Python Math Module
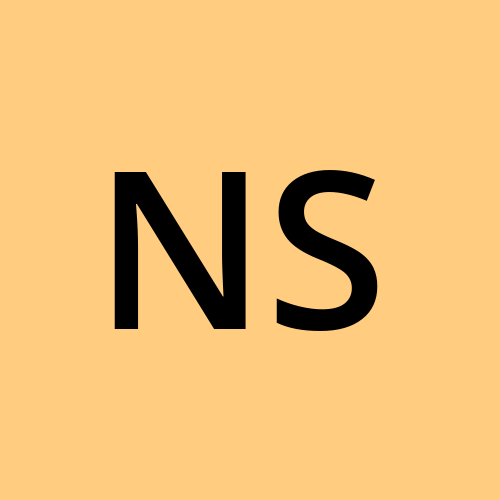
Basic Mathematical Functions:
math.sqrt()
: Calculates the square root of a number.math.pow()
: Raises a number to a specified power.math.exp()
: Calculates the exponential of a number.math.log()
: Calculates the natural logarithm of a number.math.log10()
: Calculates the base-10 logarithm of a number.math.ceil()
: Rounds a number up to the nearest integer.math.floor()
: Rounds a number down to the nearest integer.math.trunc()
: Truncates a number to an integer.math.degrees()
: Converts radians to degrees.math.radians()
: Converts degrees to radians.
Trigonometric Functions:
math.sin()
: Calculates the sine of an angle in radians.math.cos()
: Calculates the cosine of an angle in radians.math.tan()
: Calculates the tangent of an angle in radians.math.asin()
: Calculates the arcsine of a value, returning the angle in radians.math.acos()
: Calculates the arccosine of a value, returning the angle in radians.math.atan()
: Calculates the arctangent of a value, returning the angle in radians.math.atan2()
: Calculates the arctangent of y/x, returning the angle in radians.math.hypot()
: Calculates the Euclidean norm (hypotenuse) of two numbers.
Mathematical Constants:
math.pi
: Mathematical constant π (pi).math.e
: Mathematical constant e (Euler's number).math.inf
: Mathematical representation of positive infinity.math.nan
: Mathematical representation of NaN (not a number).
Hyperbolic Functions:
math.sinh()
: Calculates the hyperbolic sine of a number.math.cosh()
: Calculates the hyperbolic cosine of a number.math.tanh()
: Calculates the hyperbolic tangent of a number.math.asinh()
: Calculates the inverse hyperbolic sine of a number.math.acosh()
: Calculates the inverse hyperbolic cosine of a number.math.atanh()
: Calculates the inverse hyperbolic tangent of a number.
Other Functions:
math.factorial()
: Calculates the factorial of a number.math.gcd()
: Calculates the greatest common divisor of two integers.math.isfinite()
: Checks if a number is finite.math.isinf()
: Checks if a number is infinite.math.isnan()
: Checks if a number is NaN.
Example of Usage:
import math
# Basic Mathematical Functions
sqrt_value = math.sqrt(25)
log_value = math.log(10)
sin_value = math.sin(math.radians(90))
# Mathematical Constants
pi_value = math.pi
e_value = math.e
# Trigonometric Functions
tan_value = math.atan(1)
hypot_value = math.hypot(3, 4)
# Other mathematical functions and constants can be used similarly
The math
module provides a wide range of mathematical functions and constants for various calculations in Python.
Subscribe to my newsletter
Read articles from Namya Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
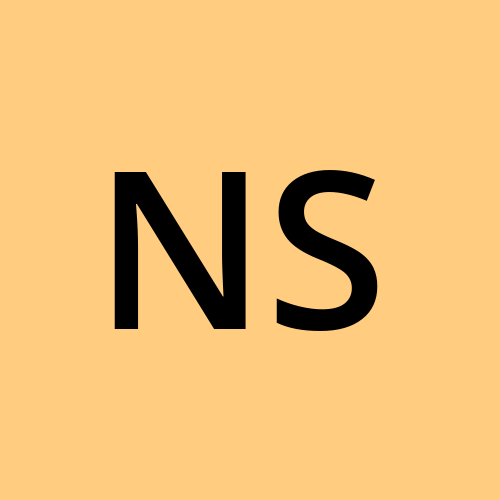
Namya Shah
Namya Shah
I am a developer who is very enthusiast about technology and coding.