Elevate Your App's Search Game: A Beginner's Guide to Supercharging Search Capabilities with Azure AI Search
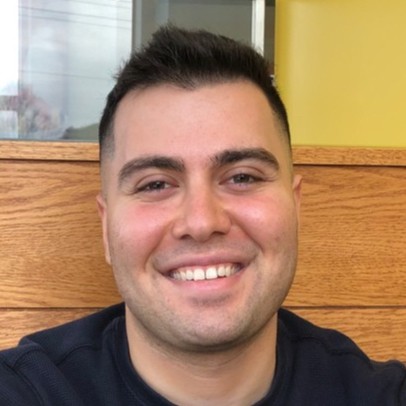
Table of contents
- Introduction to Azure AI Search
- Setting Up the Foundations
- Preparing for Coding
- Diving into the Code
- Running the Project and Viewing Results
- Navigating Challenges and Opportunities with Azure AI Search Indexes
- Essential Tips & Best Practices
- Troubleshooting & Additional Information
- Looking Ahead: Advanced Search Index Management
- Wrapping Up Our Journey with Azure AI Search
- Additional Resources and Further Reading
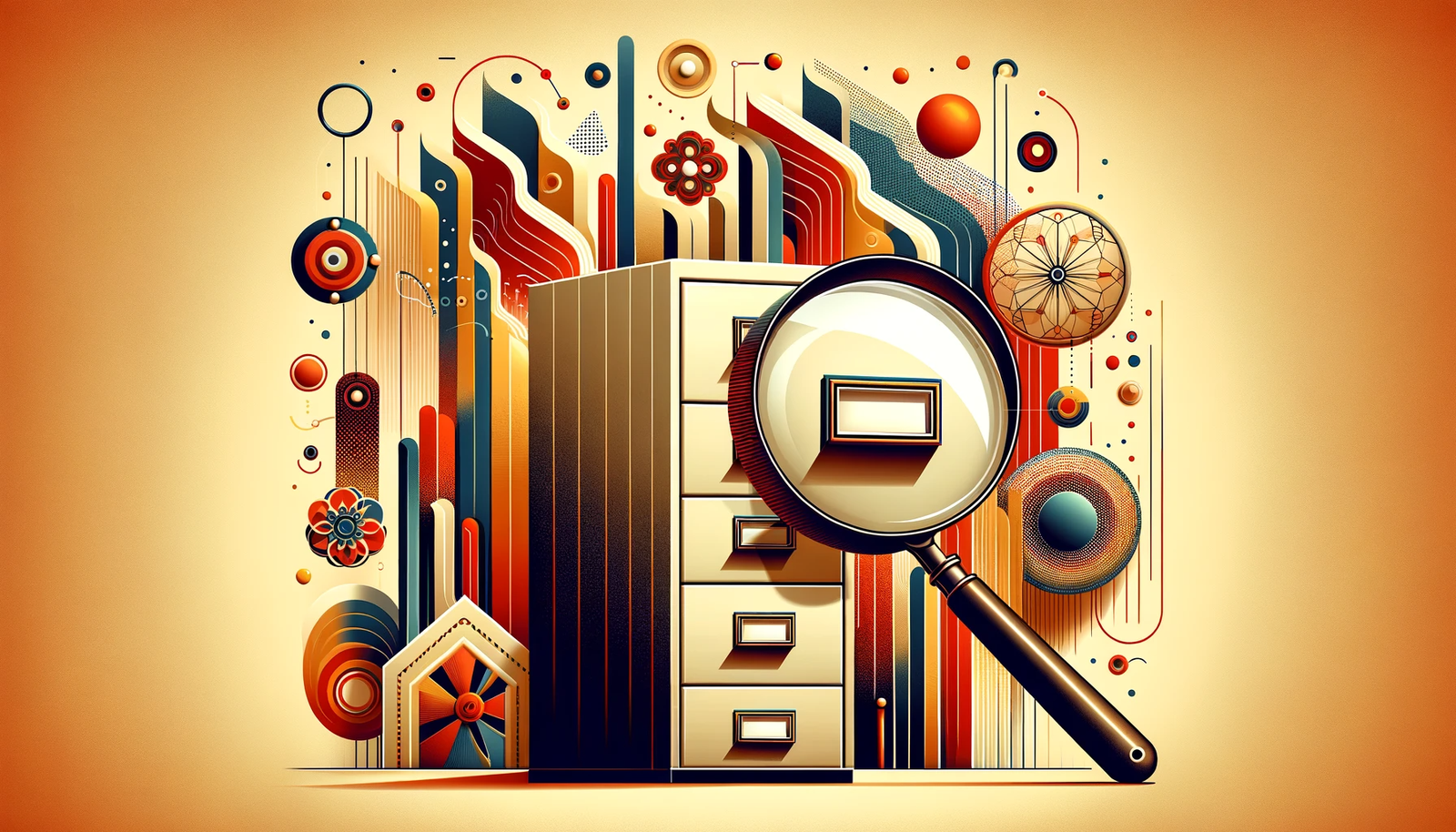
Introduction to Azure AI Search
Azure AI Search, formerly Azure Cognitive Search, is your versatile toolkit for data management. It's an AI-driven platform that optimizes data accessibility while easing database load. My first encounter with Azure AI Search was as a solution for database overload. Its capacity for handling and rapidly retrieving vast data sets, coupled with a user-friendly search process, makes it a standout choice.
But there's more to it than just easing database load. Azure AI Search acts like a digital librarian, swiftly and efficiently organizing and pulling up information. The AI-powered search not only improves accuracy but also enhances the user experience. Plus, it integrates smoothly with other Azure services, adding flexibility across various applications.
Azure AI Search revolutionizes the way we handle, search, and interact with large data sets. It has become an indispensable tool in application development, especially crucial in scenarios where enhanced search functionality plays a pivotal role.
Key Features
Efficient Data Handling: Acts as your digital librarian, swiftly organizing and retrieving large datasets.
AI-Enhanced Search Precision: Boosts search accuracy for an improved user experience.
Seamless Azure Integration: Ideal for integration within the Azure ecosystem.
Advanced Data Querying: Features like searchable, filterable, sortable, and facetable fields enable sophisticated data queries.
Database Relief: It significantly lightens the load on your databases, particularly in search-heavy applications, by efficiently managing complex queries that would traditionally tax your database resources.
Versatile Search Capabilities: Excels in text and vector similarity searches, ideal for pattern analysis in large datasets.
Complex Data Type Modeling: Handles intricate data structures with ease. For instance, it can manage complex objects like a hotel's 'Room' attributes, where each room is characterized by its description, room number, and rate.
Content Transformation: Converts text or image files into searchable content, ideal for assets stored in Azure Blob Storage or Azure Cosmos DB.
Customizable Text Analysis: Supports various linguistic tools and customizes text analysis for multi-language and format content.
Now that we've covered the features, let's delve into understanding a Search Index in Azure AI Search.
Understanding a Search Index
A Search Index is like the index of a book but for digital data. It lists topics and keywords, guiding you to the information you need. This specialized database is tailored for large data sets, organizing data for efficient searching and retrieval. It's the go-to tool for finding that 'needle in the digital haystack'.
Azure AI Search Enhanced Features and Application Scenarios
Azure AI Search elevates search functionality with features for full-text searches (searchable fields), refining results (filterable fields), organizing results (sortable fields), and aiding in search refinement (facetable fields). It excels in various application scenarios, including full-text and vector similarity searches, making it suitable for generative AI applications. Additionally, it streamlines the implementation of search features like relevance tuning and faceted navigation and transforms extensive files into searchable formats. It also accommodates diverse linguistic and custom text analyses, ensuring versatility in content processing.
With an understanding of Azure AI Search's capabilities, it's time to turn theory into action by building our own Search Index.
What's the Plan?
I am a firm believer in learning by doing. We're diving into the process of building a Search Index in C#. I'll take you through each step, breaking down the code along the way. After we explore each part, we'll run the project and check out what we've accomplished. Here’s what we’ll be doing:
Creating the Azure Data Source (Via Azure Portal): We'll start by creating the data source for our Search Index, using Azure Table Storage.
Creating the Azure AI Search Service (Via Azure Portal): Next, we establish the Azure Search Service, the home for our Search Index and its components.
Cloning the Repository & Project Preparation (Optional): For hands-on experience, clone the repository and align your appsettings.json with your Azure setup.
Populating the Data Source (Via Code): With Azure Table Storage ready, we'll populate it with 'Books' table data, perfect for filtering, sorting, and searching.
Building the Search Index Infrastructure (Via Code): We then shift our focus to creating a Search Index that aligns with our 'Books' table's fields.
Populating the Search Index (Via Code): Now, we're at the data loading phase. Here, we'll set up an Indexer within the Azure Search Service. Think of the Indexer as a bridge: it's provided by Azure free of charge, and it connects two key points. On one end, we have our Data Source Connection, our source, where we've got our 'Books' table data. On the other end, there's our Search Index, the target, ready to receive and organize this data. Once we link the Indexer to our data source, it'll funnel data into the Search Index, transforming raw data into a structured, searchable format.
Querying Data from the Search Index (Via Code): Finally, with our Search Index fully stocked, we'll run various queries to explore Azure AI's querying capabilities, giving us a practical understanding of its potential.
I'm Assuming
You Have an Azure Subscription: To create resources, an Azure Subscription is required. If you don't have one yet, signing up is straightforward, and you'll immediately receive $200 in credits, which is more than enough for this project.
A Prepared Resource Group: This is where we'll host our resources. If you’re not familiar with setting up a resource group in Azure, you can follow the steps outlined here.
Basic Programming Knowledge (Optional): It's helpful to know some programming basics, especially how to use languages with external libraries. We'll be using C# and SDKs here. If not, don't worry, I'll guide you through everything.
.NET IDE Ready to Go (Optional): Ideal to have Visual Studio or Rider installed.
Note: If coding isn't your thing or you prefer to learn passively, you can skip the optional steps and still grasp the key concepts.
Setting Up the Foundations
Azure Data Source Creation
We begin by establishing a data source for our Azure Search Index. Azure supports a variety of them, including Azure Blob Storage, Cosmos DB, Data Lake Storage Gen2, and several others, with some like Azure Files and Azure MySQL currently in preview. You can dive deeper into these options here.
For simplicity, we're going with Azure Table Storage. It's straightforward to set up. Just head to your Resource Group, click '+ Create', and find 'Storage Account' in the Azure Marketplace.
Choose the same Region as your Resource Group and opt for 'Locally-redundant storage' under Redundancy to minimize costs. The rest? Just leave it as is and hit 'Create'.
Feel free to explore the official documentation for more details.
Azure AI Search Service Creation
Next, we set up the Azure AI Search Service. In your Resource Group, select '+ Create' and search for 'Azure AI Search' in the Azure Marketplace.
Again select the same Region as your Resource Group, but this time, pick the 'Free' Pricing Tier. Leave the other settings default and proceed with 'Review + Create'. This setup won't cost you a dime.
Feel free to explore the official documentation for more details.
Preparing for Coding
Cloning the Repository & Project Preparation (Optional)
For those who prefer hands-on coding, clone the azure-search-index-builder repository. Make sure to align the settings in the appsettings.json file with your newly created Storage Account and Search Service.
Here's how the appsettings.json file should look based on my previous steps:
{
"AzureStorageAccount": {
"Name": "mystorageaccount9999", // Your Azure Storage Account name - Can be found on the very top of the Storage Account overview
"Key": "xxxxxxx" // Your Azure Storage Account key - Can be found in the Storage Account settings, under "Access keys"
},
"AzureSearchService": {
"Url": "https://my-searchservice9999.search.windows.net/", // Your Azure Search Service URL, in the format of https://<service name>.search.windows.net
"AdminApiKey": "xxxxxxx" // Your Azure Search Service Admin API key, can be found in the Azure Search Service settings, under "Keys"
}
}
If you're more inclined to learn passively, feel free to skip this step.
Diving into the Code
Populating the Data Source
Now, we dive into the code. In the Program.cs file, you'll find the CreateAndPopulateBooksTable()
method where the Data Source preparation happens. We set up and fill the 'Books' table in Azure Table Storage, using data from the books.json file.
Here’s a quick look at what we're doing:
string CreateAndPopulateBooksTable(string azureStorageAccountName, string azureStorageAccountKey)
{
// Create Books table in Azure Storage
const string books = "Books";
var tableServiceManager = new TableServiceManager(azureStorageAccountName, azureStorageAccountKey);
tableServiceManager.CreateTable(books);
// Insert data from JSON file into Books table
tableServiceManager.InsertRecordsIntoTable(books);
return books;
}
We kick things off by creating an instance of the TableServiceManager
class, which sets up our connection to Azure Table Storage. This connection is crucial as it's our gateway to interacting with the storage service.
Here's how the Table Service Client looks like:
private readonly TableServiceClient _tableServiceClient = new
(
new Uri($"https://{storageAccountName}.table.core.windows.net/"),
new TableSharedKeyCredential
(
storageAccountName,
storageAccountKey
)
);
We then call CreateTable()
to create our 'Books' table, followed by InsertRecordsIntoTable()
to populate it.
public void CreateTable(string tableName)
{
_tableServiceClient.CreateTableIfNotExists(tableName);
}
The InsertRecordsIntoTable
method is where we read the books.json file, convert its contents into Book
objects, and then insert them into our table.
Here's the InsertRecordsIntoTable
method:
public void InsertRecordsIntoTable(string tableName)
{
var tableClient = _tableServiceClient.GetTableClient(tableName);
var jsonFilePath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "Assets", "books.json");
var jsonFileContent = File.ReadAllText(jsonFilePath);
var books =
JsonConvert.DeserializeObject<List<Book>>(jsonFileContent)
??
throw new Exception("Unable to deserialize books. Check JSON file and make sure it was included in the build.");
foreach (var book in books)
{
book.PartitionKey = book.Genre;
book.RowKey = book.Id;
tableClient.AddEntity(book);
}
}
The Book
class is straightforward. It inherits from ITableEntity
, a requirement for any entity you want to store in Azure Table Storage. Here's what our Book
class looks like:
public class Book : ITableEntity
{
[JsonIgnore] // Ignored by Index & Indexer.
public string PartitionKey { get; set; } = null!;
[JsonIgnore] // Ignored by Index & Indexer.
public string RowKey { get; set; } = null!;
[JsonIgnore] // Ignored by Index & Indexer.
public DateTimeOffset? Timestamp { get; set; }
[JsonIgnore] // Ignored by Index & Indexer.
public ETag ETag { get; set; }
[SimpleField(IsKey = true)]
public string Id { get; set; } = null!;
[SearchableField(AnalyzerName = LexicalAnalyzerName.Values.EnLucene)]
public string Title { get; set; } = null!;
[SearchableField(IsFilterable = true)]
public string Author { get; set; } = null!;
[SimpleField(IsFilterable = true)]
public string Genre { get; set; } = null!;
[SimpleField(IsFilterable = true, IsSortable = true)]
public int PublishedYear { get; set; }
[SimpleField(IsSortable = true, IsFilterable = true)]
public double Price { get; set; }
public override string ToString()
{
return $"Id: {Id}, Title: {Title}, Author: {Author}, Genre: {Genre}, PublishedYear: {PublishedYear}, Price: {Price}";
}
}
And that's the walkthrough of the setup! By following these steps in the project, you will have created a table in Azure Table Storage and populated it with data, setting the stage for indexing once you run the project.
Once you've executed the code, you can verify the results in your Storage Account. Simply navigate to the 'Books' table by going to your Storage Account > Storage Browser > Table > Books. There, you should find 30 Book records, showcasing the successful data population.
Build the Search Index Infrastructure
We then focus on constructing the Search Index infrastructure. The trickiest part? Defining the Index fields.
Index Field Preparation
Imagine a Search Index as similar to a database table, where fields in the index parallel the columns in a database. Just like defining column types in a database, we define field types in a Search Index. However, there's an additional layer in Search Indexes as we also need to specify the query attributes for each field. This distinction is what sets the Search Index fields apart and tailors them for efficient searching.
Now, let's circle back to our Book
class. The fields in this class come with specific annotations, demonstrating how Azure AI Search interprets and utilizes each field. Here’s a quick glance at some of these annotations:
JsonIgnore: This annotation tells Azure to exclude specific fields during index creation. For instance, RowKey
is not necessary for our querying purposes and can be overlooked.
[JsonIgnore] // Ignored by Index & Indexer.
public string RowKey { get; set; } = null!;
SimpleField: Used for fields that we want to retrieve but not necessarily search through. The Id
field is a perfect example; it's retrievable but not intended for searching.
[SimpleField(IsKey = true)]
public string Id { get; set; } = null!;
SearchableField: Assigned to fields that should be both retrievable and searchable. Consider the Author
field, which is also marked as filterable.
[SearchableField(IsFilterable = true)]
public string Author { get; set; } = null!;
These examples illustrate just a few of the annotations and field setups used in Search Indexes. For a comprehensive understanding of how to set field attributes and to explore all the options available, I recommend diving into the documentation on Field definitions and Field attributes.
Index Construction
In Program.cs, we use the CreateAndPopulateBooksSearchIndex
method to build our Search Index and its components. The SearchServiceManager
class sets up the connection to our Azure Cognitive AI Search service. In this section, we focus on the Index creation, happening in CreateIndex
method, called from Program.cs on line 62.
The process:
Initialize the Search Index Client.
Build the index fields using FieldBuilder.
Define the index name and fields.
Either create a new index or update an existing one using CreateOrUpdateIndex.
public SearchIndex CreateIndex()
{
var searchIndexClient = new SearchIndexClient(_searchServiceAuthentication.Uri, _searchServiceAuthentication.Credentials);
// Define & build the index fields
var fieldBuilder = new FieldBuilder();
var searchFields = fieldBuilder.Build(typeof(Book));
// Set the index name and fields
var searchIndex = new SearchIndex("my-index", searchFields);
searchIndexClient.CreateOrUpdateIndex(searchIndex);
return searchIndex;
}
Populate the Search Index
Next up: transferring data from our Azure Storage Book table to the Search Index.
We start by setting up a Data Source Connection for our Indexer. This connection links our data source (the Azure Storage Book table) to the Indexer. The setup happens in Program.cs, line 65 when we run CreateIndexerDataSource
.
The process involves:
Creating a Search Indexer Client.
Building the Data Source Connection.
Either creating a new Data Source Connection or updating the existing one using CreateOrUpdateDataSourceConnection.
public SearchIndexerClient CreateIndexerDataSource
(
string storageAccountName,
string storageAccountKey,
string tableName,
out SearchIndexerDataSourceConnection searchIndexerDataSourceConnection
)
{
var searchIndexerClient = new SearchIndexerClient(_searchServiceAuthentication.Uri, _searchServiceAuthentication.Credentials);
searchIndexerDataSourceConnection = new SearchIndexerDataSourceConnection
(
"my-index-data-source",
SearchIndexerDataSourceType.AzureTable,
$"DefaultEndpointsProtocol=https;AccountName={storageAccountName};AccountKey={storageAccountKey};EndpointSuffix=core.windows.net",
new SearchIndexerDataContainer(tableName)
);
searchIndexerClient.CreateOrUpdateDataSourceConnection(searchIndexerDataSourceConnection);
return searchIndexerClient;
}
After establishing the Data Source Connection, we create the Indexer by calling the CreateIndexer
method from Program.cs, line 68. This sets up where the data is pulled from and where it's pushed to through the SearchIndexer class instantiation.
public static SearchIndexer CreateIndexer(SearchIndexerDataSourceConnection dataSourceConnection, SearchIndex searchIndex, SearchIndexerClient searchIndexerClient)
{
var searchIndexer = new SearchIndexer("my-index-indexer", dataSourceConnection.Name, searchIndex.Name);
searchIndexerClient.CreateOrUpdateIndexer(searchIndexer);
return searchIndexer;
}
Finally, to start the data transfer, we run the PopulateIndex
method in Program.cs, line 71, which resets and then runs the Indexer.
public static void PopulateIndex(SearchIndexerClient searchIndexerClient, SearchIndexer searchIndexer)
{
searchIndexerClient.ResetIndexer(searchIndexer.Name);
searchIndexerClient.RunIndexer(searchIndexer.Name);
}
This function does two key things:
Resets the change tracking state of indexed documents.
Runs the Indexer to begin transferring data into our Search Index.
The Indexer may take around 30 seconds to finish transferring data to the Index. To view results in your Cognitive AI Search service navigate to Indexes > my-index and hit Search.
Querying Data From the Search Index
Before we run the code, let's take a look at some queries that highlight the capabilities of the Search Index. I've set up four distinct queries for us to execute. They're called from Program.cs (lines 85-95), each designed to demonstrate the versatility of our Search Index.
void RunQueriesOnSearchIndexAndPrintResults(string azureSearchServiceEndpoint, string azureSearchServiceAdminApiKey, SearchIndex azureSearchIndex)
{
var searchIndexDataRetriever = new SearchIndexDataRetriever
(
azureSearchServiceEndpoint,
azureSearchServiceAdminApiKey,
azureSearchIndex
);
var queryOneResults = searchIndexDataRetriever.SearchBooksForGeorgeOrwell();
queryOneResults.PrintValue();
var queryTwoResults = searchIndexDataRetriever.FilterBooksCheaperThanTwentyFiveDollarsInDescendingOrder();
queryTwoResults.PrintValue();
var queryThreeResults = searchIndexDataRetriever.SortBooksByPublishedYearInAscendingOrderAndGrabTopFive();
queryThreeResults.PrintValue();
var queryFourResults = searchIndexDataRetriever.SearchBooksForTitleMatchingMockingBird();
queryFourResults.PrintValue();
}
Let's break down these queries:
Query 1 - Searching for 'George Orwell': This query rummages through all fields for any mention of 'George Orwell'.
public SearchResults<Book> SearchBooksForGeorgeOrwell()
{
Console.WriteLine("Query 1: Search for 'George Orwell':");
var options = new SearchOptions();
GrabAllBookFieldsFromTheIndex(options);
var results = _searchClient.Search<Book>("George Orwell", options);
return results;
}
Query 2 - Filtering Books Under $25: Here, we filter for books priced below $25 and sort them by price in descending order.
public SearchResults<Book> FilterBooksCheaperThanTwentyFiveDollarsInDescendingOrder()
{
Console.WriteLine("Query 2: Apply a filter to find books cheaper than $25, order by Price in descending order:");
var options = new SearchOptions
{
Filter = "Price lt 25",
OrderBy = { "Price desc" }
};
GrabAllBookFieldsFromTheIndex(options);
var results = _searchClient.Search<Book>("*", options);
return results;
}
Query 3 - Sorting by Published Year, Top 5: This query sorts books by their publication year in ascending order, grabbing the first five.
public SearchResults<Book> SortBooksByPublishedYearInAscendingOrderAndGrabTopFive()
{
Console.WriteLine("Query 3: Search all the books, order by published year in ascending order, take the top 5 results:");
var options = new SearchOptions
{
Size = 5,
OrderBy = { "PublishedYear asc" }
};
GrabAllBookFieldsFromTheIndex(options);
var results = _searchClient.Search<Book>("*", options);
return results;
}
Query 4 - Title Search for 'Mockingbird': We focus on the 'Title' field to find books with 'Mockingbird' in their title.
public SearchResults<Book> SearchBooksForTitleMatchingMockingBird()
{
Console.WriteLine("Query 4: Search the Title field for the term 'Mockingbird':");
var options = new SearchOptions
{
SearchFields = { "Title" }
};
GrabAllBookFieldsFromTheIndex(options);
var results = _searchClient.Search<Book>("Mockingbird", options);
return results;
}
Running the Project and Viewing Results
Executing the Project
After configuring the variables in your appsettings.json file, simply run the project. That's all there is to it. Should you encounter any issues, refer to the Troubleshooting & Additional Information section for guidance.
Observing the Query Outputs
After running the project, If everything has gone smoothly, we should now see the following results from our queries:
Query 1: Finds '1984' by George Orwell.
Id: 2, Title: 1984, Author: George Orwell, Genre: Dystopian, PublishedYear: 1949, Price: 19.99
Query 2: Lists books under $25, like 'The Great Gatsby' by F. Scott Fitzgerald and 'Moby Dick' by Herman Melville, sorted by price.
Id: 1, Title: The Great Gatsby, Author: F. Scott Fitzgerald, Genre: Fiction, PublishedYear: 1925, Price: 24.99
Id: 9, Title: Book Title 9, Author: Author 9, Genre: Other Genre, PublishedYear: 1959, Price: 24.99
Id: 8, Title: Moby Dick, Author: Herman Melville, Genre: Adventure, PublishedYear: 1851, Price: 22.99
Id: 5, Title: Brave New World, Author: Aldous Huxley, Genre: Dystopian, PublishedYear: 1932, Price: 22.99
Id: 6, Title: The Hobbit, Author: J.R.R. Tolkien, Genre: Fantasy, PublishedYear: 1937, Price: 21.99
Id: 4, Title: The Catcher in the Rye, Author: J.D. Salinger, Genre: Fiction, PublishedYear: 1951, Price: 20.99
Id: 2, Title: 1984, Author: George Orwell, Genre: Dystopian, PublishedYear: 1949, Price: 19.99
Id: 3, Title: To Kill a Mockingbird, Author: Harper Lee, Genre: Fiction, PublishedYear: 1960, Price: 18.99
Id: 7, Title: Pride and Prejudice, Author: Jane Austen, Genre: Romance, PublishedYear: 1813, Price: 18.99
Query 3: Brings up the top 5 books sorted by year, starting with the oldest, like 'Pride and Prejudice' by Jane Austen.
Id: 7, Title: Pride and Prejudice, Author: Jane Austen, Genre: Romance, PublishedYear: 1813, Price: 18.99
Id: 8, Title: Moby Dick, Author: Herman Melville, Genre: Adventure, PublishedYear: 1851, Price: 22.99
Id: 1, Title: The Great Gatsby, Author: F. Scott Fitzgerald, Genre: Fiction, PublishedYear: 1925, Price: 24.99
Id: 5, Title: Brave New World, Author: Aldous Huxley, Genre: Dystopian, PublishedYear: 1932, Price: 22.99
Id: 6, Title: The Hobbit, Author: J.R.R. Tolkien, Genre: Fantasy, PublishedYear: 1937, Price: 21.99
Query 4: Successfully locates 'To Kill a Mockingbird' by Harper Lee.
Id: 3, Title: To Kill a Mockingbird, Author: Harper Lee, Genre: Fiction, PublishedYear: 1960, Price: 18.99
Query Outputs Explained
Alright, let's break down what's happening in our query method calls. It's simpler than it looks, I promise!
We're basically doing two things in each query:
Setting Search Options: We tailor these options to meet our specific query needs. This is where we decide how we want to search, filter, sort or in other ways, find the data we need.
Selecting Fields to Receive: We select which Search Index fields we want to receive in our results.
Each query is crafted to showcase a different aspect of Azure Search's data retrieval prowess. Let me walk you through a couple of examples:
Filtering by Price, Sorted in Descending Order
Consider this query:
var options = new SearchOptions
{
Filter = "Price lt 25",
OrderBy = { "Price desc" }
};
var results = _searchClient.Search<Book>("*", options);
Here, we're implementing a filter to identify books priced below (less than, denoted as 'lt') $25, and we're sorting these results in descending (abbreviated as 'desc') order by price. The asterisk '*', used as a wildcard, signifies a broad search without pinpointing a specific term. This approach depends on the 'Price' field being set as both filterable and sortable in our Book class:
[SimpleField(IsSortable = true, IsFilterable = true)]
public double Price { get; set; }
Searching by Title for 'Mockingbird'
Now, let's look at a title-specific search:
var options = new SearchOptions
{
SearchFields = { "Title" }
};
var results = _searchClient.Search<Book>("Mockingbird", options);
In this query, we're zeroing in on the 'Title' field to find matches for 'Mockingbird'. This approach narrows our search scope to titles, seeking the best match for the given term.
If you're still feeling a bit fuzzy on these concepts, I recommend running the code yourself and tweaking the queries. It's a hands-on way to see how flexible and powerful Azure Search can be. For further reading and more examples, check out the official Azure Search documentation.
By following these steps, you're well-equipped to embark on your own Proof of Concept (POC) projects. The best way to learn is by doing, so dive in and experiment! And hey, once you've mastered it, feel free to flaunt 'Expert Azure Search Index Developer' on your resume – you've earned it!
A Quick Tour of Our Index, Indexer, and Data Source Connection (Optional)
Let's briefly navigate the key components you've set up in Azure AI Search. This guide is focused on where to find these elements and what to expect when you get there.
Take a Closer Look at Your Index
After checking the search results in the Cognitive AI Search service, why not explore a bit more? In your Azure Search Service, navigate to the 'Indexes' section and select 'my-index' once more. Here, you can casually browse through the layout and settings. It's a good spot to see how your data is structured and get familiar with the finer details of your index setup.
Finding the Indexer
For the Indexer, head to the 'Indexers' section within your Search Service. Click on 'my-index-indexer' to see the indexing status and details. This is where the process of feeding data into your index takes place.
Locating the Data Source Connection
To check your Data Source Connection, navigate to 'Data Sources' in the Azure Search Service. Select 'my-index-data-source' to view the configuration that connects your data source to the Indexer.
This is a quick orientation to help you locate and recognize the components you've configured. Each plays a vital role in your Azure AI Search setup, and knowing where to find them is key to managing your search capabilities efficiently.
Navigating Challenges and Opportunities with Azure AI Search Indexes
Balancing Pros and Cons of Azure Indexer
Azure Indexers are a double-edged sword. On the one hand, they offer a quick start, letting you dive into the Search Service without getting bogged down in the complexities of index population. However, as you scale, limitations and errors can become prominent, especially with multiple indexes handling extensive data. While perfect for beginners, for larger-scale operations involving millions of records across numerous indexes, consider investing time in developing a custom indexer.
Caution with Index Infrastructure Changes
Modifying the Search Index Infrastructure requires careful planning. Changes, especially altering field properties, often require a complete index rebuild from scratch. This means losing all data and needing a full re-index, particularly challenging with large datasets. Adding new fields is generally safer, but always plan your index structure with future changes in mind. For detailed guidance, see Azure's index rebuild documentation.
Utilizing Azure AI Search Thoughtfully
Azure AI Search is indeed a powerful tool, yet it's essential to approach its integration thoughtfully. Building your entire application around it can introduce risks. While it's not discouraged, it's crucial to proceed with awareness of these potential challenges.
Preparedness and Backup Strategies
Having backup strategies and contingency plans is sensible, especially for scenarios where the service might face issues. This doesn't diminish the tool's value but highlights the importance of a well-rounded approach to application architecture.
Informed Integration and Exploration
From my own positive experiences with Azure AI Search, I've learned the importance of understanding both its strengths and complexities. It's beneficial to explore Microsoft's documentation in depth and also consider other search service providers. This broader perspective helps in making an informed decision, ensuring Azure AI Search is integrated into your application in a way that maximizes its advantages while being mindful of potential risks.
Essential Tips & Best Practices
Start Slow and Steady
Begin with small-scale Proof of Concept (POC) projects. This approach allows you to test different configurations and understand the tool's capabilities before fully integrating it into your system. As Robert C. Martin aptly puts it, 'The only way to go fast, is to go well.'
Deep Dive into Documentation
Familiarizing yourself with Azure's documentation is key. It helps you understand not just the basics but also the underlying principles of the tool. Don't hesitate to explore offerings from other providers and compare their features and pricing.
Comprehensive Testing is Key
Thorough testing is crucial for long-term success with Search Indexes. This includes validating Search Index data, ensuring smooth index creation during deployments, handling updates, managing indexer timeouts, and more. A well-planned and rigorously tested system can significantly enhance your search capabilities.
Troubleshooting & Additional Information
Dealing with Deployment Issues
If you run into deployment problems, start by checking the deployment logs in your Resource Group. Ensure you're following all the prerequisites as outlined in the documentation for each resource.
Solving Code-Related Problems
The repository provided has been extensively tested. Should you face any issues, please report them on the GitHub issues page, and I'll assist as soon as possible. Remember to configure the appsettings.json file correctly before running the project.
A key point to note: the Books table only supports unique identifiers, so you'll need to delete it if you need to rerun the project.
Looking Ahead: Advanced Search Index Management
In my upcoming posts, I will guide you through the advanced elements of managing Azure AI Search Indexes, catering to those who are incorporating this tool into their systems. We'll navigate together through topics like custom data indexing, complex data modeling, strategies for updating Search Index data, and effective testing methods for Search Indexes and their various components. And there’s more to it. Considering that each business faces unique challenges, we will also explore diverse and complex scenarios. My aim is to provide a path based on my experiences, enabling you to achieve a comprehensive understanding of Search Index management and beyond. And remember, there's always more to learn, so keep exploring on your own!
Wrapping Up Our Journey with Azure AI Search
Our exploration of Azure AI Search has opened a window into efficient, intelligent data management. From creating data sources to building, populating, and querying Search Indexes, we’ve laid the groundwork for you to harness this powerful tool effectively.
The hands-on experience with C# is just the beginning. As we progress, we'll delve into more complex topics such as custom data indexing and dynamic Search Index updates. This exploration will tailor Azure AI Search to suit your specific needs, enhancing your data management strategies.
The road to expertise in Azure AI Search, like any other tool, is a continuous learning process. Embrace the challenges, dive into the documentation, and don't shy away from thorough testing. Each step is an opportunity to grow and innovate.
Thank you for joining this journey. Keep learning and experimenting with Azure AI Search. It's a versatile tool for data management and you're just scratching the surface. Here's to your success in harnessing its full potential!
Additional Resources and Further Reading
General Microsoft Azure AI Search Documentation
Best Practices & Improving Performance
Visual Resources and Tutorials
Azure Cognitive Search (more of an overview)
Cognitive Search - Azure Search with AI (decent demo)
Subscribe to my newsletter
Read articles from Farzam Mohammadi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
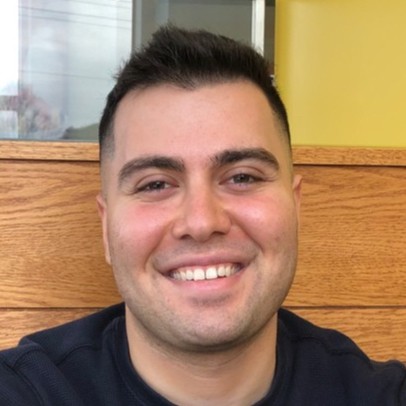
Farzam Mohammadi
Farzam Mohammadi
I'm Farzam, a Software Engineer specializing in backend development. My mission: Collaborate, share proven tricks, and help you avoid the pricey surprises I've encountered along the way.