JavaScript Object & it's Methods
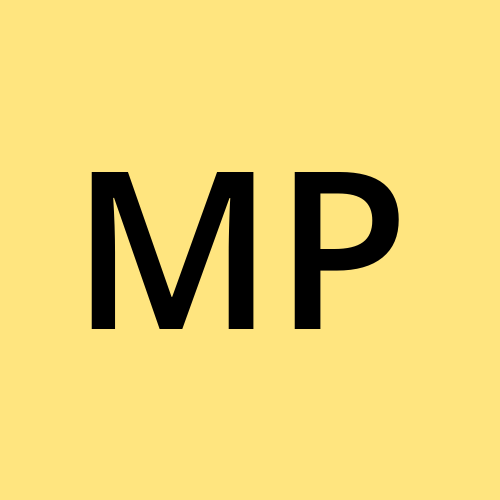
What is Object in JavaScript ?
In JavaScript, an object is a fundamental data type that allows developers to store collections of key-value pairs. It's a versatile and dynamic entity used to represent real-world entities, data structures, and more complex entities in the language. Objects in JavaScript are a cornerstone of the language's flexibility and are used extensively across various applications.
Properties of JavaScript Objects:
Key-Value Pairs:
An object consists of key-value pairs where the key is a string and the value can be any data type: primitives, objects, functions, etc.
let person = { name: 'John', age: 30, city: 'New York' };
Dynamic Nature:
- Objects in JavaScript are mutable, meaning their properties can be added, updated, or deleted after creation.
javascriptCopy codeperson.email = 'john@example.com';
// Adding a new property
person.age = 31; // Updating an existing property
delete person.city; // Deleting a property
Accessing Object Properties:
- Properties of an object can be accessed using dot notation or bracket notation.
console.log(person.name); // Output: 'John'
console.log(person['age']); // Output: 31
Object Methods:
- Objects can contain functions as values, which are referred to as methods.
let car = {
brand: 'Toyota',
year: 2020,
start: function() {
console.log('Engine started');
}
};
car.start(); // Output: 'Engine started'
Object Constructors:
JavaScript also allows object creation using constructor functions or class syntax.
// Constructor function function Person(name, age) { this.name = name; this.age = age; } let person1 = new Person('Alice', 25);
Object as a Reference Type:
In JavaScript, objects are reference types. When assigned to a variable or passed as an argument, they are passed by reference, meaning the variable holds a reference (address) to the object in memory, not the object itself.
let obj1 = { name: 'John' };
let obj2 = obj1; // obj2 now references the same object as obj1
obj2.name = 'Jane';
console.log(obj1.name); // Output: 'Jane' (Both obj1 and obj2 reference the same object)
The Object Prototype:
Each object in JavaScript has a prototype from which it inherits methods and properties. The prototype property allows you to add properties and methods to an object.
let student = {
name: 'Alice',
age: 20
};
// Adding a new method to the prototype
Object.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}.`);
};
student.greet(); // Output: 'Hello, my name is Alice.'
In essence, objects in JavaScript provide a powerful and flexible way to store and manipulate data, making them a fundamental aspect of the language's functionality and versatility.
Subscribe to my newsletter
Read articles from MAYANK VIKRAMBHAI PARMAR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
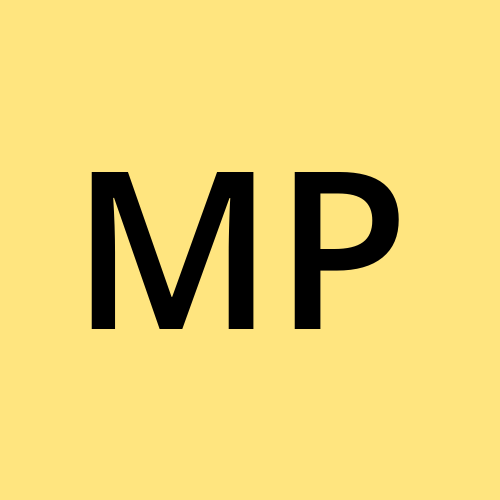