Ever Wondered How JavaScript Handles Memory?๐ค
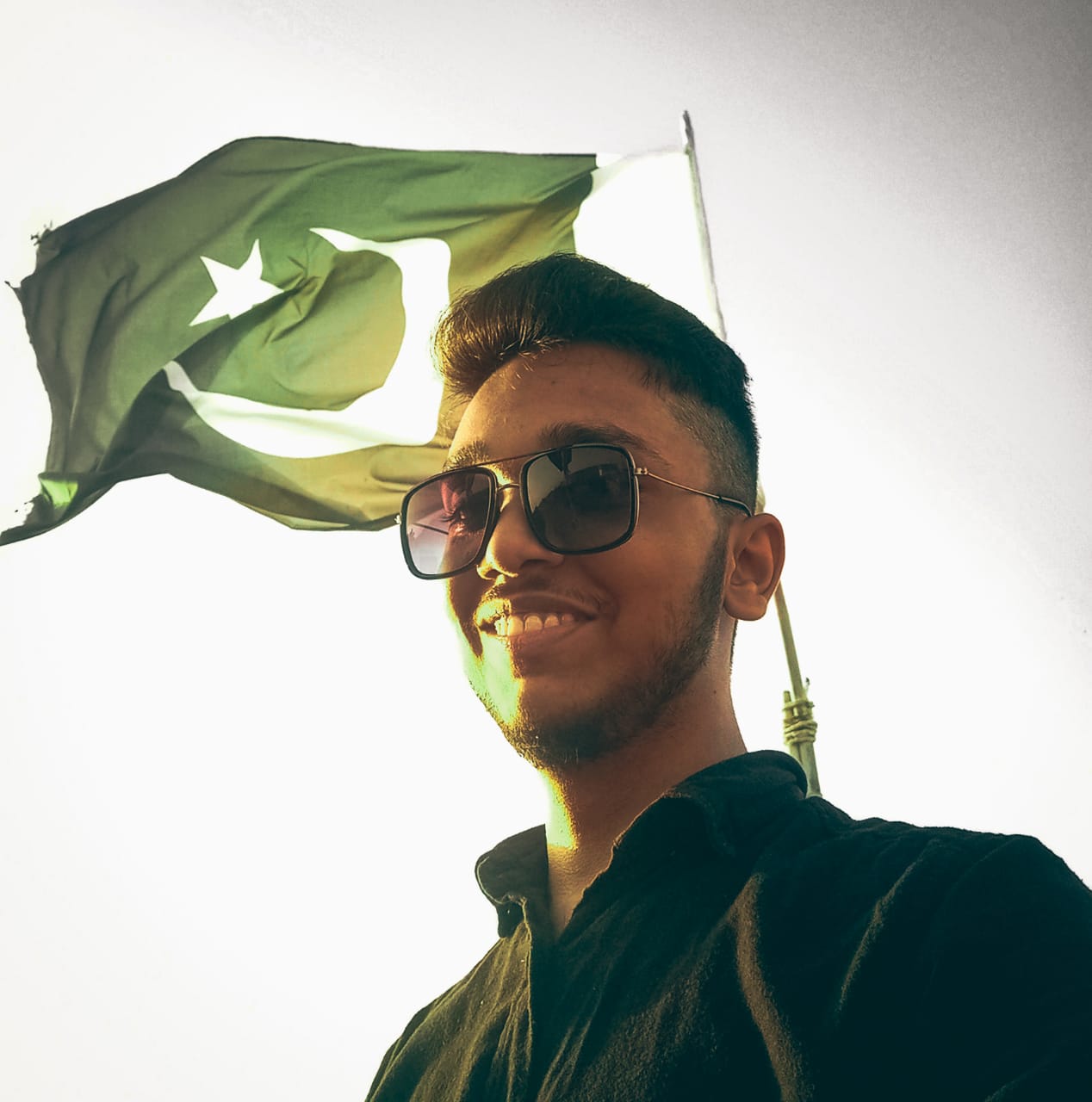
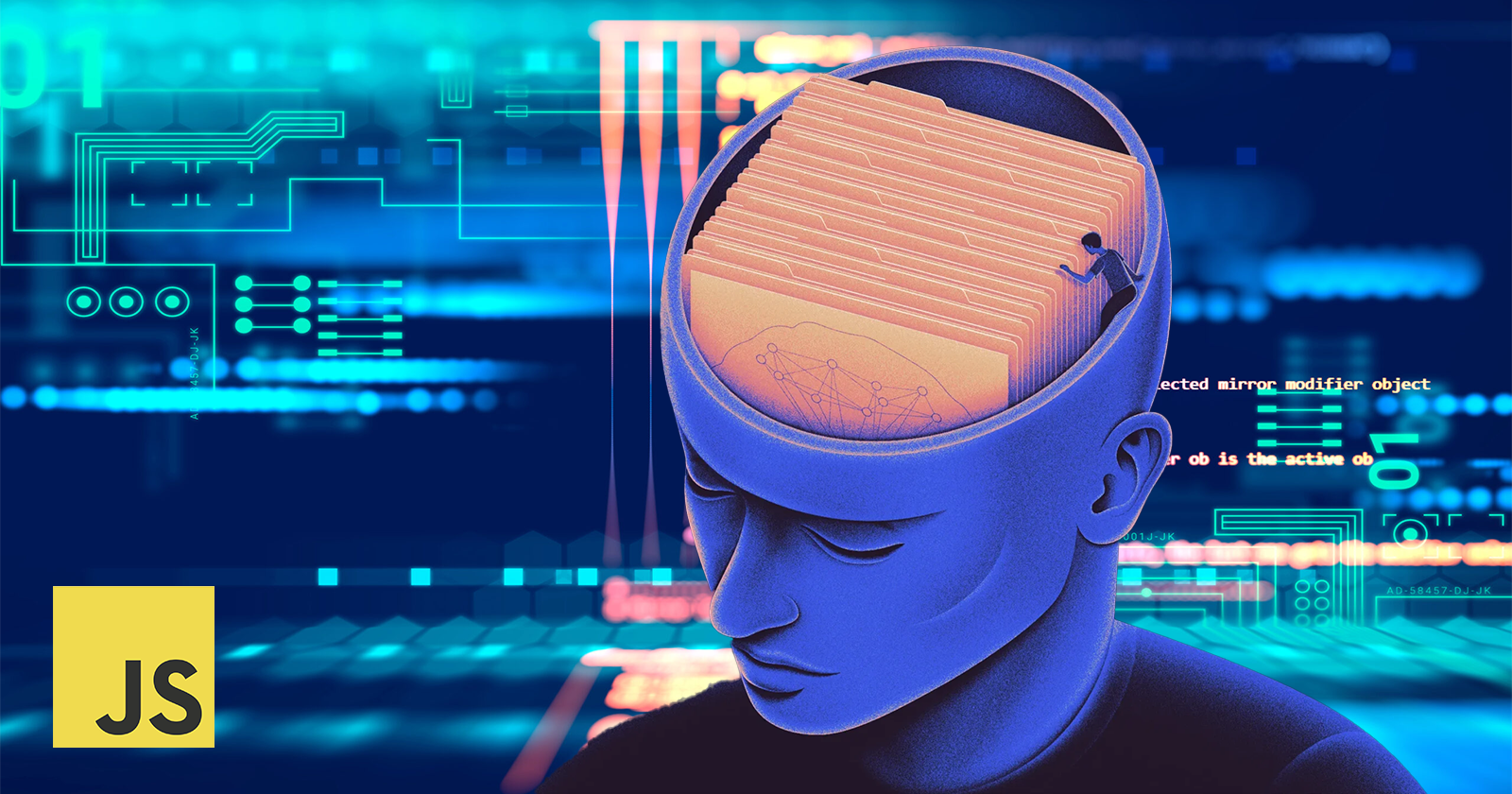
Hey, fellow devs! ๐ Today, let's embark on a memory magic carpet ride, unravelling the mystery of Stack and Heap memory in JavaScript. Now, you might wonder, "Why should I care about memory?" Well, sit tight because understanding this unlocks secret coding powers! ๐งโโ๏ธ
When I started coding, grasping these ideas felt like wrangling quantum physics theories. ๐คฏ But after learning the hard way, I realized how critical having this foundation is. So in this guide, we'll simplify heap vs stack in JavaScript with some wacky analogies, examples, and even a cautionary tale of the time I took down production. After reading, you'll have mastered a powerful skill to analyze code for performance wins. Let's get started!
The Stack: Your Code's Short-Term Memory
Imagine your code as a stack of pancakes. Each function call adds a pancake, and when the function is done, we remove the top pancake. Voila! That's stack memoryโneat, organized, and like a magical pancake tower.
The stack stores temporary variables created by each function. It's self-contained and local.
Key Takeaway: Stack memory is like building a tower of pancakes; add, flip, remove!
function multiply(x, y) {
let result = x * y; // stored on the stack!
return result;
}
multiply(2, 3) // -> Returns 6
// stack frame cleared!
Any variables created inside multiply() get popped off when it finishes running, like clearing off desk papers once your work is done (hopefully into the recycling bin!). Stacks are self-cleaning - no need to manually allocate or deallocate memory. But they only last as long as the function's scope.
Stack Values:
Primitive literals: numbers, booleans, strings
Primitive variables: let, const declarations
References to heap objects
The Heap: Your Code's Long-Term Memory
Now, let's stroll into the heap memory playground. Picture a vast field where you can set up tents (store data) dynamically. It's like a coding campsite with endless possibilities, Capable of storing data in the form of long-term memory.
Key Takeaway: Heap memory is your dynamic campsiteโpitch tents (allocate memory) as needed!
In JavaScript, primitives like strings get stored on the stack. But complex objects and arrays get allocated on the heap:
let colorPalette = ['red', 'blue'] // stored on the heap
function printColors() {
console.log(colorPalette)
}
printColors() // Palette persists!
The heap stays around letting the colour palette be used across contexts.
You do have to manually free up heap memory though when finished. Otherwise, objects hang around hogging resources - we call this a "leak". Not fun!
Heap Values:
Object literals {}
Array literals []
Class instances
Closures
STACK VS HEAP
Stack | Heap | |
Storage Type | Temporarily stores primitive types & references. | Stores complex objects like arrays or objects. |
Size | Small & Fixed Size per Execution Context. | Can grow dynamically as needed. |
Lifetime | As long as the Execution Context is active. | Can persist across different Execution Contexts. |
Access | Very Fast | Relatively Slower lookup speed |
Management | RAM automatically allocates and frees up stack space | The programmer manually allocates and frees heap space with new / delete |
Use Case | Great for creating temporary single-use variables or primitives with small scope | Well suited for objects used across multiple contexts or functions |
Advantages of Stack:
Allocates/deallocates automatically & efficiently with limited programmer control
Very fast access relative to heap
Advantages of Heap:
Key engine for dynamic memory allocation with the ability to scale as needed
Supports longer-lived objects for global data persistence
Why This Should Even Matter to You
I used to get puzzled by memory concepts. But fear not, my fellow wizards! Understanding memory in JavaScript unleashes your true coding powers.
Why care?
Memory Efficiency: Optimize for faster, responsive JavaScript spells.
Debugging Magic: Easily spot and banish memory-related gremlins.
Performance Enchantment: Craft code that dances to your command!
Interviews: Impress recruiters by elegantly discussing memory management
As our whirlwind tour of heap and stack magic draws to an end, you may be wondering, was it all worth it?
Walking away, I hope your mind is already spinning with ideas on how to optimize and secure your next web app through the lens of efficient allocation. Perhaps you've uncovered a budding excitement within to learn other seemingly dry concepts too - one click at a time transforming into mastery. โจ
Just like Gandalf refined his wizarding prowess over centuries, we too are always in training. So stay curious, my coding fellows! With consistent practice, even the most complex incantations will begin flowing from your fingertips. Now go unleash your new skills out into the world - and let the memory magic continue! ๐งโโ๏ธ.
Subscribe to my newsletter
Read articles from Muhammad Wasif Malik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
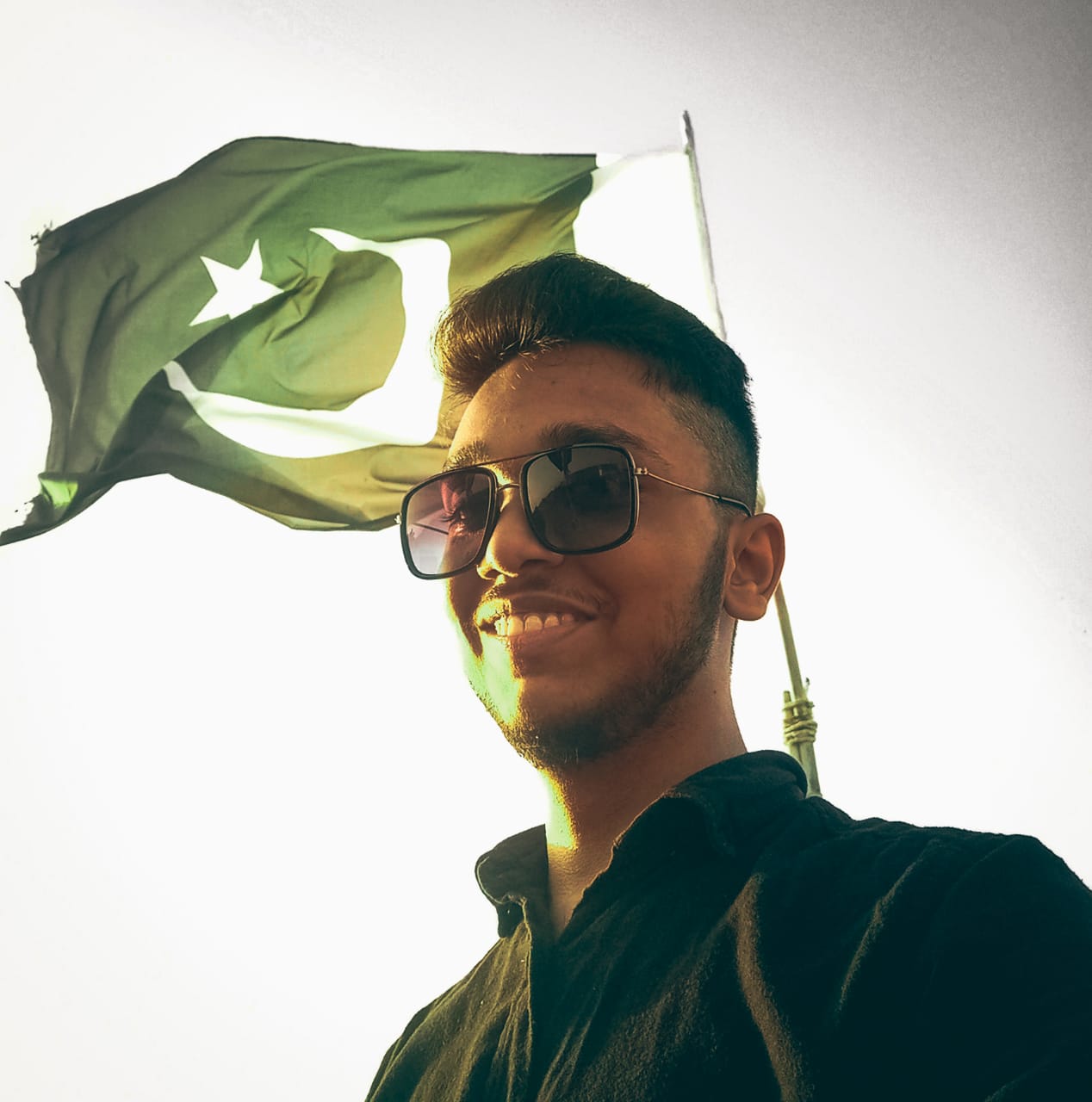
Muhammad Wasif Malik
Muhammad Wasif Malik
Hello World ๐, I'm Wasif (aka wosmo)! I'm a 3rd-year Software Engineering ๐จโ๐ป student with a passion for building captivating user experiences through code. My superpower so far has been front-end web development with React and Next.jsโ๏ธ, As I level up my skills, I'm now venturing into the exciting world of mobile app development ๐ฑ with React Native. I find it empowering to make people's lives easier through intuitive app UIs that delight. When I'm not coding, you'll find me unwinding with video editing ๐ฅ or Photoshop ๐๏ธ - I love getting creative! I also enjoy working out ๐๏ธโโ๏ธ, reading sci-fi novels ๐, and gazing at the stars ๐ since I'm fascinated by astronomy ๐ญ. Feel free to reach out if you'd like to code, chat, or even grab a chai!โ