Automating JIRA Creation on a GitHub Event using Python | Python For DevOps

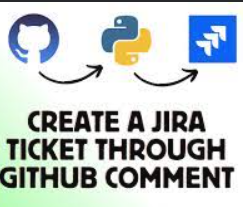
Introduction
In the ever-evolving landscape of DevOps, the emphasis on automation has become a cornerstone for improving efficiency and collaboration within development teams. One powerful way to achieve this is by integrating popular tools like GitHub and JIRA. In this blog post, we'll guide you through the process of creating a Flask app with GitHub-JIRA integration using Python, enabling the automatic creation of JIRA issues when a comment is added to a GitHub issue.
Prerequisites
Before we dive into the implementation details, make sure you have the following prerequisites in place:
A GitHub repository with issues enabled.
A JIRA account and an API token for authentication.
Python installed on your local machine.
Flask and Requests Python packages installed (
pip install Flask requests
).
Creating the Flask App
Let's begin by setting up a simple Flask app that listens for GitHub events. The app will trigger the creation of a JIRA issue when a comment is added to a GitHub issue. You can find the complete code for the Flask app here.
# Import necessary libraries
from flask import Flask, request
import requests
from requests.auth import HTTPBasicAuth
import json
# Create a Flask app
app = Flask(__name__)
# Define a route that handles POST requests
@app.route('/createJira', methods=['POST'])
def create_jira():
# JIRA API endpoint and credentials
url = "https://your-jira-instance.atlassian.net/rest/api/3/issue"
API_TOKEN = "your-jira-api-token"
auth = HTTPBasicAuth("<email>", API_TOKEN)
# Headers for the JIRA API request
headers = {
"Accept": "application/json",
"Content-Type": "application/json"
}
# Get the JSON payload from the GitHub webhook
webhook = request.json
# Check if the comment body starts with /jira
if webhook.get('comment') and webhook['comment'].get('body', '').startswith("/jira"):
# Prepare payload for JIRA issue creation
payload = json.dumps({
"fields": {
"description": {
"content": [
{
"content": [
{
"text": "Order entry fails when selecting supplier.",
"type": "text"
}
],
"type": "paragraph"
}
],
"type": "doc",
"version": 1
},
"project": {
"key": "KKPP"
},
"issuetype": {
"id": "10006"
},
"summary": "Main order flow broken in kp environment",
},
"update": {}
})
# Make a request to create a JIRA issue
response = requests.post(
url,
data=payload,
headers=headers,
auth=auth
)
# Check if the JIRA issue creation was successful
if response.ok:
return json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(",", ": "))
else:
return f"Failed to create JIRA issue. Status code: {response.status_code}"
return "JIRA issue will be created if the comment starts with /jira"
# Run the Flask app
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
GitHub-JIRA Integration
Setting up GitHub Webhooks
To receive GitHub events, we need to set up a webhook in our GitHub repository. Navigate to your repository settings, go to the "Webhooks" section, and add a new webhook. Set the Payload URL to the public URL of your Flask app/or url of EC2 Instance in my case i am working on ec2 so i added a url of my ec2.
JIRA API Integration
For JIRA integration, generate an API token in your JIRA account. Replace the placeholder API token in the Flask app code with your actual token.
Making It Work
Once everything is set up, start your Flask app. It will now listen for GitHub events. When a comment is added to a GitHub issue and the comment body starts with "/jira," the Flask app will make a request to the JIRA API to create a new issue.
run python file
add comment and see the results on jira-
Conclusion
In this tutorial, we've demonstrated how to automate JIRA issue creation based on GitHub events using Python and Flask. This integration enhances collaboration and ensures that project management remains efficient. As you explore and adapt this solution for your team, consider experimenting with different triggers, customizations, and error handling to tailor the solution to your specific needs.
Subscribe to my newsletter
Read articles from krishnapal rawat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

krishnapal rawat
krishnapal rawat
Pushing code to its limits, one test at a time - I'm a QA engineer with a passion for coding and testing