JavaScript Interview Related Important Topics
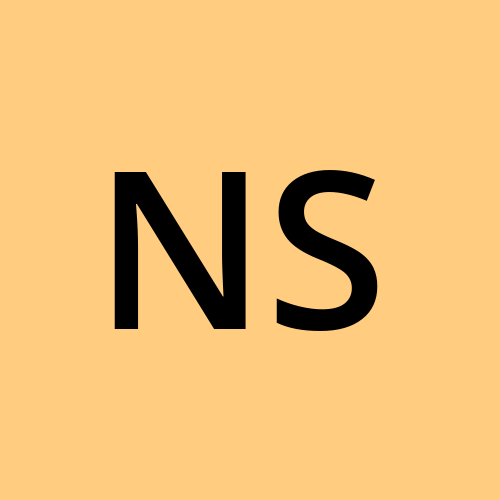
Core JavaScript Concepts:
Data Types and Variables: Understand primitive and reference data types, variable declaration, and scoping (var, let, const).
Functions: Function declaration, expression, anonymous functions, arrow functions, closures, and higher-order functions.
Object-Oriented Concepts: Prototypal inheritance, constructors, classes (ES6), methods, and properties.
Arrays and Objects: Manipulating arrays, array methods (map, filter, reduce), object manipulation, and iteration.
Asynchronous JavaScript:
Promises: Understand promises, chaining, error handling, and working with asynchronous operations.
Async/Await: Working with asynchronous code using async/await syntax for handling promises.
Callbacks: Understanding callback functions and their usage in asynchronous operations.
Event Loop: Understand the event loop, single-threaded nature, and non-blocking I/O in JavaScript.
DOM Manipulation and Events:
DOM Selection and Manipulation: Accessing and manipulating DOM elements, modifying content, and attributes.
Event Handling: Managing events, event propagation, event listeners, and handling user interactions.
Event Bubbling and Capturing: Understanding event flow and bubbling, event delegation, and capturing.
ES6+ Features:
Arrow Functions: Syntax and benefits of arrow functions.
Template Literals: Creating dynamic strings using template literals.
Destructuring: Extracting data from arrays/objects using destructuring.
Spread and Rest Operators: Usage and benefits of spread and rest operators.
Modules: Understanding ES6 modules for better code organization.
Error Handling and Debugging:
Error Handling: Handling errors using try...catch and throwing custom errors.
Debugging Tools: Familiarity with browser developer tools for debugging JavaScript.
Web APIs and Asynchronous Operations:
AJAX and Fetch API: Making asynchronous requests using Fetch API or XMLHttpRequest.
Local Storage and Session Storage: Working with browser storage for client-side data storage.
Web Workers: Understanding the use of web workers for multi-threaded JavaScript execution.
Frameworks and Libraries:
- React, Vue, Angular: Familiarity with popular JavaScript frameworks and libraries.
JavaScript Design Patterns:
Module Pattern: Implementing modular code using design patterns like Module Pattern, Revealing Module Pattern, etc.
Singleton Pattern: Understanding and implementing the Singleton design pattern.
Observer Pattern: Understanding event-driven architectures using Observer pattern concepts.
Security and Performance:
Cross-site Scripting (XSS): Understanding and preventing XSS attacks.
Performance Optimization: Techniques for optimizing JavaScript code for better performance.
Testing and Debugging:
Unit Testing: Writing and executing unit tests using testing libraries/frameworks (Jest, Jasmine, Mocha, etc.).
Debugging Tools: Proficiency with debugging tools and techniques for JavaScript.
JavaScript Interview Practice:
- Coding Challenges: Practice solving coding challenges and algorithms in JavaScript.
Subscribe to my newsletter
Read articles from Namya Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
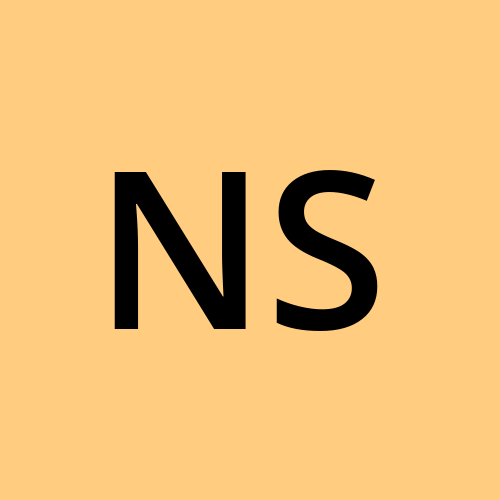
Namya Shah
Namya Shah
I am a developer who is very enthusiast about technology and coding.