151 DSA Problem journey

1 min read
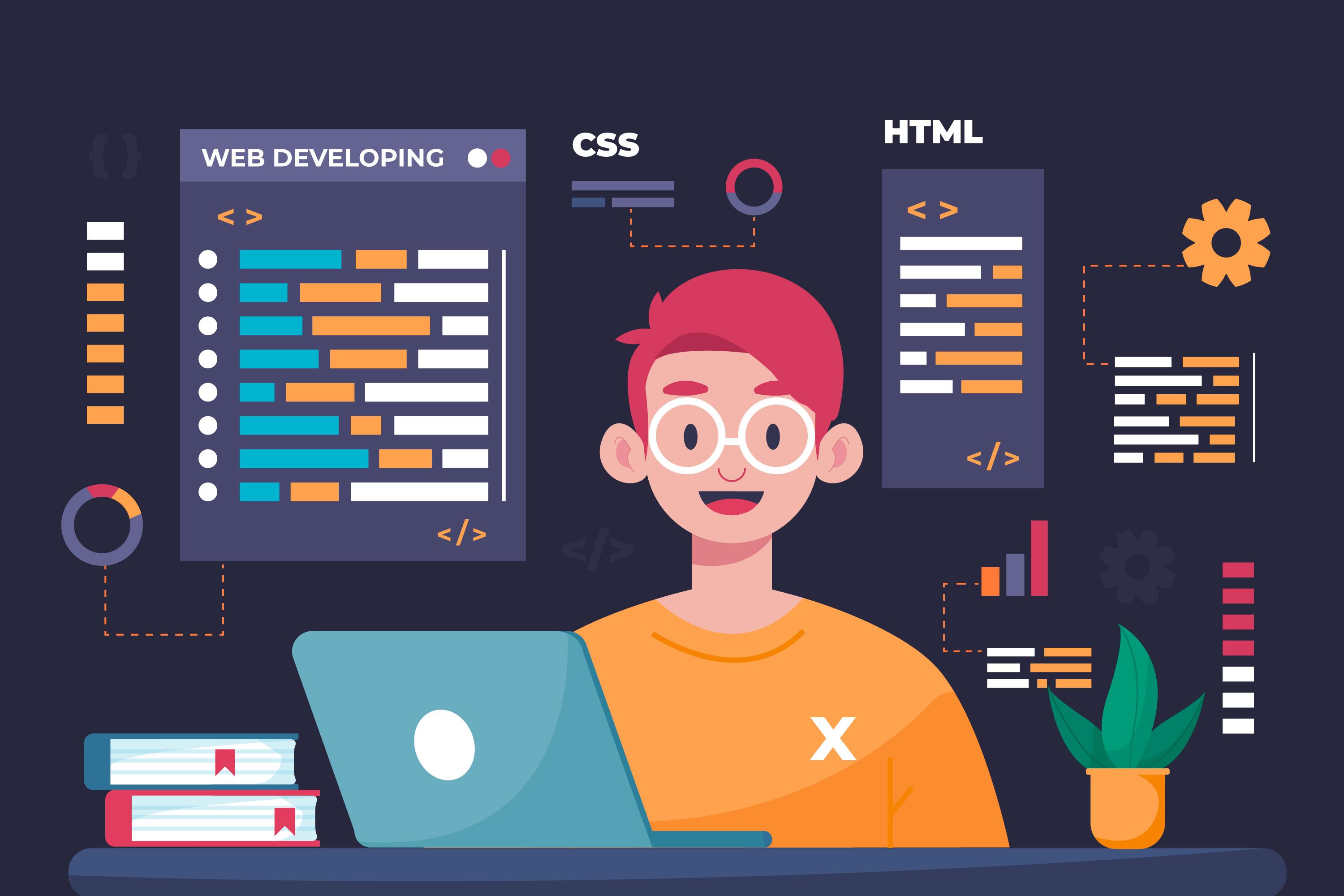
Q17:Given an array Arr[] of size L and a number N, you need to write a program to find if there exists a pair of elements in the array whose difference is N.
Example :
Input:
L = 6, N = 78
arr[] = {5, 20, 3, 2, 5, 80}
Output: 1
Explanation: (2, 80) have difference of 78.
Solution:
bool findPair(int arr[], int size, int n){
sort(arr, arr + size);
int i = 0, j = 1;
while (i<size && j< size){
if(arr[j] - arr[i] == n && j != i){
return true;
}
if(arr[j] - arr[i] > n){
i++;
}
else{
j++;
}
}
return false;
}
Explantion:
>Sorting: The array arr is sorted in ascending order.
>Pointers: Two pointers, i and j, are initialized to 0 and 1, respectively.
>Comparison Loop:
>While i and j are within the array bounds:
>If arr[j] - arr[i] equals n and j is not equal to i, return true.
>If arr[j] - arr[i] is greater than n, increment i.
>If arr[j] - arr[i] is less than or equal to n, increment j.
>Termination: If no such pair is found, return false
17
Subscribe to my newsletter
Read articles from GAURAV YADAV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
C++cppCpp Tutorial #cpp #guideDSAdsa trainingcodingcoding challenge#codenewbiescoding journeyComputer ScienceProgramming Blogsprogramming languagesLearning Journeylearn coding
Written by

GAURAV YADAV
GAURAV YADAV
Experienced Full Stack Developer with proficiency in comprehensive coding skills, adept at crafting end-to-end solutions.