Arrays in Javascript: Its Methods and Properties in Depth
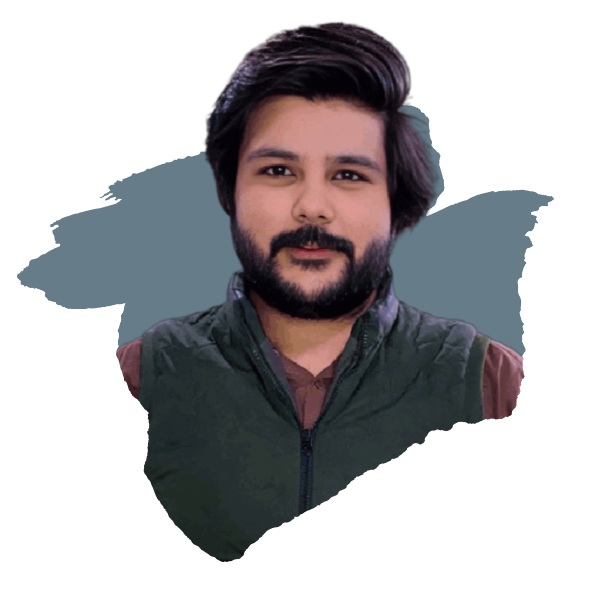
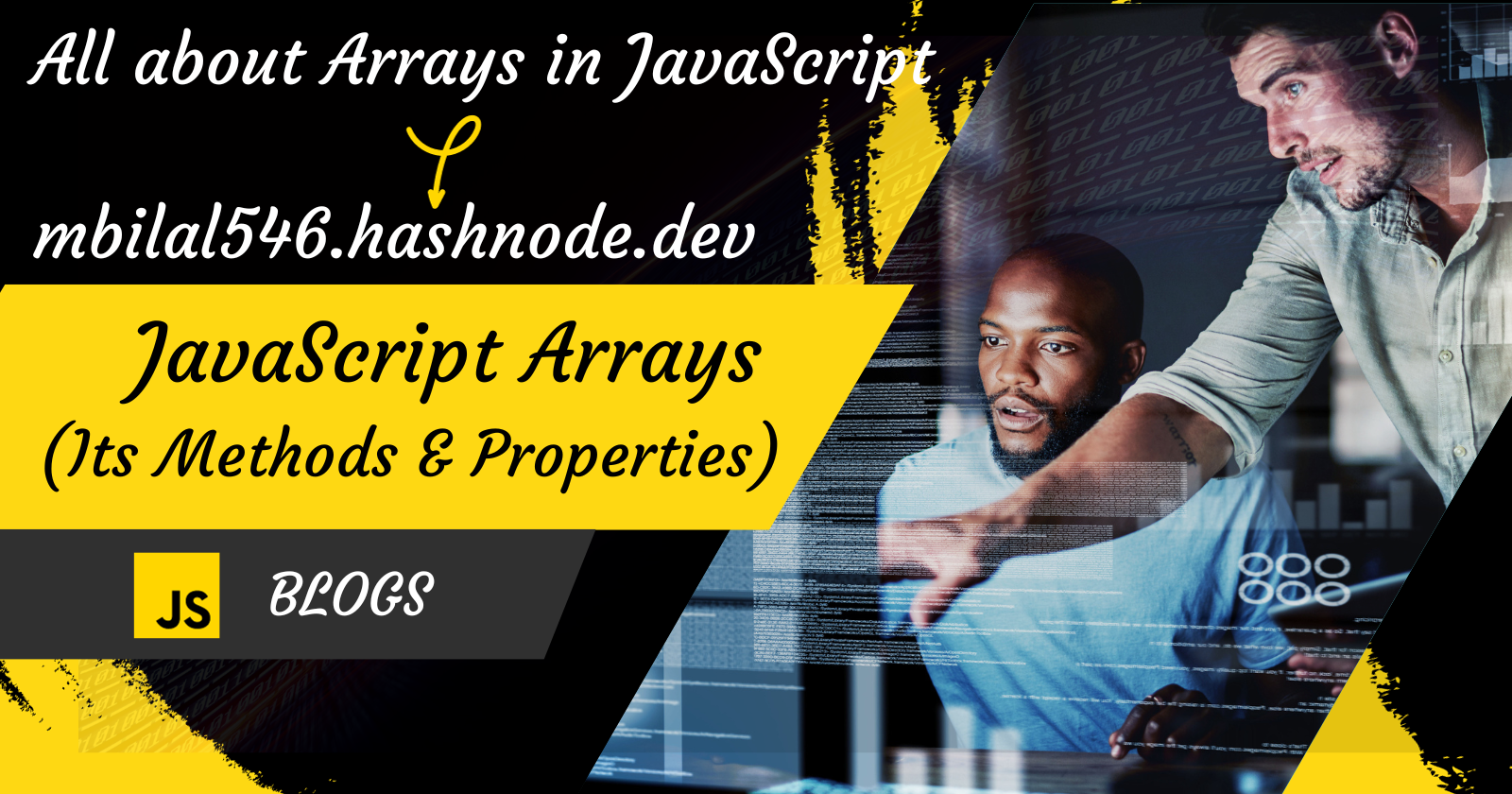
What is an array?
Arrays in JavaScript are a fundamental data structure that allows you to store and manipulate collections of data. They are objects in JavaScript, and the elements in an array can be of any data type (numbers, strings, objects, or even other arrays). You can check the type of the array using an typeof
operator.
For Example:
const myArr = [1, 2, "JavaScript Blogs", true];
console.log(myArr);
Output: [ 1, 2, 'JavaScript Blogs', true ]
// Checking the type of this array
console.log(typeof myArr);
Output: object
Arrays are zero-indexed (The elements can be accessed by using numerical indexes starting from 0).
For Example:
const myArr = [1, 2, "JavaScript Blogs", true];
console.log(myArr[0]);
Output: 1
console.log(myArr[2]);
Output: JavaScript Blogs
You can also create an array first and then insert elements into it through indexes.
For Example:
const myAarr = []; // Create an empty array.
// Now inserting elements into it.
myAarr[0] = "JavaScript Blogs";
myAarr[1] = 2;
myAarr[2] = false;
console.log(myAarr);
Output: [ 'JavaScript Blogs', 2, false ]
Multidimensional Array in JavaScript
In JavaScript, you can create multi-dimensional arrays using nested arrays. Unlike some programming languages, JavaScript does not have true multi-dimensional arrays; instead, you use arrays within arrays to achieve a similar effect.
For example:
// Creating a 2D array (matrix)
const twoDimen = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
// Accessing elements in a 2D array
console.log(twoDimen[0][0]);
Output: 1
console.log(twoDimen[1][2]);
Output: 6
// -------------------------------------------------------------------
// Creating a 3D array (cube)
const threeDimen = [
[
[1, 2, 3],
[4, 5, 6]
],
[
[7, 8, 9],
[10, 11, 12]
]
];
// Accessing elements in a 3D array
console.log(threeDimen[0][0][0]);
Output: 1
console.log(threeDimen[1][0][2]);
Output: 9
Modify and Remove array elements
Modify array elements
In JavaScript, you can modify array elements using their index.
For Example:
const myArr = [2024, "JavaScript Blogs", 1, 2, 4, 6]
myArr[1] = "Best Blog";
console.log(myArr);
Output: [ 2024, 'Best Blog', 1, 2, 4, 6 ]
Remove array elements
You can use the delete
keyword to remove an element from a JavaScript array, but it's important to note that it won't remove the element or change the array's length. Instead, it will replace the element with undefined
. This can lead to unexpected behavior, and it's generally not recommended to use delete
for removing array elements.
For Example:
const myArr = [2024, "JavaScript Blogs", 1, 2, 4, 6]
delete myArr[1];
console.log(myArr);
Output: [ 2024, <1 empty item>, 1, 2, 4, 6 ]
// empty item is undefined
Note:
Instead of usingdelete
, it's more common to use array methods like splice()
or filter()
to effectively remove elements from an array. We will explore these methods.
Array Properties and Methods
There are some properties and methods in JavaScript. Let's discuss them one by one.
1. array.push()
In JavaScript, the push()
method is used to add one or more elements to the end of an array. It modifies the original array and returns the new length of the array.
For Example:
const myArr = [1, 2, "JavaScript Blogs", true];
myArr.push("Best Blog");
console.log(myArr);
Output: [ 1, 2, 'JavaScript Blogs', true, 'Best Blog' ]
// You can add more than one values
const myArr = [1, 2, "JavaScript Blogs", true];
myArr.push("Best Blog", 2024);
console.log(myArr);
Output: [ 1, 2, 'JavaScript Blogs', true, 'Best Blog', 2024 ]
2. array.pop()
In JavaScript, the pop()
method is used to remove the last element from an array and return that removed element. This method changes the length of the array. If you try to pop()
method to an empty array, it returns undefined.
For Example:
const myArr = [1, 2, "JavaScript Blogs", true];
myArr.pop(); // removed true which is the last element.
console.log(myArr);
Output: [ 1, 2, 'JavaScript Blogs' ]
3. array.unshift()
In JavaScript, the array.unshift()
method is used to add one or more elements to the beginning of an array. It modifies the array in place and returns the new length of the array.
For Example:
const myArr = [1, 2, "JavaScript Blogs", true];
myArr.unshift("Muhammad Bilal");
console.log(myArr);
Output: [ 'Muhammad Bilal', 1, 2, 'JavaScript Blogs', true ]
Note:
Use unshift
is not a good practice. Because, as you can see when we add the value to the start of an array, we have to shift all the values forward and then add that element to the start.
4. array.shift()
In JavaScript, the array.shift()
method is used to remove the first element from an array and return that removed element. This method modifies the original array. If the array is empty, undefined
is returned.
For Example:
const myArr = [1, 2, "JavaScript Blogs", true];
myArr.shift(); // It remove 1 because it is first element.
console.log(myArr);
Output: [ 2, 'JavaScript Blogs', true ]
5. array.flat()
In JavaScript, the flat()
method is used to flatten an array by a specified depth. In short, it flattens a nested array into a single-level array. flat()
combines elements from subarrays into a single, continuous array.
For Example:
const myArr = [1, 2, ["JavaScript Blogs"], true, [1, 2]];
const flatArr = myArr.flat(); // Convert all subarrays into single array.
console.log(flatArr);
Output: [ 1, 2, 'JavaScript Blogs', true, 1, 2 ]
I said, flatten an array by a specified depth. Let's see it by an example
Let's suppose you have a nested array ( array into another array) with a depth of 4, as you can see in the example below.
const nestedArray = [1, 2, [3, 4, [5, 6, 7, [8, 9, 10]]]];
If you flatten this array by using array.flat()
method then.
const nestedArray = [1, 2, [3, 4, [5, 6, 7, [8, 9, 10]]]];
const flattenedArray = nestedArray.flat();
console.log(flattenedArray);
Output: [ 1, 2, 3, 4, [ 5, 6, 7, [ 8, 9, 10 ] ] ]
By default, it flattens the array to a depth of 1. If you want to flatten in more, then you have to give an argument.
For example, if you want to flatten to a depth of 2, then:
const nestedArray = [1, 2, [3, 4, [5, 6, 7, [8, 9, 10]]]];
const flattenedArray = nestedArray.flat(2);
console.log(flattenedArray);
Output: [ 1, 2, 3, 4, 5, 6, 7, [ 8, 9, 10 ] ]
If you need a fully flattened it, then it takes 3 as an argument.
const nestedArray = [1, 2, [3, 4, [5, 6, 7, [8, 9, 10]]]];
const flattenedArray = nestedArray.flat(3);
console.log(flattenedArray);
Output: [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 ]
Note:
It is introduced in ECMAScript 2019 (ES10). It is useful for functional programming paradigms and data manipulation.
Alternatives for older JavaScript versions include using concat()
or reduce()
methods, but flat()
offers a more concise and efficient approach.
6. array.toString()
In JavaScript, the toString()
method is used to convert an array to a string. This method is part of the Array
prototype, and when called on an array, it returns a string representation of the array elements, separated by commas.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2];
const strArr = myArr1.toString();
console.log(strArr);
Output: 2024,JavaScript Blogs,1,2
// To check its type use typeof
console.log(typeof strArr);
Output: string
7. array.join()
In JavaScript, the join()
method is used to join all elements of an array into a string. It takes an optional parameter, which is a string that specifies how to separate the array elements in the resulting string. If no separator is provided, the default is a comma (,
).
For Example:
const myArr1 = [2024, "JavaScript Blogs"];
const joinArr = myArr1.join(" and ");
console.log(joinArr);
Output: 2024 and JavaScript Blogs
// If you want to check its type
console.log(typeof joinArr);
Output: string
// If there is no parameter then
const myArr1 = [2024, "JavaScript Blogs"];
const joinArr = myArr1.join(); // By default seprated by comma (,)
console.log(joinArr);
Output: 2024,JavaScript Blogs
Note:
It's important to note that the original array is not modified by the join()
method; instead, it returns a new string representing the joined elements.
Keep in mind that if the array contains undefined
or null
values, they will be converted to empty strings in the resulting string.
For Example:
const myArr1 = [2024, null, "JavaScript Blogs", undefined, "Informative"];
const joinArr = myArr1.join();
console.log(joinArr);
Output: 2024,,JavaScript Blogs,,Informative
8. array.concat()
In JavaScript, the concat()
method is used to merge two or more arrays, creating a new array as a result. The original arrays are not modified by this operation.
For Example:
const myArr1 = [2024, "JavaScript Blogs"];
const myArr2 = [1, "JavaScript Info"];
const ConcatArr = myArr1.concat(myArr2);
console.log(ConcatArr);
Output: [ 2024, 'JavaScript Blogs', 1, 'JavaScript Info' ]
//You can concat more tan two arrays also
const myArr1 = [2024, "JavaScript Blogs"];
const myArr2 = [1, "JavaScript Info"];
const myArr3 = [1, 2, 3, 4];
const ConcatArr = myArr1.concat(myArr2, myArr3);
console.log(ConcatArr);
Output: [ 2024, 'JavaScript Blogs', 1, 'JavaScript Info', 1, 2, 3, 4 ]
9. array.slice()
In JavaScript, the array.slice()
method is used to extract a portion of an array and return a new array containing the extracted elements. The original array is not modified by this operation.
The slice()
method takes two arguments: start
and end
. The start
parameter specifies the index at which to begin extraction, and the end
parameter specifies the index at which to stop extraction (not included). If the end
parameter is missed, slice()
will extract elements from the start
index to the end of the array.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const sliceArr = myArr1.slice(2, 5);
console.log(sliceArr);
Output: [ 1, 2, 4 ]
// If there is no end parameter then it extract element from end of array.
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const sliceArr = myArr1.slice(2);
console.log(sliceArr);
Output: [ 1, 2, 4, 6 ]
10. array.splice()
The array.splice()
method in JavaScript modifies the contents of an existing array by removing and/or adding elements. It directly alters the original array, unlike methods like slice()
, that create new arrays.
The splice()
method takes two arguments: start
and end
. The start
parameter specifies the index at which to begin extraction, and the end
parameter specifies the index at which to stop extraction (included). If the end
parameter is missed, splice()
will extract elements from the start
index to the end of the array.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const spliceArr = myArr1.splice(1, 3);
console.log(spliceArr);
Output: [ 'JavaScript Blogs', 1, 2 ]
// If there is no end parameter then it extract element from end of array.
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const sliceArr = myArr1.slice(1);
console.log(sliceArr);
Output: [ 'JavaScript Blogs', 1, 2, 4, 6 ]
Main difference b/w slice and splice?
slice()
It is used to extract a portion of an array and return a new array. The original array is not modified by this operation.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const sliceArr = myArr1.slice(2);
console.log(sliceArr);
Output: [ 1, 2, 4, 6 ]
console.log(myArr1); // Printing Original Array
Output: [ 2024, 'JavaScript Blogs', 1, 2, 4, 6 ]
// this is as it is, Not modified.
splice()
It modifies the contents of an existing array by removing and/or adding elements. It directly alters the original array.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const spliceArr = myArr1.splice(1, 3);
console.log(spliceArr);
Output: [ 'JavaScript Blogs', 1, 2 ]
console.log(myArr1); // Printing Original Array
Output: [ 2024, 4, 6 ] // this is modified directly.
11. array.length
In JavaScript, the length
property of arrays returns or sets the number of elements in an array. It indicates the length or size of the array, which is the total number of elements currently present in the array.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
console.log(myArr1.length);
Output: 6
We can also set its length. For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
myArr1.length = 3
console.log(myArr1);
Output: [ 2024, 'JavaScript Blogs', 1 ]
If we try to expand its length, then it will print empty items or undefined items.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
myArr1.length = 8
console.log(myArr1);
Output: [ 2024, 'JavaScript Blogs', 1, 2, 4, 6, <2 empty items> ]
12. array.sort()
In JavaScript, the array.sort()
method is used to sort the elements of an array in place. The default sorting order is based on the Unicode code points of the elements.
For Example:
const myArr1 = ["Muhammad", "JavaScript Blogs", "Bilal"];
console.log(myArr1.sort()); // sort in alphabetical order.
Output: [ 'Bilal', 'JavaScript Blogs', 'Muhammad' ]
Note: Sorting Numbers
By default, thesort()
function sorts values as strings. This works well for strings ("Muhammad", "JavaScript Blogs", "Bilal"). However, if numbers are sorted as strings, "25" is bigger than "100" because "2" is bigger than "1". Because of this, the sort()
method will produce incorrect results when sorting numbers.
For Example:
const myArr2 = [2, 8, 4, 6, 10, 9, 7];
console.log(myArr2.sort()); // It sorted 10 through 1
Output: [ 10, 2, 4, 6, 7, 8, 9 ]
12. array.reverse()
In JavaScript, the reverse()
method is used to reverse the elements of an array. This method changes the order of the elements in the array in place, meaning it modifies the original array.
For Example:
const myArr1 = [2024, "JavaScript Blogs", 1, 2, 4, 6];
myArr1.reverse();
console.log(myArr1);
Output: [ 6, 4, 2, 1, 'JavaScript Blogs', 2024 ]
13. array.fill()
In JavaScript, the array.fill()
method is used to fill all the elements of an array with a specified value. It changes all elements of the array to the provided value, starting from a specified index (defaulting to 0) and ending at a specified index (defaulting to the array's length). This modified the original array.
For Example:
const myArr = [2024, "JavaScript Blogs", 1, 2, 4, 6];
myArr.fill(5); // It replace/fill all the elements with 5
console.log(myArr);
Output: [ 5, 5, 5, 5, 5, 5 ]
14. Array.isArray()
In JavaScript, the Array.isArray()
method is a built-in function that is used to determine whether a given value is an array. It was introduced in ECMAScript 5 (ES5) and is a reliable way to check if a variable is an array or not. It gives output in terms of a boolean ( true
or false
).
For Example:
const myArr = [2024, "JavaScript Blogs", 1, 2, 4, 6];
const checkArray = Array.isArray(myArr);
console.log(checkArray);
Output: true
const myStr = "JavaScript Blogs";
const checkArray1 = Array.isArray(myStr);
console.log(checkArray1);
Output: false
15. array.indexOf()
The indexOf()
method in JavaScript is used to find the index(position) of the first occurrence of a specified element within an array. If the element is not found, it returns -1.
For Example:
// first occurrence of a specified element
const myArr1 = ["JavaScript Blogs", "Muhammad", "Bilal", "Muhammad", "JS", "Blog"];
console.log(myArr1.indexOf("Muhammad"));
Output: 1
The indexOf()
takes an optional parameter, an index, at which to start the search. Defaults to 0 (beginning of the array).
For Example:
// Here we searched the same name with the starting index now its output change
const myArr1 = ["JavaScript Blogs", "Muhammad", "Bilal", "Muhammad", "JS", "Blog"];
console.log(myArr1.indexOf("Muhammad", 2));
Output: 3
16. array.IastIndexOf()
In JavaScript, the lastIndexOf()
method is used to find the last occurrence of a specified element within an array. It returns the index of the last occurrence of the specified element in the array, or -1 if the element is not found.
For Example:
const myArr1 = ["JavaScript Blogs", "Muhammad", "Bilal", "Muhammad", "JS", "Blog"];
console.log(myArr1.lastIndexOf("Muhammad"));
Output: 3
// If something is not present in it then
const myArr1 = ["JavaScript Blogs", "Muhammad", "Bilal", "Muhammad", "JS", "Blog"];
console.log(myArr1.lastIndexOf("All"));
Output: -1
17. array.includes()
The array.includes()
method in JavaScript is used to check if an array includes a certain element. It returns a boolean value (true
or false
) indicating whether the array contains the specified element. The method was introduced in ECMAScript 2016 (ES7) and is supported in modern browsers and Node.js environments.
For Example:
const myArr1 = ["JavaScript Blogs", "Bilal", "Muhammad", "JS", "Blog"];
console.log(myArr1.includes("JS"));
Output: true
const myArr1 = ["JavaScript Blogs", "Bilal", "Muhammad", "JS", "Blog"];
console.log(myArr1.includes("All"));
Output: false
18. array.some()
The array.some()
method in JavaScript is used to check whether at least one element in the array passes the test of the condition implemented by the provided function (callback function). It returns a Boolean value ( true
or false
) indicating whether the test passed for at least one element in the array.
For Example:
Example 1
const numbers = [1, 2, 3, 4, 5];
const hasEvenNumber = numbers.some(function (num) {
return num % 2 === 0;
});
console.log(hasEvenNumber);
Output: true
// (because there is at least one even number in the array)
In the above example, you directly pass the callback function into the some()
method. You can also pass the function's reference to the some()
after-making function separately.
Let's see with the same example:
Example 2
const numbers = [1, 2, 3, 4, 5];
const hasEvenNumber = numbers.some(checkEvenNum);
// Here in some() method you passes the reference of checkEvenNum function.
function checkEvenNum(number) {
return number % 2 === 0;
}
console.log(hasEvenNumber);
Output: true
// (because there is at least one even number in the array)
19. array.every()
The array.every()
method in JavaScript is used to test whether all elements in an array pass a certain condition implemented by a provided function. It returns a Boolean value ( true
or false
) indicating whether all the elements meet the specified condition.
For Example:
const ages = [22, 24, 16, 18];
const checkAdult = ages.every(function (num) {
return num >= 18;
});
console.log(checkAdult);
Output: false
// (because all ages in the array are not greater or equal than 18)
// Example 2 with all ages greater than 18
const ages = [22, 24, 26, 18];
const checkAdult = ages.every(function (num) {
return num >= 18;
});
console.log(checkAdult);
Output: true
// (because all ages in the array are greater or equal than 18)
20. array.find()
In JavaScript, the array.find()
method is used to return the first element in the array that satisfies a provided testing function. It stops searching the array as soon as it finds an element that meets the criteria specified in the callback function. If no such element is found, undefined
is returned.
For Example:
const ages = [2, 4, 6, 18, 22];
const checkNum = ages.find(function (num) {
return num >= 18;
});
console.log(checkNum); // It find 18 first and then stop searching
Output: 18
You can also do this with an implicit return. For Example:
const ages = [2, 4, 6, 18, 22];
const checkNum = ages.find( num => num >= 18 ); // implicit return
console.log(checkNum); // It find 18 first and then stop searching
Output: 18
21. array.findIndex()
ThefindIndex()
method in JavaScript is an array method introduced in ECMAScript 2015 (ES6). It is used to find the index of the first element in an array that satisfies a provided testing function. If the element is not found, it returns-1
.
For Example:
const ages = [2, 4, 6, 20, 24];
const checkNum = ages.findIndex(function (num) {
return num >= 18;
});
console.log(checkNum); // It find 20 first and then stop searching
Output: 3 // It find 20 first and 20 is on the index of 3
Higher-Order Array Methods
There are more higher-order array methods like map()
, filter()
, and reduce()
, which we will discuss in an upcoming blog.
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
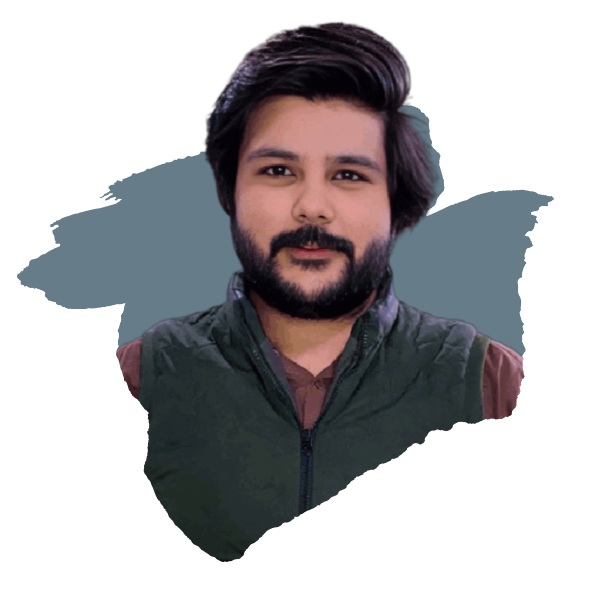
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. 📈 Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. 🔊 Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub 🖥 Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. ✨ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. 🎓 Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. 👥 Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.