Object Oriented Programming in Python (PART-2)
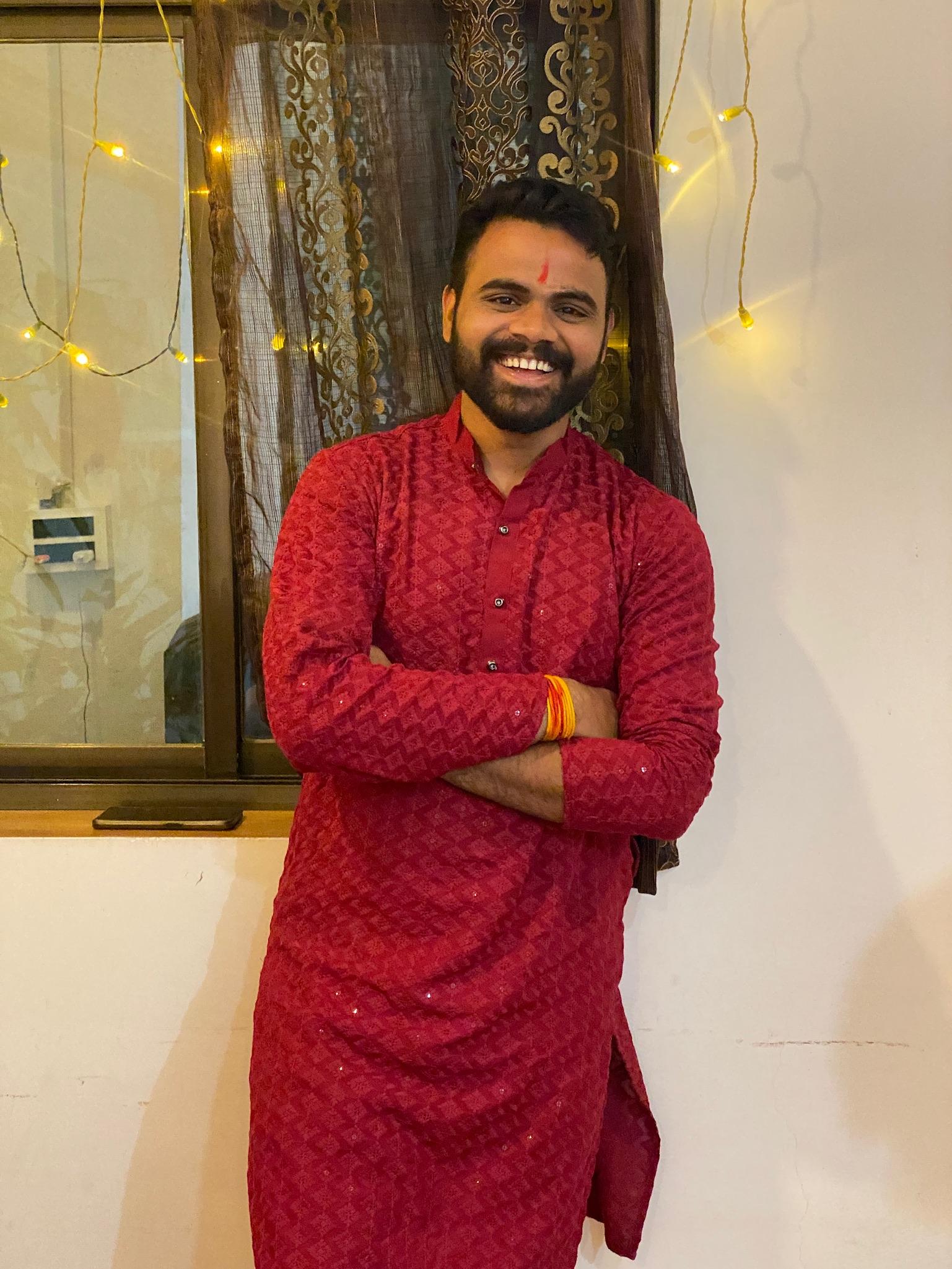
Table of contents
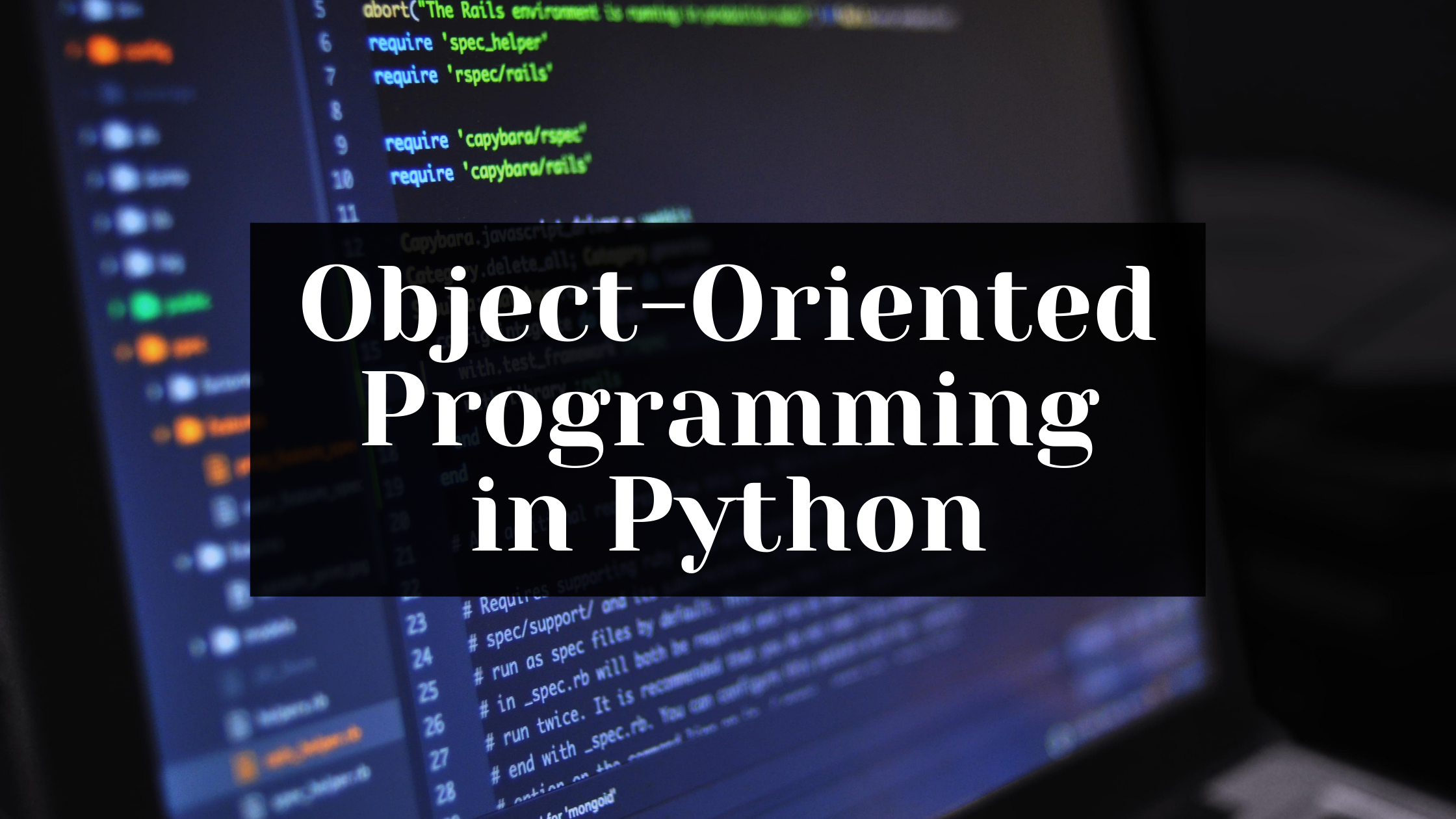
What is Self?
A self is a reference variable that points to the current class object.
When we instantiate an object, it automatically passes to the default parameter(self).
class test: #class definition
def m1(self):
print(self)
t1=test() #object creation
print(t1)
t1.m1() #method Calling
t2=test() #object creation
print(t2)
t2.m1() #method Calling
The Output of the above code is:
By seeing the diagrams below, we can clearly understand the self.
The first argument to the constructor and instance method should be self.
Python virtual Environment is responsible for providing value for self argument and we are not required to provide expitictely
By using self we can declare instance variables
By using self we can access instance variables
Instead of self, we can use any name ,,, but it is recommended to use self
Variables in OOPs
In Object oriented mechanism variables can be categorized into 3-types, they are,
Static/class variable
non-static/instance variable
local/method variable
1)Static variable
We can define a variable within the class and above the method/constructor definitions, that type of variable is called a static variable
The data that is common for all the objects of a class, that type of data represented as a static variable
The static variables allocate the memory only once
We can access the static variables within the methods by using the class name
We can access the static variables from outside the class by using the class name or reference variable
Example:-
class student:
col_name = "SVVV"
col_add = "Indore"
def col_info(self):
print(student.col_name)
print(student.col_add)
s1=student() #Object Creation
s1.col_info() #method calling
print(student.col_name)
print(student.col_add)
print(s1.col_name)
print(s1.col_add)
Here is the output of the above code:
2)Non-static variable
We can define a variable within the method/constructor with the help of the default parameter name(self), that type of variable is called a non-static variable.
The data which is changing from one object to another object, that type of data we are represented as non-static variables
The non-static variables memory allocation depends on the number of objects we are created
1 object ------> 1-time memory allocation
2 object ------> 2-time memory allocation
3 object ------> 3-time memory allocation
n object ------> n times memory allocation
We can access the non-static variables within the methods by using the default parameter(self)
We can access the non-static variables from outside the class by using reference variables only.
Example:-
class student:
def std_info(self):
self.sid = 101
self.sname = "Kunal"
print(self.sid)
print(self.sname)
s1=student()
s1.std_info()
print(s1.sid)
print(s1.sname)
Here is the Output of the above code:
3) Local variable
We can define a variable within the method directly that type of variable is called a local variable.
We can access the local variable data within that particular method only
Example:-
class test:
def m1(self):
self.x=10
y=20
print(self.x)
print(y)
def m2(self):
print(self.x)
print(y)
t1=test() #object creation
t1.m1() #method calling
t1.m2() #method calling
Here is the Output of the above code
Example of static and nonstatic in one Program
class student:
col_name="SVVV" #static variable
col_add="Indore" #static variable
def std_info(self):
self.sid=101 #non-static variable
self.sname="Kunal" #non-static variable
print(self.sid)
print(self.sname)
print(student.col_name)
print(student.col_add)
s1=student()
s1.std_info()
Here is the output of the above code
Example 2 Static and nonstatic in one Program
class student:
col_name="SVVV" #static variable
col_add="Indore" #static variable
def std_info(self,sid,sname):
self.sid=sid #non-static variable
self.sname=sname #non-static variable
print(self.sid)
print(self.sname)
print(student.col_name)
print(student.col_add)
s1=student()
s1.std_info(101,"Kunal")
s2=student()
s2.std_info(102,"Shivam")
Here is the output of the above code
Note:
Whenever we define the non-static variables within the method, in case, the non-static variable memory allocation depends on no.of times we are calling a method, this is a problem (to consume more memory)
Let's understand this by an example:-
class test:
x=[1,2,3]
def m1(self):
self.y=[4,5,6]
print("This is X address in memory ",id(test.x))
print("This is Y address in memory ",id(self.y))
t1=test()
t1.m1()
t1.m1()
t1.m1()
t2=test()
t2.m1()
t2.m1()
Here is the output of the above code
now, let's understand the same program by using the constructor
class test:
x=[1,2,3]
def __init__(self):
self.y=[4,5,6]
def m1(self):
print("This is X address in memory ",id(test.x))
print("This is Y address in memory ",id(self.y))
t1=test()
t1.m1()
t1.m1()
t1.m1()
t2=test()
t2.m1()
t2.m1()
Here is the Output of the above code
Stay tuned for more insights into leveraging Python's OOP principles to build efficient and scalable application.
Subscribe to my newsletter
Read articles from Kunal Joshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
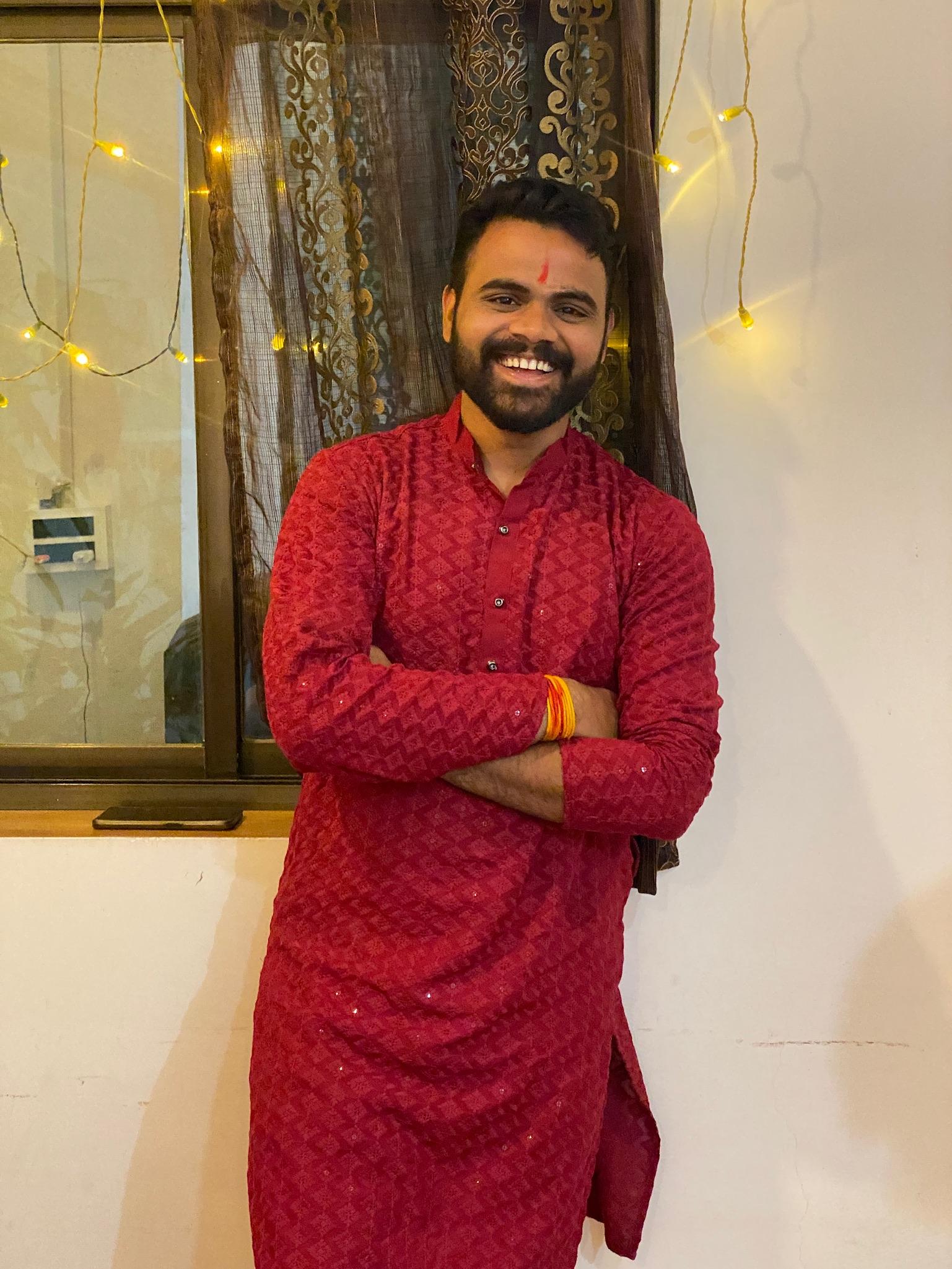