Functions in Javascript
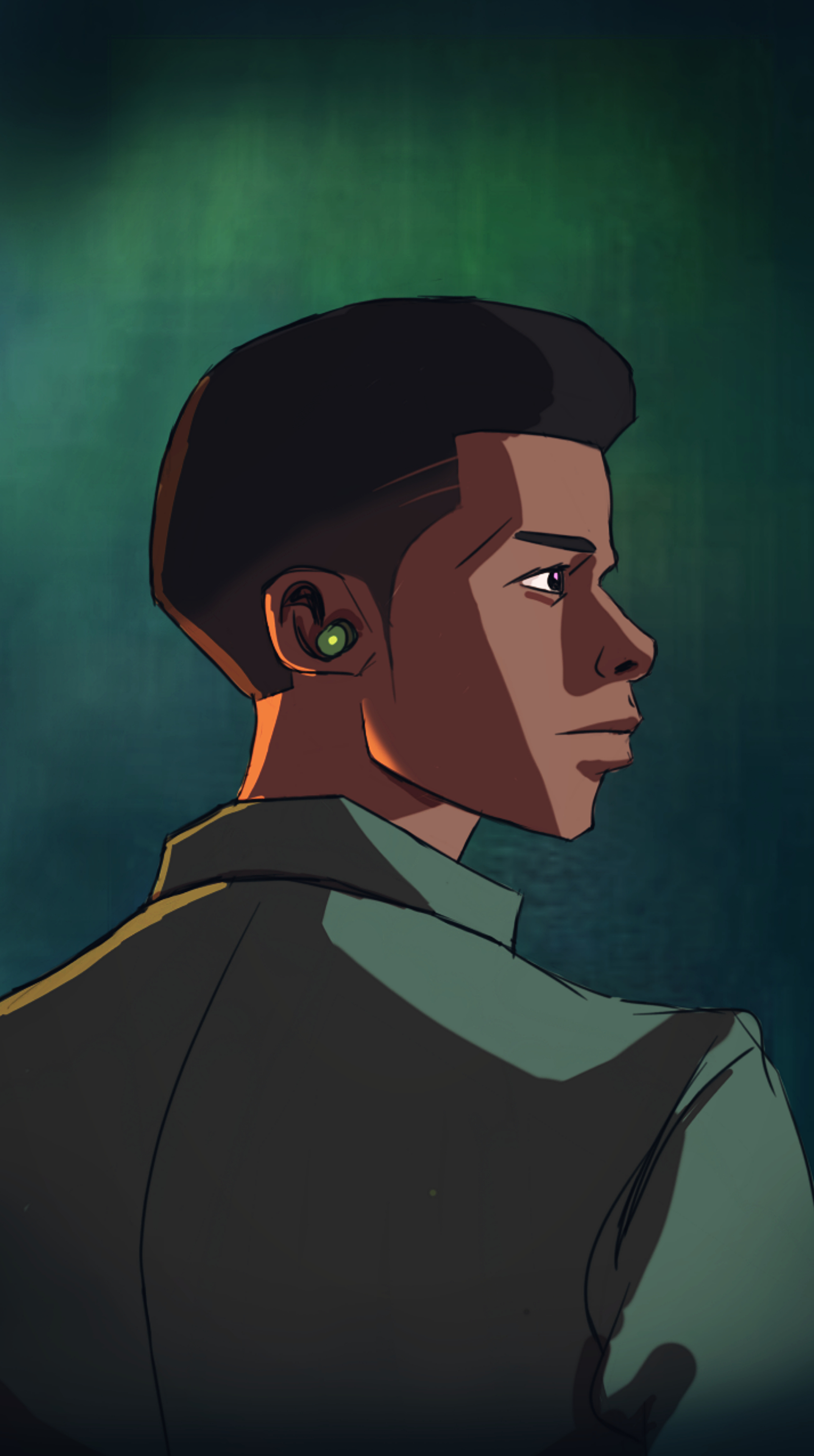
Table of contents
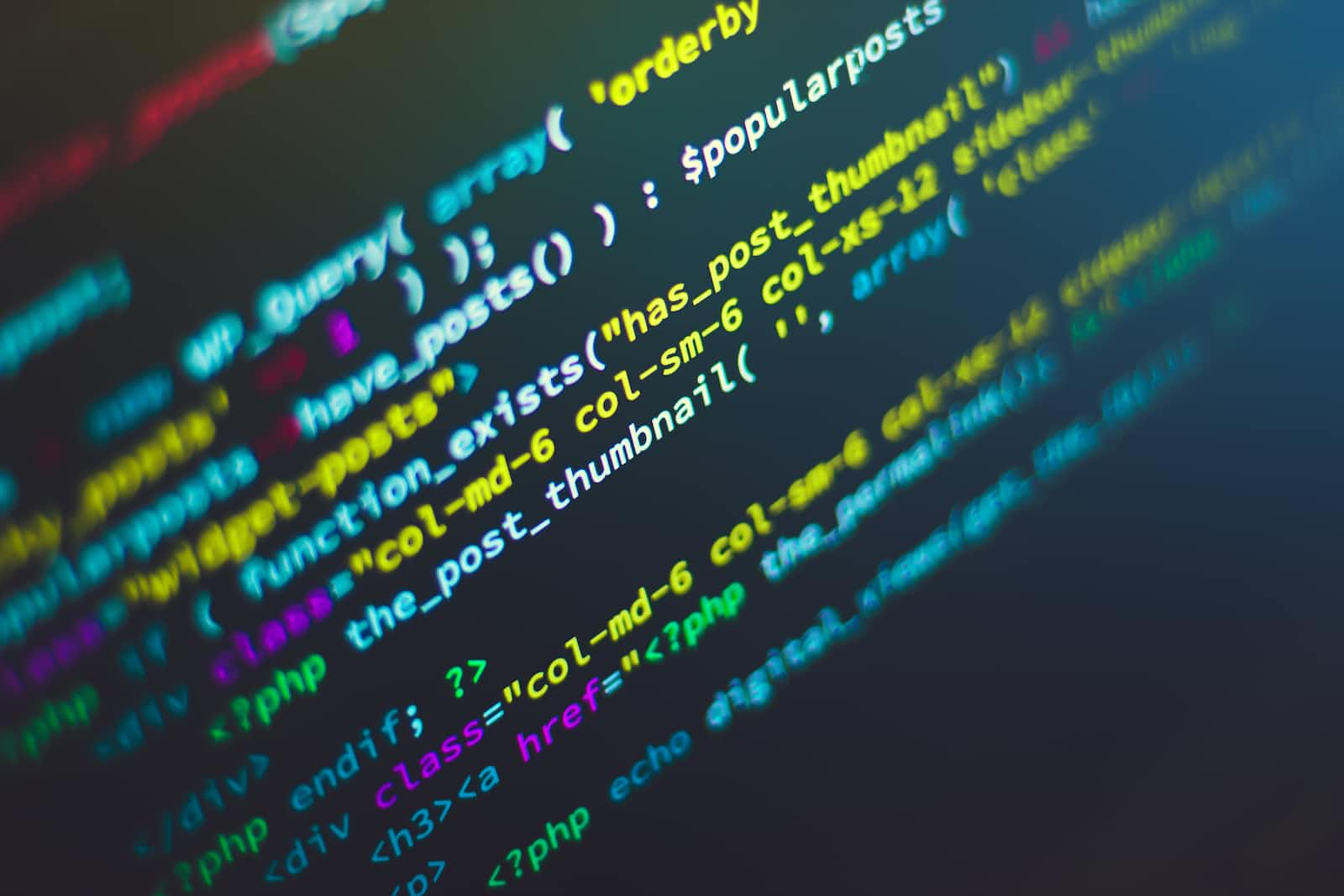
Functions in programming are one of the most important control structures for ensuring optimal and readable code. To understand the concept of functions, we will take a little trip to the world of mathematics where we derive formulas to solve problems. These functions usually take certain values we call parameters and perform some operation on them as defined by the formula.
Functions are similar as they define a series of instructions to be performed by the computer on some values called parameters or arguments. It enables us to have reusable code which we can use at any point in our program and as many times as we want to by simply calling it at any point. Functions are the main “building blocks” of a program. They allow the code to be called many times without repetition.
Some built in functions are the alert(message)
, prompt(message, default)
and confirm(question)
. We can also create functions of our own in every programming language but we'll be using Javascript.
Function Declaration
To create a function, we use something called a function declaration, it looks like this
function sayHello() {
alert("Hello world")
}
The function
keyword goes first, next is the name of the function, then a list of parameters between the parentheses (it can also be empty as in this example, we'll see examples with parameters) and finally the code of the function which is executed when the function is executed, also named “the function body”, between curly braces.
To call our function, we simply use it's name with curly braces after it sayHello()
function sayHello() {
alert("Hello world")
}
//calling our function
sayHello()
sayHello()
We can call the functions as many times as we want and it calls the code in it every time.
The call sayHello()
executes the code of the function. Here we will see the message two times.
This example clearly demonstrates one of the main purposes of functions: to avoid code duplication.
If we ever need to change the message or the way it is shown, it’s enough to modify the code in one place: the function which outputs it.
VARIABLES
There are two types of variables accessed by functions. Global variables and local variables.
Global variables are declared outside of the function block and are accessed and used inside of the function.
let message = "Hello World"
function(){
alert(message)
}
Local variables are declared and used inside of the function.
function(){
let message = "Hello World"
alert(message)
}
If a variable is declared globally and declared again locally, the local variable will override the global declarartion,
let message = "Good morning"
function(){
let message = "Hello World"
alert(message) // alerts "Hello world"
}
Both ways will work but it's usually good practice to use local variables to prevent unexpected behavior and keep track of variables. Modern code has few or no globals. Most variables reside in their functions. Sometimes though, they can be useful to store project-level data.
PARAMETERS
Parameters act as placeholder variables which will be used in the function and they are passed into the function when it is called.
The function below adds two numbers
function addTwoNumbers(num1, num2) {
let sum = num1 + num2
}
addTwoNumbers(2, 3)
The variables num1 and num2 are the arguments which are added and assigned to the sum variable. When the function is called the values 2 and 3 are passed to num1 and num2 respectively. The value of sum will be 5 in this case.
A parameter is the variable listed inside the parentheses in the function declaration (it’s a declaration time term).
An argument is the value that is passed to the function when it is called (it’s a call time term).
We declare functions listing their parameters, then call them passing arguments.
In the example above, one might say: "the function addTwoNumbers
is declared with two parameters, then called with two arguments: 2 and 3".
THE RETURN STATEMENT
The return
statement in functions is used to stop function execution and return a result to the function caller. Think of it this way, when a function is called the return statement takes the value and stores it inside the function, this value is then either passed to a variable or printed to the console.
function addTwoNumbers(num1, num2) {
let sum = num1 + num2
return sum
}
addTwoNumbers(2, 3) //stores the value but doesn't print
console.log(addTwoNumbers(2, 3)) // prints the return value
NB:
Functions can also be written as values to variables.
const addTwoNumbers = function (num1, num2) {
let sum = num1 + num2
return sum
}
addTwoNumbers(2, 3)
Here the name of the function is used as a variable to hold the function and the return value is passed to the variable. The function is called in the same way using the name.
You have learnt the basics of functions in Javascript, they are very useful for making code easier to write and reuse. They are also the building blocks for React, a JavaScript frontend framework. Try out and experiment other ways you can use functions.
Happy Coding ✈️
Subscribe to my newsletter
Read articles from Obadimeji David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
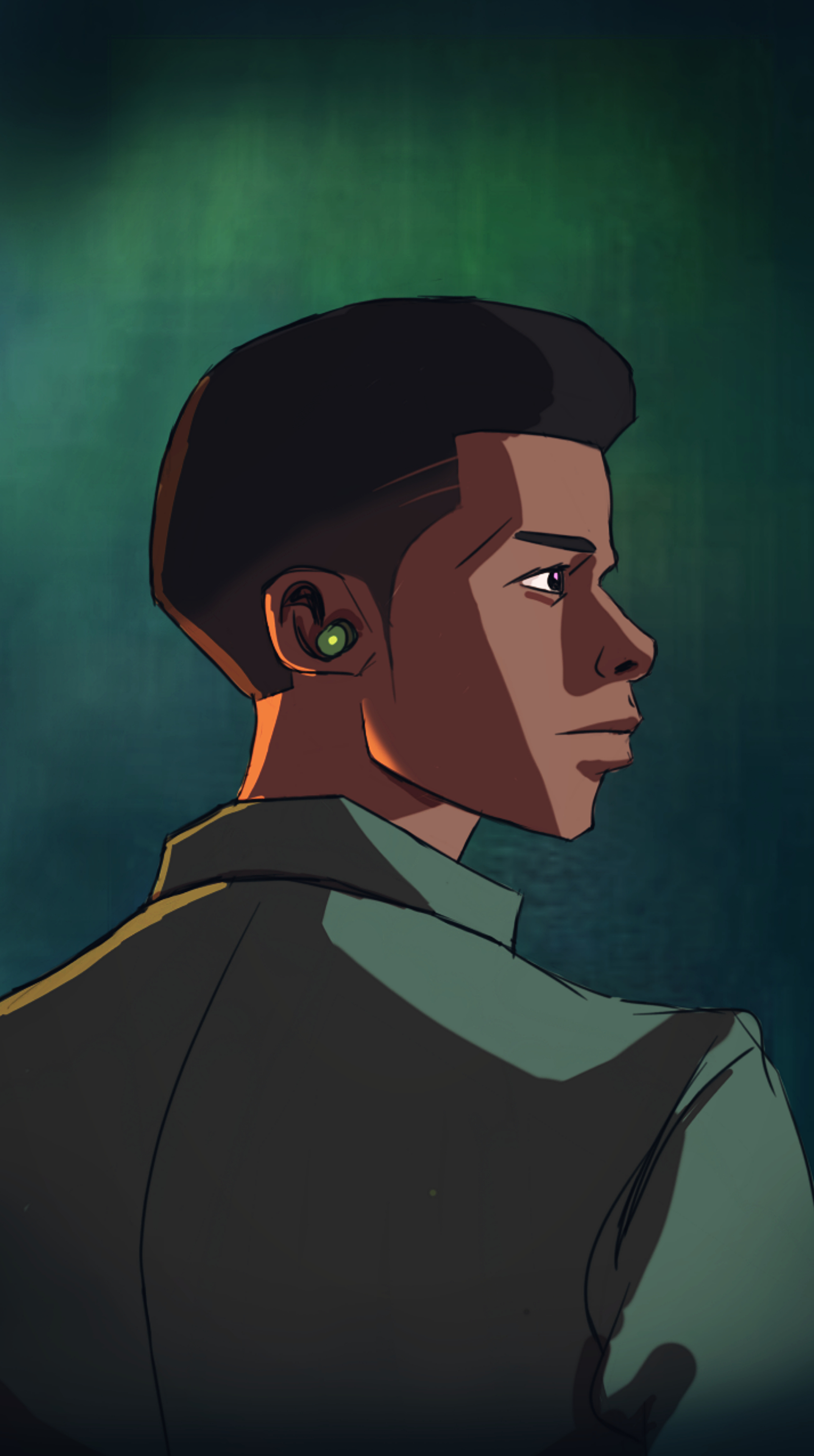
Obadimeji David
Obadimeji David
I am a Full-stack software developer with a passion for using tech to solve real world problems by building impactful software solutions to improve lives.