Create a function that checks if a given path, represented by 'N', 'S', 'E', 'W', crosses itself at any point on a 2D plane.
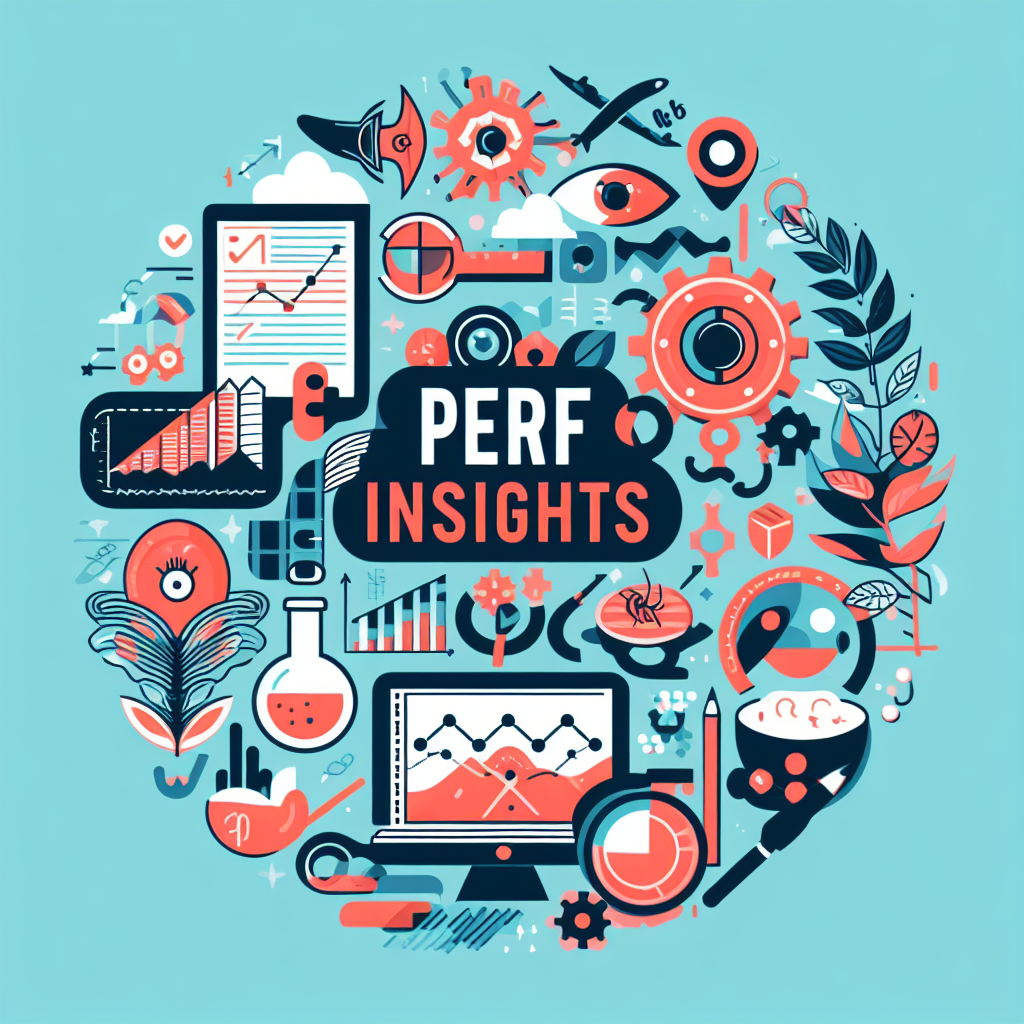
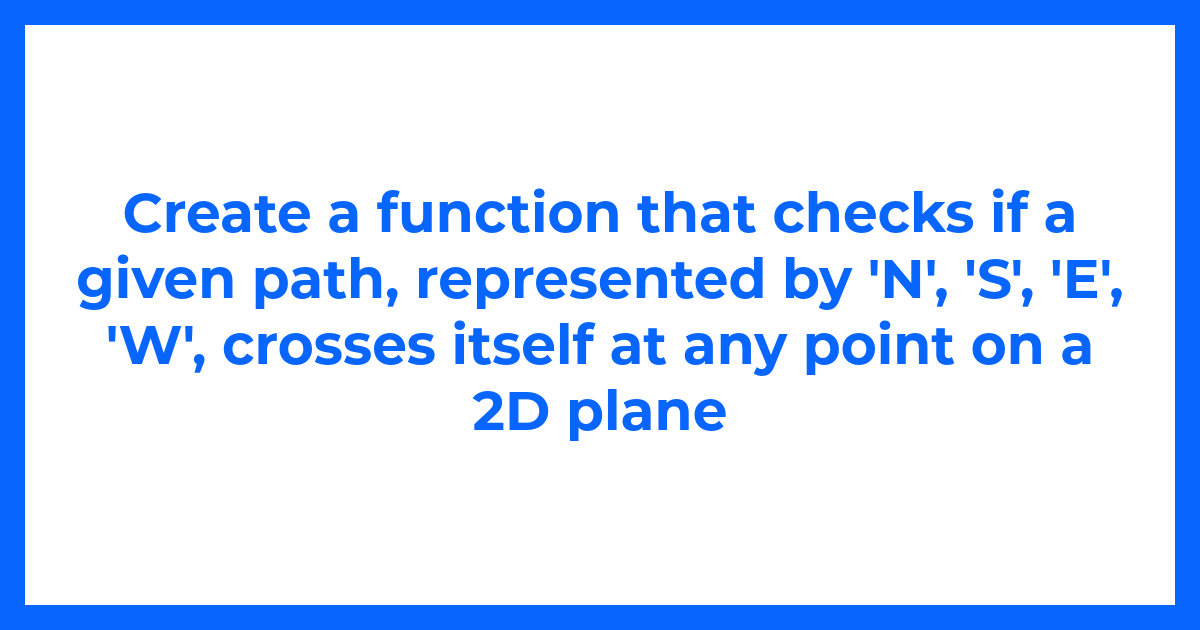
Q - Given a string path
, where path[i] = 'N'
, 'S'
, 'E'
or 'W'
, each representing moving one unit north, south, east, or west, respectively. You start at the origin (0, 0)
on a 2D plane and walk on the path specified by path
.
Return true
if the path crosses itself at any point, that is, if at any time you are on a location you have previously visited. Return false
otherwise.
LeetCode Problem: Link | Click Here
class Solution {
public boolean isPathCrossing(String path) {
// Calculate the length of the input path
int pathLength = path.length();
// Create an array to store visited coordinates, initialized with pathLength+1 capacity
String[] pathCrossed = new String[pathLength + 1];
// Initialize variables to track current coordinates and result status
boolean result = false;
int xCoordinate = 0;
int yCoordinate = 0;
// Start position is at (0,0)
pathCrossed[0] = "0,0";
// Iterate through each character in the input path
for (int i = 0; i < pathLength; i++){
// Update coordinates based on the direction specified in the path
if (path.charAt(i) == 'N'){
yCoordinate++;
} else if (path.charAt(i) == 'E') {
xCoordinate++;
} else if (path.charAt(i) == 'S') {
yCoordinate--;
} else if (path.charAt(i) == 'W') {
xCoordinate--;
}
// Create a string representing current coordinates (x,y)
String check = xCoordinate + "," + yCoordinate;
// Check if the current coordinates have been visited before
for (int j = 0; j <= i; j++) {
if (check.equals(pathCrossed[j])) {
result = true; // Set result to true if the coordinates are revisited
break; // Exit the loop once a match is found
}
}
// If result is true, no need to continue checking, break from the loop
if (result) {
break;
}
// Store the current coordinates in the visited path array
pathCrossed[i + 1] = xCoordinate + "," + yCoordinate;
}
return result; // Return the final result indicating if the path crosses itself
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
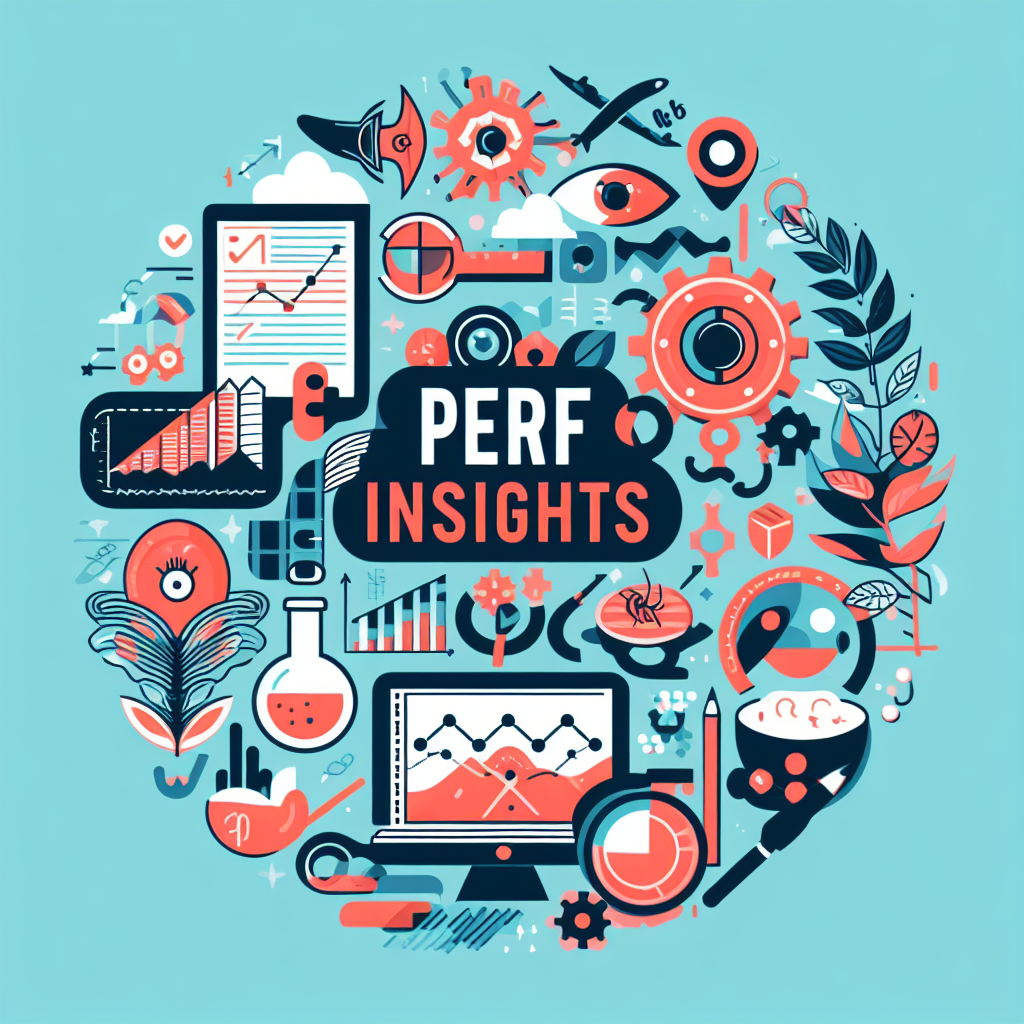
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.