Configmaps & Secrets | How To Use Configmaps & Secrets Inside Pod

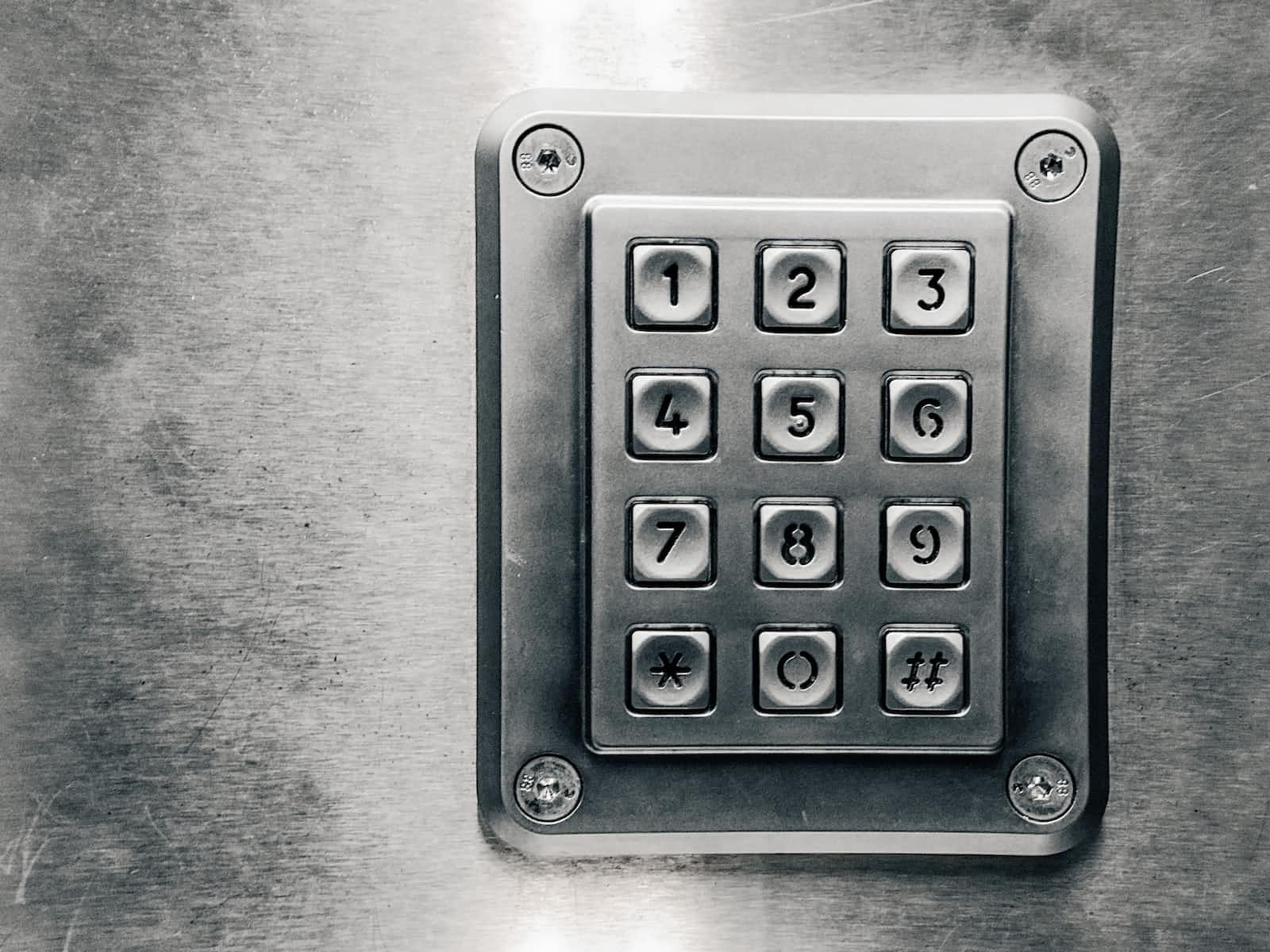
ConfigMaps and Secrets are Kubernetes resources used to manage configuration data and sensitive information within your applications. ConfigMaps are used for storing non-sensitive configuration data, while Secrets are specifically designed to handle sensitive information, such as passwords, API keys, and certificates.
Here's a step-by-step guide on how to use ConfigMaps and Secrets inside a pod:
ConfigMaps:
Create a ConfigMap: You can create a ConfigMap from literal values or by specifying a file.
Literal Values:
kubectl create configmap my-configmap --from-literal=key1=value1 --from-literal=key2=value2
From File:
kubectl create configmap my-configmap --from-file=path/to/config-files/
View ConfigMap:
kubectl get configmap my-configmap kubectl describe configmap my-configmap
Use ConfigMap in a Pod: You can use ConfigMaps in your pod definition YAML file:
apiVersion: v1 kind: Pod metadata: name: mypod spec: containers: - name: mycontainer image: myimage envFrom: - configMapRef: name: my-configmap
Secrets:
Create a Secret: Secrets can be created using literals, files, or a combination of both.
Literal Values:
kubectl create secret generic my-secret --from-literal=username=myuser --from-literal=password=mypassword
From File:
kubectl create secret generic my-secret --from-file=path/to/secret-files/
View Secret:
kubectl get secret my-secret kubectl describe secret my-secret
Use Secret in a Pod: Reference the secret in your pod definition YAML file:
apiVersion: v1 kind: Pod metadata: name: mypod spec: containers: - name: mycontainer image: myimage envFrom: - secretRef: name: my-secret
Mount Secret as Volume: You can also mount a secret as a volume in a pod:
apiVersion: v1 kind: Pod metadata: name: mypod spec: containers: - name: mycontainer image: myimage volumeMounts: - name: my-secret-volume mountPath: /etc/secrets volumes: - name: my-secret-volume secret: secretName: my-secrets
Mounting a Secret as a Volume in a Pod:
Explanation:
Volumes Section: In the
volumes
section of the pod definition, a volume namedmy-secret-volume
is defined. This volume is associated with themy-secret
Secret.volumes: - name: my-secret-volume secret: secretName: my-secret
VolumeMounts Section: In the
volumeMounts
section of the container definition, the volumemy-secret-volume
is mounted at the path/etc/secrets
within the container.volumeMounts: - name: my-secret-volume mountPath: /etc/secrets
This means that the contents of the Secret will be available to the application running in the container at the path
/etc/secrets
. You can then reference these files in your application's configuration.Creating a Generic Secret:
bashCopy codekubectl create secret generic my-api-key-secret --from-literal=api-key=your-api-key-value
This command creates a generic secret named my-api-key-secret
with a key-value pair for the API key.
Using the Secret in a Pod:
Now, let's create a pod that uses this secret:
apiVersion: v1
kind: Pod
metadata:
name: mypod
spec:
containers:
- name: mycontainer
image: myimage
env:
- name: API_KEY
valueFrom:
secretKeyRef:
name: my-api-key-secret
key: api-key
Explanation:
Environment Variable: We're defining an environment variable named
API_KEY
in the pod's container.yamlCopy codeenv: - name: API_KEY valueFrom: secretKeyRef: name: my-api-key-secret key: api-key
SecretKeyRef: The
valueFrom
field specifies that the value of the environment variable comes from a secret. ThesecretKeyRef
field then indicates the name of the secret (my-api-key-secret
) and the specific key (api-key
) within that secret.ConfigMap Interview Questions:
What is a ConfigMap in Kubernetes?
- Answer: A ConfigMap is a Kubernetes resource used to store configuration data in key-value pairs. It allows you to decouple configuration from application code and manage it independently.
How can you create a ConfigMap from literal values using
kubectl
?Answer: You can use the
kubectl create configmap
command with the--from-literal
flag. For example:bashCopy codekubectl create configmap my-configmap --from-literal=key1=value1 --from-literal=key2=value2
Explain how to use a ConfigMap in a pod.
Answer: You can use a ConfigMap in a pod by referencing it in the pod definition's
env
orenvFrom
field. For example, usingenv
:yamlCopy codeenv: - name: KEY1 valueFrom: configMapKeyRef: name: my-configmap key: key1
What are the advantages of using ConfigMaps?
- Answer: ConfigMaps provide a centralized and decoupled way to manage configuration data, making it easier to update configurations without modifying the application code. They also support dynamic updates without restarting pods.
Secrets Interview Questions:
What is a Secret in Kubernetes?
- Answer: A Secret is a Kubernetes resource designed to store and manage sensitive information, such as passwords, API keys, and certificates, in a secure manner.
How can you create a Secret using
kubectl
from literal values?Answer: You can use the
kubectl create secret generic
command with the--from-literal
flag. For example:bashCopy codekubectl create secret generic my-secret --from-literal=username=myuser --from-literal=password=mypassword
Explain how to use a Secret in a pod.
Answer: You can use a Secret in a pod by referencing it in the pod definition's
env
orenvFrom
field. For example, usingenv
:yamlCopy codeenv: - name: DB_PASSWORD valueFrom: secretKeyRef: name: my-secret key: password
What are the differences between ConfigMaps and Secrets?
- Answer: ConfigMaps are intended for non-sensitive configuration data, while Secrets are designed for storing sensitive information. Secrets provide additional security measures, such as encryption, and are base64-encoded by default.
Real-Time Scenario Questions:
In a scenario where an application requires access to an API key, would you use a ConfigMap or a Secret, and why?
- Answer: I would use a Secret because API keys are considered sensitive information, and Secrets provide additional security features, such as encryption, making them more suitable for storing confidential data.
Explain a situation where you might choose to use a ConfigMap to configure an application.
Answer: ConfigMaps are suitable when dealing with non-sensitive configuration data, such as environment-specific settings, feature flags, or other parameters that do not involve sensitive information. For instance, setting the base URL for an external service.
NOTE-
Remember that secrets encode data in base64 format. Anyone with the base64 encoded secret can easily decode it. As such the secrets can be considered as not very safe.
The concept of safety of the Secrets is a bit confusing in Kubernetes. The kubernetes documentation page and a lot of blogs out there refer to secrets as a "safer option" to store sensitive data. They are safer than storing in plain text as they reduce the risk of accidentally exposing passwords and other sensitive data. In my opinion it's not the secret itself that is safe, it is the practices around it.
Secrets are not encrypted, so it is not safer in that sense. However, some best practices around using secrets make it safer. As in best practices like:
Not checking-in secret object definition files to source code repositories.
Enabling Encryption at Rest for Secrets so they are stored encrypted in ETCD.
Also the way kubernetes handles secrets. Such as:
A secret is only sent to a node if a pod on that node requires it.
Kubelet stores the secret into a tmpfs so that the secret is not written to disk storage.
Once the Pod that depends on the secret is deleted, kubelet will delete its local copy of the secret data as well.
Read about the protections and risks of using secrets here
Having said that, there are other better ways of handling sensitive data like passwords in Kubernetes, such as using tools like Helm Secrets, HashiCorp Vault.
Subscribe to my newsletter
Read articles from krishnapal rawat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

krishnapal rawat
krishnapal rawat
Pushing code to its limits, one test at a time - I'm a QA engineer with a passion for coding and testing