Arithmetic operator | Java
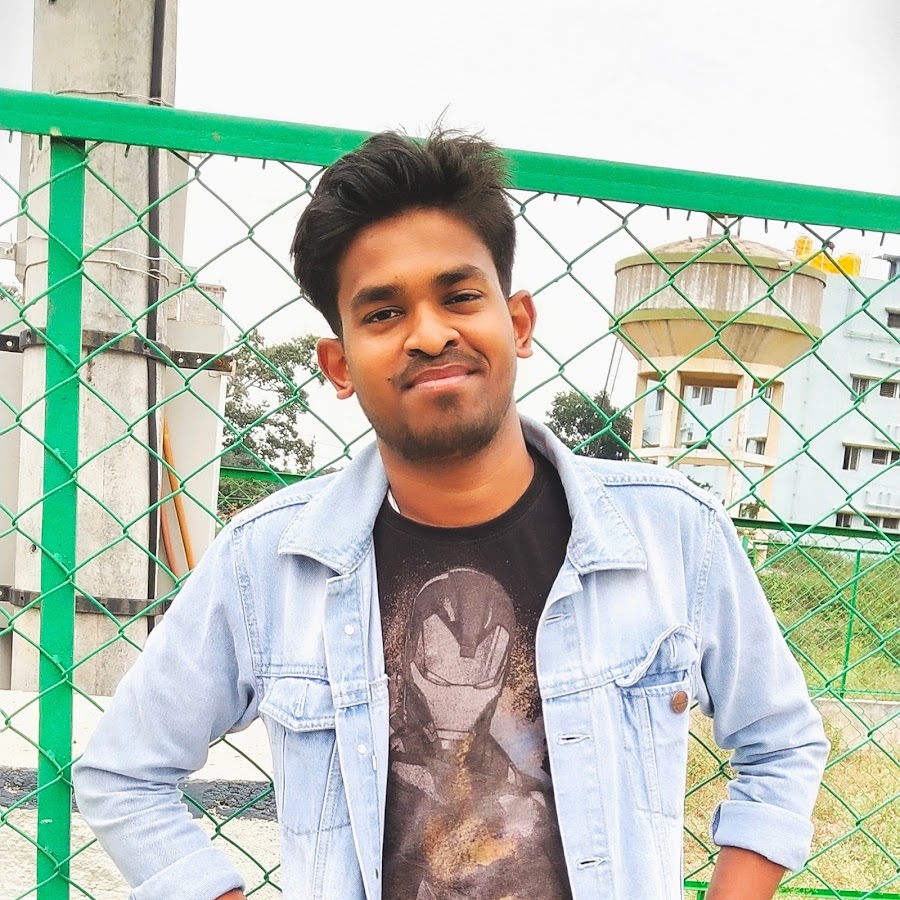
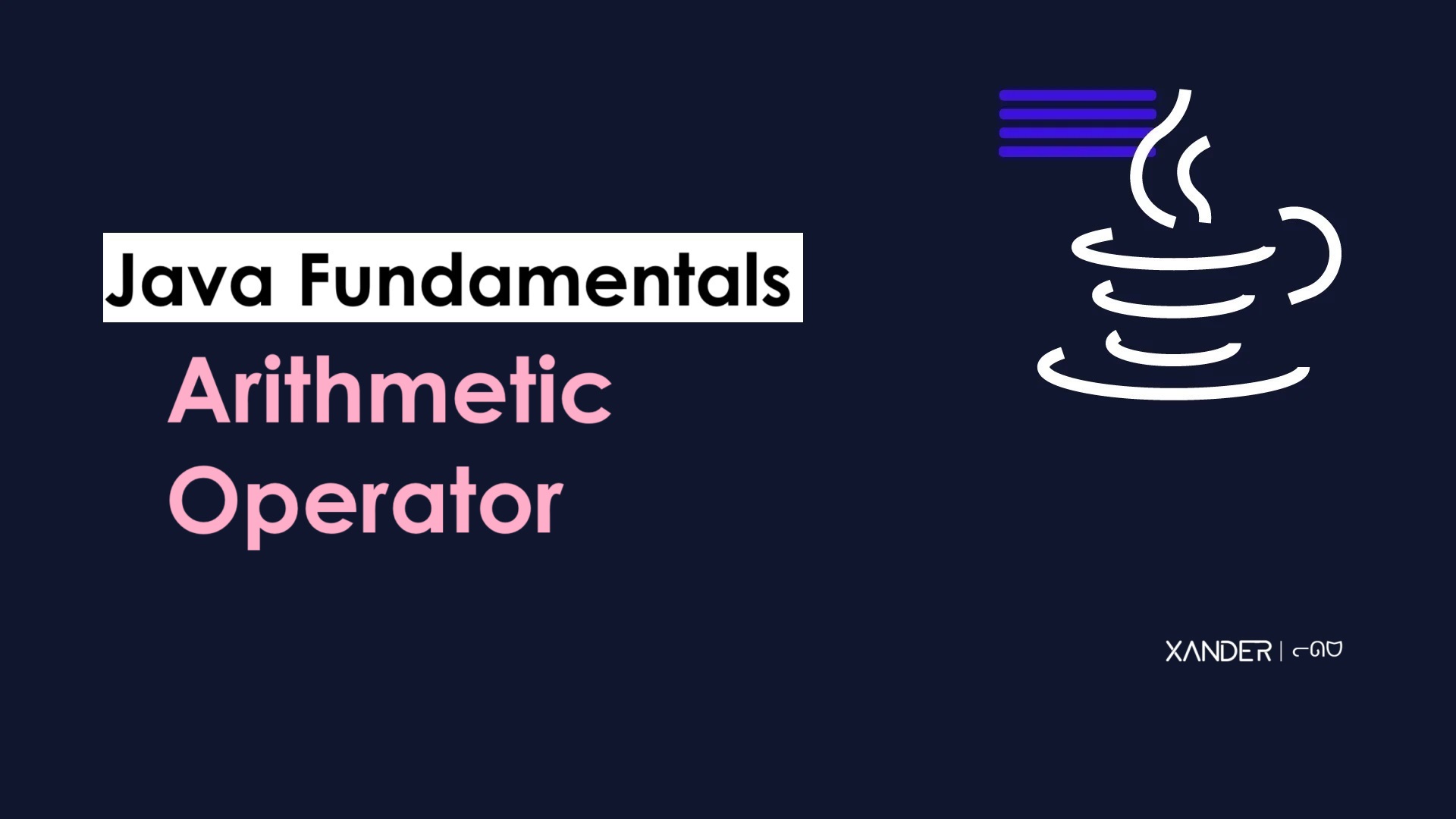
Arithmetic operators in Java are used to perform mathematical operations on numerical values. These operations include addition, subtraction, multiplication, division, and modulo (remainder). Here's an explanation of each arithmetic operator:
Addition
+
:Adds two operands.
Example:
int sum = 5 + 3; // sum is 8
Subtraction
-
:Subtracts the right operand from the left operand.
Example:
int difference = 7 - 4; // difference is 3
Multiplication
*
:Multiplies two operands.
Example:
int product = 2 * 6; // product is 12
Division
/
:Divides the left operand by the right operand.
Example:
int quotient = 10 / 2; // quotient is 5
Modulo
%
:Returns the remainder of the division of the left operand by the right operand.
Example:
int remainder = 10 % 3; // remainder is 1
Here's a more comprehensive example that demonstrates the use of various arithmetic operators:
public class ArithmeticExample {
public static void main(String[] args) {
int a = 10;
int b = 4;
int sum = a + b; // 14
int difference = a - b; // 6
int product = a * b; // 40
int quotient = a / b; // 2
int remainder = a % b; // 2
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
System.out.println("Remainder: " + remainder);
}
}
In this example, the variables a
and b
are used to demonstrate the various arithmetic operations, and the results are printed to the console. Keep in mind that integer division in Java truncates the fractional part, so the result of a / b
is an integer. If you need a floating-point result, you can use double
or float
variables.
If we apply any arithmetic operator between two variables
a
andb
the result type will be always -
max(type a, type b)
According to flowchart -
Data Type a | Data Type b | Resulting Data Type |
byte | byte | int |
byte | short | int |
short | byte | int |
char | char | int |
char | int | int |
byte | long | long |
long | double | double |
char | double | double |
Data type have same size less than int or equal to int will always result
int
.Data type have size more than the highest values will be considered.
Example -
public class ArithmeticExample {
public static void main(String[] args) {
System.out.println('a' + 'b'); //195
System.out.println('a' + 0.89); // 97.89
}
}
Infinity
It results in numbers divided by zero.
In Integral arithmetic that is (byte, short, int, long) there is no way to represent infinity. Hence, if Infinity is result we will get arithmetic exception i.e.,
ArithmeticException: / by zero
public class ArithmeticExample {
public static void main(String[] args) {
System.out.println(5 / 0); // ArithmeticException: / by zero
}
}
In floating point arithmetic (float and double) there is always a way to represent infinity.
For this float and double classes contains the following constants that is -
POSITIVE_INFINITY NEGATIVE_INFINITY
Health even though result is Infinity and we wouldn't get any arithmetic exception in floating point arithmetic.
public class Test {
public static void main(String[] args) {
System.out.println(10.0 / 0); // Infinity
System.out.println(-5 / 0.0); // -Infinity
}
}
Not a Number (NaN
)
It results when we divide zero by zero.
An integral arithmetic there is no way to undefine the result.
If the result is undefined we will get arithmetic exception i.e.,
ArithmeticException: / by zero
public class ArithmeticExample {
public static void main(String[] args) {
System.out.println(0 / 0); // ArithmeticException: / by zero
}
}
But in floating point arithmetic there is a way to represent undefined result.
For this flute and double classes contain
NaN
constant hence if the result is undefined we won't get any runtime exception in floating point arithmetic.
public class ArithmeticExample {
public static void main(String[] args) {
System.out.println(0.0 / 0); // NaN
System.out.println(-0.0 / 0); // NaN
}
}
Note:
For any X value including
NaN
the following expression returnsfalse
-x < NaN
,x > NaN
,x <= NaN
,x >= NaN
andx == NaN
.For any X value including
NaN
the following expression returnstrue
-x != NaN
.
public class Test {
public static void main(String[] args) {
System.out.println(10 < Float.NaN); // false
System.out.println(10 > Float.NaN); // false
System.out.println(10 <= Float.NaN); // false
System.out.println(10 >= Float.NaN); // false
System.out.println(10 == Float.NaN); // false
System.out.println(Float.NaN == Float.NaN);// false
System.out.println(10 != Float.NaN); // true
System.out.println(Float.NaN == Float.NaN);// false
}
}
Arithmetic exception
it is runtime exception not compile time error
It only occurred integral arithmetic but not in floating point.
The only operator which cause arithmetic exception are
/
and%
String concatenation operator (+
)
The only overrated operator in Java is
+
operator.sometime it act as arithmetic addition operator and some time it acts as a string concatenation operator.
If at least one argument is a string type then
+
operator act as Concatenation Operator and if both arguments are number type then+
operator act as arithmetic addition operator.
public class Test {
public static void main(String[] args) {
String s = "Hello";
int a = 10, b = 20, c = 30;
System.out.println(s + a + b + c); // Hello102030
System.out.println(a + s + b + c); // 10Hello2030
System.out.println(a + b + s + c); // 30Hello30
System.out.println(a + b + c + s); // 60Hello
}
}
Subscribe to my newsletter
Read articles from Xander Billa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
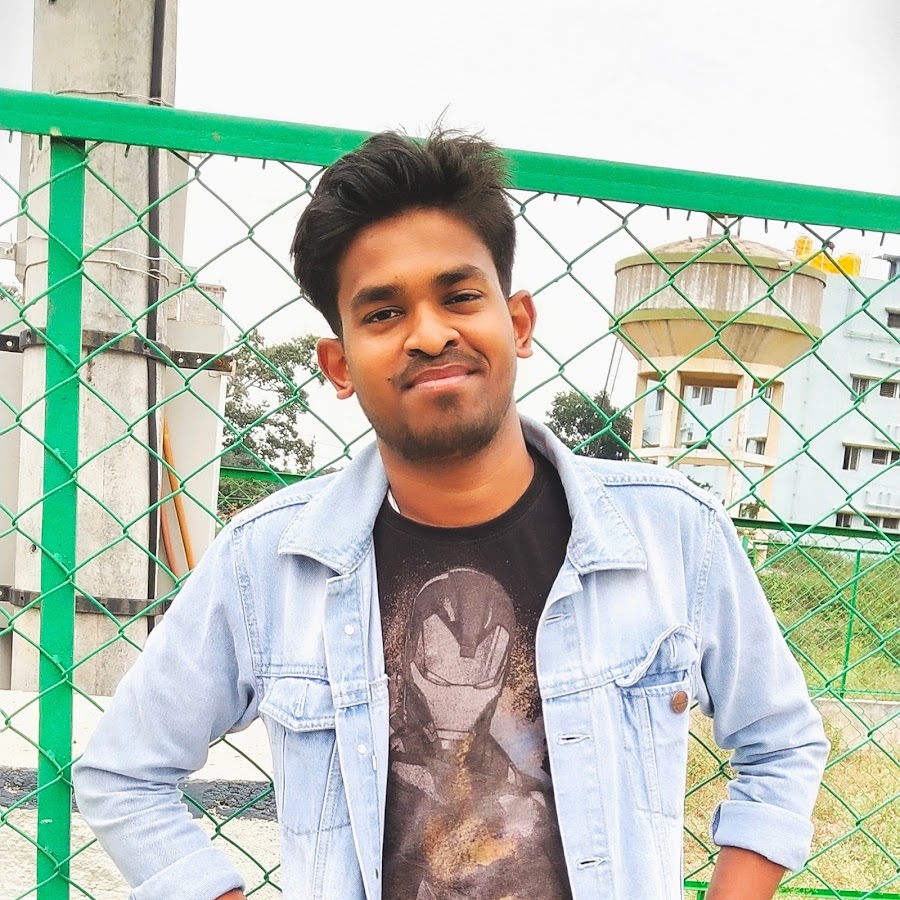
Xander Billa
Xander Billa
Myself Vikas Singh from Varanasi, Uttar Pradesh. Learning and exploring technical domains at Acharya Institute, Bangalore (IN) from the last two years. The main goal is to learn as much domains, tool