A Developer's Guide to Async Wrapper Functions: From Basics to Best Practices
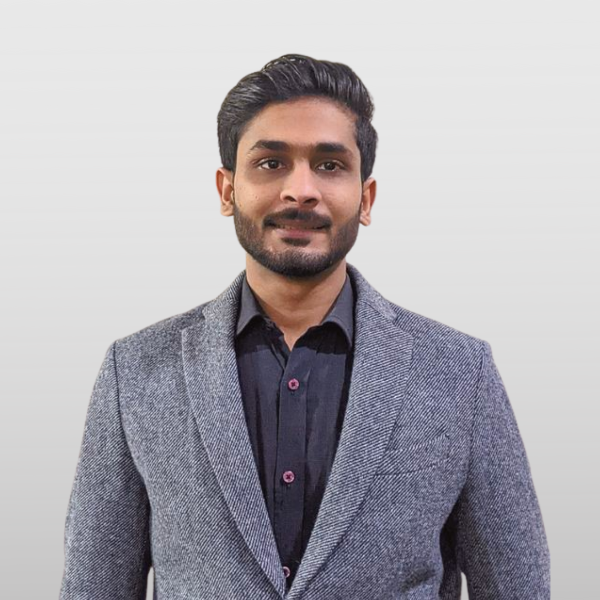
Table of contents
- Introduction:
- Understanding Async/Await:
- Problem Statement: Streamlining Error Handling in Asynchronous Operations:
- Solution Statement: Empowering Asynchronous Operations with Async Wrapper Functions
- Unveiling the Versatility: Various Approaches to Crafting Async Wrapper Functions in JavaScript
- Summary: Elevating Asynchronous JavaScript with Async Wrappers:
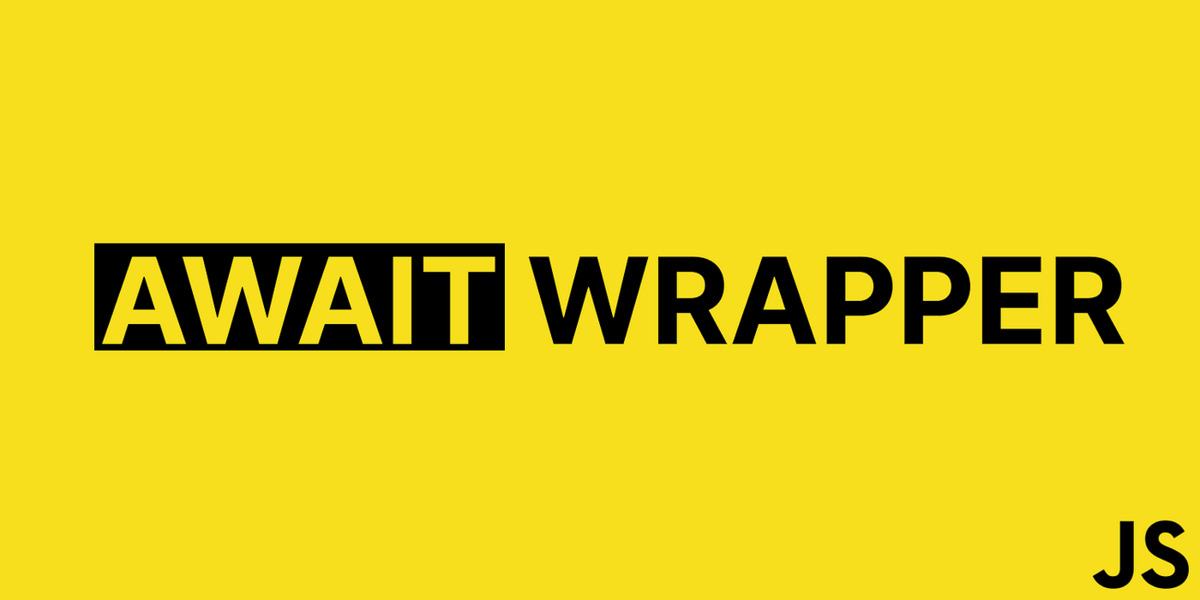
Introduction:
In the dynamic world of JavaScript, managing asynchronous operations efficiently is crucial for building responsive and scalable applications. One powerful technique to handle asynchronous code elegantly is through the use of async wrapper functions. In this blog post, we'll explore what async wrapper functions are, why they're beneficial, and how to implement them with real-world examples.
Understanding Async/Await:
Before diving into async wrapper functions, let's briefly review the async/await syntax in JavaScript. The async
keyword is used to define a function that returns a promise, allowing the use of the await
keyword within that function. await
is used to pause the execution of the async function until the awaited promise is resolved, making asynchronous code appear more synchronous and easier to comprehend.
async function fetchData() {
const data = await fetch('https://api.example.com/data');
const result = await data.json();
console.log(result);
}
While async/await simplifies asynchronous code, it may lead to repetitive error handling.
Problem Statement: Streamlining Error Handling in Asynchronous Operations:
Consider a scenario where you are developing a web application that relies heavily on asynchronous operations, such as fetching data from multiple APIs, handling user interactions, and performing complex computations. As your codebase grows, managing errors becomes a challenging task, with try-catch blocks scattered across different functions. You find it cumbersome to maintain a consistent and centralized error-handling strategy.
Your goal is to enhance the maintainability and readability of your code by implementing a solution that streamlines error handling in asynchronous operations. The solution should allow for:
Centralized Error Handling: Errors occurring in any asynchronous operation should be handled in a single, centralized location to provide a clear overview of potential issues.
Code Reusability: The solution should promote code reusability, enabling you to apply a standardized error-handling approach across various asynchronous functions without duplicating error-handling logic.
Improved Readability: The main business logic of your functions should remain focused on the core tasks, with error-handling details abstracted away to enhance overall code readability.
Your task is to design and implement an approach that addresses these challenges. Provide a concise and reusable solution that developers can integrate seamlessly into their codebase, promoting best practices for error handling in asynchronous JavaScript operations.
Solution Statement: Empowering Asynchronous Operations with Async Wrapper Functions
In response to the challenge of streamlining error handling in asynchronous operations, we propose the use of Async Wrapper Functions as a powerful and elegant solution. Async wrapper functions act as a higher-order function that encapsulates asynchronous logic, providing a centralized and reusable approach to error handling.
Async Wrapper Function:
An async wrapper is a higher-order function in JavaScript designed to encapsulate asynchronous operations and streamline error handling. Its primary purpose is to enhance the readability, maintainability, and reusability of asynchronous code by providing a centralized mechanism for handling errors.
Here's a brief breakdown of how an async wrapper typically works:
Higher-Order Function:
- An async wrapper is a higher-order function, meaning it takes another function (typically an asynchronous one) as an argument.
Returns a Function:
- The async wrapper returns a new function, often wrapping the original asynchronous function.
Centralized Error Handling:
The wrapper function contains a try-catch block or utilizes the
catch
method on the Promise returned by the async function.If an error occurs during the execution of the asynchronous function, it is caught, logged, and then rethrown for further handling.
Usage with Async/Await:
- Developers can use the wrapped asynchronous function with the
await
keyword, allowing them to await the resolution of the wrapped asynchronous operation.
- Developers can use the wrapped asynchronous function with the
Here's a simplified example of an async wrapper:
function asyncWrapper(asyncFunction) {
return async function (...args) {
try {
return await asyncFunction(...args);
} catch (error) {
console.error('Error:', error);
throw error;
}
};
}
const wrappedAsyncFunction = asyncWrapper(async function exampleAsyncFunction() {
// Asynchronous logic here
});
// Usage
try {
const result = await wrappedAsyncFunction();
console.log(result);
} catch (error) {
// Handle errors if needed
console.error('Error:', error);
}
In this example, asyncWrapper
takes an asynchronous function as an argument and returns a new function that wraps the original one. If an error occurs during the execution of exampleAsyncFunction
, it is caught and logged within the wrapper, providing a centralized error-handling mechanism.
Key Features of the Solution:
Centralized Error Handling:
By wrapping asynchronous functions with an async wrapper, errors are consistently handled in one central location.
The async wrapper catches and logs errors, offering a clear overview of potential issues in your application.
function asyncWrapper(asyncFunction) {
return function (...args) {
return asyncFunction(...args).catch((error) => {
console.error('Error:', error);
throw error;
});
};
}
const wrappedAsyncFunction = asyncWrapper(async function exampleAsyncFunction() {
// Asynchronous logic here
});
Code Reusability:
The generic nature of async wrapper functions promotes code reusability across various asynchronous operations.
The same error-handling strategy can be applied consistently to different async functions without duplicating error-handling logic.
const wrappedFetchData = asyncWrapper(async function fetchData(url) {
const data = await fetch(url);
const result = await data.json();
console.log(result);
});
const wrappedProcessData = asyncWrapper(async function processData(data) {
// Core logic without error handling concerns
const result = await processDataAsync(data);
return result;
});
Improved Readability:
Async wrapper functions abstract away error-handling details, allowing the main code to focus on essential business logic.
The separation of concerns enhances the overall readability of the codebase, making it more accessible and maintainable.
try {
const result = await wrappedAsyncFunction();
console.log(result);
} catch (error) {
// Handle errors if needed
console.error('Error:', error);
}
Unveiling the Versatility: Various Approaches to Crafting Async Wrapper Functions in JavaScript
Introduction:
In the realm of JavaScript development, async wrapper functions stand out as indispensable tools for streamlining error handling in asynchronous operations. However, the beauty of these wrappers lies not only in their utility but also in the diverse ways they can be implemented. In this blog section, we'll explore different approaches to crafting async wrapper functions, showcasing their versatility and empowering developers with multiple techniques to enhance their asynchronous code.
1. Classic Function Declaration:
One straightforward method for creating an async wrapper function is through a classic function declaration. This approach is simple and provides a clear structure for error handling.
function asyncWrapper(asyncFunction) {
return async function (...args) {
try {
return await asyncFunction(...args);
} catch (error) {
console.error('Error:', error);
throw error;
}
};
}
const wrappedAsyncFunction = asyncWrapper(async function exampleAsyncFunction() {
// Asynchronous logic here
});
// Usage
try {
const result = await wrappedAsyncFunction();
console.log(result);
} catch (error) {
// Handle errors if needed
console.error('Error:', error);
}
2. Arrow Function Expression:
For a more concise syntax, an arrow function expression can be used to define the async wrapper. This approach is particularly beneficial for creating compact and expressive code.
const asyncWrapper = (asyncFunction) => async (...args) => {
try {
return await asyncFunction(...args);
} catch (error) {
console.error('Error:', error);
throw error;
}
};
const wrappedAsyncFunction = asyncWrapper(async () => {
// Asynchronous logic here
});
// Usage remains the same as in the previous example
3. Custom Error Handling Logic:
Async wrapper functions can be tailored to include custom error-handling logic beyond simple logging. This could involve sending error reports to a server, displaying user-friendly messages, or taking specific actions based on the type of error.
const advancedAsyncWrapper = (asyncFunction, errorHandler) => async (...args) => {
try {
return await asyncFunction(...args);
} catch (error) {
errorHandler(error);
throw error;
}
};
const customErrorHandler = (error) => {
// Custom error handling logic
console.error('Custom Error Handling:', error);
};
const wrappedAsyncFunction = advancedAsyncWrapper(async () => {
// Asynchronous logic here
}, customErrorHandler);
// Usage remains the same as in the first example
Conclusion:
The art of crafting async wrapper functions is not limited to a single approach. Developers can choose the style that aligns with their preferences and project requirements. Whether opting for classic function declarations, concise arrow function expressions, or incorporating custom error handling, the versatility of async wrappers empowers developers to wield these tools effectively in diverse scenarios. By exploring these different methods, developers can discover the approach that best fits their coding style and project needs, ultimately contributing to more maintainable and error-resilient asynchronous JavaScript code.
Summary: Elevating Asynchronous JavaScript with Async Wrappers:
In this comprehensive exploration, we've navigated the dynamic landscape of JavaScript's asynchronous operations, emphasizing their pivotal role in building responsive and scalable applications. The blog introduces async wrapper functions as a sophisticated tool to elegantly handle asynchronous code, addressing the challenges posed by the repetitive nature of error handling in async/await syntax.
The problem statement highlights the complexities of managing errors in a growing codebase, setting the stage for the proposed solution: Async Wrapper Functions. This solution, characterized by centralized error handling, code reusability, and improved readability, emerges as a robust approach to streamline error management in asynchronous operations.
Taking a closer look, the blog offers a diverse array of crafting approaches for async wrappers. Whether through classic function declarations, concise arrow function expressions, or the integration of custom error-handling logic, developers are encouraged to tailor their async wrappers to align with their coding preferences and project requirements.
In essence, this exploration is an invitation to JavaScript developers to embrace the versatility of async wrappers. By doing so, they can elevate their asynchronous code to new heights, fostering maintainability and resilience in the face of errors. Armed with a deeper understanding of async wrappers and various crafting techniques, developers are empowered to shape a more efficient and readable codebase, contributing to the success of their JavaScript projects.
As we conclude our exploration of async wrappers, we encourage you to enhance your asynchronous coding. Experiment, share your experiences, and let us know your thoughts for future articles.
Stay connected with JavaScript best practices by subscribing to our blog. Join a community of passionate developers dedicated to continuous learning.
Thank you for being part of this journey. Your commitment fuels the coding community. Until our next exploration, happy coding! Subscribe for updates and continue your quest for mastery in JavaScript.
Subscribe to my newsletter
Read articles from Muhammad Umair directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
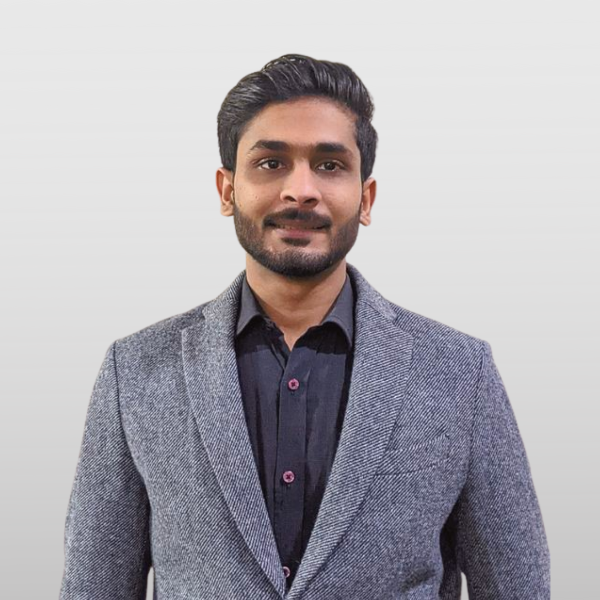
Muhammad Umair
Muhammad Umair
JS Developer | Web Developer