Demystifying JavaScript's Event Loop: Navigating the Event Loop and Callback Queues (Part 1)
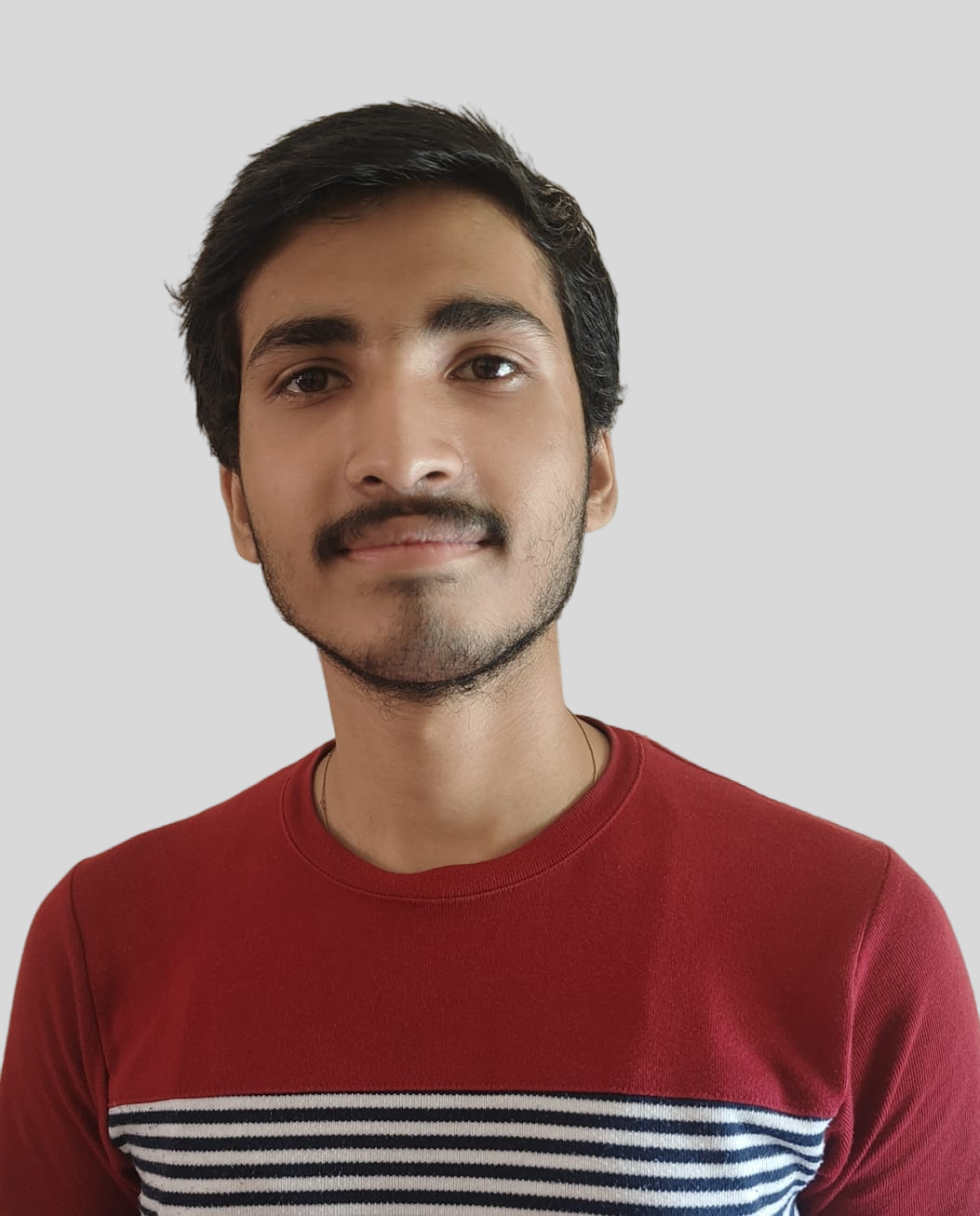
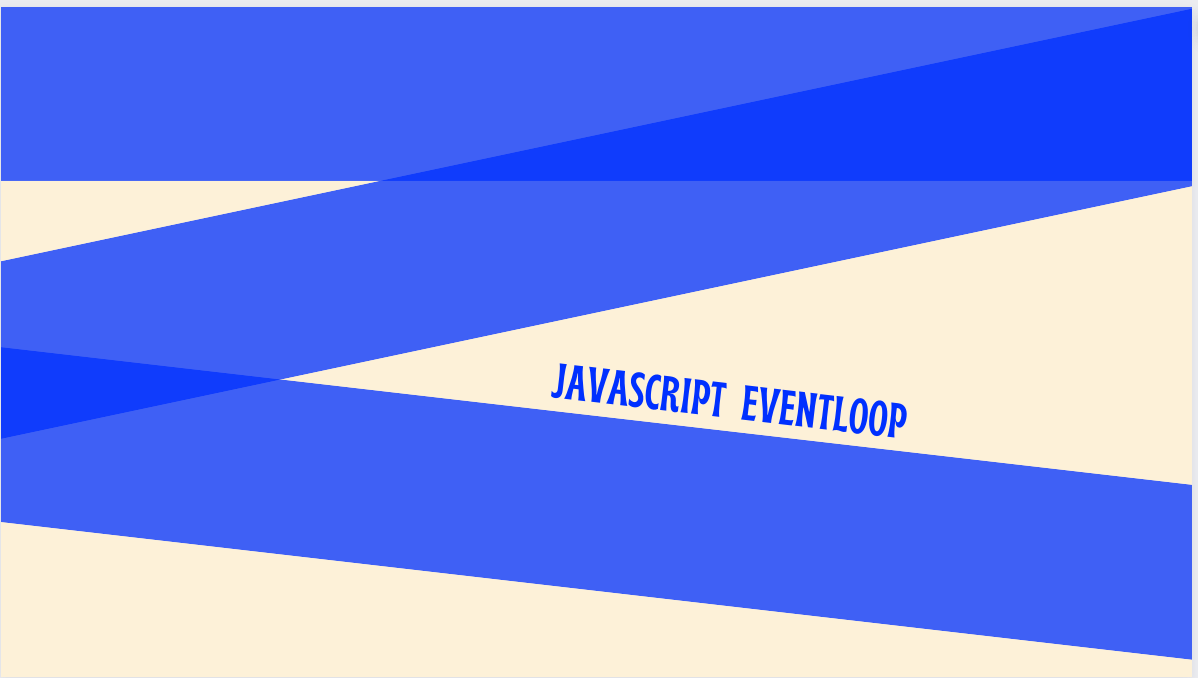
Welcome, techies! Now, let me ask you a question, and it is very important to answer this before continuing: Do you know what EventLoop or callBack queue is?
Don't know the answer? No worries at all! Because we are going to get it, but the approach to learning or understanding will be quite different. Here, I will ask you questions, and you have to honestly answer them because this article aims to give you confidence in it.
Let's enjoy...
Situation (Node.js)
function getT()
{
return (new Date()).getTime();
}
const start = getT();
console.log("start: ",getT()-start);
let t = setTimeout(()=>{
console.log("executed(setTimout): ",(getT() - start))
},500)
//time consuming task
while(getT() != start+1000){}
console.log("end ", getT() - start);
Output:
So, based on the above example, do you really think that JS is asynchronous? Because if it is, then the setTimeout should have executed much earlier than 1 second.
From the above, we can observe two things:
The setTimeout is never executed when it should be.
The setTimeout waits for the execution of the last line of code, and when it is finished, it outputs its response to the console.
Trust me, JS is not an asynchronous language. It just appears to be asynchronous with the help of the event loop and the callback queue, and you have the proof.
Okay, but how?
By ensuring that all tasks, whether they are within the control of JavaScript (loops, if-else statements, etc.) or not (features provided by the browser commonly known as Web APIs, like setTimeout, fetch, promises, etc.), should be completed, regardless of any delay (after finishing up the Global Execution Context), but before that, the Web API's tasks are considered pending tasks for the running Js.
So, where these pending task goes before the Js come to them?
These tasks are managed by a data structure called the "callback queue". Whenever JavaScript is executing a program and finds some features that are not controlled by JavaScript itself, it puts those tasks aside and focuses on other tasks. These tasks are then handled by the browser. When the browser receives a response for these tasks, it puts those responses in the callback queue. After JavaScript finishes all its tasks, including finishing the global execution context, the event loop checks for any pending responses in the callback queue and puts them in the call stack.
And this is what is happening to our code as well. When the JavaScript (JS) encounters the "setTimeout" function, it simply provides it to the Browser (in our case, it is Node.js). Then, the Browser ensures that the function reaches the callback queue after the specified period. Since the time taken for the completion of our code is more than the targeted time of setTimeout, it has to wait in the queue for almost half a second. When the Global Execution Context finishes, the event loop puts the setTimeout function in the call stack, and we get the output.
In browser?
Conclusion
So, the event loop seems to be the best work scheduler for JavaScript. It says, "Hey JavaScript! You just do your work that you can control, and leave the work that you can't control (web APIs) for me and the browser. When the task is completed, I will let you know about its output.”
Must check the second part!!!
Thanks for reading !!!
Subscribe to my newsletter
Read articles from Sahitya Aryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
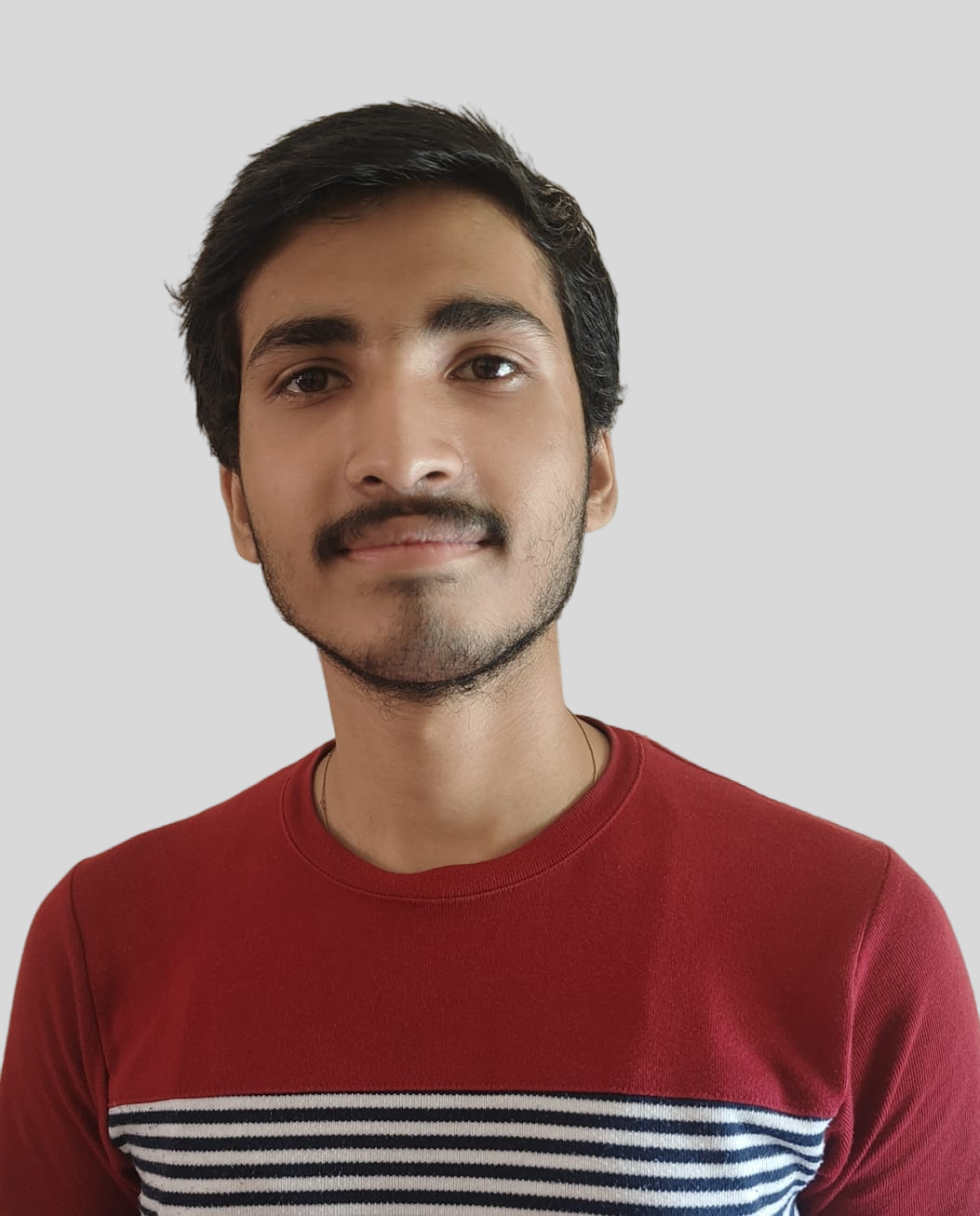
Sahitya Aryan
Sahitya Aryan
I am a full-stack developer, and open-source contributor, undergrad at the NIT Patna. Having hands-on experience in developing great and complex sites some of my projects include the Training and Placement Cell site of NIT Patna, the JalChakshu (a water source and distribution mapping app) Worked as an intern for the Trailshopy Marketplace Pvt Ltd. as a software developer