Beginner's Guide to GraphQL: Setting Up Your Node.js Project with Ease

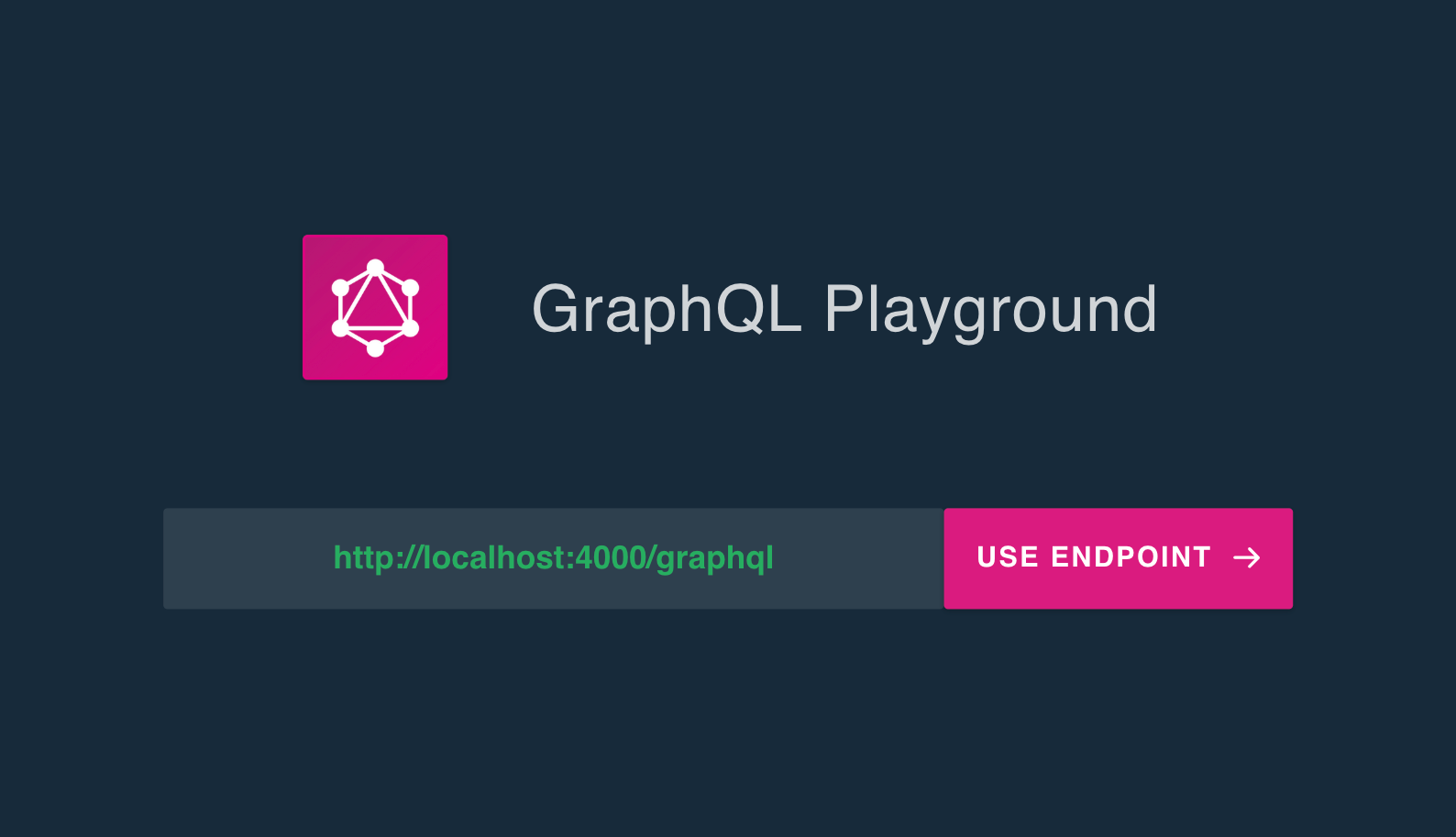
GraphQL has gained tremendous popularity in recent years for its flexibility and efficiency in handling data. In this guide, we will walk you through the basics of GraphQL, the advantages it offers, and the steps to set up a Node.js project using GraphQL.
What is GraphQL?
GraphQL is a query language for APIs that was developed by Facebook. Unlike traditional REST APIs, which expose fixed endpoints for various resources, GraphQL allows clients to request only the data they need. This results in more efficient and precise data retrieval, reducing the over-fetching or under-fetching of information.
Advantages of GraphQL:
1. Efficient Data Fetching:
GraphQL allows clients to specify the structure of the response, enabling them to get all the required data in a single request. This minimizes the need for multiple round-trips to the server.
2. Flexible Schema:
With GraphQL, the client defines the structure of the response. This means that as requirements change, clients can request additional or different data without requiring changes to the server.
3. Strongly Typed:
GraphQL APIs are strongly typed, meaning that the types of data that can be queried are explicitly defined. This ensures data consistency and provides clear documentation for the available operations.
Setting Up Your Node.js Project with GraphQL:
Now, let's dive into the practical aspect of setting up a Node.js project with GraphQL. We have two popular options for integrating GraphQL into a Node.js application: Express and Apollo Server.
In this series, we will be working with Apollo Server
Apollo Server is a community-driven, open-source GraphQL server that works with any GraphQL schema. Here's a basic setup:
Install Dependencies :
npm install @apollo/server graphql npm install --save-dev typescript @types/node
Create tsconfig.json :
npx tsc --init --rootDir src --outDir dist --lib dom,es6 --module commonjs --removeComments
Create an Apollo Server:
Create a 'src' directory with a 'server.ts' file
import { ApolloServer } from '@apollo/server'; import { startStandaloneServer } from '@apollo/server/standalone'; // A schema is a collection of type definitions (hence "typeDefs") // that together define the "shape" of queries that are executed against // your data. const typeDefs = `#graphql # Comments in GraphQL strings (such as this one) start with the hash (#) symbol. # This "Book" type defines the queryable fields for every book in our data source. type Book { title: String author: String } # The "Query" type is special: it lists all of the available queries that # clients can execute, along with the return type for each. In this # case, the "books" query returns an array of zero or more Books (defined above). type Query { books: [Book] } `; const books = [ { title: 'The Awakening', author: 'Kate Chopin', }, { title: 'City of Glass', author: 'Paul Auster', }, ]; // Resolvers define the technique for fetching the types defined in the // schema. This resolver retrieves books from the "books" array above. const resolvers = { Query: { books: () => books, }, }; // The ApolloServer constructor requires two parameters: your schema // definition and your set of resolvers. const server = new ApolloServer({ typeDefs, resolvers, }); startStandaloneServer(server, { listen: { port: 4000 } }) .then(({url}:any)=>{ console.log(`๐ Server listening at: ${url}`); });
Adjust the package.json scripts :
"scripts": { "start:dev": "npm run build:dev", "build:dev": "nodemon 'src/server.ts' --exec 'ts-node' src/server.ts -e ts,graphql" }
Run Your Server :
npm run start:dev
Final Output :
Congratulations! You've taken the first steps into the world of GraphQL. You now have a basic understanding of GraphQL, its advantages, and how to set up a Node.js project using either Express or Apollo Server. In the upcoming articles, we'll explore GraphQL schemas and resolvers and dive deeper into building robust APIs with this powerful technology.
You can view and fork the complete example on Github: Setup graphql server
Stay tuned for more exciting content on mastering GraphQL with Node.js!
Subscribe to my newsletter
Read articles from Gaurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Kumar
Gaurav Kumar
Hey! I'm Gaurav, a tech writer who's all about MERN, Next.js, and GraphQL. I love breaking down complex concepts into easy-to-understand articles. I've got a solid grip on MongoDB, Express.js, React, Node.js, and Next.js to supercharge app performance and user experiences. And let me tell you, GraphQL is a game-changer for data retrieval and manipulation. I've had the opportunity to share my knowledge through tutorials and best practices in top tech publications. My mission? Empowering developers and building a supportive community. Let's level up together with my insightful articles that'll help you create top-notch applications!