Django 101
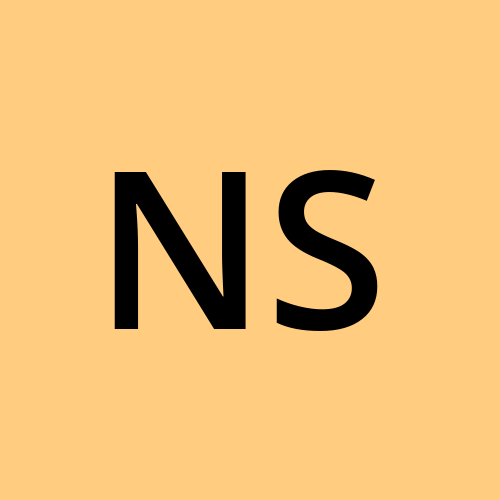
What is Django?
Purpose: Django is used for building web applications, emphasizing reusability, rapid development, and the principle of DRY (Don't Repeat Yourself).
Components: It includes an ORM (Object-Relational Mapping) layer, a template system, URL routing, forms handling, authentication, and more.
Key Features:
MTV Architecture: Django follows the Model-Template-View architectural pattern.
ORM (Object-Relational Mapping): Allows interacting with databases using Python objects, abstracting away SQL queries.
Admin Interface: Provides an auto-generated admin interface for managing site content.
Templates: Includes a powerful template system for rendering HTML.
Forms: Offers a forms library to simplify forms handling and data validation.
Authentication and Authorization: Built-in tools for user authentication, permissions, and sessions.
Security: Implements security features like protection against common web vulnerabilities (XSS, CSRF, SQL injection), password hashing, etc.
URL Routing: Uses a clean, elegant way to map URLs to views.
Middleware: Allows modifying request/response globally for applications.
Caching: Provides a caching framework for improving website performance.
Internationalization and Localization: Supports multi-language and multi-timezone applications.
Django Components:
Models: Define data models using Python classes that map to database tables.
Views: Handle user requests and process data before rendering them into templates.
Templates: HTML files with Django template language that defines the structure of the UI.
Forms: Create HTML forms and handle form data.
Admin Interface: Auto-generated admin interface for managing models and data.
URL Dispatcher: Maps URLs to views using regular expressions.
Middleware: Customizable hooks for modifying request/response globally.
Development Workflow:
Start a Project: Use
django-admin
ormanage.py
to start a new project.Create Apps: Divide functionality into smaller apps within a project.
Define Models: Define data models in Python classes using Django's ORM.
Write Views and Templates: Create views to handle user requests and templates for UI.
Configure URLs: Map URLs to views using URL patterns.
Run Migrations: Update the database schema based on model changes.
Testing: Django provides a testing framework for writing and running tests.
Deployment: Deploy Django applications to servers using various methods (e.g., WSGI, ASGI).
Community and Ecosystem:
Documentation: Comprehensive and well-structured official documentation.
Packages and Libraries: A rich ecosystem of third-party packages and libraries to extend Django's functionality.
Community Support: Active community providing forums, tutorials, and resources.
Versions:
Django Versions: Django evolves with new releases, maintaining backward compatibility and introducing new features.
Compatibility: Django 2.x, 3.x, and later versions are compatible with different Python versions.
Use Cases:
Content Management Systems (CMS): Building CMS platforms.
Web Applications: From small-scale to enterprise-level web applications.
API Development: Creating RESTful APIs using Django REST framework.
Conclusion:
Django is a robust, versatile, and popular web framework that simplifies web development in Python, offering a comprehensive toolkit and a well-defined development workflow. Its adherence to best practices, security features, and scalability make it a preferred choice for building web applications.
Subscribe to my newsletter
Read articles from Namya Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
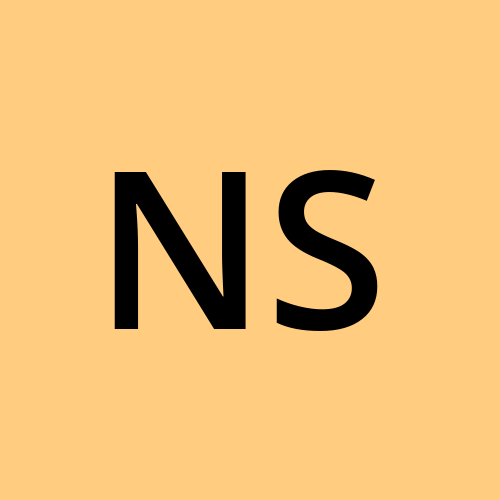
Namya Shah
Namya Shah
I am a developer who is very enthusiast about technology and coding.