Unleashing Speed: A Guide to Asynchronous Programming in API Queries
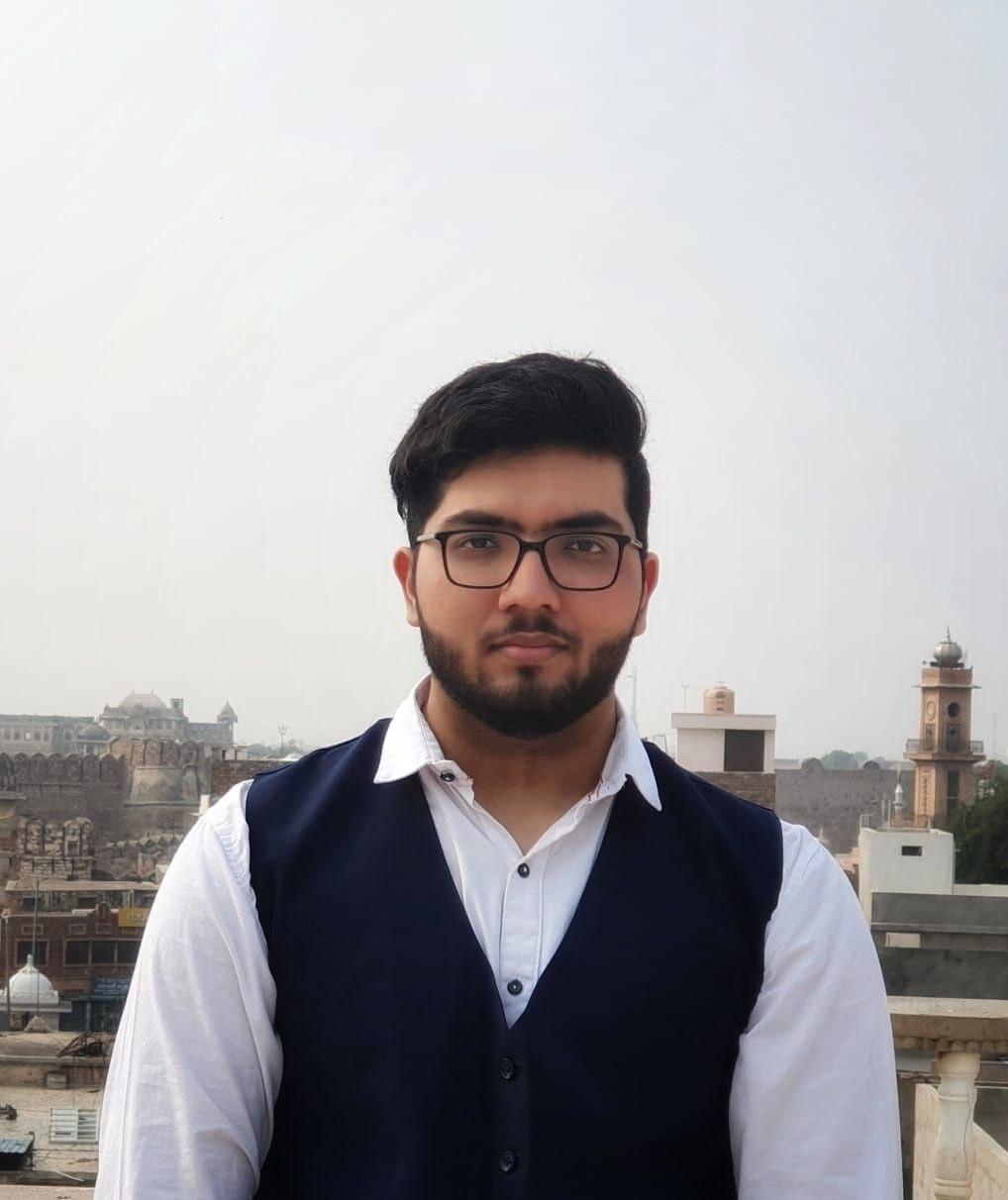
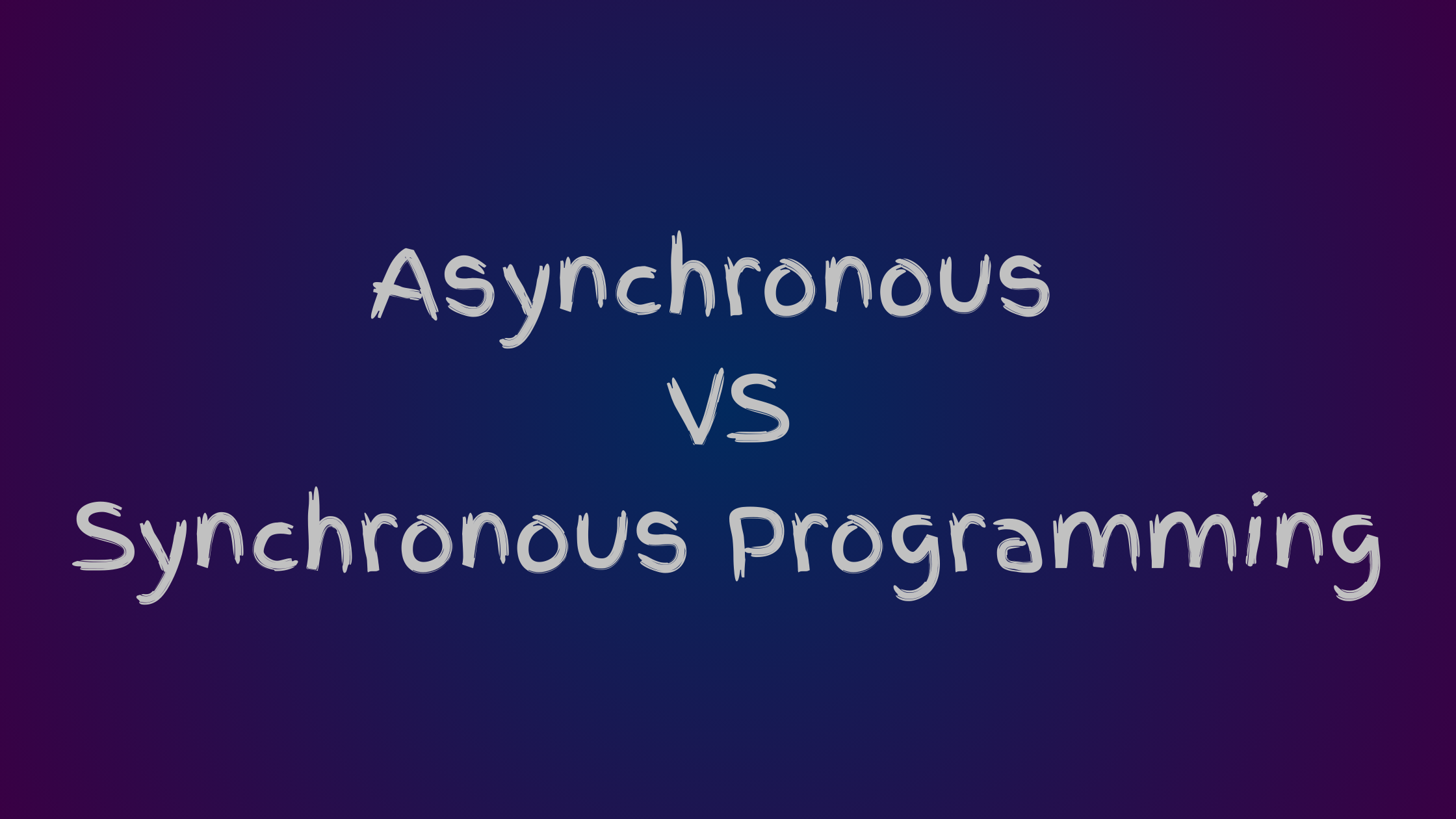
In the realm of software development, optimizing API interactions for speed is paramount. Asynchronous programming emerges as a game-changer in accelerating API queries. Explore the concepts and implementation of asynchronous programming to significantly enhance the pace of your API interactions.
Understanding Asynchronous Programming
Traditional synchronous programming follows a sequential path. When a request is made, the program waits for it to complete before moving on to the next task. asynchronous programming allows tasks to run independently without waiting for each other to finish. This parallel execution enables optimization and reduces idle time.
The Need for Speed in API Queries
When interacting with APIs, especially in scenarios where multiple requests are made sequentially, the process can be time-consuming. Each request typically involves waiting for a response before sending the next one. Asynchronous programming enters the scene to revolutionize this pace.
How Asynchronous Programming Accelerates API Queries
By leveraging asynchronous programming, you can initiate multiple API requests concurrently. Instead of waiting for each request to complete before starting the next, the program can continue with other tasks while waiting for responses. This concurrent execution significantly reduces the overall time required to fetch data from APIs.
Implementing Asynchronous Programming in Python
Python offers efficient tools for asynchronous programming, such as the asyncio
library. Below is an example demonstrating asynchronous querying of multiple APIs using asyncio
and aiohttp
:
import asyncio
import aiohttp
async def fetch_data(session, url):
async with session.get(url) as response:
return await response.json()
async def fetch_all_data(urls):
async with aiohttp.ClientSession() as session:
tasks = [fetch_data(session, url) for url in urls]
return await asyncio.gather(*tasks)
# Example usage
urls_to_query = [ 'https://jsonplaceholder.typicode.com/posts/1', 'https://jsonplaceholder.typicode.com/posts/2']
result = asyncio.run(fetch_all_data(urls_to_query))
print(result)
Best Practices and Considerations
While asynchronous programming offers remarkable speed improvements, it's essential to consider a few best practices:
Understand the APIs: Some APIs may limit the number of concurrent requests or have rate limits. Adhere to these restrictions.
Proper error handling: Asynchronous code can make error handling more complex. Implement robust error handling to ensure graceful failure.
Balance between concurrency and resources: While increasing concurrency speeds up processing, it might consume more system resources. Find an optimal balance.
Comparing Sync and Async Queries
We can write a simple Python program to see which of the two works faster. This speed difference is what makes async queries so special and useful.
import requests
import asyncio
import time
import aiohttp
# Synchronous fetching of data
def synchronous_fetch(urls):
start_time = time.time()
for url in urls:
response = requests.get(url)
# Simulate processing data (in this case, just printing the length of response)
print(f"URL: {url}, Length: {len(response.content)}")
end_time = time.time()
return end_time - start_time
# Asynchronous fetching of data using asyncio
async def asynchronous_fetch(urls):
async def fetch_data(session, url):
async with session.get(url) as response:
return await response.read()
start_time = time.time()
async with aiohttp.ClientSession() as session:
tasks = [fetch_data(session, url) for url in urls]
await asyncio.gather(*tasks)
end_time = time.time()
return end_time - start_time
# URLs to fetch (example URLs, replace with actual ones)
urls_to_fetch = [
'https://jsonplaceholder.typicode.com/posts/1',
'https://jsonplaceholder.typicode.com/posts/2',
'https://jsonplaceholder.typicode.com/posts/3',
# Add more URLs if needed
]
# Synchronous fetching and measure time
sync_time_taken = synchronous_fetch(urls_to_fetch)
print(f"Synchronous fetch took {sync_time_taken:.4f} seconds")
# Asynchronous fetching and measure time
async_time_taken = asyncio.run(asynchronous_fetch(urls_to_fetch))
print(f"Asynchronous fetch took {async_time_taken:.4f} seconds")
Upon running this code we see the following output:
Which is 3 times faster than synchronous query!
Conclusion
Asynchronous programming in API queries is a potent tool for software developers aiming to enhance performance. By allowing concurrent execution of tasks, it transforms the speed at which data is fetched from APIs. With Python's asyncio
and related libraries, you can unlock the full potential of asynchronous programming and elevate your application's performance to new heights.
Subscribe to my newsletter
Read articles from Osaf Ali Sayed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
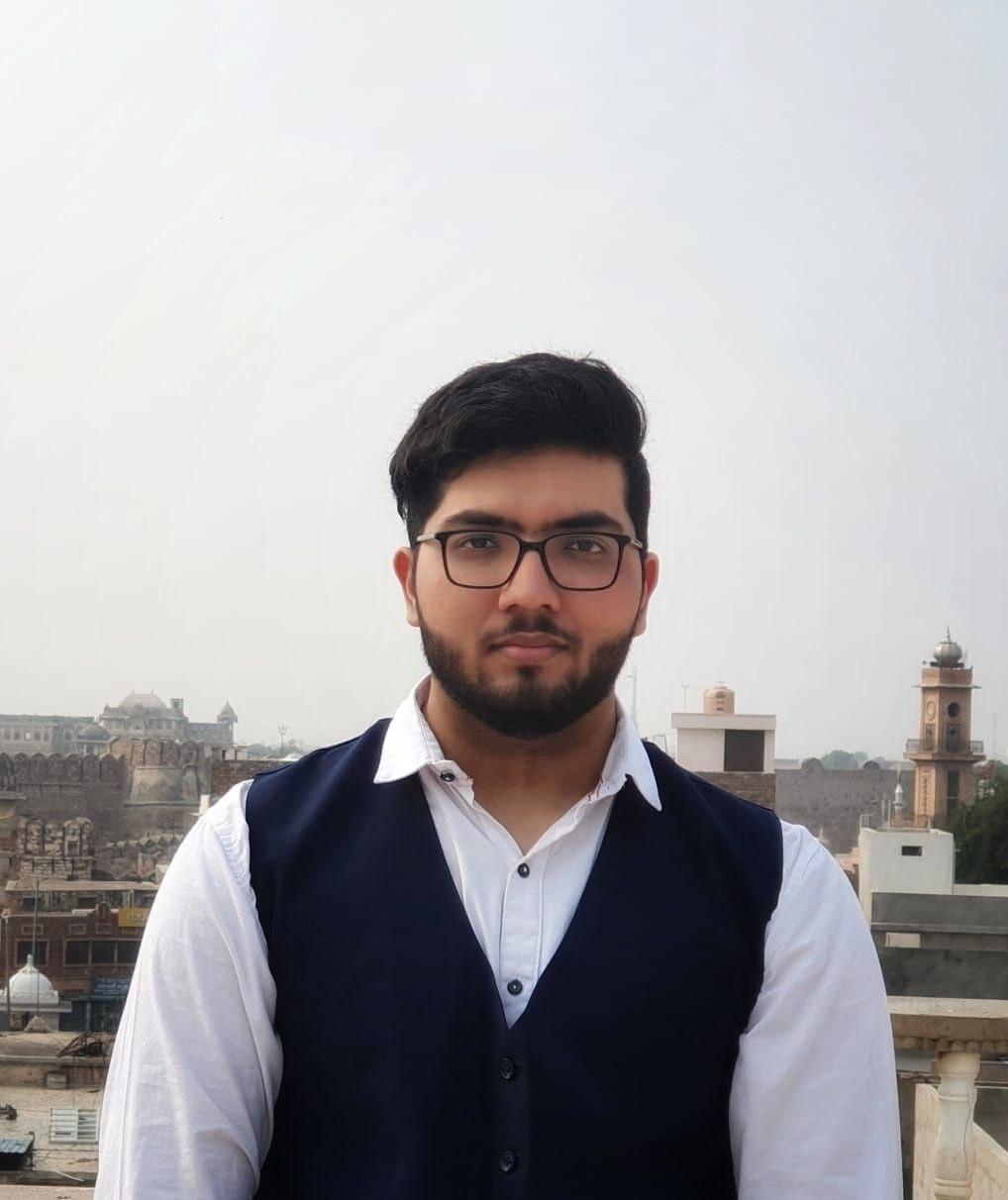
Osaf Ali Sayed
Osaf Ali Sayed
I am a Full stack developer with 2 years of experience in freelancing. I have worked on Frontend (HTML, CSS, Bootstrap, JavaScript, ReactJs) and Backend technologies (Python, Django, Flask, SQL) for almost two years and had done several projects. I am also skillful with Java in Data Structures and Algorithms. Aspiring to a challenging career in software development to use my learned skills and experience for the best results. I love to teach people and pass on my programming knowledge to others in the mean time.