)
// Here the Month is feb that you set above
26. setHours()
The setHours()
method in JavaScript is used to manipulate the hour value of an Date
object. It allows you to set the hour to a specific value, and optionally adjust the minutes, seconds, and milliseconds as well. It takes the arguments (0-23) and returns the updated Date
object.
For Example:
const setHour = new Date();
setHour.setHours(5);
console.log(`The Set Hours is : ${setHour}`);
Output:
The Set Hours is : Sun Dec 17 2023 05:35:43 GMT+0500 (Pakistan Standard Time)
// Here the Hour is 05 that you set above
27. setMinutes()
The setMinutes()
method in JavaScript is used to modify the minute value of an Date
object. It changes the minutes according to the local time, and can also indirectly affect the hour, seconds, and milliseconds if values wrap around due to the change. It takes the arguments (0-59) and returns the updated Date
object.
For Example:
const setMinute = new Date();
setMinute.setMinutes(33);
console.log(`The Set Minutes is : ${setMinute}`);
Output:
The Set Minutes is : Sun Dec 17 2023 16:33:01 GMT+0500 (Pakistan Standard Time)
// Here the year is 33 that you set above
28. setSeconds()
The setSeconds()
method in JavaScript is used to set the seconds of an Date
object. It takes argument (0-59) and returns the updated Date
object.
For Example:
const setSecond = new Date();
setSecond.setSeconds(21);
console.log(`The Set Minutes is : ${setSecond}`);
Output:
The Set Minutes is : Sun Dec 17 2023 16:45:21 GMT+0500 (Pakistan Standard Time)
// Here the year is 21 that you set above
29. setMilliseconds()
The setMilliseconds()
method in JavaScript is used to change the milliseconds component of an Date
object. It updates the date according to the local time zone. It takes a single numeric parameter between 0 and 999 as the new milliseconds value and returns the updated Date
object.
For Example:
const setMilliSecond = new Date();
setMilliSecond.setMilliseconds(111);
console.log(setMilliSecond);
Output: 2023-12-17T11:50:29.111Z
// Here the Milliseconds is 111 that you set above
30. setUTCDate()
The setUTCDate()
method in JavaScript is used to modify the day of the month for an Date
object based on Universal Coordinated Time (UTC). It's useful when you need to work with dates and times independent of the local time zone. It takes values are 1 (first day) to 31 (depending on the month and year) and returns the updated Date
object.
For Example:
const setUTCDate = new Date();
setUTCDate.setUTCDate(20);
console.log(`The Set UTCDate is : ${setUTCDate}`);
Output:
The Set Minutes is : Wed Dec 20 2023 16:56:33 GMT+0500 (Pakistan Standard Time)
// Here the UTCDate is 20 that you set above
31. setUTCFullYear()
The setUTCFullYear()
method in JavaScript is used to change the year for a specified date object according to Universal Coordinated Time (UTC). It's useful for manipulating dates and times without worrying about local time zone differences. It takes arguments from 0 to 9999 and returns the updated Date
object.
For Example:
const setUTCFullYear = new Date();
setUTCFullYear.setUTCFullYear(2027);
console.log(`The Set UTCFullYear is : ${setUTCFullYear}`);
Output:
The Set UTCFullYear is : Fri Dec 17 2027 17:00:52 GMT+0500 (Pakistan Standard Time)
// Here the UTCFullYear is 2027 that you set above
32. setUTCMonth()
The setUTCMonth()
method in JavaScript is used to change the month of an Date
object based on Universal Coordinated Time (UTC). It takes argument (0-11) and returns the updated Date
object.
For Example:
const setUTCMonth = new Date();
setUTCMonth.setUTCMonth(8);
console.log(`The Set UTCMonth is : ${setUTCMonth}`);
Output:
The Set UTCMonth is : Sun Sep 17 2023 17:17:42 GMT+0500 (Pakistan Standard Time)
// Here the UTCMonth is sep that you set above
33. setUTCHours()
The setUTCHours()
method in JavaScript is used to set the hour component of a Date object in Coordinated Universal Time (UTC). It takes arguments from 0 (midnight UTC) to 23 and returns the updated Date
object.
For Example:
const setUTCHour = new Date();
setUTCHour.setUTCHours(18);
console.log(setUTCHour);
Output: 2023-12-17T18:43:33.758Z
// Here the UTCHour is 18 that you set above
34. setUTCMinutes()
The setUTCMinutes()
method is used to set the minutes of a date object in JavaScript, specifically according to Universal Coordinated Time (UTC). It takes arguments between (0-59) and returns the updated Date
object.
For Example:
const setUTCMinute = new Date();
setUTCMinute.setUTCMinutes(40);
console.log(`The Set UTCMinute is : ${setUTCMinute}`);
Output:
The Set UTCMinute is : Sun Dec 17 2023 17:40:52 GMT+0500 (Pakistan Standard Time)
// Here the UTCMinute is 40 that you set above
35. setUTCSeconds()
The setUTCSeconds()
method in JavaScript is used to set the seconds and milliseconds of an Date
object based on Universal Coordinated Time (UTC). It takes arguments between (0-59) and returns the updated Date
object.
For Example:
const setUTCSecond = new Date();
setUTCSecond.setUTCSeconds(20);
console.log(`The Set UTCSecond is : ${setUTCSecond}`);
Output:
The Set UTCSecond is : Sun Dec 17 2023 17:32:20 GMT+0500 (Pakistan Standard Time)
// Here the UTCSecond is 20 that you set above
36. setUTCMilliseconds()
The setUTCMilliseconds()
method in JavaScript is used to set the millisecond value of an Date
object according to Coordinated Universal Time (UTC). It takes one argument, a number between 0 and 999, and returns the updated Date
object.
For Example:
const setUTCMilliSecond = new Date();
setUTCMilliSecond.setUTCMilliseconds(115);
console.log(setUTCMilliSecond);
Output: 2023-12-17T13:50:05.115Z
// Here the UTCMilliSecond is 115 that you set above
37. toString()
The toString()
the method in JavaScript for Date
objects return a string representation of the date interpreted in the local timezone. It's important to be aware of this because it includes both the date and the time, unlike some of the other date formatting methods.
For Example:
const toStringDate = new Date();
console.log(`The Set UTCMilliSecond is : ${toStringDate.toString()}`);
Output:
The toStringDate is : Sun Dec 17 2023 20:58:01 GMT+0500 (Pakistan Standard Time)
// If you want to check its type then use typeof operator
const str = toStringDate.toString();
console.log(typeof str);
Output: string
38. toUTCString()
The toUTCString()
method is used in JavaScript to convert a Date
object into a string representation, specifically formatted according to Coordinated Universal Time (UTC).
For Example:
const toStringUTCDate = new Date();
console.log(`The toStringUTCDate is : ${toStringUTCDate.toUTCString()}`);
Output:The toStringUTCDate is : Sun, 17 Dec 2023 16:11:12 GMT
// It is UTC time not the Local.
// If you want to check its type then use typeof operator
const str = toStringUTCDate.toString();
console.log(typeof str);
Output: string
39. toTimeString()
The toTimeString()
method of the Date
object in JavaScript returns a string representation of the time portion of the given date object. It formats the time based on the local timezone and in English. It only includes the time portion.
For Example:
const toTimeString = new Date();
console.log(`The toTimeString is : ${toTimeString.toTimeString()}`);
Output: The toTimeString is : 21:33:09 GMT+0500 (Pakistan Standard Time)
// If you want to check its type then use typeof operator
const str = toTimeString.toTimeString();
console.log(typeof str);
Output: string
40. toDateString()
The toDateString()
method in JavaScript is used to convert an Date
object into a string representation of the date, formatted in the user's local timezone and language. It only includes the date portion (day, month, and year).
For Example:
const toDateString = new Date();
console.log(`The toDateString is : ${toDateString.toDateString()}`);
Output: The toDateString is : Sun Dec 17 2023
// If you want to check its type then use typeof operator
const str = toDateString.toDateString();
console.log(typeof str);
Output: string
41. toISOString()
The toISOString()
method in JavaScript is a very handy tool for converting an Date
object into a string in ISO 8601 format. This format is widely used for exchanging date and time information between different systems and programming languages because it is standardized. The resulting string will always be either 24 or 27 characters long, depending on whether milliseconds are included.
For Example:
const toISOString = new Date();
console.log(`The toISOString is : ${toISOString.toISOString()}`);
Output: The toISOString is : 2023-12-17T17:20:42.869Z
// If you want to check its type then use typeof operator
const str = toISOString.toISOString();
console.log(typeof str);
Output: string
42. toLocaleString()
The toLocaleString()
the method in JavaScript for specifically formats an Date
object as a string, using the current locale and default formatting options.
For Example:
const toLocaleString = new Date();
console.log(`The toLocaleString is : ${toLocaleString.toLocaleString()}`);
Output: The toLocaleString is : 12/18/2023, 1:23:55 AM
// If you want to check its type then use typeof operator
const str = toLocaleString.toLocaleString();
console.log(typeof str);
Output: string
43. toLocaleDateString()
The toLocaleDateString()
method in JavaScript is used to format a date object as a string, according to the conventions of the user's locale (language and region). It returns a string representation of the date portion of the Date
object, without the time.
For Example:
const toLocaleDtString = new Date();
console.log(`The toLocaleDtString is : ${toLocaleDtString.toLocaleDateString()}`);
Output: The toLocaleDtString is : 12/18/2023
// If you want to check its type then use typeof operator
const str = toLocaleDtString.toLocaleDateString();
console.log(typeof str);
Output: string
44. toLocaleTimeString()
The toLocaleTimeString()
method in JavaScript used to format the time portion of an Date
object according to the current locale settings. It returns a string representation of the time.
For Example:
const toLocaleTString = new Date();
console.log(`The toLocaleTString is : ${toLocaleTString.toLocaleTimeString()}`);
Output: The toLocaleTString is : 1:42:38 AM
// If you want to check its type then use typeof operator
const str = toLocaleTString.toLocaleTimeString();
console.log(typeof str);
Output: string
45. toJSON()
The toJSON()
the method in JavaScript is available on all Date
objects and plays a crucial role in converting dates to a format suitable for JSON serialization. This string follows the ISO 8601 format, which is a standardized format for representing dates and times. The format looks like this: YYYY-MM-DDTHH:MM:SS.mmmZ.
For Example:
const toJson = new Date();
console.log(`The toJson is : ${toJson.toJSON()}`);
Output: The toJson is : 2023-12-17T20:57:38.937Z
// If you want to check its type then use typeof operator
const str = toJson.toJSON();
console.log(typeof str);
Output: string
Note:
When you use JSON.stringify()
on an object containing a Date
object, toJSON()
is automatically called on the Date
object to convert it to the ISO 8601 format string. This ensures seamless integration of dates within your JSON data.
46. valueOf()
The valueOf()
method in JavaScript that returns the primitive value of the Date
object. In this case, the primitive value is the number of milliseconds that have elapsed since midnight on January 1, 1970 UTC (Unix epoch).
For Example:
const valOf = new Date();
console.log(`The valOf is : ${valOf.valueOf()}`);
Output: The valOf is : 1702847166375
Note:
valueOf()
is functionally equivalent to the getTime()
method of Date objects. Both methods return the same timestamp value.
47. UTC()
Date.UTC()
is a static method in JavaScript that accepts several parameters representing date and time components (similar to the Date constructor) and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC. It doesn't create a Date object. If there is no parameter then it returns NaN
.
For Example:
const UTCDate = Date.UTC(2024, 11, 20);
console.log(`The UTCDate is : ${UTCDate}`);
Output: The UTCDate is : 1734652800000
// With no parameter
const UTCDate = Date.UTC();
console.log(`The UTCDate is : ${UTCDate}`);
Output: The UTCDate is : NaN
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
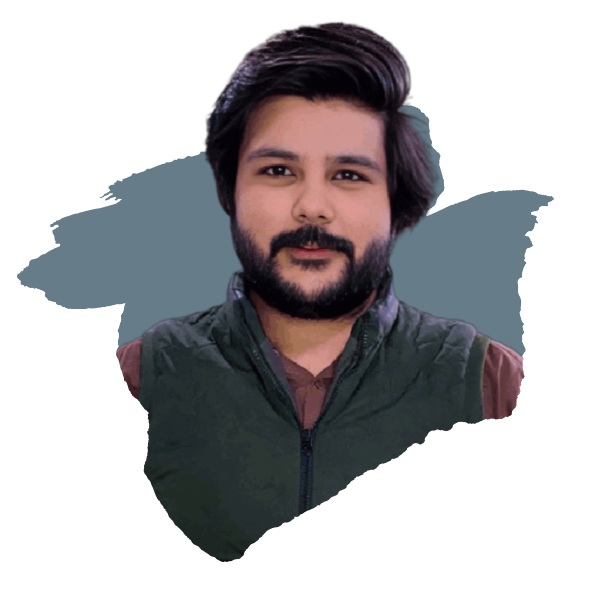
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. 📈 Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. 🔊 Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub 🖥 Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. ✨ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. 🎓 Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. 👥 Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.