Flow of code execution in JavaScript
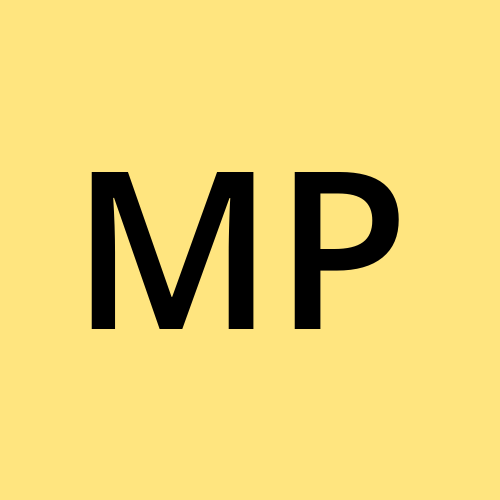
Six concise points outlining JavaScript code execution:
Parsing and Creation Phase:
Reading the code and understanding its structure.
Creation of variable and function declarations in memory.
Global Execution Context:
Execution of code outside of functions.
Sequential execution from top to bottom.
Function Execution Context:
Creation of a new context when a function is called.
Execution of code within the function's scope.
Scope Chain and Variable Lookup:
Searching for variables in nested scopes.
Lookup mechanism moving from local to global scopes.
Synchronous Execution:
Single-threaded execution, handling one operation at a time.
Blocking nature where operations are completed sequentially.
Asynchronous Operations:
Handling non-blocking operations like fetching data or timers.
Utilizing callbacks or promises to manage asynchronous tasks.
Example :
Picture JavaScript as a chef following a recipe step by step.
1. Reading the Recipe:
Imagine the chef reading a recipe book (your JavaScript code). They start at the beginning and read each line carefully. This is similar to how JavaScript reads and understands your code.
2. Getting Ingredients Ready (Global Scope):
Before cooking anything, the chef gathers all the ingredients needed for the entire recipe. Similarly, JavaScript checks and prepares all the variables and functions that are needed throughout the whole script. These are kept in the 'pantry' of memory.
3. Cooking a Dish (Function Execution):
When the chef reaches a step that says "cook a dish" (calls a function), they move to a new part of the recipe book dedicated to that dish. In JavaScript, when a function is called, the code jumps to that specific part of the script and starts following the instructions there.
4. Following the Steps (Synchronous Execution):
The chef follows the steps of the recipe one by one, in order. Similarly, JavaScript executes your code line by line, in the order it's written, without skipping anything.
5. Asking for Help (Scope and Scope Chain):
If the recipe mentions an ingredient (variable) that's not in the kitchen (current scope), the chef asks someone nearby (scope chain) to get it. If they can't find it nearby, they'll keep asking different people until they find what they need or check the main pantry (global scope).
6. Handling Multiple Dishes (Async Operations):
Sometimes, while cooking, the chef might put something in the oven and continue working on other dishes. Similarly, in JavaScript, certain actions like waiting for a timer or fetching data can happen 'in the background', allowing other parts of the code to keep running without waiting for them to finish.
7. Juggling Tasks (Event Loop and Callback Queue):
Imagine the chef cooking multiple dishes at once. When one dish needs to bake (asynchronous operation), they don't wait in front of the oven. Instead, they check on other dishes until the oven beeps (callback). In JavaScript, the event loop works like this, checking if there are any finished tasks (callbacks) while the code continues.
Example: Making a Sandwich 🥪
Imagine writing JavaScript code to make a sandwich. Here's how the flow of code execution might look:
Step 1: Ingredients (Global Scope)
let sandwich = ""; // Global variable to hold the final sandwich
function addIngredient(ingredient) {
sandwich += ingredient + " ";
}
addIngredient("Bread"); // Calling the function to add ingredients
addIngredient("Cheese");
addIngredient("Tomato");
addIngredient("Lettuce");
- Explanation: Here, we have a function
addIngredient
that takes an ingredient and adds it to thesandwich
variable. We call this function multiple times to add ingredients to the sandwich.
Step 2: Function Execution
function prepareSandwich() {
console.log("Preparing the sandwich...");
console.log("Adding ingredients: " + sandwich);
}
prepareSandwich(); // Call the function to prepare the sandwich
- Explanation: Now, we have another function
prepareSandwich
that logs the process of preparing the sandwich and displays the final list of ingredients.
Understanding the Flow:
Reading the Recipe (Code): JavaScript reads the code from top to bottom.
Global Scope: It first prepares the
addIngredient
function and thesandwich
variable.Function Execution: When
addIngredient
is called, it adds each ingredient to thesandwich
.Calling
prepareSandwich
: Finally, it executesprepareSandwich
, which logs the process and displays the prepared sandwich.
Output:
Preparing the sandwich...
Adding ingredients: Bread Cheese Tomato Lettuce
Conclusion:
This example mimics the flow of code execution in JavaScript. Just like following a recipe to make a sandwich, JavaScript starts from the top, prepares functions and variables, executes functions when called, and progresses through the code step by step, producing the desired result—in this case, a delicious sandwich!
Subscribe to my newsletter
Read articles from MAYANK VIKRAMBHAI PARMAR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
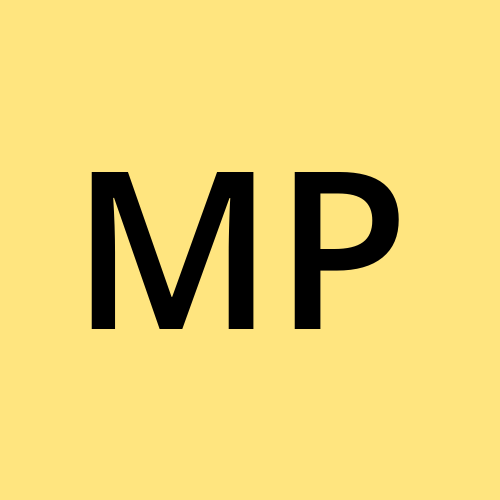