Objects their Properties and Methods with destructuring in JavaScript in depth
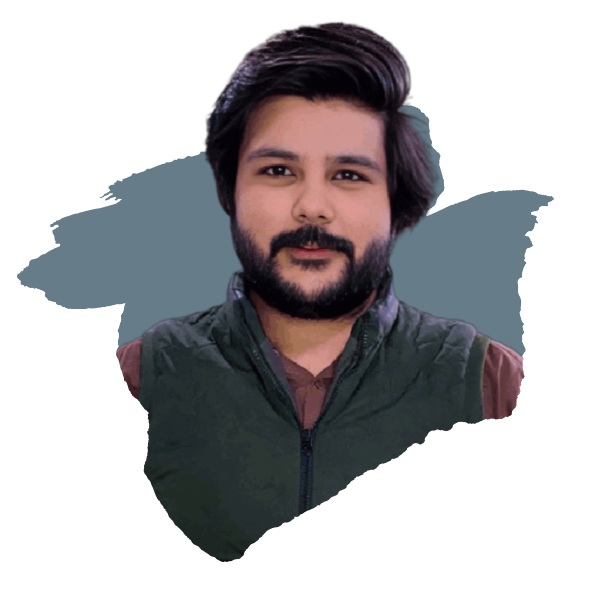
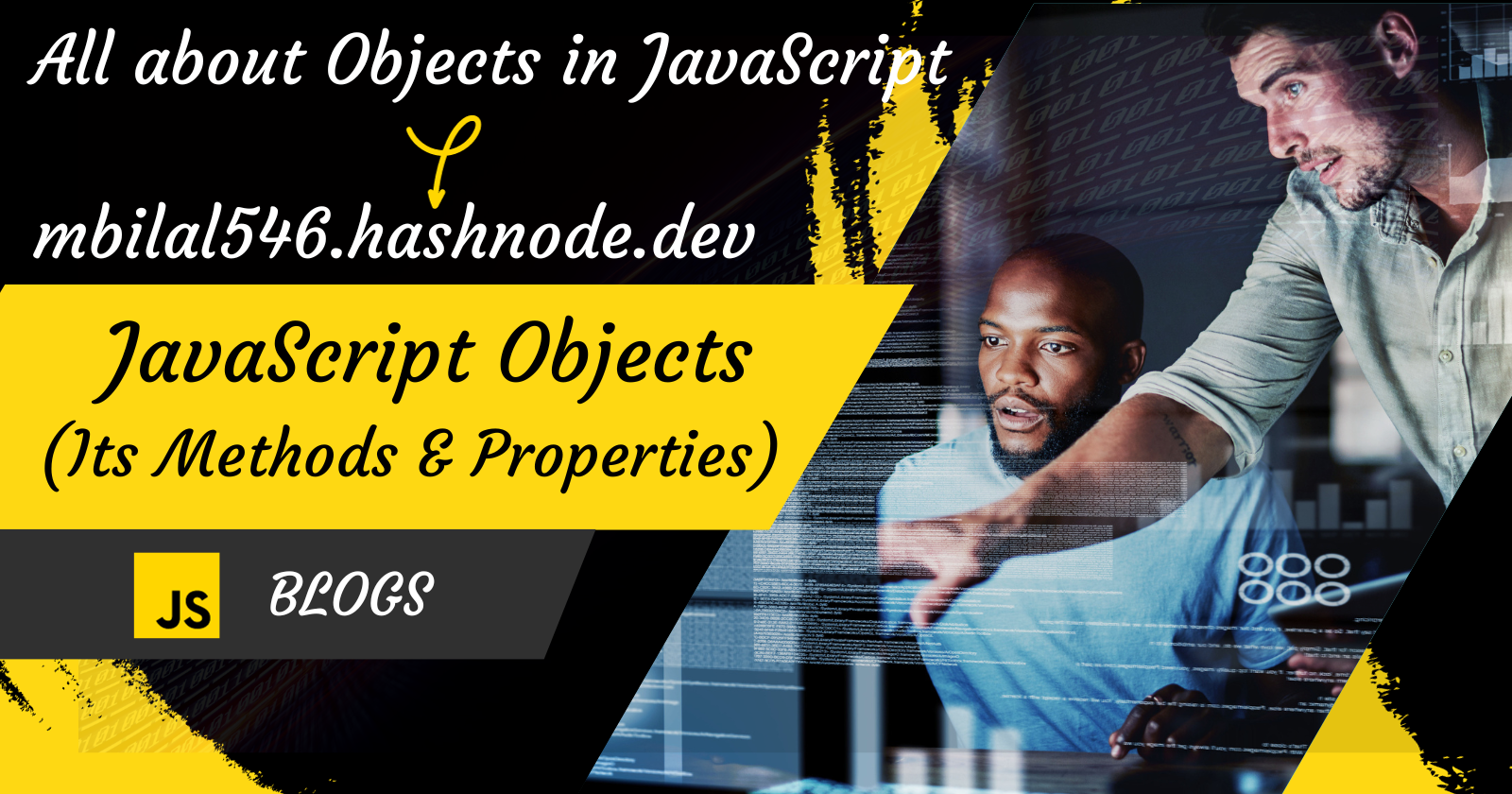
What is an object in JavaScript?
In JavaScript, objects consist of key-value pairs, where keys are strings (or symbols) and values can be any data type, including other objects. The keys are also referred to as properties, and the values associated with them can be of various types, such as numbers, strings, functions, arrays, or even other objects. An object is a complex data type that allows you to store and organize data. Objects are a fundamental part of the language and are used to represent real-world entities, concepts, or structures. They are instances of the Object type, which is one of the primitive data types in JavaScript.
There are two ways of creating an object. Through the object literal and the constructor method.
Object Literals:
Through object literals, the object is created with multiple instances.
For Example:
// Let's create an object of a person through object literals.
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(person);
Output: { firstName: 'Muhammad', lastName: 'Bilal', age: 21 }
In the above example, the firstName
, lastName
, and age
are the keys and Muhammad
, Bilal
, and 21
are the values of the object person. The values are kept behind in JavaScript as a string
.
The second way of using the object literal is:
// The second way is
let person2 = {}; // This create an empty object
person2.firstName = "Muhammad";
person2.lastName = "Bilal";
person2.age = 21;
console.log(person2);
Output: { firstName: 'Muhammad', lastName: 'Bilal', age: 21 }
Both have the same results.
Constructor Method:
Through the constructor method, the singleton object is created. It is the only object of its kind. It has not had multiple instances.
For Example:
const user = new Object(); // it is the singleton object.
user.firstName = "Muhammad";
user.lastName = "Bilal";
user.age = 21;
console.log(user);
Output: { firstName: 'Muhammad', lastName: 'Bilal', age: 21 }
Note:
This question is important from the interview perspective, and we will continue our object blog with template literals.
Accessing properties of the object:
There are two ways to access the properties of objects.
1. Dot Notation
The first way to access the properties of objects is dot notation
.
For Example:
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(person.lastName);
Output: Bilal
console.log(person.age);
Output: 21
2. Square Brackets
The second way to access the properties of objects is square bracket
.
For Example:
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(person[lastName]);
// If you access like this you will get an error
Output: lastName is not defined.
// Because the values are kept behind in JavaScript as a string.
// So, you have to access like a string.
console.log(person["lastName"]);
Output: Bilal
Let's see an example by creating the symbol, that is why square brackets are necessary.
let mySymbol = Symbol("mySymbol"); // Create a symbol
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
mySymbol: "JsBlogs!", // Here mySymbol is considered as a string.
}
console.log(person);
Output:
{ firstName: 'Muhammad', lastName: 'Bilal', age: 21, mySymbol: 'JsBlogs!' }
// Here mySymbol in object peson is considered as a string.
When we write mySymbol
in square brackets in a person object then it will be considered as Symbol
and can only be accessed by square brackets.
let mySymbol = Symbol("mySymbol");
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
[mySymbol]: "JsBlogs!", // Here it is a symbol you can see in result.
}
console.log(person);
Output:
{ firstName: 'Muhammad', lastName: 'Bilal', age: 21, [Symbol(mySymbol)]: 'JsBlogs!' }
// Now if you want to access symbol woth dot notation, it is not possible
console.log(person.mySymbol);
Output: undefined
// It can only be accessed by square brackets.
console.log(person[mySymbol]);
Output: JsBlogs!
Updating / Overwriting the object value
You can update and overwrite the pre-assigned value of an object.
For Example:
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(person);
Output: { firstName: 'Muhammad', lastName: 'Bilal', age: 21 }
// If you want to update / overwrite firstName then
person.firstName = "Mr";
console.log(person);
Output: { firstName: 'Mr', lastName: 'Bilal', age: 21 }
Object.freeze()
If you don't want to overwrite or update the value of your object then you can freeze that object by using Object.freeze()
method.
For Example:
let person = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(person);
Output: { firstName: 'Muhammad', lastName: 'Bilal', age: 21 }
Object.freeze(person); // It will freeze your object
person.firstName = "Mr"; // After freezing the object this will not work.
console.log(person);
Output: { firstName: 'Muhammad', lastName: 'Bilal', age: 21 }
Let's create a function in an object
In JavaScript, objects can have properties and methods. Methods are functions that are associated with an object. When a function is a property of an object, it's known as a method.
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
myFunc: function() { // This is here known as method
console.log("This is the JavaScript Object Blog!");
}
}
console.log(user.myFunc);
Output: [Function: myFunc]
// If you want to access it then you need to call the function
console.log(user.myFunc());
Output: This is the JavaScript Object Blog!
You can write a function in an object directly with its name to make it shorter.
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
myFunc() { // This is short form of method, it will give same output.
console.log("This is the JavaScript Object Blog!");
}
}
console.log(user.myFunc());
Output: This is the JavaScript Object Blog!
If you want to refer firstName
and lastName
of an object into the function then it is only possible by using this
keyword.
Let's see with an example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
myFunc() { // This is short form of method
console.log(`Hello User, ${this.firstName} ${this.lastName}`);
}
}
console.log(user.myFunc());
Output: Hello User, Muhammad Bilal
// If you try to access without this kayword it will give an error
ReferenceError: firstName is not defined, lastName is not defined.
Example of calculator with object and method
In this example, the first two methods are in short form and the last two are simple.
const calculator = {
add(a, b) { // This is short form of method
return a + b;
},
subtract(a, b) { // This is short form of method
return a - b;
},
multiply: function(a, b) {
return a * b;
},
divide: function(a, b) {
return a / b;
},
};
console.log(calculator.add(5, 3));
Output: 8
console.log(calculator.subtract(5, 3));
Output: 2
console.log(calculator.multiply(5, 3));
Output: 15
console.log(calculator.divide(6, 3));
Output: 2
Array in an object
In JavaScript, you can create an object that contains an array as one of its properties.
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
hobbies: ["Badminton", "Watching Movies", "Travelling"],
}
console.log(user.hobbies);
Output: [ 'Badminton', 'Watching Movies', 'Travelling' ]
// If you want access hobby index then access it through array indexes
console.log(user.hobbies[0]);
Output: Badminton
Object in an object (Nested object)
In JavaScript, a nested object refers to an object that is a property of another object. This allows you to create a hierarchy of objects, where each nested object can have its properties and methods.
For Example:
let user = {
fullName: {
userFullName: {
firstName: "Muhammad",
lastName: "Bilal",
}
},
age: 21,
}
console.log(user.);
Output: {
fullName: { userFullName: { firstName: 'Muhammad', lastName: 'Bilal' } },
age: 21
}
// let's access the lastName from the object user step by step
// Step 1
console.log(user.fullName);
Output: { userFullName: { firstName: 'Muhammad', lastName: 'Bilal' } }
// Step 2
console.log(user.fullName.userFullName);
Output: { firstName: 'Muhammad', lastName: 'Bilal' }
// Step 3
console.log(user.fullName.userFullName.lastName);
Output: Bilal // You can access name like this in nested objects.
Combining two objects
There is a method for combining two objects but if you try like this
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const obj3 = {obj1, obj2};
console.log(obj3);
// this will combine two object not their values in single object
Output: { obj1: { a: 1, b: 2 }, obj2: { b: 3, c: 4 } }
There is an object method for combining them.
Object.assign()
Object.assign()
is a method in JavaScript that is used to copy the values of all enumerable properties from one or more source objects to a target object. It returns the target object.
For Example:
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const obj3 = Object.assign(obj1, obj2);
// this will copy obj2 value and merge into obj1
console.log(obj3);
Output: { a: 1, b: 3, c: 4 }
// Now if you check obj1 and obj3, they are equal
console.log(obj1 === obj3);
Output: true
There is an optional parameter like an empty object {}
. Providing it at the start is a good practice. It means Object.assign()
the method copies values from obj1 and obj2 and then puts them into the empty object.
For Example:
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const obj3 = Object.assign({}, obj1, obj2); // This is good practce.
// this will copy obj1 and obj2 values and puts into empty object.
console.log(obj3);
Output: { a: 1, b: 3, c: 4 }
// Now if you check obj1 and obj3, they are not equal
console.log(obj1 === obj3);
Output: false
Spread Operator
In JavaScript, the spread operator (...
) is a powerful tool that allows you to expand elements of an object's properties. When it comes to combining two objects, you can use the spread operator to create a new object with the properties of both objects. This is the most used operator nowadays instead of Object.assign()
method.
For Example:
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const obj3 = {...obj1, ...obj2};
// this will copy obj1 and obj2 values and puts into obj3.
console.log(obj3);
Outout: { a: 1, b: 3, c: 4 }
// You can merge more than two values also
const obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
const obj3 = { e: 5, f: 6 };
const obj4 = { g: 7, h: 8 };
const obj5 = {...obj1, ...obj2, ...obj3, ...obj4};
console.log(obj5);
Outout: { a: 1, b: 2, c: 3, d: 4, e: 5, f: 6, g: 7, h: 8 }
Some other Methods:
Object.keys()
Object.keys()
is used to return an array of a given object's enumerable property names, in the same order as we get with a normal loop.
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
let keys = Object.keys(user)
console.log(keys);
Output: [ 'firstName', 'lastName', 'age' ]
Object.values()
In JavaScript, the Object.values()
method is a built-in method that was introduced with ECMAScript 2017 (ES8). It is used to return an array containing the values of an object's enumerable property values.
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
let values = Object.values(user)
console.log(values);
Output: [ 'Muhammad', 'Bilal', 21 ]
Object.entries()
In JavaScript, the Object.entries()
method is used to return an array of a given object's enumerable property [key, value]
pairs.
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
let entry = Object.entries(user);
console.log(entry);
Output:
[ [ 'firstName', 'Muhammad' ], [ 'lastName', 'Bilal' ], [ 'age', 21 ] ]
object.hasOwnProperty()
In JavaScript, the hasOwnProperty()
method is used to check whether an object has a specific property as its property. It returns a boolean value (true
or false
).
For Example:
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(user.hasOwnProperty("lastName"));
Output: true
// If property is no present in an object
console.log(user.hasOwnProperty("email"));
Output: false
object.propertyIsEnumerable()
In JavaScript, the propertyIsEnumerable
method is used to check if a particular property of an object is enumerable. An enumerable property will be included during the iteration of the object's properties, such as in a for...in
loop.
For Example:
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
}
console.log(user.propertyIsEnumerable("firstName"));
Output: true
// Check if 'toString' (an inherited property) is enumerable
console.log(user.propertyIsEnumerable("toString"));
Output: false
Destructuring of Objects in JavaScript
Object destructuring is a feature in JavaScript that allows you to extract values from objects and assign them to variables more concisely and conveniently. It provides a shorter syntax for extracting values from objects.
Without destructuring, you are going to extract the values from an object using dot notation.
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
address: {
city: "myCity",
zipCode: "12345",
}
}
// Without destructuring when you are going to extract values,
// You will extract like this every time when you need.
console.log(user.firstName);
console.log(user.lastName);
console.log(user.age);
console.log(user.address.city);
console.log(user.address.zipCode);
Output:
Muhammad
Bilal
21
myCity
12345
With the concept of destructuring, it is easy, but it will use too much while working in react. Let's see an example of a destructuring object.
let user = {
firstName: "Muhammad",
lastName: "Bilal",
age: 21,
address: {
city: "myCity",
zipCode: "12345",
}
}
let {} = user;
// Now the object user is destructured. Now the property you want to access.
// put in the curly braces
let {firstName, lastName, age} = user;
console.log(firstName);
Output: Muhammad
console.log(lastName);
Output: Bilal
console.log(age);
Output: 21
If you want to access nested objects, then
let {address: { city, zipCode }} = user;
console.log(city);
Output: mtCity
console.log(zipCode);
Output: 12345
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
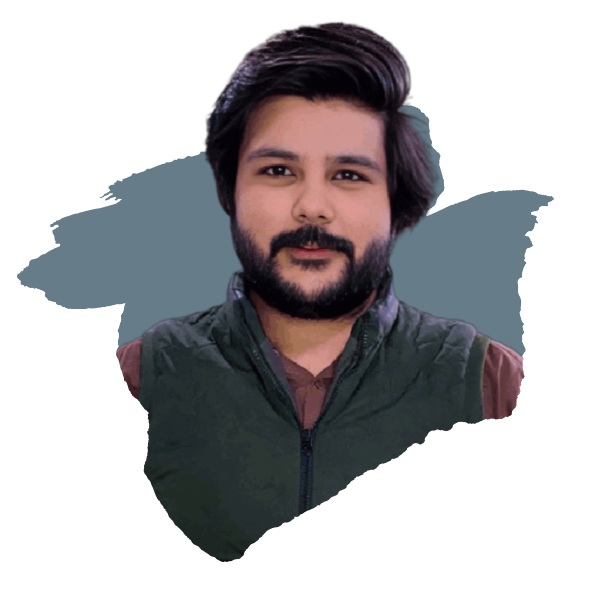
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. 📈 Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. 🔊 Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub 🖥 Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. ✨ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. 🎓 Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. 👥 Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.