Introduction to AsyncStorage SQL And SQLite in React Native
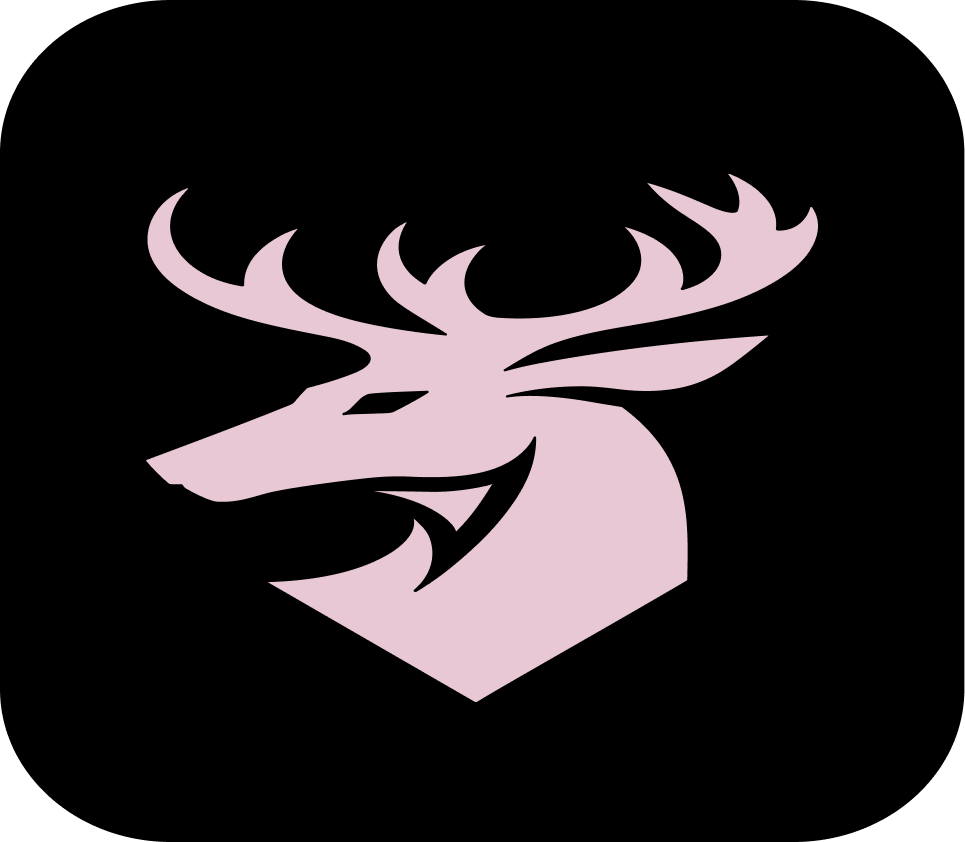
AsyncStorage is a simple, unencrypted, asynchronous, persistent, key-value storage system in React Native that is global to the app. It is used to store small amounts of data persistently, which means the data will still be available even if the app is closed or the device is restarted. It’s similar to LocalStorage in web development.
Here’s a simple example of how to use AsyncStorage:
import AsyncStorage from '@react-native-async-storage/async-storage';
// Store data
const storeData = async (value) => {
try {
await AsyncStorage.setItem('@storage_Key', value)
} catch (e) {
// saving error
}
}
// Read data
const getData = async () => {
try {
const value = await AsyncStorage.getItem('@storage_Key')
if(value !== null) {
// value previously stored
}
} catch(e) {
// error reading value
}
}
However, AsyncStorage is not designed to store large amounts of structured data. For that, you might want to use a database. React Native doesn’t support SQL databases out of the box, but you can use libraries like react-native-sqlite-storage
for SQLite, or ORMs like WatermelonDB
or TypeORM
that support SQLite and can provide a more structured and powerful way to interact with your data.
For example, with react-native-sqlite-storage
, you can open a database and execute SQL commands like this:
import SQLite from 'react-native-sqlite-storage';
// Open database
let db = SQLite.openDatabase({name: 'my.db', location: 'default'});
// Execute SQL
db.transaction((tx) => {
tx.executeSql('CREATE TABLE IF NOT EXISTS MyTable (id INTEGER PRIMARY KEY, name TEXT)', [], (tx, results) => {
console.log('Table created successfully');
});
});
Remember, choosing between AsyncStorage and a SQL database depends on your specific needs. If you just need to store small amounts of data and don’t need complex queries, AsyncStorage might be enough. But if you need to store large amounts of structured data and perform complex queries, a SQL database might be a better choice.
Some popular SQL databases that can be used with React Native? and integration code
There are several popular SQL databases that can be used with React Native. Here are a few along with their integration code:
- SQLite with react-native-sqlite-storage: SQLite is a C library that provides a lightweight disk-based database. It doesn’t require a separate server process and allows accessing the database using a nonstandard variant of the SQL query language.
import SQLite from 'react-native-sqlite-storage';
let db = SQLite.openDatabase({name: 'my.db', location: 'default'});
db.transaction((tx) => {
tx.executeSql('CREATE TABLE IF NOT EXISTS MyTable (id INTEGER PRIMARY KEY, name TEXT)', [], (tx, results) => {
console.log('Table created successfully');
});
});
2. WatermelonDB: WatermelonDB is a high-performance, observable database for React and React Native apps. It’s built on top of SQLite, but provides a more user-friendly API and advanced features like lazy loading and advanced querying.
import { Database } from '@nozbe/watermelondb';
import SQLiteAdapter from '@nozbe/watermelondb/adapters/sqlite';
const adapter = new SQLiteAdapter({
dbName: 'WatermelonDemo',
schema: mySchema,
});
const database = new Database({
adapter,
modelClasses: [MyModel],
actionsEnabled: true,
});
3. TypeORM: TypeORM is an ORM that can run in NodeJS, Browser, Cordova, PhoneGap, Ionic, React Native, NativeScript, Expo, and Electron platforms and can be used with TypeScript and JavaScript. It supports several SQL databases, including SQLite.
import {createConnection} from "typeorm";
createConnection({
type: "react-native",
database: "test",
location: "default",
logging: ["error", "query", "schema"],
synchronize: true,
entities: [
// Your entities here
]
}).then(() => {
// You can start to use your connection here
}).catch(error => console.log(error));
Remember, choosing a database depends on your specific needs. If you need to store large amounts of data and perform complex queries, a SQL database might be a better choice. But if you just need to store small amounts of data and don’t need complex
Some popular NoSQL databases that can be used with React Native? and integrateion code
Here are some popular NoSQL databases that can be used with React Native along with their integration code:
- Firebase with react-native-firebase: Firebase is a NoSQL cloud database to store and sync data between your users in realtime.
import firebase from '@react-native-firebase/app';
import '@react-native-firebase/database';
// Initialize Firebase
const firebaseConfig = {
// Your firebase configuration
};
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
}
// Get a reference to the database service
const database = firebase.database();
// Write data
database.ref('/path').set({
key: 'value',
});
// Read data
database.ref('/path').on('value', (snapshot) => {
console.log(snapshot.val());
});
2. PouchDB: PouchDB is a JavaScript database that syncs. It’s modeled after CouchDB — a NoSQL database that powers npm.
import PouchDB from 'pouchdb-react-native';
const db = new PouchDB('mydb');
// Write data
db.put({
_id: 'doc1',
name: 'name',
});
// Read data
db.get('doc1').then((doc) => {
console.log(doc);
});
- MongoDB with mongodb-stitch-react-native-sdk: MongoDB is a source-available cross-platform document-oriented database program. MongoDB has a cloud version called MongoDB Atlas.
import {Stitch, RemoteMongoClient} from 'mongodb-stitch-react-native-sdk';
// Initialize the MongoDB stitch app
const client = Stitch.initializeDefaultAppClient('<your-app-id>');
// Get a MongoDB service client
const mongoClient = client.getServiceClient(
RemoteMongoClient.factory,
'mongodb-atlas',
);
// Get a reference to the database
const db = mongoClient.db('dbname');
// Write data
db.collection('collection').insertOne({
key: 'value',
});
// Read data
db.collection('collection')
.find({key: 'value'}, {limit: 1})
.asArray()
.then(docs => {
console.log(docs);
});
Remember, choosing a database depends on your specific needs. If you need to store large amounts of data and perform complex queries, a NoSQL database might be a better choice. But if you just need to store small amounts of data and don’t need complex queries, AsyncStorage might be enough.
The advantages of using a NoSQL database over a SQL database in a React Native app?
There are several advantages of using a NoSQL database over a SQL database in a React Native app:
- Scalability: NoSQL databases are generally more scalable and provide superior performance, and thus are a good choice for large or ever-changing data sets.
2. Flexibility: NoSQL databases allow you to store complex, unstructured, and semi-structured data with a dynamic schema. This is useful when the data doesn’t fit neatly into a table, or when the schema may change over time.
3. Real-time: NoSQL databases, like Firebase, often offer real-time capabilities, making them a good choice for apps that require real-time updates.
4. Offline Support: Some NoSQL databases, like PouchDB and Firebase, provide out-of-the-box support for offline synchronization. This is useful for mobile apps, which often need to work offline.
5. JSON Data: Many NoSQL databases, like MongoDB and CouchDB, store data in a format similar to JSON, which can be easier to work with in a JavaScript environment like React Native.
6. Speed: NoSQL databases can be faster than SQL databases for certain types of operations, particularly when dealing with large amounts of unstructured data.
However, SQL databases also have their own advantages, such as ACID transactions, support for complex joins, and a standardized language that is well-known among developers. The choice between SQL and NoSQL depends on the specific needs of your application.
Some popular libraries for working with SQLite in React Native?
There are several libraries available for working with SQLite in React Native. Here are a few popular ones:
- react-native-sqlite-storage: This is a robust SQLite client for React Native. It supports both iOS and Android, and provides a simple, promise-based API for SQLite operations.
import SQLite from 'react-native-sqlite-storage';
let db = SQLite.openDatabase({name: 'my.db', location: 'default'});
db.transaction((tx) => {
tx.executeSql('CREATE TABLE IF NOT EXISTS MyTable (id INTEGER PRIMARY KEY, name TEXT)', [], (tx, results) => {
console.log('Table created successfully');
});
});
2. react-native-sqlite-2: This is a lightweight plugin that provides a WebSQL-compatible API. It’s a good choice if you’re porting an existing WebSQL database to React Native.
import SQLite from 'react-native-sqlite-2';
const db = SQLite.openDatabase('test.db', '1.0', '', 1);
db.transaction(function(txn) {
txn.executeSql('CREATE TABLE IF NOT EXISTS Users(user_id INTEGER PRIMARY KEY NOT NULL, name VARCHAR(30))', []);
});
3. WatermelonDB with SQLite Adapter: WatermelonDB is a high-performance, observable database for React and React Native apps. It’s built on top of SQLite, but provides a more user-friendly API and advanced features
import { Database } from '@nozbe/watermelondb';
import SQLiteAdapter from '@nozbe/watermelondb/adapters/sqlite';
const adapter = new SQLiteAdapter({
dbName: 'WatermelonDemo',
schema: mySchema,
});
const database = new Database({
adapter,
modelClasses: [MyModel],
actionsEnabled: true,
});
4. TypeORM with React Native: TypeORM is an ORM that can run in NodeJS, Browser, Cordova, PhoneGap, Ionic, React Native, NativeScript, Expo, and Electron platforms and can be used with TypeScript and JavaScript. It supports several SQL databases, including SQLite.
import {createConnection} from "typeorm";
createConnection({
type: "react-native",
database: "test",
location: "default",
logging: ["error", "query", "schema"],
synchronize: true,
entities: [
// Your entities here
]
}).then(() => {
// You can start to use your connection here
}).catch(error => console.log(error));
Each of these libraries has its own strengths and weaknesses, so the best one to use depends on your specific needs.
Advantage and different between AsyncStorage SQL And SQLite in React Native:
AsyncStorage:
AsyncStorage is a simple, unencrypted, asynchronous, persistent, key-value storage system that is global to the app. It’s used for small data storage.
It’s not recommended for storing sensitive information, as it’s not encrypted.
It’s not meant for storing large amounts of structured data. For that, consider using a database.
It doesn’t support querying the data, you can only save, retrieve, and remove items.
SQLite:
SQLite is a C library that provides a lightweight disk-based database. It doesn’t require a separate server process and allows accessing the database using a nonstandard variant of the SQL query language.
It’s a good choice for storing larger amounts of structured data.
It supports complex queries, transactions, and other features you’d expect from a SQL database.
It’s stored in a single file, making it easy to share or copy.
SQL (General):
SQL databases are relational databases that use structured query language (SQL) for defining and manipulating the data.
They are good for handling complex queries and transactions.
They provide ACID (Atomicity, Consistency, Isolation, Durability) properties.
They are generally more robust and feature-rich compared to SQLite and AsyncStorage.
In summary, the choice between AsyncStorage, SQLite, and a full SQL database depends on your specific needs. If you just need to store small amounts of data and don’t need complex queries, AsyncStorage might be enough. If you need to store larger amounts of data and perform complex queries, a SQL database might be a better choice. SQLite sits somewhere in between, providing more
The performance considerations when choosing between AsyncStorage and SQLite in React Native?
When choosing between AsyncStorage and SQLite in React Native, there are several performance considerations to keep in mind:
Data Size: AsyncStorage is designed for small amounts of data. It’s not efficient for large data sets. SQLite, on the other hand, is a disk-based database that can handle larger amounts of data more efficiently.
Data Structure: AsyncStorage only supports key-value pairs, which can be inefficient if you have complex or relational data. SQLite supports structured data with tables, which can be more efficient for complex data.
Query Capability: AsyncStorage does not support queries. Every time you need data, you have to load it into memory. SQLite supports SQL queries, which can be more efficient because you only load the data you need.
Concurrency: AsyncStorage operations are asynchronous and non-blocking, but only one operation can be active at a time, which can lead to performance issues if you have a lot of concurrent operations. SQLite operations can also be asynchronous, and multiple queries can be executed at the same time.
Transactions: SQLite supports transactions, which can be more efficient if you have multiple operations that need to be performed together. AsyncStorage does not support transactions.
Data Persistence: Both AsyncStorage and SQLite provide data persistence, but SQLite might be a better choice if you need to ensure data integrity and reliability, as it provides ACID (Atomicity, Consistency, Isolation, Durability) properties.
In general, if you only need to store small amounts of simple data and don’t need advanced querying or transaction capabilities, AsyncStorage might be sufficient. However, if you’re dealing with larger amounts of data, complex data structures, or need advanced querying or transaction capabilities, SQLite would be a better choice.
Subscribe to my newsletter
Read articles from Ofido Hub directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
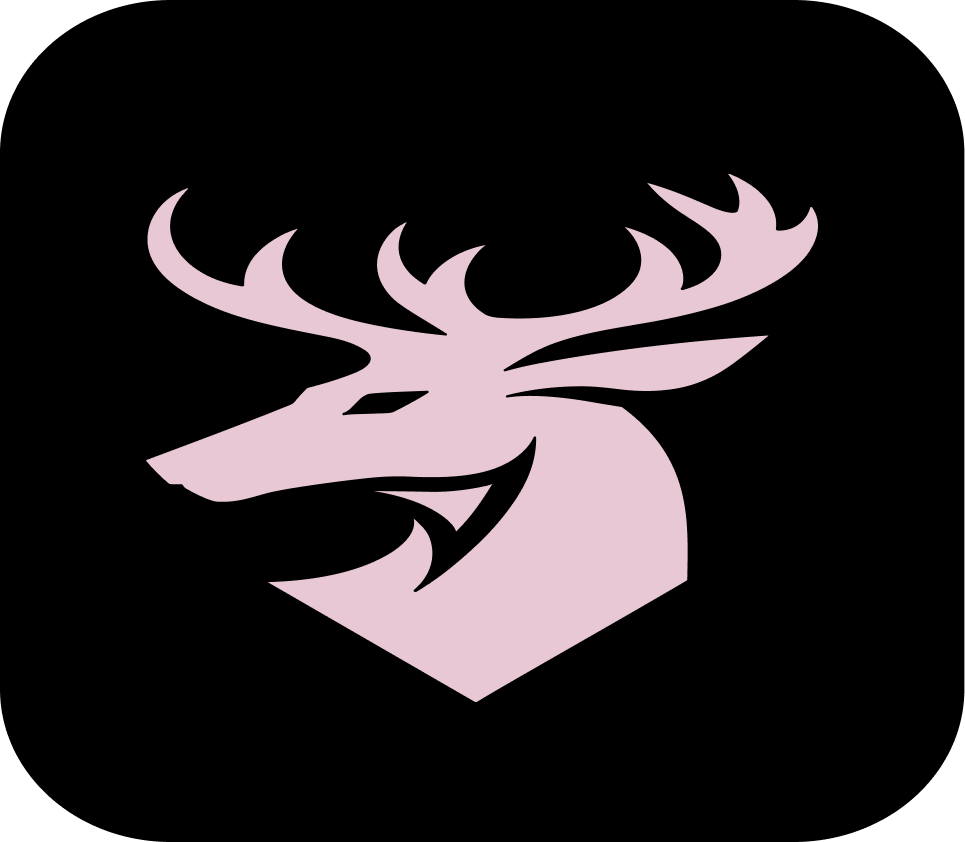
Ofido Hub
Ofido Hub
Passionate web and software developer with a knack for transforming ideas into innovative digital solutions. With a deep understanding of coding languages such as HTML, CSS, JavaScript, and proficiency in various frameworks and libraries, I specialize in creating dynamic and user-friendly websites and applications. With a keen eye for design and a focus on efficient functionality, I strive to deliver seamless user experiences. Constantly exploring emerging technologies and staying up-to-date with industry trends, I am committed to delivering high-quality, scalable solutions that empower businesses and drive their success. Let's collaborate and bring your digital vision to life!"