Implementing Uni_Link Feature in Flutter App: A Comprehensive Guide
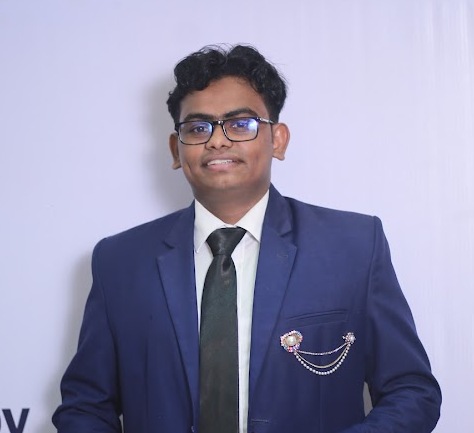

Uni_Link is a powerful feature that enables seamless integration between our app and web content. The ability to activate our app through web-browser-like links provides users with a smooth transition from the web to our app. This documentation will guide us through the steps of implementing Uni_Link on both iOS and Android platforms.
Uni_Link Implementation in Flutter
Uni_Link integration in Flutter involves using the uni_links
package. This package lets you handle deep links on Android and iOS platforms.
Installation
Add the uni_links
package to your pubspec.yaml
file:
dependencies:
uni_links: ^0.6.0
Run:
flutter pub get
Android Setup:
- You need to declare at least one of the two intent filters in
android/app/src/main/AndroidManifest.xml
<manifest ...>
<!-- ... other tags -->
<application ...>
<activity ...>
<!-- ... other tags -->
<!-- Deep Links -->
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accepts URIs that begin with YOUR_SCHEME://YOUR_HOST -->
<data
android:scheme="[YOUR_SCHEME]"
android:host="[YOUR_HOST]" />
</intent-filter>
<!-- App Links -->
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accepts URIs that begin with https://YOUR_HOST -->
<data android:scheme="http" />
<data android:scheme="https" />
<data android:host="[YOUR_HOST]" />
</intent-filter>
</activity>
</application>
</manifest>
Connect with the website:
A Digital Asset Links JSON file must be published on your website to indicate the Android apps associated with the website and verify the app's URL intents. The JSON file uses the following fields to identify related apps:
[{ "relation": ["delegate_permission/common.handle_all_urls"], "target": { "namespace": "android_app", "package_name": "com.example", "sha256_cert_fingerprints": ["14:6D:E9:83:C5:73:06:50:D8:EE:B9:95:2F:34:FC:64:16:A0:83:42:E6:1D:BE:A8:8A:04:96:B2:3F:CF:44:E5"] } }]
[**Note:** need to change
package_name
andsha256_cert_fingerprints
as per your app configuration]*Verify Android App Links:
To verify or generate
assetlinks.json
file, you can go to this site: Generator and TesterPublishing the JSON verification file
You must publish your JSON verification file at the following location:
https://domain.name/.well-known/assetlinks.json
Be sure of the following:
The
assetlinks.json
file is served with content-typeapplication/json
.The
assetlinks.json
file must be accessible over an HTTPS connection, regardless of whether your app's intent filters declare HTTPS as the data scheme.The
assetlinks.json
file must be accessible without any redirects (no 301 or 302 redirects).You must publish the file on each domain if your app links support multiple host domains. See Supporting app linking for various hosts.
Do not publish your app with dev/test URLs in the manifest file that may not be accessible to the public (such as any accessible only with a VPN). A workaround in such cases is to configure build variants to generate a different manifest file for dev builds.
iOS Setup:
For Universal Links, you need to add or create a com.apple
.developer.associated-domains
entitlement - either through Xcode (see below) or by editing (or creating and adding to Xcode) ios/Runner/Runner.entitlements
file.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<!-- ... other keys -->
<key>com.apple.developer.associated-domains</key>
<array>
<string>applinks:[YOUR_HOST]</string>
</array>
<!-- ... other keys -->
</dict>
</plist>
This allows for your app to be started from https://YOUR_HOST
links.
Creating the entitlements file in Xcode:
Open up Xcode by double-clicking on your
ios/Runner.xcworkspace
file.Go to the Project navigator (Cmd+1) and select the
Runner
root item at the very top.Select the
Runner
target and then theSigning & Capabilities
tab.Click the
+ Capability
(plus) button to add a new capability.Type 'associated domains` and select the item.
Double-click the first item in the Domains list and change it from
webcredentials:
example.com
To:applinks:
+ your host (ex: my-fancy-domain.com).A file called
Runner.entitlements
will be created and added to the project.Done.
2. Create an apple-app-site-association
file that contains JSON data about the URLs that your app can handle.
Creating and Uploading the Association File
To create a secure connection between your website and your app, you establish a trust relationship between them. You establish this relationship in two parts:
- An
apple-app-site-association
file that you add to your website
{
"applinks": {
"apps": [],
"details": [
{
"appID": "9JA89QQLNQ.com.apple.wwdc",
"paths": [ "/wwdc/news/", "/videos/wwdc/2015/*"]
},
{
"appID": "ABCD1234.com.apple.wwdc",
"paths": [ "*" ]
}
]
}
}
Don'tDon't append .json
to the apple-app-site-association
filename.
- After you create the
apple-app-site-association
file, upload it to the root of your HTTPS web server or the.well-known
subdirectory. The file needs to be accessible via HTTPS—without any redirects—athttps://<domain>/apple-app-site-association
orhttps://<domain>/.well-known/apple-app-site-association
. Next, you need to handle universal links in your app.
Usage
1. Import the Package
import 'package:uni_links/uni_links.dart';
2. Initialize Link Stream
StreamSubscription sub;
void initUniLinks() {
sub = getLinksStream().listen((String link) {
// Handle the received link
handleLink(link);
}, onError: (err) {
// Handle any errors
print(err);
});
}
void handleLink(String link) {
// Implement logic to handle the Uni_Link
print('Received link: $link');
}
3. Call initUniLinks
in initState
@override
void initState() {
super.initState();
initUniLinks();
}
4. Dispose Stream Subscription
@override
void dispose() {
sub.cancel();
super.dispose();
}
Subscribe to my newsletter
Read articles from Md. Al - Amin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
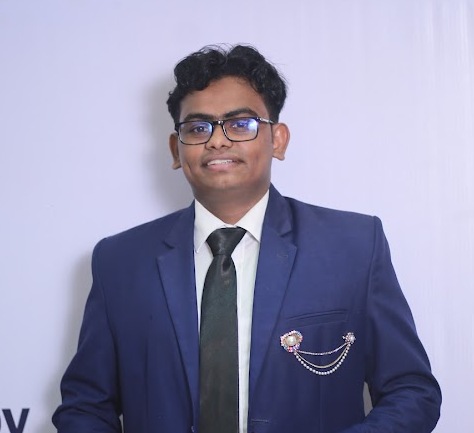
Md. Al - Amin
Md. Al - Amin
Experienced Android Developer with a demonstrated history of working for the IT industry. Skilled in JAVA, Dart, Flutter, and Teamwork. Strong Application Development professional with a Bachelor's degree focused in Computer Science & Engineering from Daffodil International University-DIU.