Why Js DOM Manipulation?
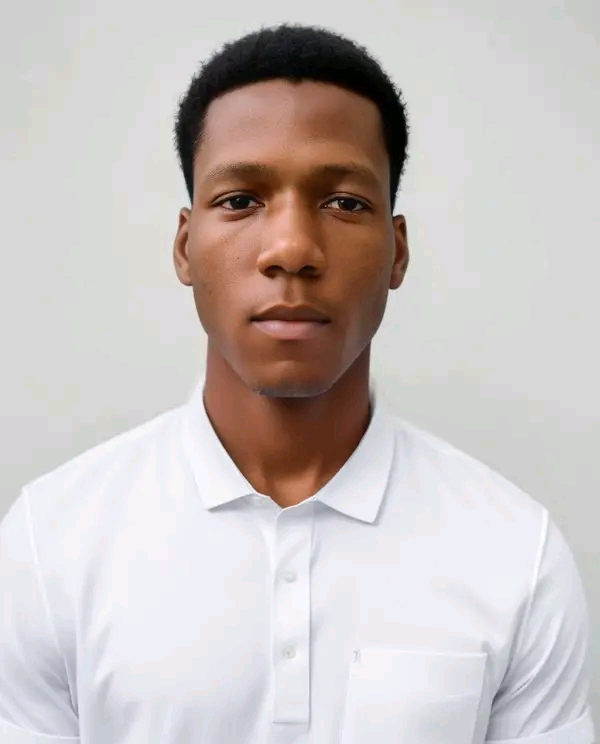
What Is The DOM?
Imagine a web page as a house with rooms and furniture. The DOM, is like a blueprint or map of that house.
It helps JavaScript understand and interact with the elements of a web page, such as text, images, buttons, and forms.
In the DOM, everything on a web page becomes an object. Each element, like a heading or paragraph, and even the attributes (like "class") becomes an object.
How Does The DOM Work?
The browser creates a tree-like structure in its memory, where each HTML element becomes a node.
Window
|
Document
|
HTML
/ \
Head Body
/ \ / | \
Title Meta H1-H6, img, P
Accessing Elements
JavaScript can talk to the DOM and ask for specific elements.
For example, it can say, "Hey DOM, can you give me the heading with the ID 'mainheading'?"
So, here is the most common method for accessing DOM elements.
• getElementByld
•getElementsByClassName
•getElementsByTagName
•querySelector
•querySelectorAll
•GetElementByld
This method allows you to select a single element by its unique id attribute. Since id values must be unique within a document.
This method always returns one element (or null if no element matches the provided id).
//HTML
<div id="myDiv">This is a div element.</div>
//JS
const myDiv = document.getElementById("myDiv");
console.log(myDiv.textContent);
// Output: "This is a div element.
•GetElementsByClassName
This method returns a collection of elements that have the specified class name.
If there are no elements with the given class, an empty collection will be returned.
//HTML
<p class="highlight">This is a highlight</p>
<p class="highlight">This is a highlight 2</p>
//JS
const elemClass = document.getElementsByClassName('highlight"); for (const element of elemClass) ( console.log(element.textContent);
//Output:
//"This is a highlight"
//"This is a highlight 2"
•GetElementsByTagName
This method returns a collection of elements with the specified tag name.
It's useful when you want to select all elements of a particular type, like <P>, <Div>, <h1>, etc.
// HTML
<h1>heading 1</h1>
<p>paragraph 1</p>
<p>paragraph 2</p>
//Js
const paragraphs = document.getElementsByTagName('p');
for (const paragraph of paragraphs) {
(console.log(paragraph.textContent);
}
/.Output:
//"Paragraph 1"
//"Paragraph 2"
•QuerySelector and QuerySelectorAll
querySelector is a method that selects the first element matching a CSS-like selector and returns it (or null if no match is found).
While querySelectorAll returns a NodeList with all elements that match the given selector, allowing for iteration using methods like forEach.
// HTML
<p class="highlight">Hello World</h1>
<ul><li class="highlight">Item 2</li>
<li class="highlight">Item 4</li></ul>
Const highlightedElement =document.querySelector(highlight'); console.log(highlightedElement.textContent);
// Output: "Hello, World!"
const highlightedItems =document.querySelectorAll('.highlight'); highlightedItems.forEach((item) { console.log(item.textContent);
// "Item 2"
// "Item 4"
Conclusion
DOM is a fantastic tool that allows JavaScript to understand, control, and modify web pages.
It enables developers to create dynamic and interactive websites that respond to user actions and provide a delightful experience.
As always, I hope you enjoyed the post and learned something new.
If you have any queries then let me know in the comment box.
Subscribe to my newsletter
Read articles from Nuel Chukwu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
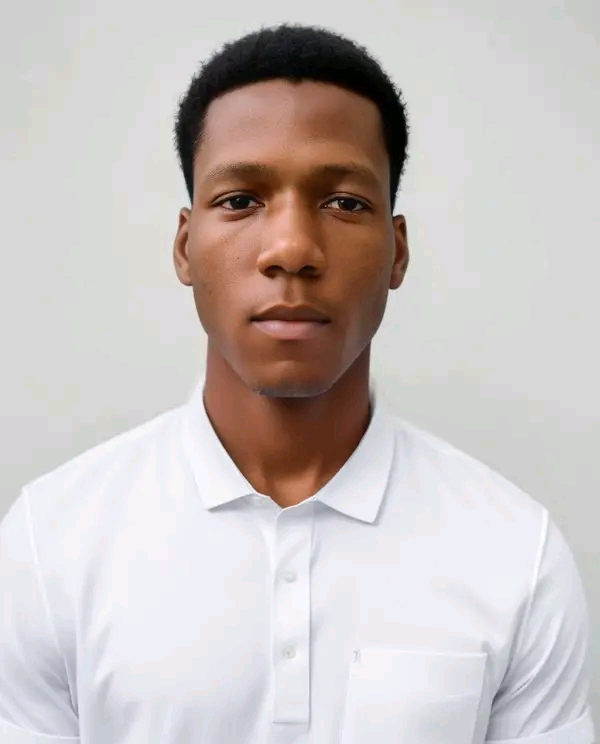
Nuel Chukwu
Nuel Chukwu
Nuel Chukwu is a dynamic Frontend Web Developer with a fervent dedication to crafting immersive user experiences and cutting-edge web solutions. Armed with an extensive skill set encompassing HTML, CSS, and JavaScript, Nuel is driven by a passion for blending creativity with functionality. His journey in web development has been marked by a commitment to staying at the forefront of design trends and technological advancements. Nuel's expertise extends beyond mere coding; he is adept at translating concepts into visually stunning and responsive interfaces. With a proven track record of successful project collaborations, Nuel thrives in a team environment, seamlessly integrating his technical prowess with creative insights. His commitment to continuous learning is evident in his ability to adapt to evolving industry standards, ensuring that every project he undertakes reflects the latest advancements in frontend development. Beyond the lines of code, Nuel Chukwu is characterized by meticulous attention to detail and a holistic approach to web design. His passion for clean, efficient code is matched only by his enthusiasm for creating digital solutions that captivate and engage users. Nuel is poised to contribute his multifaceted skills and innovative mindset to push the boundaries of frontend development in a fast-paced digital landscape.