Relational operator | Java
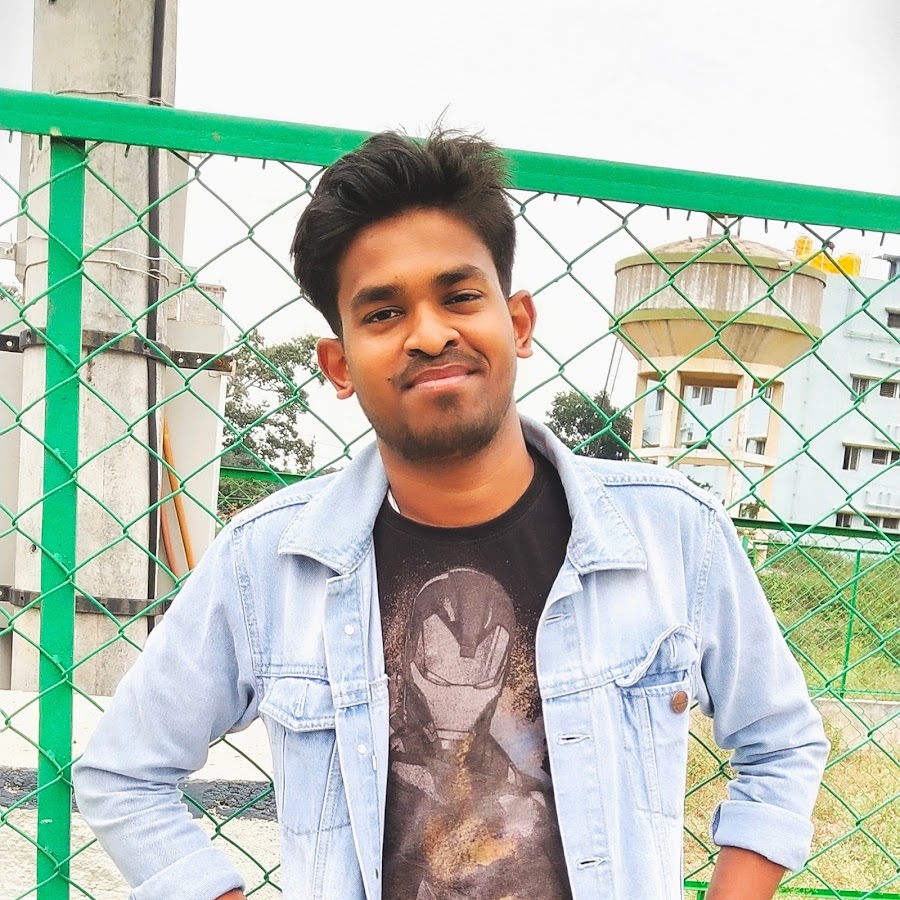
Table of contents
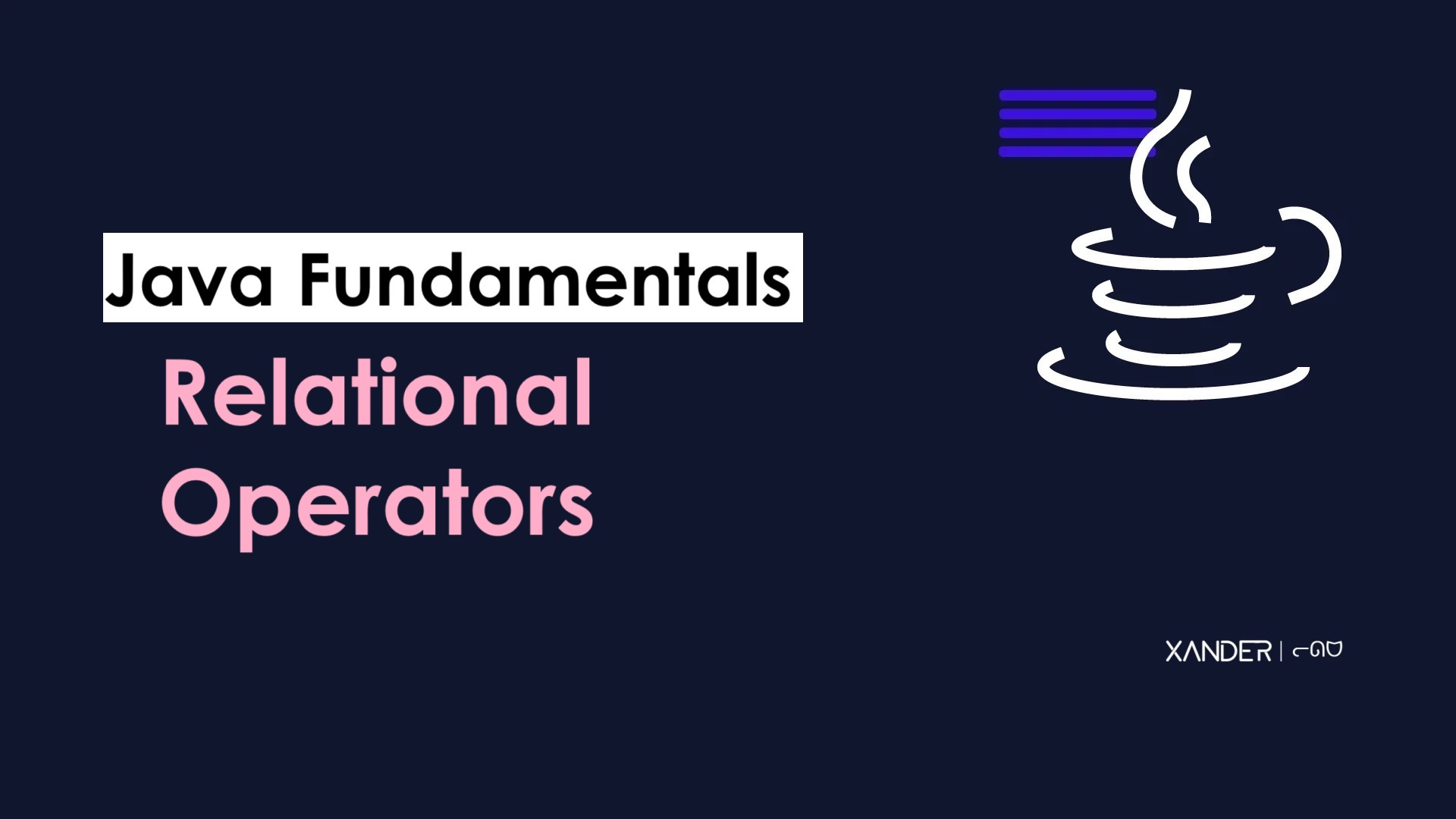
Relational operators in Java are used to compare two values and determine the relationship between them. These operators return a boolean result, indicating whether the specified relationship holds true or false. Here are the relational operators in Java:
Equality (
==
):- Checks if two values are equal.
int a = 5;
int b = 7;
boolean isEqual = (a == b); // false
Inequality (
!=
):- Checks if two values are not equal.
int x = 10;
int y = 10;
boolean isNotEqual = (x != y); // false
Greater Than (
>
):- Checks if the left operand is greater than the right operand.
int p = 15;
int q = 8;
boolean isGreaterThan = (p > q); // true
Less Than (
<
):- Checks if the left operand is less than the right operand.
int m = 5;
int n = 9;
boolean isLessThan = (m < n); // true
Greater Than or Equal To (
>=
):- Checks if the left operand is greater than or equal to the right operand.
int num1 = 12;
int num2 = 12;
boolean isGreaterOrEqual = (num1 >= num2); // true
Less Than or Equal To (
<=
):- Checks if the left operand is less than or equal to the right operand.
int value1 = 7;
int value2 = 10;
boolean isLessOrEqual = (value1 <= value2); // true
Remember
- We can apply relational operator for every primitive except boolean.
Example -
System.out.println(10 < 20); // true
System.out.println('a' < 20); // false
System.out.println('a' > 97); // false
System.out.println(true < false); // '<' cannot be applied to boolean
If we are comparing smaller data type and bigger data type - automatically smaller data type will be promoted to bigger data type.
We cant apply relational operators for object types.
System.out.println("true" < "false"); // '<' cannot be applied to boolean
In Java, a
String
is considered an object. While strings in many programming languages are often treated as primitive data types, Java takes a different approach. In Java, strings are instances of theString
class, which is part of the Java Standard Library (java.lang
package).
- Nesting of relation of it is not allowed otherwise we will get compiled time error.
System.out.println(10 < 20 < 30); // '<' cannot be applied to boolean
Relational operators are commonly used in decision-making structures, such as if
statements, loops, and conditional expressions. They help control the flow of a program based on the relationships between different values. The result of a relational expression is a boolean value (true
or false
).
Subscribe to my newsletter
Read articles from Xander Billa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
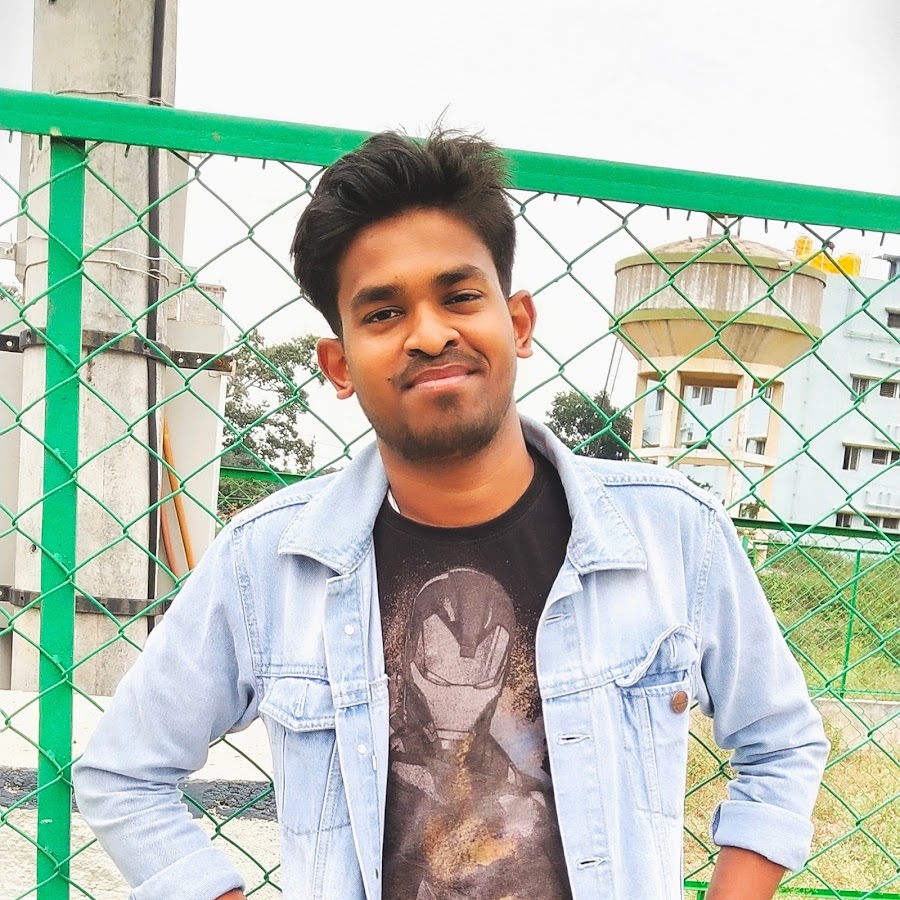
Xander Billa
Xander Billa
Myself Vikas Singh from Varanasi, Uttar Pradesh. Learning and exploring technical domains at Acharya Institute, Bangalore (IN) from the last two years. The main goal is to learn as much domains, tool